Master Sorting HashMaps by Value in Java 8!
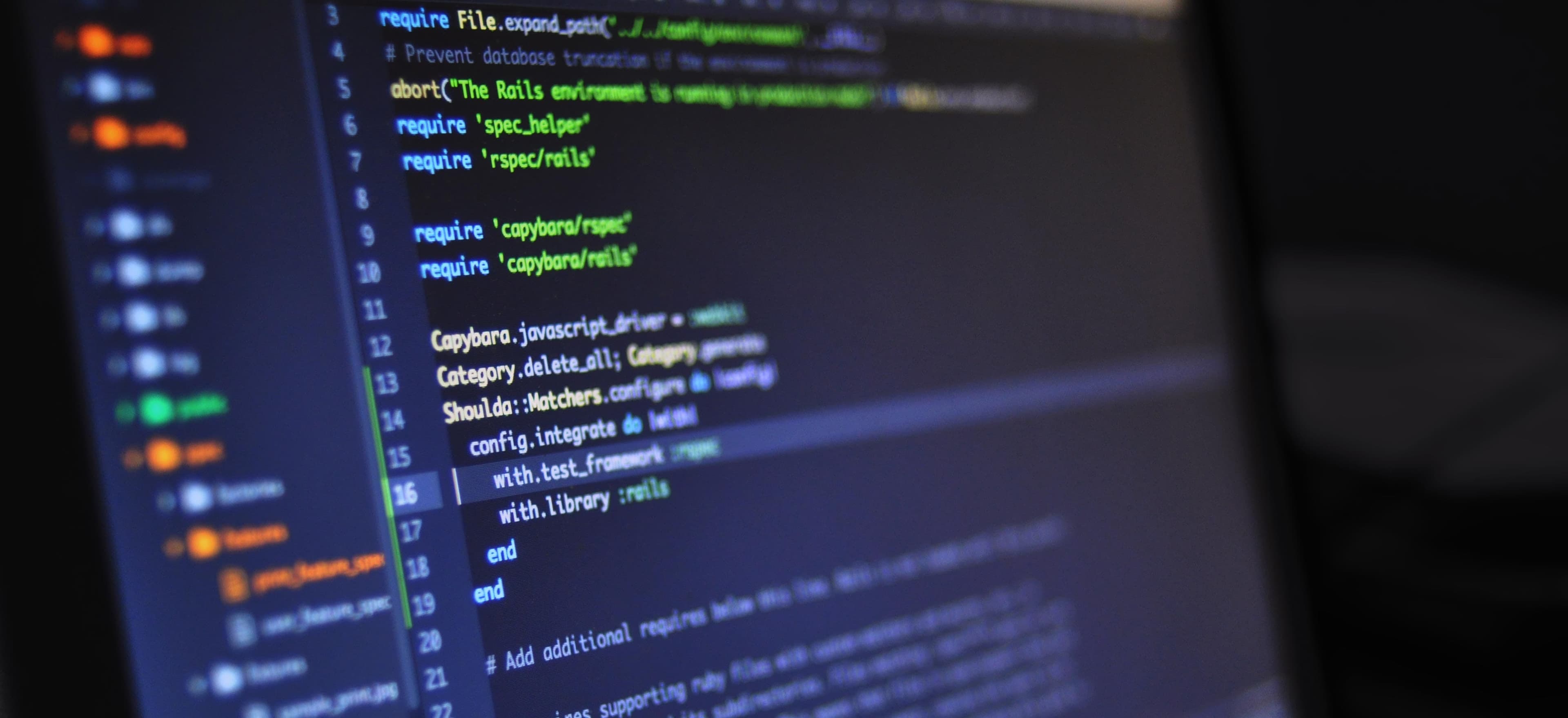
- Published on
Sorting HashMaps by Value in Java 8
Setting the Scene
Java 8 brought about significant changes to the language, especially in terms of data manipulation. With the introduction of Lambdas and the Streams API, Java developers gained powerful tools for streamlining their code and performing advanced operations on collections. One common operation is sorting HashMaps by their values. In this article, we will explore how to sort HashMaps by value in Java 8, step-by-step, using the Streams API. By the end of this article, you will have a clear understanding of how to efficiently sort HashMaps and optimize your code.
