Achieving Idempotency: Navigating Temporal Challenges
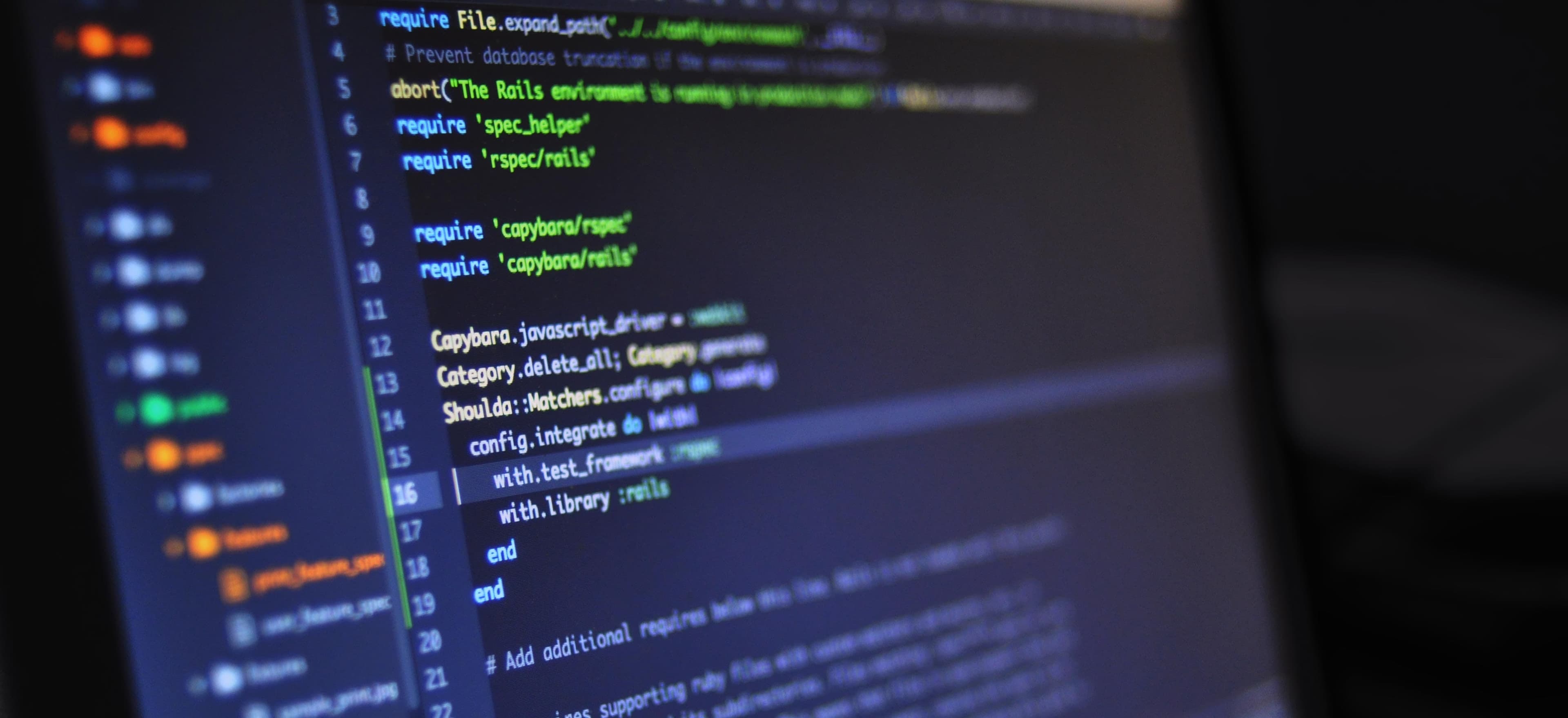
- Published on
Achieving Idempotency: Navigating Temporal Challenges in Java Applications
In the fast-paced world of modern software engineering, ensuring predictability and consistency in distributed systems is a critical challenge. This is particularly true in the realm of Java applications, where developers often grapple with idempotency – a fundamental concept in building reliable and resilient systems. In this article, we will delve into the temporal challenges Java developers face when implementing idempotency and explore strategies to overcome these obstacles.
Understanding Idempotency
Definition and Real-world Application
Idempotency is a concept in computer science and mathematics that describes the property of certain operations where the result remains the same upon multiple executions. In the context of software development, idempotency ensures that performing an action multiple times has the same effect as performing it once. This concept is pivotal in guaranteeing consistency and reliability, especially in distributed systems where retry mechanisms and duplicated requests are common.
One real-world application of idempotency can be observed in payment processing systems. When a payment transaction is initiated, it's crucial that the system can handle retries or duplicates without inadvertently charging a customer multiple times or causing inconsistencies in financial records. Another common application is in RESTful APIs, where idempotent operations ensure that repeated requests to the same endpoint yield consistent outcomes.
Challenges in Ensuring Idempotency
Implementing idempotency poses several challenges for developers, particularly in the realm of temporal aspects. Clock skew and event ordering are two primary culprits that complicate the task of achieving idempotency in distributed systems.
Clock skew refers to the variation in time between different system clocks or components within a distributed system. When events are processed or transactions are executed across multiple nodes with slightly out-of-sync clocks, ensuring idempotency becomes significantly more complicated. Event ordering, on the other hand, pertains to the sequencing of events in distributed systems. In scenarios where events can arrive out of order or where network delays can cause discrepancies in event timelines, maintaining idempotency becomes a non-trivial endeavor.
