Unifying Builds: Jenkins for Multi-Repo Git Projects
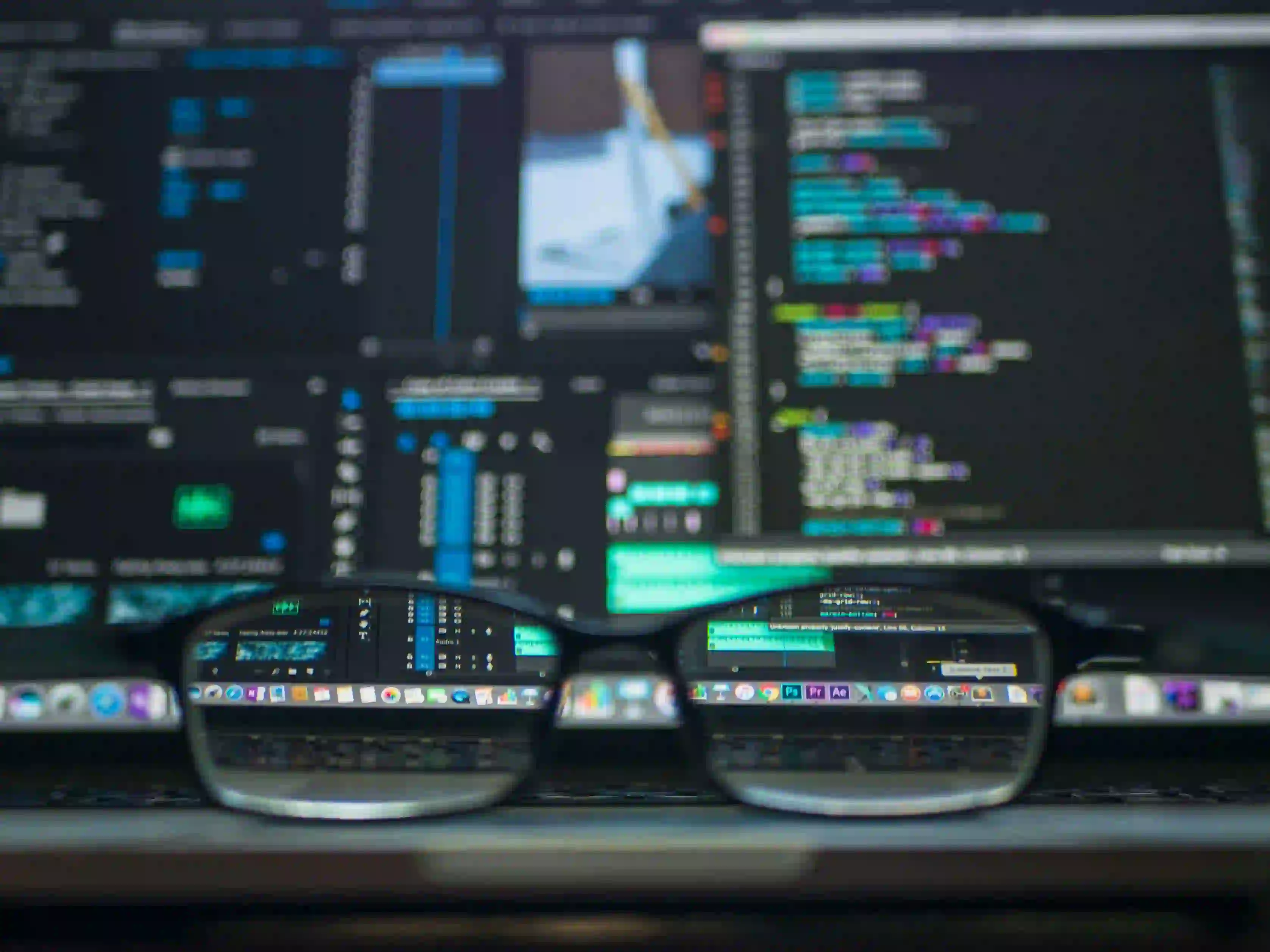
Unifying Builds: Jenkins for Multi-Repo Git Projects
When it comes to managing builds in complex software systems, Jenkins stands as a colossus, reigning over the continuous integration/continuous deployment (CI/CD) landscape. For developers juggling multiple Git repositories, Jenkins offers a streamlined avenue to guarantee stable and coherent build processes. In this post, we delve into the effective use of Jenkins to conquer the often daunting challenge of managing multi-repo Git projects.
The Struggle with Multi-Repo Builds
In the wilderness of software development, managing dependencies and synchronization across multiple Git repositories can feel like herding cats. Each repository may follow its own branching strategy, maintain varying commit histories, and require different build tools. The moment you think everything's lined up, a new code merge could awaken a new series of integration headaches.
Enter Jenkins - your shepherd through the chaos. By implementing a multi-repo build strategy within Jenkins, developers can ensure all the independent pieces of their multi-repo projects come together harmoniously.
Setting Up Jenkins for a Multi-Repo Build
Establishing the Foundation
Before diving into Jenkins’ pool, ensure you have a Jenkins server up and running. If you're new to this, start by visiting the official Jenkins documentation for installation guidance.
Once settled, ensure that the necessary plugins, particularly the Git plugin and any others relevant to your build environment, are installed.
Embracing the Multi-Branch Pipeline
The multi-branch pipeline feature in Jenkins is particularly well-suited for handling multiple repositories. Here’s how it works:
- Create a New Item: Within Jenkins, select "New Item" at the top left of the dashboard.
- Select Multi-branch Pipeline: Name your pipeline and choose "Multibranch Pipeline" from the options.
- Configure Source Repository: Under the "Branch Sources" section, add a new source, choosing "Git" as your repository type.
// Example of configuring a Git repository in Jenkinsfile
pipeline {
agent any
stages {
stage('Checkout') {
steps {
git branch: 'main', url: 'https://github.com/YourProject/YourRepo.git'
}
}
// Define other stages such as build, test, and deploy...
}
}
In the snippet above, Jenkins is directed to check out the main branch of the specified repository. The elegance of this method shines through the ability to script your entire build process within a Jenkinsfile, committed to your repository.
The Magic of Webhooks
For an even smoother CI experience, couple your setup with webhooks. By configuring webhooks in your Git repository, you trigger Jenkins to initiate a new build with each push to the repository, maintaining real-time integration.
Tackling Challenges with Scripted Solutions
Jenkinsfiles are your scripts for success. Be it a declarative pipeline or a scripted one, this Groovy-based domain-specific language allows you to define your build, test, and deployment stages with precision.
Here’s an example of what a Jenkinsfile may look like for a Java project:
pipeline {
agent any
environment {
JAVA_HOME = tool 'AdoptOpenJDK 11'
}
stages {
stage('Build') {
steps {
sh './gradlew build'
}
}
stage('Test') {
steps {
sh './gradlew test'
}
}
}
post {
success {
echo 'Build and Test Stages executed successfully.'
}
failure {
echo 'The build or test has failed. Check console output for details.'
}
}
}
In the environment
directive, we set JAVA_HOME
to an installed JDK, while sh
steps execute shell commands like Gradle build tasks.
Why use scripted steps? It provides custom control over the build process, allowing it to be dynamic and react to different conditions.
Integration with Multi-Repo Configurations
What happens when your project spans multiple repositories, each containing a piece of the puzzle?
For these multi-repo architectures, shared libraries come to the rescue. Shared libraries are codebases hosted in their own Git repositories, which can be referenced in Jenkinsfiles across different projects. This drives reuse and consistency across your builds.
Here’s an expedited glance at how you could tackle this:
- Create a shared library repository that stores common code and scripts.
- Reference the shared library in your Jenkinsfile like so:
@Library('your-shared-library') _
pipeline {
agent any
stages {
stage('Use Shared Library') {
steps {
yourSharedLibraryScript()
}
}
}
}
This script invokes functions from the shared library, streamlining your multi-repo builds.
Advanced Considerations: Dependencies and Artifact Management
Jenkins alone isn't always enough. Real-world projects often depend on specific versions of libraries or modules located in other repositories. Maven, Gradle, and Ivy can play crucial roles in managing these dependencies, especially when combined with artifact repositories like Nexus or Artifactory.
By integrating Jenkins with these additional tools, you embark on a harmonious symphony of dependency resolution, artifact storage, and build automation.
Continuous Integration Best Practices
To wrap up your multi-repo Jenkins strategy with a bow, incorporate these best practices:
- Keep Jenkinsfiles Dry: Don't repeat yourself. Use shared libraries wherever possible to avoid duplicating pipeline code.
- Automate Quality Checks: Integrate static code analysis and other quality checks into your pipeline.
- Plan for Disaster: Implement disaster recovery strategies, including regular backups of your Jenkins configuration.
In Conclusion
Migrating to a Jenkins-powered CI/CD pipeline for multi-repo Git projects brings organization to chaos, transforming the complexity into an orchestrated ballet of automated builds.
It might seem intimidating at first, but Jenkins, complemented by proper configuration, shared libraries, and real-time feedback via webhooks, can tame even the wildest of multi-repo setups.
For those ready to take their CI/CD to the next level, further exploration into Jenkins' capabilities can be pursued through a compendium of resources found at the Jenkins Handbook.
Remember: the ultimate goal is seamless integration. With Jenkins as your steadfast companion, multiple repositories can function as cogent, coordinated facets of your broader software ecosystem, rather than disparate code silos.
Today, you are not just a software developer. You are a maestro, conducting an ensemble of repositories with the wave of your Jenkins wand. Happy building!