Boost Your Test Automation: Enhancing JUnit 5 & Selenium Setup
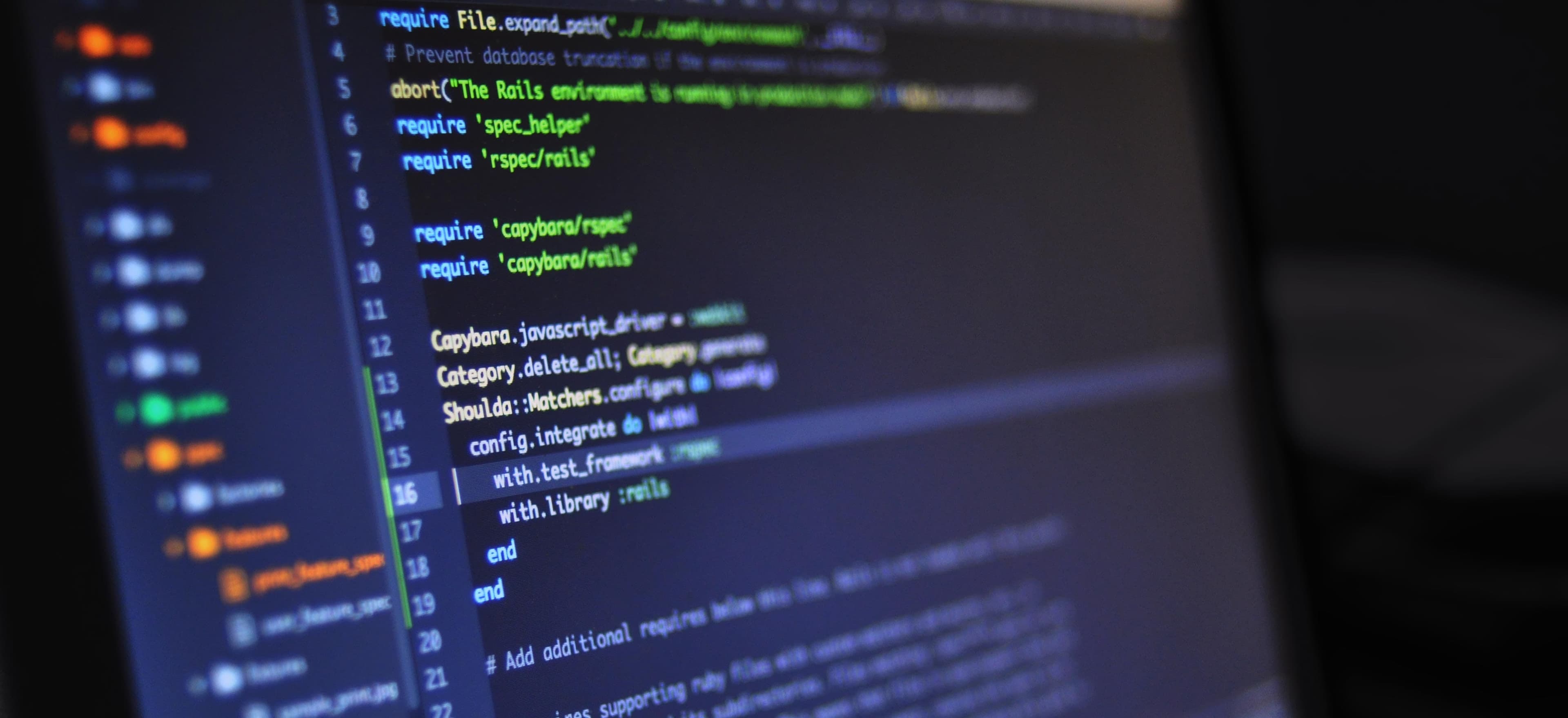
- Published on
Boost Your Test Automation: Enhancing JUnit 5 & Selenium Setup
The Starting Line
In the ever-evolving landscape of software development, test automation has become an essential practice for ensuring the quality and robustness of applications. Among the myriad of tools available, JUnit 5 and Selenium stand out as popular choices for implementing automated tests. In this article, we will delve into the nuances of JUnit 5 and Selenium, and explore how to enhance their setup for more efficient test automation.
Understanding JUnit 5 and Selenium
JUnit 5 Overview
JUnit 5, the latest version of the widely-used testing framework for Java, comes with a plethora of features and improvements over its predecessors. Its modular architecture consists of three main components - the JUnit Platform, JUnit Jupiter, and JUnit Vintage, enabling developers to write cleaner, more modular tests and extensions.
Selenium Overview
Selenium is a powerful tool for automating browser actions to test web applications. At the heart of Selenium is the WebDriver, which provides a programming interface for interacting with web browsers. Its ability to simulate user interactions with web elements makes it an indispensable asset for automated web application testing.