The Temporal Challenges
Clock Skew and Event Ordering
To better understand the impact of clock skew and event ordering on idempotency, let's consider a simplified scenario. Imagine a distributed system where multiple nodes process incoming requests and update a shared database. Due to clock skew, the timestamps associated with these requests might not perfectly align. Consequently, when determining the idempotency of a given request, the system must account for these temporal discrepancies to avoid unintended side effects.
Event ordering, on the other hand, introduces complexities in orchestrating the processing of received events. As events traverse the network, they may encounter varying latencies, leading to inconsistencies in their arrival order at different nodes. Ensuring idempotency in such an environment requires careful consideration of event timestamps and sequence identifiers to disambiguate potentially out-of-order events.
Handling Temporal Challenges in Java
Java offers robust capabilities and libraries to tackle the temporal challenges associated with implementing idempotency in distributed systems. Leveraging Java's date and time APIs, such as the java.time package introduced in Java 8, developers can effectively manage timestamps and reconcile temporal variations across the system. Additionally, third-party libraries like Apache Commons Lang provide utility classes for manipulating timestamps and addressing clock skew issues.
Beyond low-level temporal handling, Java frameworks like Spring provide features for implementing idempotent services and managing retries in distributed environments. Leveraging these features, developers can design robust and resilient components capable of withstanding the temporal challenges innate to distributed systems.
Implementing Idempotency in Java Applications
Idempotency Patterns
In Java applications, several patterns can be employed to achieve idempotency. One common approach involves utilizing unique keys or request identifiers to distinguish between unique and duplicate requests. By associating each request with a globally unique identifier, the system can discern between distinct requests and redundancies. Additionally, leveraging database constraints, such as unique indexes and primary keys, can enforce idempotency at the persistence layer, ensuring that duplicate records are not inadvertently created.
Another effective pattern is the use of idempotent receivers, which are designed to handle incoming requests such that their idempotent nature is preserved. By encapsulating the idempotency logic within the receiver, the system can abstract the complexities of temporal challenges away from the application logic, simplifying the overall implementation.
// Example of using a unique key to achieve idempotency
public class IdempotentService {
public void processRequest(Request request) {
if (!isRequestProcessed(request.getId())) {
// Process the request
markRequestAsProcessed(request.getId());
} else {
// Request has already been processed
}
}
}
Leveraging Java Libraries and Frameworks
Java boasts a rich ecosystem of libraries and frameworks that streamline the implementation of idempotency. For instance, Spring Framework provides support for implementing idempotent services through its resilient and fault-tolerant features. By incorporating Spring's retry and circuit breaker capabilities, developers can design robust services that gracefully handle temporal challenges and ensure consistency in the face of retries.
Additionally, Hibernate, the popular Java Object-Relational Mapping (ORM) framework, offers mechanisms for managing idempotency at the data access layer. By defining unique constraints and utilizing Hibernate's transaction management features, developers can enforce idempotency constraints at the database level, safeguarding against duplicate data and inconsistent states.
Case Study: Achieving Idempotency in a Java-Based Payment System
Let's consider a hypothetical case study to illustrate the practical application of idempotency principles in a Java-based payment system. In our scenario, the payment system encounters intermittent network delays, resulting in requests reaching the processing nodes out of order. To address this, the development team leverages unique request identifiers and employs idempotent receivers to ensure that payment processing remains consistent regardless of event ordering.
// Example of using idempotent receivers in a Java-based payment system
public class PaymentProcessor {
private Set<String> processedRequests = new HashSet<>();
public void processPayment(Request request) {
if (!processedRequests.contains(request.getId())) {
// Process the payment
markRequestAsProcessed(request.getId());
} else {
// Payment request has already been processed
}
}
private void markRequestAsProcessed(String requestId) {
// Update the processing logs or database
processedRequests.add(requestId);
}
}
In this case study, the team successfully mitigates the impact of event ordering by implementing idempotent receivers, ensuring that payment requests are processed consistently despite temporal challenges.
The Closing Argument
In navigating temporal challenges to achieve idempotency in Java applications, developers must confront the intricacies of clock skew, event ordering, and distributed processing. By leveraging Java's built-in capabilities and adopting effective idempotency patterns, such as using unique keys and idempotent receivers, developers can address these challenges and build reliable and resilient systems. Through the case study of a Java-based payment system, we've seen how these principles can be applied in practice to ensure consistent outcomes in the face of temporal fluctuations.
In closing, it's evident that achieving idempotency is paramount in ensuring the robustness and predictability of Java applications, particularly in the context of distributed systems. By embracing the principles and patterns outlined in this article, developers can fortify their applications against temporal challenges and lay the groundwork for stable and reliable systems.
Additional Resources
For further exploration, consider the following resources:
By diving deeper into these resources, readers can expand their understanding of idempotency and explore additional strategies for navigating temporal challenges in Java applications.
In crafting this article, the aim was to provide an engaging and informative exploration of idempotency and its relevance in ensuring the robustness of Java applications. By seamlessly integrating practical examples, code snippets, and actionable insights, the article endeavors to equip readers with the knowledge and tools to tackle the temporal challenges inherent in distributed systems.