Master the Chaos: Ensuring Data Consistency in Microservices
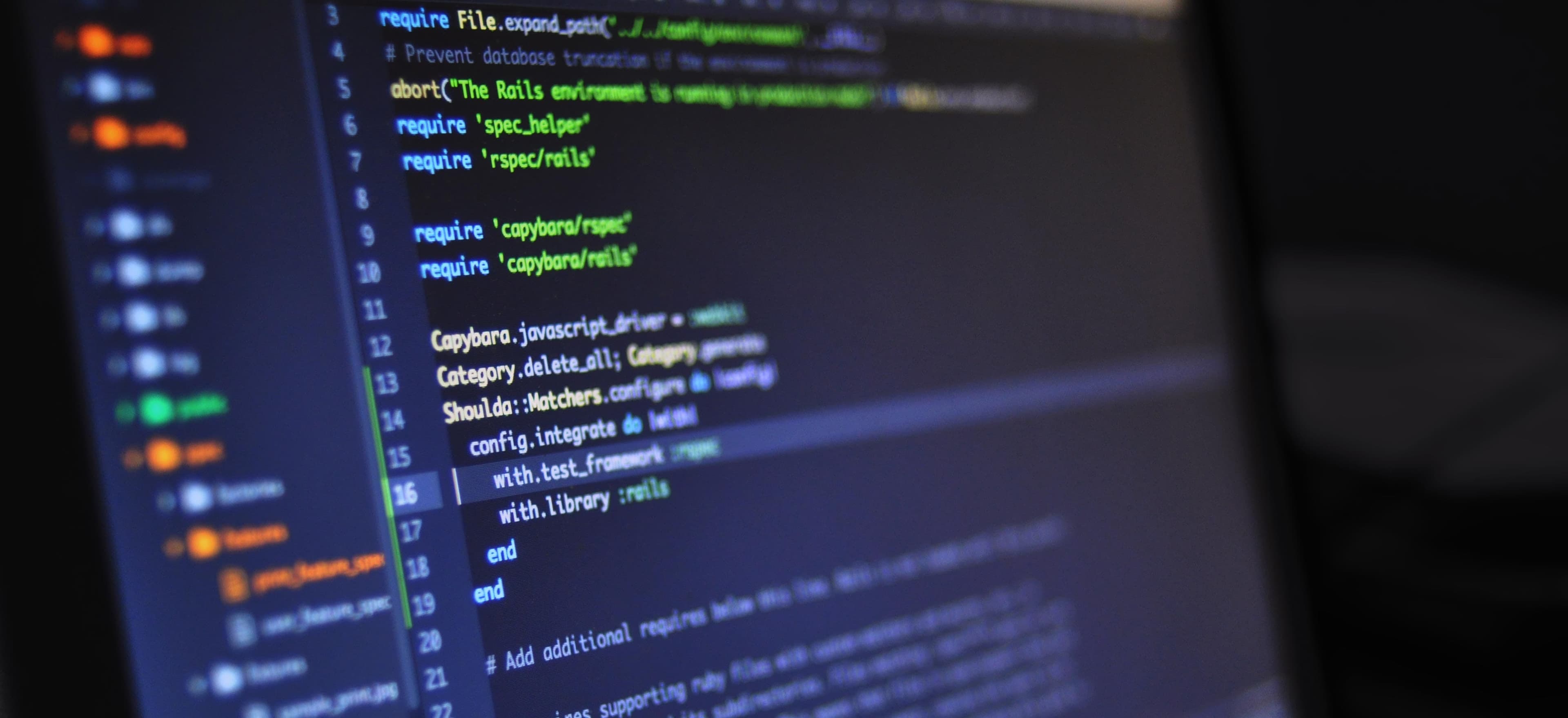
- Published on
Master the Chaos: Ensuring Data Consistency in Microservices
Microservices architecture has gained immense popularity due to its scalability, agility, and flexibility. However, it brings its own set of challenges, particularly when it comes to ensuring data consistency across multiple services. In this article, we will explore strategies to tackle this issue specifically in the context of Java-based microservices.
