Mastering Spring Beans: Singleton vs. Request vs. Session
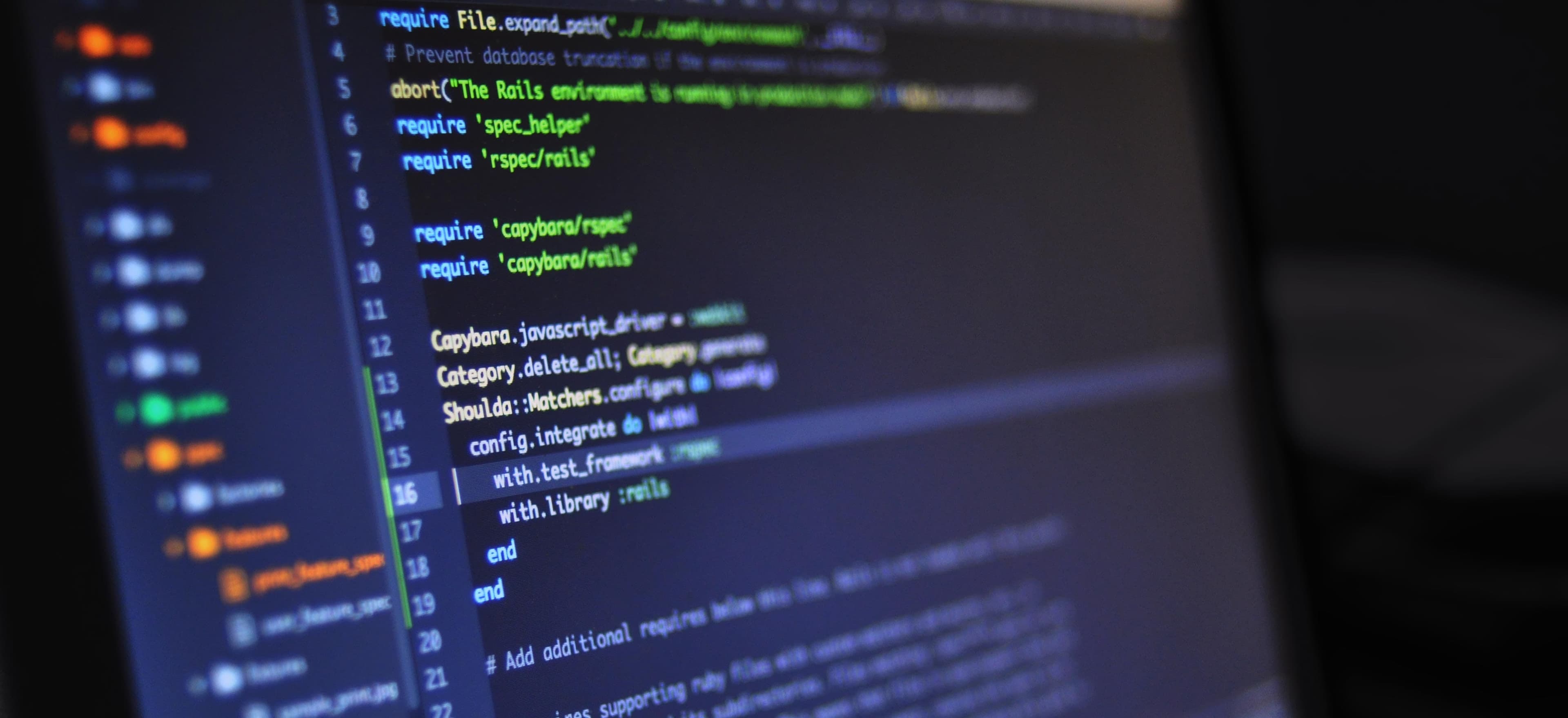
- Published on
Mastering Spring Beans: Singleton vs. Request vs. Session
When working with Spring framework, understanding the different scopes of beans is crucial. Spring beans can be created with different scopes, such as singleton, request, and session. Each scope determines how long a bean instance will be available and how it will be shared across multiple requests or sessions. In this blog post, we will dive deep into these three scopes and explore their use cases, advantages, and disadvantages.
1. Singleton Scope
By default, Spring beans are singleton scoped. This means that a single instance of the bean is created for the entire application context. Whenever a bean is requested, Spring returns the same instance. The singleton scope is suitable for stateless beans that can be safely shared across multiple threads and requests.
Advantages of Singleton Scope
The advantages of using singleton scope for your beans are:
- Efficiency: Since only one instance of a bean is created, it reduces memory consumption and improves performance by reusing the same instance for multiple requests.
