ECC Encryption: Boost Security with OpenJDK!
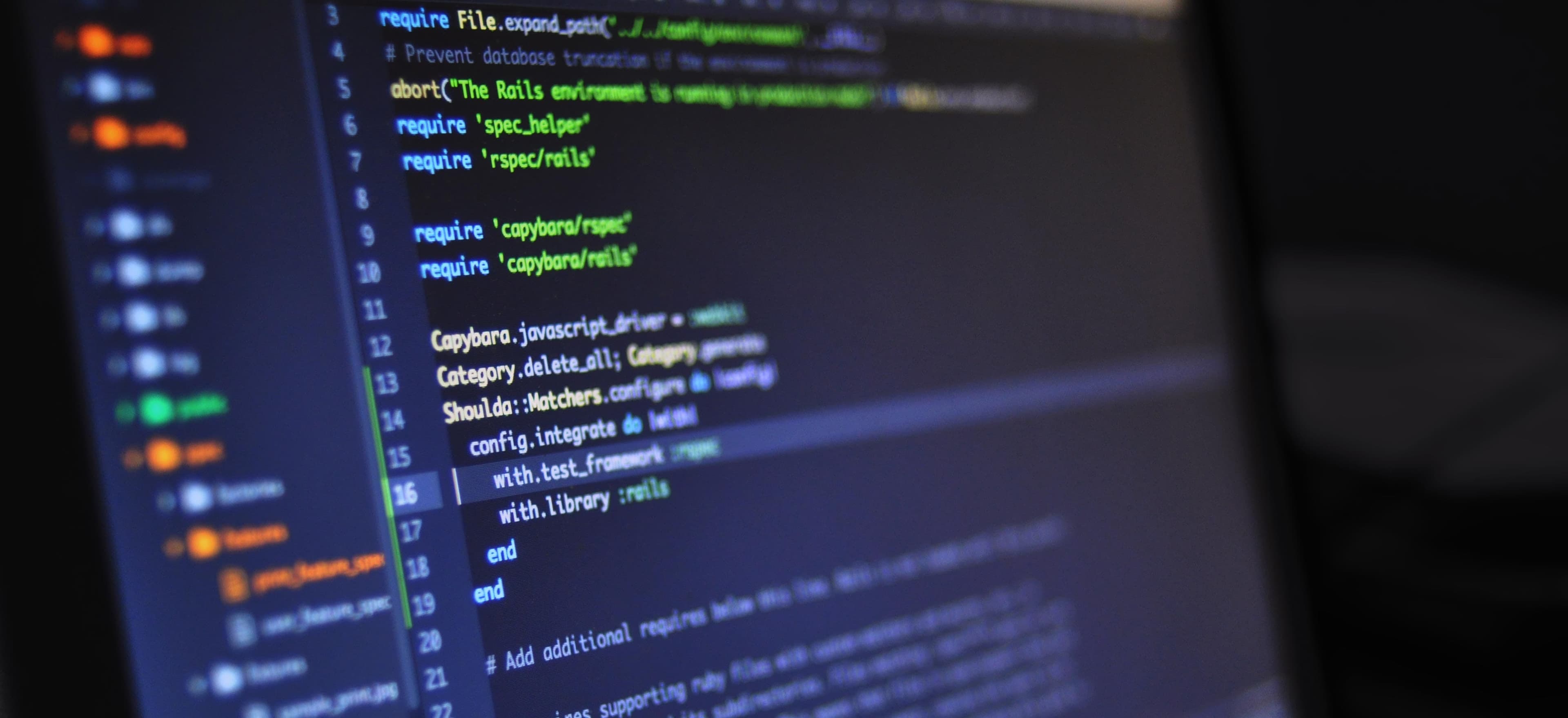
- Published on
ECC Encryption: Boost Security with OpenJDK!
In an increasingly digital world, the importance of robust security can't be overstated. Encryption is the cornerstone of secure data transmission, and yet, it's a constantly evolving landscape. Enter Elliptic Curve Cryptography (ECC)—a cutting-edge approach to encryption that offers superior security with smaller keys compared to traditional methods like RSA. In this post, we'll dive into the world of ECC encryption and demonstrate how you can implement it using OpenJDK, the open-source implementation of the Java Platform.
Why ECC Encryption?
ECC offers several advantages that make it an attractive option for modern encryption needs:
Smaller Yet Stronger Keys: ECC provides the same level of security as RSA but with much smaller key sizes. This translates into faster computations and lower processing power requirements—an essential trait in mobile and IoT devices.
Adaptability: As cyber threats evolve, ECC's ability to provide stronger encryption without a significant hit to performance means systems can remain secure without a constant need for hardware upgrades.
Industry Support: ECC is widely supported and recommended by industry standards and is viewed as the future-forward choice for digital security.
Implementing ECC with OpenJDK
Java, with its OpenJDK runtime, provides a solid framework for implementing ECC encryption. Below is a step-by-step guide to implementing ECC encryption using OpenJDK.
