Beat Bots: Integrating reCAPTCHA in Spring Boot Apps
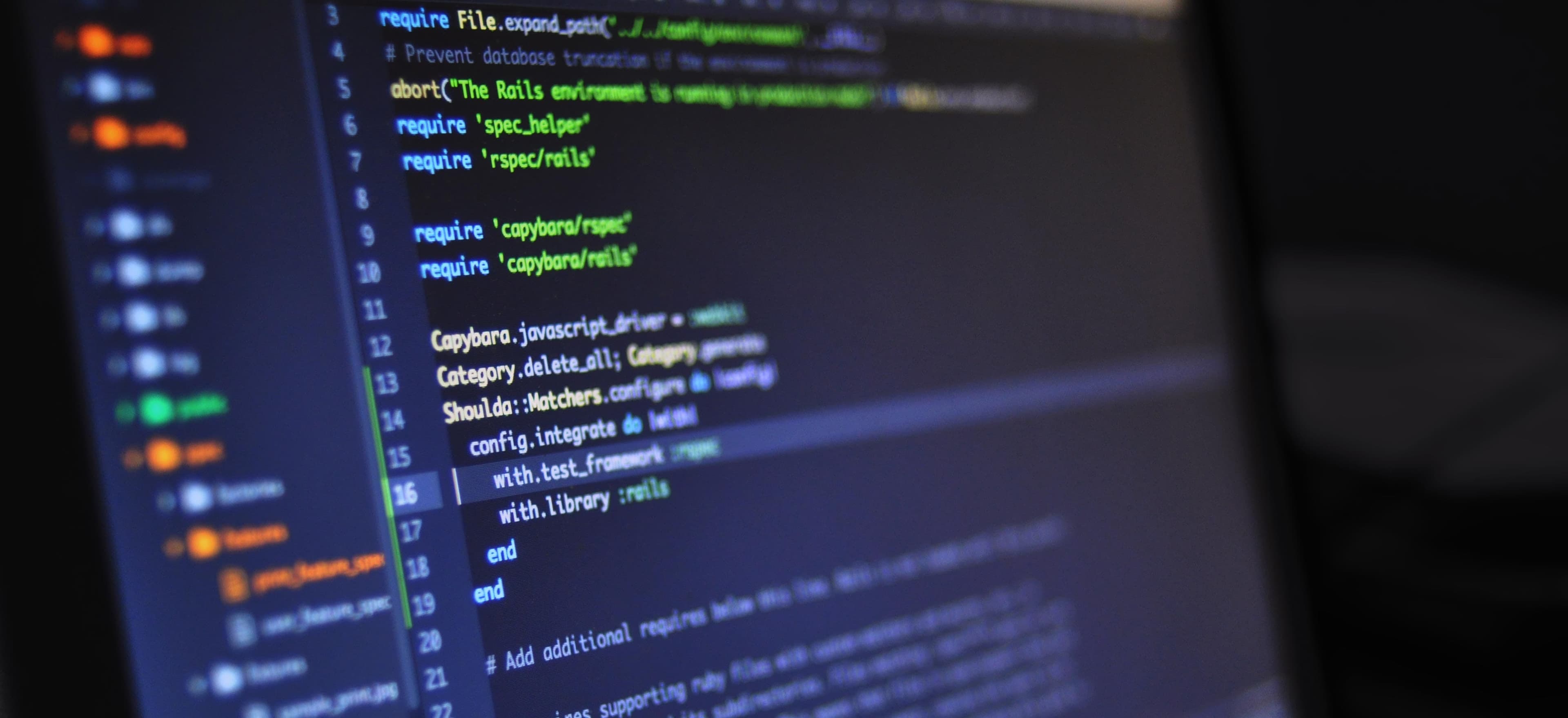
- Published on
Beat Bots: Integrating reCAPTCHA in Spring Boot Apps
The Approach
In today's digital world, web applications are constantly targeted by bots and automated scripts. These malicious actors can carry out activities that range from stealing sensitive information to overloading the system with spam or DDoS attacks. To protect against these threats, it is crucial to implement CAPTCHA mechanisms in web applications. CAPTCHA stands for "Completely Automated Public Turing test to tell Computers and Humans Apart" and is designed to distinguish between humans and bots.
One of the most popular and widely used CAPTCHA mechanisms is Google's reCAPTCHA. It provides a simple and effective solution to defend against automated threats. reCAPTCHA uses advanced risk analysis techniques and machine learning algorithms to determine whether a user is a human or a bot. With its easy integration and seamless user experience, reCAPTCHA is an ideal choice for Java developers using Spring Boot to secure their applications.
In this article, we will explore how to integrate reCAPTCHA into Spring Boot applications. We will cover everything from setting up the environment, configuring reCAPTCHA, building the reCAPTCHA service, adding reCAPTCHA to web forms, handling errors and logging, and testing the integration. Whether you are a Spring Boot developer looking to enhance the security of your application or someone interested in understanding how reCAPTCHA works, this article is for you.
What is reCAPTCHA?
reCAPTCHA is a widely-used system that protects websites from spam and abuse. It was first introduced by Carnegie Mellon University in 2007 and was later acquired by Google in 2009. reCAPTCHA utilizes various techniques to differentiate between humans and bots, including image and audio recognition, behavioral analysis, and machine learning algorithms.
There are different versions of reCAPTCHA available, each catering to specific use cases. reCAPTCHA v2 is the most commonly used version and requires users to select images that fulfill a specific criteria (such as identifying all images containing a car). Invisible reCAPTCHA is a user-friendly option that runs in the background and only requires users to solve a challenge if they are flagged as suspicious. reCAPTCHA v3, on the other hand, provides a score between 0.0 and 1.0 for each user interaction and allows developers to set custom actions based on the score.
reCAPTCHA has evolved over the years to become smarter and more efficient at identifying bots. It has become an essential tool for website owners to safeguard their applications and prevent automated attacks.
Why integrate reCAPTCHA in a Spring Boot application?
Integrating reCAPTCHA in a Spring Boot application offers multiple benefits for both developers and users.
By incorporating reCAPTCHA in your application, you can enhance the security of your website. It helps protect against automated attacks that aim to exploit vulnerabilities in the system. With reCAPTCHA, you can validate that the user interacting with your application is indeed a human and not a bot. This helps prevent unauthorized access, spam registrations, and other malicious activities.
Apart from the security advantages, reCAPTCHA also improves the overall user experience. Instead of relying solely on traditional security measures such as CAPTCHA codes or complex puzzles, reCAPTCHA provides a more user-friendly and frictionless experience. The newer versions, such as Invisible reCAPTCHA and reCAPTCHA v3, work seamlessly in the background without inconveniencing genuine users.
Spring Boot is a popular choice among Java developers for building robust and scalable web applications. It provides a comprehensive framework that simplifies the development process and enables the creation of high-quality applications. By integrating reCAPTCHA in your Spring Boot application, you can leverage the advantages of both technologies and ensure the security and smooth functioning of your application.
Now that we understand the importance of integrating reCAPTCHA in a Spring Boot application, let's dive into the process of setting up the environment.
Setting up the Environment
Prerequisites
Before we begin integrating reCAPTCHA in a Spring Boot application, ensure that you have the following prerequisites:
-
Java Development Kit (JDK): Install the latest version of JDK to run Spring Boot applications.
-
Spring Boot: Install the Spring Boot framework, which provides a platform for developing Spring-based applications.
-
Maven or Gradle: Choose either Maven or Gradle as the build automation tool for managing dependencies and building the project.
-
IDE or Text Editor: Choose your preferred IDE or text editor for writing code and managing the project. Popular choices include IntelliJ IDEA, Eclipse, and Visual Studio Code.
These prerequisites are essential for setting up the environment and developing the Spring Boot application.
Creating a Spring Boot Application
Once you have the prerequisites in place, we can proceed to create a new Spring Boot application. There are two ways to generate a Spring Boot project: using start.spring.io or using an Integrated Development Environment (IDE) such as IntelliJ IDEA or Eclipse.
Option 1: Using start.spring.io
-
Open your web browser and navigate to https://start.spring.io.
-
Fill in the necessary details such as Group, Artifact, and Project Metadata. Select the desired Spring Boot version.
-
Choose the project type (Maven or Gradle) and language (Java or Kotlin).
-
Specify the project dependencies. For reCAPTCHA integration, you will need to add the
spring-boot-starter-web
andspring-boot-starter-thymeleaf
dependencies. -
Click on the "Generate" button to download the project as a ZIP file.
-
Extract the ZIP file to a location on your local machine.
Option 2: Using an IDE
-
Open your preferred IDE (IntelliJ IDEA, Eclipse, etc.).
-
Create a new Spring Boot project using the IDE's project wizard. Provide the necessary details such as Group, Artifact, and Project Metadata.
-
Choose the project type (Maven or Gradle) and language (Java or Kotlin).
-
Select the desired Spring Boot version and specify the project dependencies. For reCAPTCHA integration, you will need to add the
spring-boot-starter-web
andspring-boot-starter-thymeleaf
dependencies. -
Click on the "Finish" button to create the project.
Regardless of the method you choose, you will end up with a basic Spring Boot project that includes the required dependencies for web development and templating.
In the next section, we will dive into integrating reCAPTCHA with Spring Boot.
Integrating reCAPTCHA with Spring Boot
To integrate reCAPTCHA with a Spring Boot application, we need to follow a few key steps. These include registering with reCAPTCHA, configuring reCAPTCHA in Spring Boot, building the reCAPTCHA service, adding reCAPTCHA to web forms, and validating the reCAPTCHA response on the server-side. Let's explore each of these steps in detail.
Registration with reCAPTCHA
The first step in integrating reCAPTCHA in your Spring Boot application is to register your website with reCAPTCHA. This will provide you with the necessary API keys required to interact with the reCAPTCHA service.
Follow these steps to register your website with reCAPTCHA:
-
Go to the reCAPTCHA website: https://www.google.com/recaptcha.
-
Click on the "Admin Console" button at the top right corner of the page.
-
Sign in with your Google account if you haven't already.
-
Once signed in, you will be redirected to the reCAPTCHA admin console. Click on the "Register a new site" button.
-
Provide a label for your site (e.g., MySpringBootApp) and specify the type of reCAPTCHA you want to use (v2, Invisible, or v3).
-
Enter the domain of your website. For local development, you can use "localhost" or any other test domain that you have set up.
-
Accept the reCAPTCHA Terms of Service and click on the "Submit" button.
-
After submitting, you will be presented with a page that displays your site key and secret key. These keys will be used in the Spring Boot application to interact with the reCAPTCHA service.
Ensure that you keep your secret key secure, as it provides access to the reCAPTCHA service and should not be shared publicly.
Configuring reCAPTCHA in Spring Boot
Now that we have obtained the API keys from reCAPTCHA, the next step is to configure the keys in our Spring Boot application.
In Spring Boot, we can store our API keys securely by utilizing the application configuration files (application.properties
or application.yml
).
To configure the reCAPTCHA API keys in application.properties
, open the src/main/resources/application.properties
file and add the following lines:
# reCAPTCHA Configuration
spring.security.recaptcha.site-key=YOUR_SITE_KEY
spring.security.recaptcha.secret-key=YOUR_SECRET_KEY
If you prefer using application.yml
, open the src/main/resources/application.yml
file and add the following lines:
# reCAPTCHA Configuration
spring:
security:
recaptcha:
site-key: YOUR_SITE_KEY
secret-key: YOUR_SECRET_KEY
Replace YOUR_SITE_KEY
with your actual site key obtained from reCAPTCHA, and YOUR_SECRET_KEY
with your secret key.
By configuring the API keys in the Spring Boot application, we can easily access them throughout the code and utilize them in the reCAPTCHA service.
Building the reCAPTCHA Service
To encapsulate the reCAPTCHA validation logic, we will create a service class called ReCaptchaService
. Following good practices, we will define an interface IReCaptchaService
that provides the contract, and then implement it in the service class.
Let's start by creating the IReCaptchaService
interface:
public interface IReCaptchaService {
boolean verifyResponse(String reCaptchaResponse, String clientIp);
}
The verifyResponse
method takes in the reCAPTCHA response and the client IP address and returns a boolean indicating whether the response is valid or not.
Next, let's implement the ReCaptchaService
class:
@Service
public class ReCaptchaService implements IReCaptchaService {
private final RestTemplate restTemplate;
private final String recaptchaSecretKey;
public ReCaptchaService(@Value("${spring.security.recaptcha.secret-key}") String recaptchaSecretKey) {
this.restTemplate = new RestTemplate();
this.recaptchaSecretKey = recaptchaSecretKey;
}
@Override
public boolean verifyResponse(String reCaptchaResponse, String clientIp) {
MultiValueMap<String, String> formData = new LinkedMultiValueMap<>();
formData.add("secret", recaptchaSecretKey);
formData.add("response", reCaptchaResponse);
formData.add("remoteip", clientIp);
String reCaptchaUrl = "https://www.google.com/recaptcha/api/siteverify";
ReCaptchaResponse response = restTemplate.postForObject(reCaptchaUrl, formData, ReCaptchaResponse.class);
return response != null && response.isSuccess();
}
}
In the ReCaptchaService
class, we inject the restTemplate
bean, which will be used to make an HTTP POST request to the reCAPTCHA API. We also inject the recaptchaSecretKey
from the application configuration files.
The verifyResponse
method sends the reCAPTCHA response, secret key, and client IP address to the reCAPTCHA API for verification. It then checks the response to determine whether the reCAPTCHA was successful or not.
Ensure that you have the necessary dependencies, such as spring-web
and spring-webmvc
, added to your pom.xml
or build.gradle
file to use the RestTemplate
class.
By creating the ReCaptchaService
, we have encapsulated the logic required to validate reCAPTCHA responses. This service can now be utilized in the controller to handle form submissions.
Adding reCAPTCHA to a Web Form
To include reCAPTCHA in a web form, we need to add the reCAPTCHA widget to the HTML form. In Spring Boot, we can achieve this using templating engines like Thymeleaf.
Let's consider a simple registration form with a reCAPTCHA widget:
<!DOCTYPE html>
<html xmlns:th="http://www.thymeleaf.org">
<head>
<title>Registration Form</title>
</head>
<body>
<h1>Registration Form</h1>
<form action="/register" method="post">
<!-- Other form fields -->
<div class="g-recaptcha" data-sitekey="YOUR_SITE_KEY"></div>
<button type="submit">Register</button>
</form>
<!-- Other scripts and styles -->
<script src="https://www.google.com/recaptcha/api.js" async defer></script>
</body>
</html>
In the example above, we have added a <div>
element with the class g-recaptcha
, which will be replaced with the actual reCAPTCHA widget. Ensure that you replace YOUR_SITE_KEY
with your actual site key obtained from reCAPTCHA.
It is also important to include the reCAPTCHA JavaScript resource by adding the following script tag before the closing </body>
tag:
<script src="https://www.google.com/recaptcha/api.js" async defer></script>
This script tag loads the reCAPTCHA API asynchronously and enables the reCAPTCHA widget to render on the page.
By incorporating the reCAPTCHA widget in the web form, users will be required to solve the reCAPTCHA challenge before submitting the form.