Boosting Camel Routes: Key Performance Tuning Tips
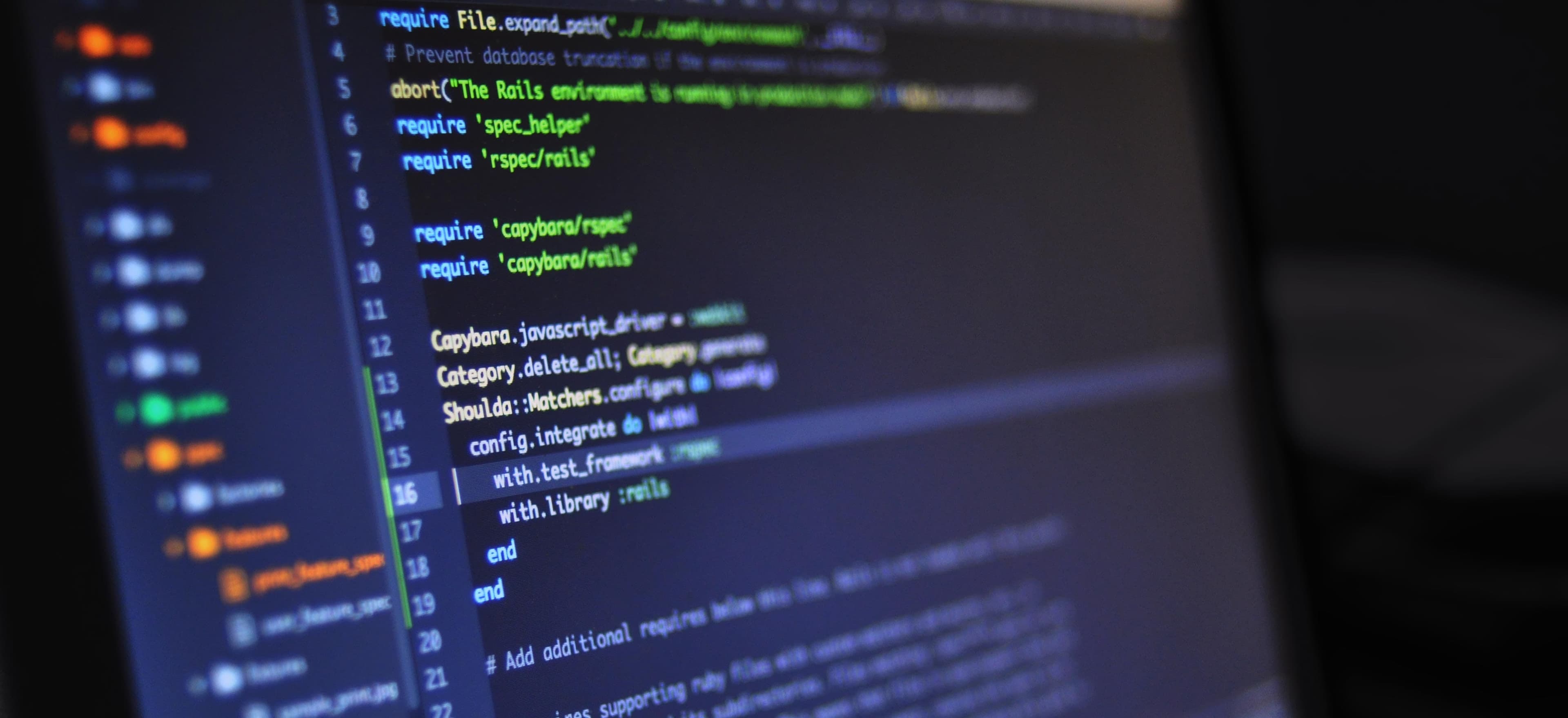
- Published on
Boosting Camel Routes: Key Performance Tuning Tips
Apache Camel is a powerful integration framework that simplifies the development of enterprise-grade applications by providing a wide range of routing and mediation capabilities. However, like any other framework, Camel performance can be impacted by various factors. In this blog post, we will explore some key performance tuning tips that can help boost the performance of your Camel routes.
1. Use Lightweight Components
The choice of Camel components greatly impacts the performance of your routes. Some components, such as direct
, seda
, and vm
, are lightweight and provide faster message delivery compared to other components like file
, jms
, or ftp
. If possible, try to use lightweight components whenever feasible.
However, keep in mind that the choice of components should also align with your specific use case and requirements. For instance, if you need persistent message storage or guaranteed message delivery, heavier components like jms
or ftp
may be the better choice.
2. Optimize Message Filtering
Message filtering is an essential aspect of Camel routes, and improper filtering can significantly impact route performance. Avoid using heavyweight filtering expressions that require extensive computation or involve external dependencies. Instead, try to use simple and efficient expressions or predicates to filter messages.
Additionally, consider using Static Expression Languages (SEL) instead of Dynamic Expression Languages (DEL) wherever possible. SEL evaluates expressions during route startup, which provides a performance advantage over DEL, which evaluates expressions dynamically.
Here's an example that demonstrates the use of SEL in a Camel route:
from("direct:start")
.filter().simple("${body} contains 'keyword'")
.to("mock:result");
In this example, the simple()
method uses SEL to filter messages based on the presence of a specific keyword in the message body.
3. Leverage Parallel Processing
Parallel processing is a powerful technique to improve the throughput and performance of Camel routes, especially when dealing with large volumes of messages. By processing messages concurrently, you can take advantage of the available computing resources and maximize the overall throughput.
Camel provides various ways to introduce parallel processing in your routes. One option is to use the parallelProcessing()
method, which allows you to specify the level of parallelism. Another option is to use the threads
DSL, which provides fine-grained control over thread pooling and concurrency.
Here's an example that demonstrates the use of parallel processing in a Camel route:
from("direct:start")
.parallelProcessing()
.process(new MyProcessor())
.to("mock:result");
In this example, the parallelProcessing()
method enables parallel processing for the subsequent route steps.
4. Enable Exchange Compression
Compression can be a useful technique to reduce network latency and improve overall route performance, especially when dealing with large payloads. Camel provides built-in support for message compression through the gzip
data format.
To enable compression in your route, you can use the marshal()
and unmarshal()
methods with the gzip
data format. This allows you to compress outgoing messages and decompress incoming messages automatically.
Here's an example that demonstrates the use of message compression in a Camel route:
from("direct:start")
.marshal().gzip()
.to("http://example.com")
.unmarshal().gzip()
.to("mock:result");
In this example, the marshal().gzip()
method compresses the outgoing message, while the unmarshal().gzip()
method decompresses the incoming message.
5. Configure Throttling
Throttling is a technique used to control the rate of message processing to prevent overload and ensure optimal performance. By properly configuring throttling, you can enforce rate limits and protect your system from excessive load.
Camel provides several options for implementing throttling, such as using the throttle()
method or the enrich()
method with a throttle policy. These options allow you to limit the number of messages processed per time unit or introduce a delay between each message.
Here's an example that demonstrates the use of throttling in a Camel route:
from("direct:start")
.throttle(10)
.to("mock:result");
In this example, the throttle(10)
method limits the route to process a maximum of 10 messages per second.
6. Optimize Error Handling
Error handling is an essential aspect of Camel routes, but improper error handling can have a significant impact on performance. When an error occurs, Camel performs additional processing to handle the error, which can add latency and reduce throughput.
To optimize error handling, it's crucial to balance between providing valuable error handling and mitigating performance impact. Avoid excessive use of error handlers, and consider using more lightweight error handlers, such as the noErrorHandler()
method, for routes that do not require complex error handling.
Additionally, you can configure error handling at the route level or the individual route steps level, depending on your requirements. Fine-tuning error handling can help reduce unnecessary processing and improve overall route performance.
7. Enable Caching
Caching is a powerful technique to improve the performance of Camel routes, especially when dealing with expensive operations or frequently accessed data. By caching results, you can reduce redundant processing and enhance route efficiency.
Camel provides built-in support for caching through its various caching components, such as ehcache
, JCache
, or Spring Cache
. By configuring the caching mechanism, you can cache results at different levels, such as route-level, endpoint-level, or message-level.
Here's an example that demonstrates the use of caching in a Camel route using the ehcache
component:
from("direct:start")
.to("ehcache:myCache")
.to("mock:result");
In this example, the ehcache:myCache
component caches the route result, allowing subsequent invocations to retrieve the result from the cache without reprocessing.
8. Monitor and Measure Performance
Monitoring and measuring the performance of your Camel routes is essential for identifying bottlenecks and tuning opportunities. By analyzing performance metrics, you can gain insights into resource utilization, message throughput, latency, and other relevant indicators.
Camel provides a comprehensive monitoring framework, which allows you to collect performance metrics from your routes. You can configure metrics collection using built-in components like Metrics
or integrate with monitoring systems like JMX
or Prometheus
for more advanced monitoring.
Additionally, proper logging and tracing can also help diagnose performance issues. By enabling debug logging or using tools like Apache ActiveMQ Artemis
or Wireshark
for message tracing, you can observe the message flow and identify any unusual patterns or delays.
A Final Look
Performance tuning is a crucial aspect of developing efficient and scalable Camel routes. By following these key performance tuning tips, you can optimize your routes and achieve better throughput and lower latency. Remember to analyze specific bottlenecks, experiment with different configurations, and continually monitor the performance of your Camel applications for continuous improvement.
For more information on Apache Camel and performance optimization, refer to the official Camel documentation. Happy routing!