Securing Spring Boot: Avoid JDBC Pitfalls!
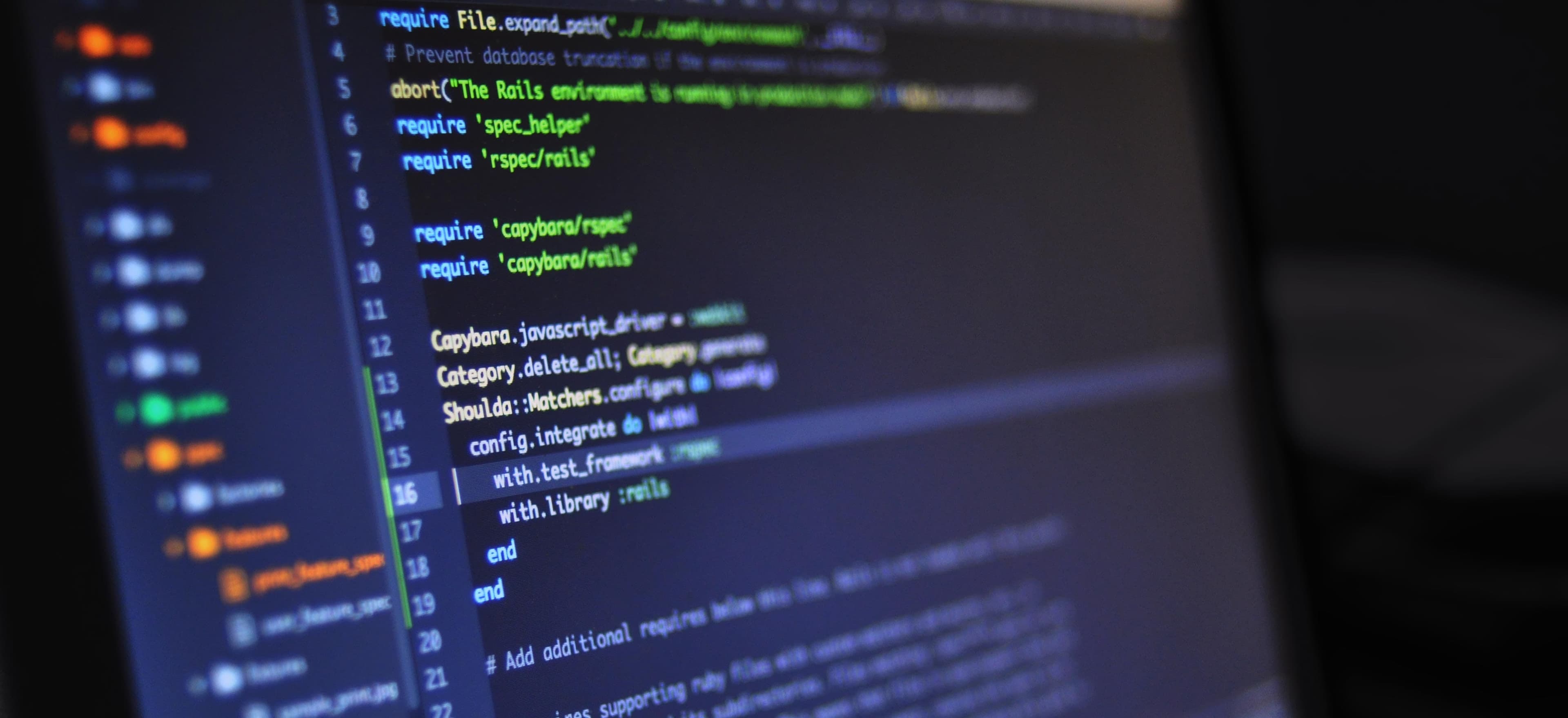
- Published on
Securing Spring Boot: Avoid JDBC Pitfalls!
When it comes to building secure web applications, Spring Boot is a popular choice among developers. With its robust architecture and extensive community support, Spring Boot makes it easy to implement security features, such as user authentication and authorization. However, there are some common pitfalls that developers can fall into when using JDBC for database connectivity in Spring Boot applications. In this article, we will discuss these pitfalls and provide solutions to avoid them.
Pitfall 1: Storing plaintext passwords in the database
One of the biggest security mistakes developers make is storing user passwords in plaintext in the database. Storing passwords in plaintext exposes them to potential attackers, who can easily gain access to the sensitive information. To overcome this issue, Spring Boot provides a convenient way to encrypt passwords using the bcrypt algorithm.