Prerequisites
Before we dive into sorting HashMaps by value, there are a few prerequisites you should be familiar with. First, you should have a basic understanding of Java 8, including Lambdas and the Streams API. Additionally, you should be comfortable working with HashMaps and have a general understanding of how they are structured.
Understanding HashMaps and Their Default Order
HashMaps in Java are a data structure that stores key-value pairs. They provide fast and efficient lookup by using hashing techniques. One important thing to note about HashMaps is that they do not have a defined order. The order of the entries in a HashMap is not determined by the order of insertion or any other factor. This lack of intrinsic order is what makes sorting HashMaps by value challenging.
Why Sorting Matters
Sorting a HashMap by its values can be beneficial in various situations. For example, you may want to display the key-value pairs of a HashMap in a sorted manner for better readability. Sorting can also help in finding the maximum or minimum value in the HashMap or perform other calculations based on the values. By sorting HashMaps, you can organize the data in a way that makes it easier to work with and analyze.
The Challenge with Sorting HashMaps
Sorting a HashMap is not as straightforward as sorting an array or a list because HashMaps do not implement the Comparable interface. Therefore, we cannot directly apply the Collections.sort()
method to sort a HashMap by its values. We need to use other techniques provided by Java 8, such as the Streams API, to accomplish this task.
Using the Streams API for Sorting
Java 8 introduced the Streams API, which provides a modern and efficient way to work with collections. Streams allow for functional-style operations on collections and enable us to easily perform complex data manipulation tasks, including sorting HashMaps by value.
To sort a HashMap by value using the Streams API, we need to leverage the Map.Entry
, stream()
, and collect()
methods. Here is a step-by-step guide to sorting a HashMap by value using the Streams API:
Step-by-Step Guide to Sorting by Value
-
Converting the EntrySet to a Stream: First, we need to convert the entry set of the HashMap into a Stream. The entry set represents the key-value pairs in the HashMap. We can use the
entrySet()
method to retrieve the set of entries and then call thestream()
method on it to convert it into a Stream.Map<String, Integer> hashMap = new HashMap<>(); // Add key-value pairs to the HashMap // Convert the entry set to a Stream Stream<Map.Entry<String, Integer>> entryStream = hashMap.entrySet().stream();
-
Sorting the Stream: Once we have the Stream of key-value pairs, we can use the
sorted()
method to sort it. In this case, we want to sort the Stream based on the values of the HashMap. We need to provide a custom Comparator that compares the values of the entries using thecomparing()
method from theComparator
interface.// Sort the Stream based on values Stream<Map.Entry<String, Integer>> sortedStream = entryStream.sorted(Comparator.comparing(Map.Entry::getValue));
-
Collecting the Results: After sorting the Stream, we can convert it back into a Map or another preferred data structure using the
collect()
method. In this case, we want to collect the sorted entries into a new LinkedHashMap, which will maintain the order of insertion.// Convert the sorted Stream into a LinkedHashMap Map<String, Integer> sortedMap = sortedStream.collect(Collectors.toMap(Map.Entry::getKey, Map.Entry::getValue, (e1, e2) -> e1, LinkedHashMap::new));
By following these steps, we can efficiently sort a HashMap by its values using the Streams API. This approach is more concise and generally faster compared to traditional methods using loops and temporary data structures.
Code Snippet Example
Map<String, Integer> hashMap = new HashMap<>();
// Add key-value pairs to the HashMap
// Convert the entry set to a Stream
Stream<Map.Entry<String, Integer>> entryStream = hashMap.entrySet().stream();
// Sort the Stream based on values
Stream<Map.Entry<String, Integer>> sortedStream = entryStream.sorted(Comparator.comparing(Map.Entry::getValue));
// Convert the sorted Stream into a LinkedHashMap
Map<String, Integer> sortedMap = sortedStream.collect(Collectors.toMap(Map.Entry::getKey, Map.Entry::getValue, (e1, e2) -> e1, LinkedHashMap::new));
Using this code snippet as a reference, you can easily adapt it to suit your specific needs and sorting requirements.
This approach has several benefits over traditional methods. It is more concise, making the code easier to read and maintain. Additionally, it leverages the power of the Streams API, which performs sorting in a more optimized and parallelizable manner.