Understanding the Challenge
In a microservices environment, each service is responsible for its own data storage, often using different databases. This leads to the problem of maintaining data consistency across services. For example, when a transaction involves multiple services, ensuring that all data is successfully updated or rolled back in case of failure becomes a challenging task.
Event Sourcing and CQRS
One popular approach to tackle data consistency in microservices is through Event Sourcing and Command Query Responsibility Segregation (CQRS). In Event Sourcing, all changes to the application state are captured as a sequence of events. These events are then stored in an event store, which serves as the system of record.
CQRS, on the other hand, separates the read and write operations for a data store. It uses separate models to update and retrieve data. This clear separation makes it easier to maintain consistency between different data stores.
Using Java and Kafka for Event Sourcing
Java, being a versatile and widely used programming language, provides excellent support for implementing Event Sourcing and CQRS patterns. When it comes to managing the streams of events, Apache Kafka, a distributed streaming platform, plays a pivotal role.
Let's take a look at a simplified example of how Java and Kafka can be used for event sourcing to ensure data consistency in a microservices architecture.
Example: Event Sourcing with Kafka in Java
public class EventProducer {
private final KafkaProducer<String, String> producer;
public EventProducer() {
Properties props = new Properties();
props.put("bootstrap.servers", "localhost:9092");
props.put("key.serializer", "org.apache.kafka.common.serialization.StringSerializer");
props.put("value.serializer", "org.apache.kafka.common.serialization.StringSerializer");
producer = new KafkaProducer<>(props);
}
public void sendEvent(String topic, String event) {
producer.send(new ProducerRecord<>(topic, event));
}
public void close() {
producer.close();
}
}
In this example, we have a simple EventProducer
class that utilizes the Kafka producer to send events to a Kafka topic.
Now let's take a look at the consumer side:
public class EventConsumer {
private final KafkaConsumer<String, String> consumer;
public EventConsumer() {
Properties props = new Properties();
props.put("bootstrap.servers", "localhost:9092");
props.put("group.id", "test-group");
props.put("key.deserializer", "org.apache.kafka.common.serialization.StringDeserializer");
props.put("value.deserializer", "org.apache.kafka.common.serialization.StringDeserializer");
consumer = new KafkaConsumer<>(props);
}
public void consumeEvents(String topic) {
consumer.subscribe(Collections.singletonList(topic));
while (true) {
ConsumerRecords<String, String> records = consumer.poll(Duration.ofMillis(100));
for (ConsumerRecord<String, String> record : records) {
// Process the event
System.out.println("Received event: " + record.value());
}
}
}
public void close() {
consumer.close();
}
}
In this example, the EventConsumer
class subscribes to a Kafka topic and processes the received events.
In a real-world scenario, the events captured by the producer and consumed by the consumer can represent the state changes in different microservices, ensuring eventual consistency across the system.
To Wrap Things Up
In the world of microservices, ensuring data consistency is a critical aspect that should not be overlooked. Event Sourcing and CQRS, along with tools like Kafka, provide robust patterns and technologies to address this challenge.
By leveraging the capabilities of Java and Kafka, developers can effectively design and implement solutions that guarantee data consistency in a distributed and scalable microservices environment.
In conclusion, mastering the chaos of data consistency in microservices is achievable through the thoughtful application of proven patterns and technologies.
To delve deeper into the topic of event-driven architectures and data consistency in microservices, consider exploring Building Microservices: Designing Fine-Grained Systems by Sam Newman, an insightful book that provides comprehensive insights into building resilient and scalable microservice-based systems.
For practical implementation guidance and in-depth understanding of Apache Kafka, refer to the official Apache Kafka documentation.
Mastering data consistency in microservices is not an easy feat, but with the right knowledge and tools, it becomes an achievable endeavor. Understanding the principles and adopting suitable technologies is the key to navigating the complexities of distributed systems and ensuring the reliability and consistency of data.