Step 1: Generate ECC Key Pair
In Java, cryptographic operations are often handled by the KeyPairGenerator
class. Here's how to initialize it for ECC:
import java.security.KeyPair;
import java.security.KeyPairGenerator;
import java.security.SecureRandom;
import java.security.spec.ECGenParameterSpec;
public class ECCKeyPairGenerator {
public static KeyPair generateECCKeyPair() throws Exception {
KeyPairGenerator keyPairGenerator = KeyPairGenerator.getInstance("EC");
ECGenParameterSpec ecSpec = new ECGenParameterSpec("secp256r1"); // NIST P-256 curve
keyPairGenerator.initialize(ecSpec, new SecureRandom());
return keyPairGenerator.generateKeyPair();
}
}
Why the 'secp256r1' curve? This is one of the most popular and well-studied curves, providing a solid balance of security and performance.
Step 2: Encrypt Data with ECC Public Key
Encryption with ECC typically involves using a shared secret derived from the recipient's public key and the sender's private key. The following outlines how to encrypt a plaintext message:
import javax.crypto.KeyAgreement;
import javax.crypto.spec.SecretKeySpec;
import javax.crypto.Cipher;
import java.security.PublicKey;
import java.security.PrivateKey;
import java.util.Base64;
public class ECCEncryption {
public static String encrypt(String plaintext, PublicKey publicKey, PrivateKey privateKey) throws Exception {
KeyAgreement keyAgreement = KeyAgreement.getInstance("ECDH");
keyAgreement.init(privateKey);
keyAgreement.doPhase(publicKey, true);
SecretKeySpec aesKey = new SecretKeySpec(keyAgreement.generateSecret(), "AES");
Cipher cipher = Cipher.getInstance("AES/GCM/NoPadding");
cipher.init(Cipher.ENCRYPT_MODE, aesKey);
byte[] encryptedBytes = cipher.doFinal(plaintext.getBytes());
return Base64.getEncoder().encodeToString(encryptedBytes);
}
}
Why AES in concert with ECC? ECC alone does not encrypt data; instead, it's used to securely exchange or derive keys. AES is a symmetric cipher used for the actual encryption of data with that key.
Step 3: Decrypt Data with ECC Private Key
Decryption is the reverse process of encryption. Here's a method to decrypt ciphertext using the recipient's private key:
public class ECCDecryption {
public static String decrypt(String ciphertext, PublicKey publicKey, PrivateKey privateKey) throws Exception {
byte[] encryptedBytes = Base64.getDecoder().decode(ciphertext);
KeyAgreement keyAgreement = KeyAgreement.getInstance("ECDH");
keyAgreement.init(privateKey);
keyAgreement.doPhase(publicKey, true);
SecretKeySpec aesKey = new SecretKeySpec(keyAgreement.generateSecret(), "AES");
Cipher cipher = Cipher.getInstance("AES/GCM/NoPadding");
cipher.init(Cipher.DECRYPT_MODE, aesKey);
byte[] decryptedBytes = cipher.doFinal(encryptedBytes);
return new String(decryptedBytes);
}
}
Why 'AES/GCM/NoPadding'? This choice provides both confidentiality and integrity by combining AES in Galois/Counter Mode (GCM), which offers an authenticated encryption with associated data (AEAD).
Step 4: Putting It All Together
To demonstrate ECC encryption and decryption in action, we bring together the above components:
public class ECCDemo {
public static void main(String[] args) {
try {
// Generate Key Pair
KeyPair keyPair = ECCKeyPairGenerator.generateECCKeyPair();
// Simulation of secure transmission of public key
PublicKey publicKey = keyPair.getPublic();
PrivateKey privateKey = keyPair.getPrivate();
// Encrypt a message
String plaintext = "Hello, ECC World!";
String encryptedText = ECCEncryption.encrypt(plaintext, publicKey, privateKey);
System.out.println("Encrypted Text: " + encryptedText);
// Decrypt the message
String decryptedText = ECCDecryption.decrypt(encryptedText, publicKey, privateKey);
System.out.println("Decrypted Text: " + decryptedText);
} catch (Exception e) {
e.printStackTrace();
}
}
}
By running this ECCDemo
class, you'll see the power of ECC encryption and decryption in a Java environment.
Enhance Your Java Security Knowledge
While this guide provides a basic introduction to ECC in Java, there's much more to explore such as key management, certificate handling, and specific use-cases. Always ensure you're up-to-date with the latest security practices and documentation provided by OpenJDK.
Final Thoughts
ECC encryption can provide a desirable combination of security and performance for modern applications, from web services to IoT devices. By leveraging OpenJDK's comprehensive APIs, Java developers can integrate advanced cryptographic techniques into their applications to ensure data confidentiality and integrity.
Remember: as the digital world evolves, so too must our approaches to security. ECC and OpenJDK provide a powerful toolkit for stepping up your security game.