Advanced Sorting Techniques
Sorting HashMaps by their values is relatively straightforward when the values are of a simple type, such as Integer or String. However, when dealing with more complex object types, a custom Comparator may be necessary to define the sorting logic.
To use a custom Comparator, you need to implement the Comparator
interface and provide your own logic for comparing the values of the entries in the HashMap. Here is an example code snippet that demonstrates sorting a HashMap of objects by a specific field:
Code Snippet for Custom Comparator
class CustomComparator implements Comparator<Map.Entry<String, Person>> {
@Override
public int compare(Map.Entry<String, Person> entry1, Map.Entry<String, Person> entry2) {
// Compare the values based on a specific field of the Person object
return entry1.getValue().getAge() - entry2.getValue().getAge();
}
}
// Sorting the values using the custom comparator
Stream<Map.Entry<String, Person>> sortedStream = entryStream.sorted(new CustomComparator());
By implementing a custom Comparator, you have full control over the sorting logic and can sort the HashMap based on any field or combination of fields in the value objects.
This advanced sorting technique provides flexibility and versatility, allowing you to handle complex sorting requirements with ease.
Potential Pitfalls and How to Avert Them
When sorting HashMaps by value, there are a few potential pitfalls you should be aware of:
-
NullPointerException: If the HashMap contains null values, sorting the HashMap using the Streams API may throw a NullPointerException. To avoid this, ensure that your HashMap does not contain null values or handle such cases explicitly in your sorting logic.
-
Duplicate Values: If your HashMap has duplicate values, sorting by values might result in unpredictable behavior. The ordering of the entries with the same value is not guaranteed to be consistent between runs. If you need a deterministic order, consider using
LinkedHashSet
or another data structure that maintains order. -
Performance Considerations: While the Streams API provides a concise and efficient way to sort HashMaps, it might not be the best choice for very large datasets. If performance is a concern, you may need to explore alternative sorting algorithms or custom data structures.
By being aware of these potential pitfalls, you can avoid common mistakes and ensure that your code performs as expected.
Alternative Approaches Pre-Java 8
Prior to Java 8 and the introduction of Lambdas and the Streams API, sorting HashMaps by value required more manual and verbose code. It often involved iterating over the entries and using temporary data structures like ArrayLists or TreeMap to perform the sorting.
Here is an example of how sorting a HashMap by value was typically done before Java 8:
Map<String, Integer> hashMap = new HashMap<>();
// Add key-value pairs to the HashMap
// Create a list of Map entries
List<Map.Entry<String, Integer>> entryList = new ArrayList<>(hashMap.entrySet());
// Sort the list based on values
Collections.sort(entryList, Comparator.comparing(Map.Entry::getValue));
// Create a LinkedHashMap to preserve the order of insertion
Map<String, Integer> sortedMap = new LinkedHashMap<>();
for (Map.Entry<String, Integer> entry : entryList) {
sortedMap.put(entry.getKey(), entry.getValue());
}
As you can see, this approach is more verbose and requires more lines of code compared to the streamlined approach using the Streams API.
Bringing It All Together
Sorting HashMaps by value can be a powerful technique in Java development. With the introduction of Lambdas and the Streams API in Java 8, sorting HashMaps has become much more straightforward and efficient. By following the step-by-step guide and leveraging the power of the Streams API, you can easily sort HashMaps by value and optimize your code.
Throughout this article, we explored various aspects of sorting HashMaps, including the challenges involved and the benefits of using the Streams API. We also discussed advanced sorting techniques, potential pitfalls to avoid, and alternative approaches used in earlier versions of Java.
By mastering the art of sorting HashMaps, you can improve the readability and efficiency of your code and enhance your overall Java development skills.
Further Reading and Resources
For more information on sorting HashMaps and related Java topics, consider exploring the following resources:
- Java API documentation on HashMap
- Java API documentation on Stream
- Java Tutorials: Lambdas and the Streams API
- Stack Overflow: How to Sort a Map by Value in Java
Remember to practice what you've learned and apply these techniques in your own Java projects to gain proficiency and deepen your understanding of sorting HashMaps by value in Java 8.
Call to Action
Now that you have learned how to sort HashMaps by value in Java 8, it's time to put this knowledge into practice. Find a project or an exercise where you can apply these techniques and start experimenting. By actively using these sorting techniques, you will become more comfortable with them and solidify your understanding.