Mastering Cascade Saves in Spring Data MongoDB & @DBRef
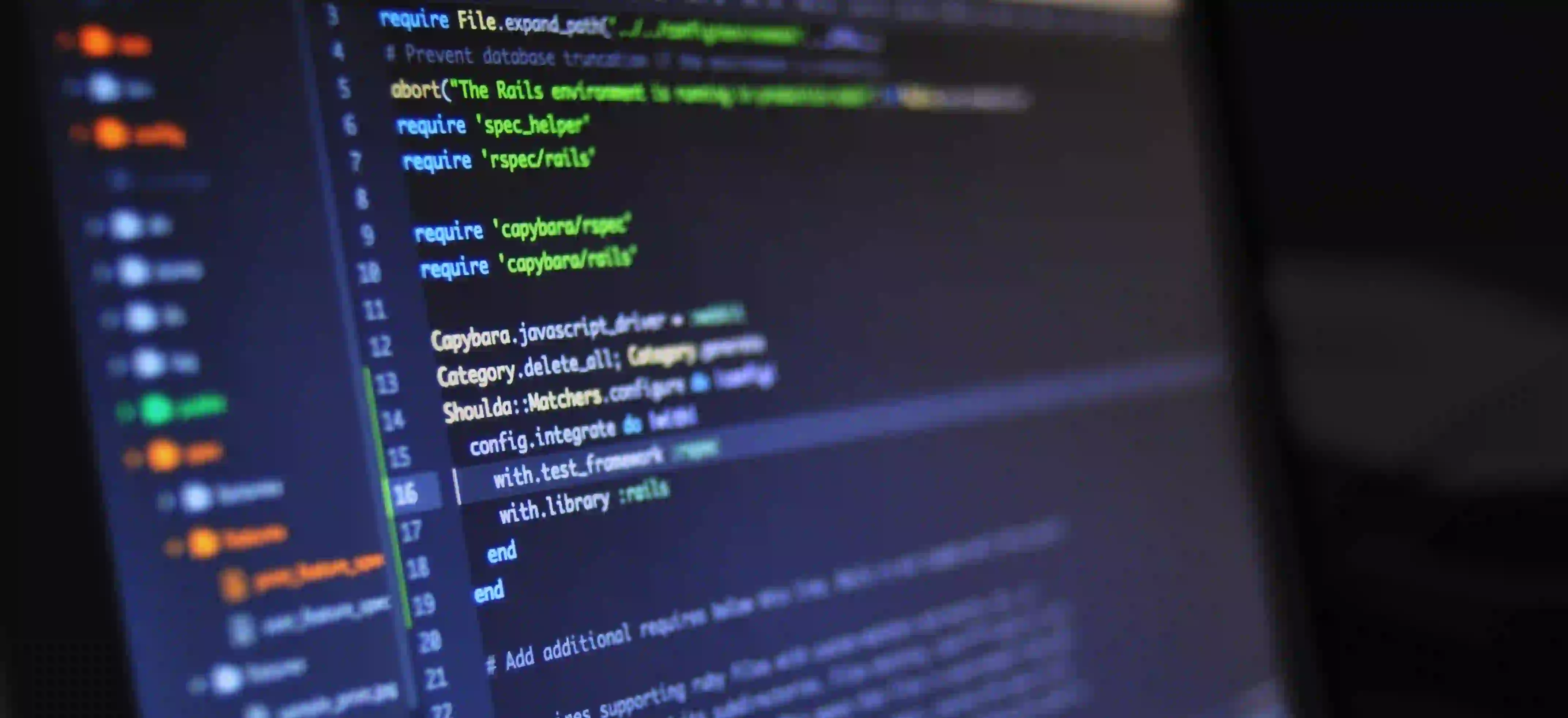
Mastering Cascade Saves in Spring Data MongoDB & @DBRef
In this blog post, we will explore how to leverage the power of cascade saves in Spring Data MongoDB along with the @DBRef annotation. Cascade saves allow us to automatically persist related objects when saving an entity. The @DBRef annotation, on the other hand, enables us to establish a relationship between two entities using references. Combining these two features can greatly simplify your data access code and make it more expressive.