- Consistency: With a singleton bean, you can ensure that all components using the bean are working with the same instance and sharing the same state.
- Easy Dependency Management: When a bean is a singleton, you can easily inject it into other beans without worrying about managing multiple instances.
Disadvantages of Singleton Scope
While singleton scope offers many advantages, it also has a few considerations:
- Thread Safety: If a singleton bean maintains mutable state, you need to consider thread safety. Multiple threads accessing and modifying the same state can lead to race conditions and data inconsistencies. Proper synchronization mechanisms like locks or atomic operations should be used to ensure thread safety.
- Limited Flexibility: Singleton beans are created when the application starts and remain in memory until the application shuts down. This may not be ideal for beans that are expensive to create or need to be recreated frequently.
@Configuration
public class AppConfig {
@Bean
public MySingletonBean mySingletonBean() {
return new MySingletonBean();
}
}
In the above example, MySingletonBean
is a singleton bean. Whenever this bean is injected elsewhere in the application, the same instance will be provided.
2. Request Scope
The request scope is used when you want a new instance of a bean for each individual HTTP request. This scope is useful when dealing with web applications, where each user request should have its own instance of a bean.
In Spring MVC, you can define a bean with request scope using the @Scope
annotation and specifying request
as the scope value.
Advantages of Request Scope
The advantages of using a request scope for your beans are:
- Isolation: Each request receives its own instance of the bean, ensuring that the state is not shared among different requests. This avoids any potential data conflicts between concurrent requests.
- Flexibility: Since each request gets its own instance, you can safely modify the state of the request-scoped bean without affecting other requests. This can be useful when dealing with user-specific data.
Disadvantages of Request Scope
There are a few considerations when using the request scope:
- Resource Consumption: Creating a new instance of a request-scoped bean for each request can consume additional memory resources, especially if the bean is heavy in terms of memory usage.
- Performance Impact: If your application has a large number of requests, the performance of creating and managing multiple bean instances may be a concern. Understand your application's requirements and load to determine if the request scope is the right choice.
@Configuration
public class AppConfig {
@Bean
@Scope(value = WebApplicationContext.SCOPE_REQUEST, proxyMode = ScopedProxyMode.TARGET_CLASS)
public MyRequestBean myRequestBean() {
return new MyRequestBean();
}
}
In the example above, MyRequestBean
is a request-scoped bean. Spring will create a new instance of this bean for each HTTP request to ensure isolation.
3. Session Scope
The session scope is used when you need a unique instance of a bean for each user session in a web application. In this scope, a new instance of the bean is created and associated with the user's session. Different users accessing the application will have their own corresponding bean instances.
Similar to the request scope, you can define a bean with session scope using the @Scope
annotation and specifying session
as the scope value.
Advantages of Session Scope
The advantages of using session scope for your beans are:
- Session Isolation: Each user session gets its own instance of the bean, ensuring that the state is isolated and not shared across different sessions. This is useful when dealing with user-specific data that should not be visible to other users.
- Session Persistence: With session scope, you can store session-specific data within the bean instance and persist it across multiple requests from the same user.
Disadvantages of Session Scope
There are a few considerations when using the session scope:
- Resource Consumption: Since each user session gets its own instance of the bean, it can consume additional memory resources, especially if the bean is heavy in terms of memory usage. Be mindful of this if you have a large number of active user sessions.
- Session Management: Managing session-scoped beans can be complex, especially when dealing with session timeouts, session invalidation, and session replication across multiple servers. Ensure that session management is properly handled in your application.
@Configuration
public class AppConfig {
@Bean
@Scope(value = WebApplicationContext.SCOPE_SESSION, proxyMode = ScopedProxyMode.TARGET_CLASS)
public MySessionBean mySessionBean() {
return new MySessionBean();
}
}
In the example above, MySessionBean
is a session-scoped bean. Each user session will have its own instance of this bean.
My Closing Thoughts on the Matter
Understanding the different scopes of Spring beans is essential for efficient and scalable application development. By choosing the appropriate scope for your beans, you can ensure optimal resource utilization and avoid potential data conflicts.
Singleton scope provides efficiency and consistency, but requires attention to thread safety and limited flexibility. Request scope offers isolation and flexibility for web applications, but may consume additional resources. Session scope provides session isolation and persistence for user-specific data, but requires careful management.
Choose the scope that best suits the requirements of your application and consider the trade-offs involved. With a good understanding of bean scopes, you can master Spring beans and build robust and efficient applications.
For more information, refer to the official Spring documentation on Bean Scopes.
Happy coding with Spring!