Why Use JUnit 5 with Selenium?
Combining JUnit 5 with Selenium yields numerous benefits. The seamless integration provides enhanced test organization, the ability to run tests across different browsers, and support for advanced test patterns such as parameterized tests.
Setting Up JUnit 5 and Selenium
Pre-requisites
Before diving into the setup, ensure that the necessary tools and versions are available, including Java SDK, JUnit 5, Selenium WebDriver, and build automation tools like Maven or Gradle.
Maven/Gradle Configuration
To include JUnit 5 and Selenium dependencies, here's a snippet for Maven configuration:
<dependencies>
<dependency>
<groupId>org.junit.jupiter</groupId>
<artifactId>junit-jupiter-api</artifactId>
<version>5.8.1</version>
<scope>test</scope>
</dependency>
<!-- Include Selenium dependencies here -->
</dependencies>
For Gradle, add the following to the build.gradle file:
dependencies {
testImplementation 'org.junit.jupiter:junit-jupiter-api:5.8.1'
// Include Selenium dependencies here
}
Initializing WebDriver
Initializing the Selenium WebDriver is pivotal for browser automation within JUnit 5 test classes. Whether it's Chrome, Firefox, or any other browser, specifying system properties for the WebDriver executables is crucial to ensure seamless interaction.
@BeforeEach
void setUp() {
WebDriverManager.chromedriver().setup(); // For Chrome
// Initialize other drivers as needed
driver = new ChromeDriver();
}
Enhancing JUnit 5 and Selenium Setup
Parameterized Tests with JUnit 5
Harnessing the power of parameterized tests in JUnit 5 allows for the execution of the same test case with different inputs, including various browser types during Selenium test execution.
@ParameterizedTest
@ValueSource(strings = {"chrome", "firefox"})
void testPageLoadSpeed(String browser) {
// Instantiate WebDriver based on browser type and perform tests
}
Effective Use of Assertions
Leveraging JUnit 5's rich set of assertion methods such as assertEquals()
and assertTrue()
enhances the validation process, ensuring the reliability of test outcomes.
@Test
void testHomePageTitle() {
String expectedTitle = "Example Title";
String actualTitle = driver.getTitle();
assertEquals(expectedTitle, actualTitle);
}
Implementing Page Object Model (POM)
The Page Object Model design pattern plays a crucial role in Selenium tests, promoting reusability and reducing maintenance overhead. By encapsulating web elements and their interactions, the POM brings clarity and organization to the test code.
public class LoginPage {
private WebDriver driver;
public LoginPage(WebDriver driver) {
this.driver = driver;
// Instantiate other web elements
}
public void enterUsername(String username) {
// Method implementation
}
// Other methods to interact with the page elements
}
Utilizing JUnit 5 Extensions
JUnit 5's extensions provide a way to extend the framework's functionality, facilitating custom lifecycle management and conditional test execution. For instance, managing WebDriver instance lifecycle can be streamlined using extensions.
public class WebDriverExtension implements BeforeAllCallback, AfterAllCallback {
private WebDriver driver;
@Override
public void beforeAll(ExtensionContext context) {
driver = new ChromeDriver();
}
@Override
public void afterAll(ExtensionContext context) {
driver.quit();
}
}
Continuous Integration Setup
Integrating automated tests into a Continuous Integration (CI) pipeline, such as Jenkins or GitHub Actions, ensures that tests are automatically run with every code change, guaranteeing the robustness of the application throughout its development lifecycle.
Best Practices for JUnit 5 and Selenium Tests
In addition to the technical setup and configurations, here are some best practices that should be embraced to harness the full potential of JUnit 5 and Selenium in test automation:
- Organize tests into logical suites
- Keep tests independent and isolated
- Use meaningful test names for clarity
- Optimize test speed by leveraging parallel execution where applicable
Bringing It All Together
In conclusion, enhancing the setup of JUnit 5 and Selenium for test automation offers a myriad of advantages – from simplified test organization and reusable test code through the POM to seamless integration into CI pipelines. By integrating the best practices and techniques discussed in this article, developers can significantly elevate the efficiency and reliability of their test automation projects.
Experiment with these tips and tricks to experience firsthand the transformative impact they can have on your test automation endeavors.
Relevant Links and Resources
For further reading and deepening your understanding of JUnit 5, Selenium WebDriver, and related technologies, here are some valuable resources:
- JUnit 5 Documentation
- Selenium Documentation
- GitHub Repository for Sample JUnit 5 and Selenium Projects
- Tutorials on Page Object Model in Selenium
- Guide to Continuous Integration with Jenkins
Remember, the true essence of enhancing test automation lies not just in the 'how' but also in understanding the 'why' behind each technique. This comprehensive understanding paves the way for building robust, efficient, and maintainable test suites.