The bcrypt algorithm is widely recognized as a secure hashing algorithm for password storage. It not only encrypts passwords but also adds a salt to the hash, which makes it extremely difficult for attackers to crack the password. To utilize bcrypt in a Spring Boot application, you can use the Spring Security library.
First, add the Spring Security dependency to your pom.xml
or build.gradle
file:
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-security</artifactId>
</dependency>
Next, configure Spring Security to use bcrypt as the password encryption algorithm. In your application.properties
file, add the following property:
spring.security.user.passwordEncoder=bcrypt
Now, when you create new user accounts, make sure to use the BCryptPasswordEncoder
to encode the passwords:
import org.springframework.security.crypto.bcrypt.BCryptPasswordEncoder;
BCryptPasswordEncoder passwordEncoder = new BCryptPasswordEncoder();
String encodedPassword = passwordEncoder.encode("password");
By using bcrypt to store passwords, you ensure that even if the database is compromised, attackers will find it extremely difficult to retrieve the original passwords.
Pitfall 2: Not properly configuring connection pool settings
Another common mistake developers make is not properly configuring connection pool settings. A connection pool is a cache of database connections that can be reused by your application, which helps improve performance. Spring Boot provides the HikariCP connection pool by default, which is known for its excellent performance and reliability.
To configure the connection pool settings in Spring Boot, you can use the spring.datasource
properties in your application.properties
file. For example, to set the maximum number of connections in the pool, you can add the following property:
spring.datasource.hikari.maximum-pool-size=10
It is important to set an appropriate maximum pool size based on the expected load on your application. Setting a very high maximum pool size can lead to resource exhaustion and adversely affect the stability of your application. On the other hand, setting a very low maximum pool size may cause performance degradation during peak usage.
Pitfall 3: Not using parameterized queries
When using JDBC in Spring Boot, it is crucial to use parameterized queries to prevent SQL injection attacks. A SQL injection attack occurs when an attacker is able to manipulate the SQL query being executed by your application, potentially leading to unauthorized access or data loss.
To illustrate the importance of parameterized queries, consider the following example:
String username = request.getParameter("username");
String password = request.getParameter("password");
String query = "SELECT * FROM users WHERE username='" + username + "' AND password='" + password + "'";
In the above example, the username and password values are concatenated directly into the SQL query, making it vulnerable to SQL injection attacks. An attacker could pass a malicious value for either the username or password parameter and manipulate the query to execute arbitrary SQL statements.
To prevent SQL injection attacks, you should use parameterized queries in JDBC. Parameterized queries separate the SQL code from the user input by using placeholders, and then binding the user input to those placeholders. Here's an example of using parameterized queries in Spring Boot:
String query = "SELECT * FROM users WHERE username=? AND password=?";
PreparedStatement statement = connection.prepareStatement(query);
statement.setString(1, username);
statement.setString(2, password);
ResultSet resultSet = statement.executeQuery();
In the above example, the placeholders ?
are used to represent the username and password values. The values are then bound to the placeholders using the setString()
method. By using parameterized queries, you eliminate the risk of SQL injection attacks, as the database engine treats the user input as data rather than executable code.
Pitfall 4: Not closing database resources properly
Not closing database resources properly can lead to resource leaks and potential security vulnerabilities. In JDBC, it is important to close database connections, statements, and result sets to free up system resources and ensure the security and performance of your application.
A common mistake is not closing the resources in a finally
block, which can result in resource leaks if an exception occurs. To avoid this pitfall, you can use the try-with-resources construct introduced in Java 7. The try-with-resources statement ensures that the resources are closed even if an exception is thrown. Here's an example:
try (Connection connection = dataSource.getConnection();
PreparedStatement statement = connection.prepareStatement(query);
ResultSet resultSet = statement.executeQuery()) {
// Process the result set
} catch (SQLException e) {
// Handle the exception
}
In the above example, the Connection
, PreparedStatement
, and ResultSet
resources are automatically closed when the try block is exited, whether normally or due to an exception. This approach not only simplifies the code but also guarantees that the resources are properly released, regardless of the execution path.
The Bottom Line
Securing Spring Boot applications with JDBC requires careful attention to details. By following best practices, such as using bcrypt for password encryption, configuring connection pool settings correctly, using parameterized queries, and properly closing database resources, you can greatly enhance the security and performance of your Spring Boot applications.
Remember, securing your application is an ongoing process. Stay up to date with the latest security best practices, regularly patch your dependencies, and conduct thorough security testing to identify and fix any vulnerabilities. With the right security measures in place, you can build robust and secure Spring Boot applications.
Happy coding!
Resources: