Boost Coding: Secrets to Writing Efficient Methods!
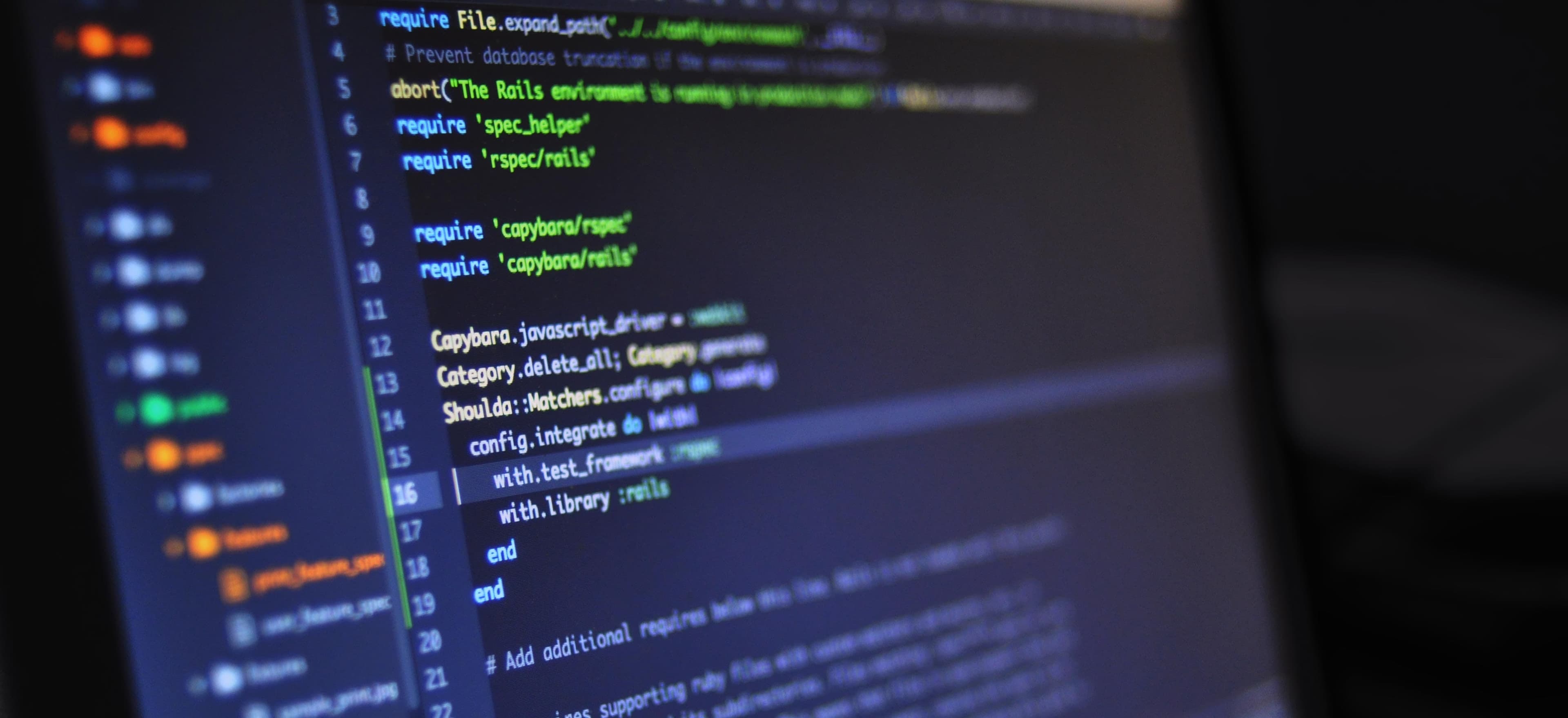
- Published on
Boost Coding: Secrets to Writing Efficient Methods in Java
The Opening Bytes
Java is a popular object-oriented programming language used for developing a wide range of applications. One of the key factors that differentiate good Java developers from the rest is their ability to write efficient and optimized code. Writing efficient methods is crucial for improving the performance and scalability of Java applications.
In this article, we will explore the secrets to writing efficient methods in Java. We will discuss the importance of algorithm complexity, memory usage, and method calling overhead. We will also explore best practices for writing efficient Java methods, such as choosing the right algorithms, keeping methods short and simple, utilizing libraries and built-in functions, avoiding unnecessary calculations inside loops, and minimizing recursion depth. Additionally, we will dive into profiling and testing techniques to ensure the efficiency of Java methods.
Understanding Java Method Efficiency
A Java method is a sequence of statements that perform a specific task. Methods can take inputs (parameters), perform computations, and return results. Understanding the efficiency of methods is crucial for optimizing the performance of Java applications.
One of the key factors that determine the efficiency of a method is its algorithm complexity, often expressed using Big O notation. The algorithm complexity measures the growth rate of the method's time and space requirements as the input size increases. For example, an algorithm with a time complexity of O(n^2) will take quadratically longer as the input size increases compared to an algorithm with a time complexity of O(n).
Memory usage is another important aspect of method efficiency. Efficient methods optimize memory allocation and deallocation to minimize unnecessary memory usage and prevent memory leaks. Managing memory efficiently is particularly important in long-running applications to avoid running out of memory or causing excessive garbage collection.
Method calling overhead also contributes to overall method efficiency. Each method call introduces a certain amount of performance overhead due to the context switching and memory management involved. Minimizing the number of method calls, especially in performance-critical sections of code, can significantly improve overall application performance.
To gain a deeper understanding of algorithm complexity, memory management, and method calling overhead, it is useful to refer to external resources such as:
- Introduction to Big O notation
- How Java manages memory
- Understanding method calling overhead
Best Practices for Writing Efficient Java Methods
Choose the Right Algorithm
The choice of algorithm has a significant impact on the efficiency of a method. Choosing the most efficient algorithm for a given task can greatly improve the performance of the code. Let's take a look at some examples:
-
Binary search vs. linear search: When searching for an element in a sorted array, a binary search algorithm has a time complexity of O(log n), whereas a linear search algorithm has a time complexity of O(n). In scenarios where the number of elements is large, using a binary search algorithm can significantly reduce the search time.
-
Sorting algorithms: Different sorting algorithms have different time complexities. For example, the selection sort algorithm has a time complexity of O(n^2), whereas the merge sort algorithm has a time complexity of O(n log n). Choosing the appropriate sorting algorithm based on the input size can make a significant difference in performance.
To gain a deeper understanding of algorithms and their efficiencies, it is recommended to refer to resources such as:
- Big-O Cheat Sheet - A quick reference guide to common algorithm complexities.
- Visualizing Algorithms - An interactive platform to visualize various algorithms and their efficiencies.
Keep It Short and Simple (KISS)
The KISS principle emphasizes the importance of simplicity and clarity in code. By keeping methods short and simple, developers can improve code maintainability and, surprisingly, performance.
Smaller methods are easier to understand and modify. They are less prone to bugs and make the code easier to test and debug. Additionally, smaller methods give the compiler more opportunities for optimization, as they can be independently analyzed and optimized.
Consider the following example method:
// Original method
public int calculateSquare(int number) {
int result = 0;
for (int i = 0; i < number; i++) {
result += number;
}
return result;
}
In the original method, the calculation is done by repeatedly adding the input number to the result. However, a more efficient method can be written using the mathematical property of squaring:
// Refactored method
public int calculateSquare(int number) {
return number * number;
}
The refactored method is shorter, easier to understand, and performs the same task efficiently. By adhering to the KISS principle, we not only improve code readability and maintainability but also make it more efficient.
Utilize Libraries and Built-in Functions
Java provides a rich set of libraries and built-in functions that are optimized for various tasks. Leveraging these standard libraries and functions can often lead to improved performance compared to custom-written code.
For example, libraries such as Apache Commons and Guava offer utility classes for common operations. These libraries have been extensively tested and optimized for performance. Suppose you need to check if a string is empty or null. Instead of writing custom code, you can use the built-in StringUtils
class from Apache Commons:
import org.apache.commons.lang3.StringUtils;
// ...
public boolean isStringEmptyOrNull(String str) {
return StringUtils.isEmpty(str);
}
The StringUtils
class provides the isEmpty
method, which handles null-safe checks for empty strings. Utilizing such libraries can save development time and ensure efficient handling of common tasks.
Avoid Unnecessary Calculations Inside Loops
Calculating values inside loops can lead to unnecessary overhead, especially if the calculations are independent of the loop variable. It is a good practice to move calculations that do not depend on the loop variable outside the loop to improve efficiency.
Consider the following example:
// Inefficient loop
public int sumValues(int[] values) {
int sum = 0;
for (int i = 0; i < values.length; i++) {
sum += values[i] * values[i];
}
return sum;
}
In the inefficient loop, the square of each value is recalculated in every iteration. However, the calculation values[i] * values[i]
does not depend on the loop variable i
. By moving the calculation outside the loop, we can optimize the method:
// Efficient loop
public int sumValues(int[] values) {
int sum = 0;
for (int i = 0; i < values.length; i++) {
int square = values[i] * values[i];
sum += square;
}
return sum;
}
By calculating the square outside the loop and storing it in a separate variable, we avoid unnecessary calculations in each iteration, leading to improved efficiency.
Minimize Recursion Depth
Recursion is a useful technique in programming, but excessive recursion can lead to performance issues such as stack overflow or excessive memory usage.
Recursion involves a method repeatedly calling itself until a specific termination condition is met. In some cases, recursive methods can be optimized or converted to iterative solutions to improve efficiency.
Consider the following example of a recursive method to calculate the factorial of a number:
// Recursive factorial method
public int factorial(int n) {
if (n == 0) {
return 1;
} else {
return n * factorial(n - 1);
}
}
The recursive factorial method calculates the factorial by repeatedly multiplying the number with the factorial of the previous number. However, this approach can be inefficient for large values of n
.