Understanding Cascade Saves
Cascade saves are a mechanism that automatically persists related objects when saving an entity. This can be particularly useful when dealing with complex object graphs containing multiple levels of nested entities. Instead of explicitly saving each individual entity, the cascade saves feature allows you to save the entire graph in one go.
Spring Data MongoDB provides two main ways to define a cascade save behavior: through annotations or through the CascadeType
enumeration.
Cascade Saves through Annotations
To use cascade saves through annotations, you can simply annotate the related fields in your entity class with the @CascadeSave
annotation. For example, consider the following entities:
@Document
public class Author {
@Id
private String id;
private String name;
@CascadeSave
private List<Book> books;
// getters and setters
}
@Document
public class Book {
@Id
private String id;
private String title;
// getters and setters
}
In this example, we have an Author
entity which has a list of Book
entities. By annotating the books
field with @CascadeSave
, we are instructing Spring Data MongoDB to automatically save any referenced Book
objects when an Author
object is saved.
Cascade Saves through CascadeType
Alternatively, you can define the cascade save behavior directly in the entity class using the CascadeType
enumeration. This can be done by annotating the field or method with the @Cascade
annotation and specifying the appropriate CascadeType
value.
@Document
public class Author {
@Id
private String id;
private String name;
@Cascade(CascadeType.SAVE_UPDATE)
private List<Book> books;
// getters and setters
}
In this example, we are using the CascadeType.SAVE_UPDATE
option to indicate that any changes made to the books
collection should be persisted when saving the Author
object.
Using @DBRef
The @DBRef annotation is used to establish relationships between entities using references. Instead of embedding objects, you can annotate a field with @DBRef and store a reference to another entity.
To better understand @DBRef and its interaction with cascade saves, let's consider the following example:
@Document
public class Author {
@Id
private String id;
private String name;
@DBRef
private List<Book> books;
// getters and setters
}
@Document
public class Book {
@Id
private String id;
private String title;
// getters and setters
}
In this scenario, we have an Author
entity with a list of Book
entities. By annotating the books
field with @DBRef
, we are instructing Spring Data MongoDB to store a reference to the Book
objects instead of embedding them within the Author
document.
The @DBRef
annotation allows us to establish a relationship between objects across different collections, but it does not enable cascade saves by default. To achieve cascade saves with @DBRef
, we need to combine it with cascade saves as shown in the previous examples.
Implementing Cascade Saves with @DBRef
To implement cascade saves with @DBRef
, we can combine the @CascadeSave
annotation and the @DBRef
annotation. This will enable us to automatically persist the related Book
objects when saving an Author
object.
@Document
public class Author {
@Id
private String id;
private String name;
@CascadeSave
@DBRef
private List<Book> books;
// getters and setters
}
In this example, the @CascadeSave
annotation instructs Spring Data MongoDB to cascade saves to the referenced Book
objects, and the @DBRef
annotation establishes the relationship between the Author
and Book
entities using references.
Now, when you save an Author
object, any changes made to the books
collection will be automatically persisted, thanks to the cascade save behavior. This allows you to simplify your data access code and ensure consistency in your data model.
In Conclusion, Here is What Matters
In this blog post, we have explored how to master cascade saves in Spring Data MongoDB and how to use the @DBRef
annotation to establish relationships between entities using references. By combining cascade saves and @DBRef
, you can simplify your data access code and ensure consistency in your data model.
Cascade saves allow you to automatically persist related objects when saving an entity, eliminating the need for manual saving. The @DBRef
annotation, on the other hand, enables you to establish relationships between entities using references instead of embedding objects.
Understanding cascade saves and @DBRef
gives you the flexibility to handle complex object graphs and establish relationships between entities in a more expressive way. This can greatly enhance the maintainability and readability of your code.
To learn more about Spring Data MongoDB and cascade saves with @DBRef
, refer to the official documentation and explore other resources such as this tutorial and this Stack Overflow thread.
Happy coding!