// Iterative factorial method
public int factorial(int n) {
int result = 1;
for (int i = 1; i <= n; i++) {
result *= i;
}
return result;
}
The iterative factorial method calculates the factorial using a loop, avoiding excessive method calls. This approach is more efficient and less prone to stack overflow for large values of n
.
It is important to note that recursion can be an elegant and efficient solution for certain problems. Whether to use recursion or not depends on the problem at hand and the specific performance requirements.
Profiling and Testing for Efficient Java Methods
Efficient coding is not just about writing optimized code upfront; it also involves profiling and testing to identify potential bottlenecks and ensure that the code performs well in practice.
Profiling Java Applications
Profiling is the process of analyzing the behavior and performance of an application to identify areas that can be optimized. It helps developers understand how the code is executing, find performance bottlenecks, and measure the impact of optimizations.
There are several profiling tools available for Java, such as JProfiler, VisualVM, and YourKit. These tools provide insights into CPU usage, memory usage, method execution times, and other performance-related metrics. By analyzing the profiling data, developers can pinpoint areas of the code that need optimization and make informed decisions.
To learn more about profiling Java applications and the available tools, refer to the following resources:
- Introduction to Java profiling - An overview of profiling techniques and tools for Java.
- JProfiler documentation - Official documentation for JProfiler.
- VisualVM documentation - Official documentation for VisualVM.
Writing Testable Code
Writing testable code is essential for maintaining method efficiency in the long run. Testable code is modular, loosely coupled, and follows good design principles, such as separation of concerns and dependency inversion. Test-driven development (TDD) practices can also help catch performance issues early on.
Unit testing frameworks like JUnit and TestNG provide tools and conventions for writing and executing tests. By writing comprehensive test cases, developers can ensure that their methods are performing as expected and quickly catch any performance regressions.
To learn more about writing testable code and using unit testing frameworks, refer to the following resources:
- Introduction to Unit Testing - An introduction to unit testing in Java with JUnit.
- TestNG documentation - Official documentation for TestNG.
Benchmarking with JMH
Benchmarking is the process of measuring the performance of a method or a system under controlled conditions. Java Microbenchmark Harness (JMH) is a popular tool for benchmarking Java code. It provides a standardized way to measure method execution time and analyze performance metrics.
JMH generates reliable benchmarking results by taking into account JVM warm-up, JIT compilation, and other factors that can affect performance. It helps developers identify the most efficient implementation among multiple alternatives and measure the impact of optimizations.
To learn more about benchmarking with JMH, refer to the following resources:
- Introduction to JMH - An introduction to JMH and its usage for benchmarking Java code.
- JMH sample benchmarks - Official sample benchmarks provided by the OpenJDK project.
Case Study: Optimizing a Java Method in the Real World
To illustrate the process of optimizing a Java method, let's consider an example from an open-source project. We will discuss the original implementation, the identified issues, and the steps taken to improve the method.
The method we will be optimizing is from a web application framework and is responsible for rendering a template by replacing placeholders with the corresponding values:
public String renderTemplate(String template, Map<String, String> values) {
for (String key : values.keySet()) {
template = template.replace("{" + key + "}", values.get(key));
}
return template;
}
The original implementation uses the replace()
method to perform placeholder substitution. However, this approach has a performance issue when the template contains many placeholders or when the template is large. The replace()
method creates a new String
object for each replacement, leading to excessive memory allocation and garbage collection.
To optimize the method, we can use a StringBuilder
for efficient string concatenation:
public String renderTemplate(String template, Map<String, String> values) {
StringBuilder sb = new StringBuilder(template);
for (Map.Entry<String, String> entry : values.entrySet()) {
String placeholder = "{" + entry.getKey() + "}";
int index = sb.indexOf(placeholder);
while (index != -1) {
sb.replace(index, index + placeholder.length(), entry.getValue());
index = sb.indexOf(placeholder, index + entry.getValue().length());
}
}
return sb.toString();
}
In the optimized version, we use StringBuilder
to avoid creating unnecessary String
objects. We iterate over the placeholders and use indexOf()
to find their occurrences in the template. We replace each occurrence with the corresponding value, iteratively searching for the next occurrence until all placeholders are replaced.
This optimization reduces the memory usage and improves the overall performance of the renderTemplate()
method.
Closing Remarks
Writing efficient methods is crucial for optimizing the performance and scalability of Java applications. By choosing the right algorithms, keeping methods short and simple, utilizing libraries and built-in functions, avoiding unnecessary calculations inside loops, minimizing recursion depth, profiling, and testing, developers can significantly improve the efficiency of their code.
Continuous learning, exploring profiling and testing tools, and benchmarking with tools like JMH are essential for identifying bottlenecks and making informed optimization decisions.
Remember that efficiency is not just about speed; it also involves managing resources and ensuring application scalability. By following the best practices and continuously optimizing their code, Java developers can boost their coding skills and develop high-performing applications.
Further Reading
- "Effective Java" by Joshua Bloch - A classic book that offers insights into writing efficient and reliable Java code.
- "Java Performance: The Definitive Guide" by Scott Oaks - A comprehensive guide to understanding and improving Java application performance.