Mastering Test Doubles: Navigating Mocks, Spies & Stubs
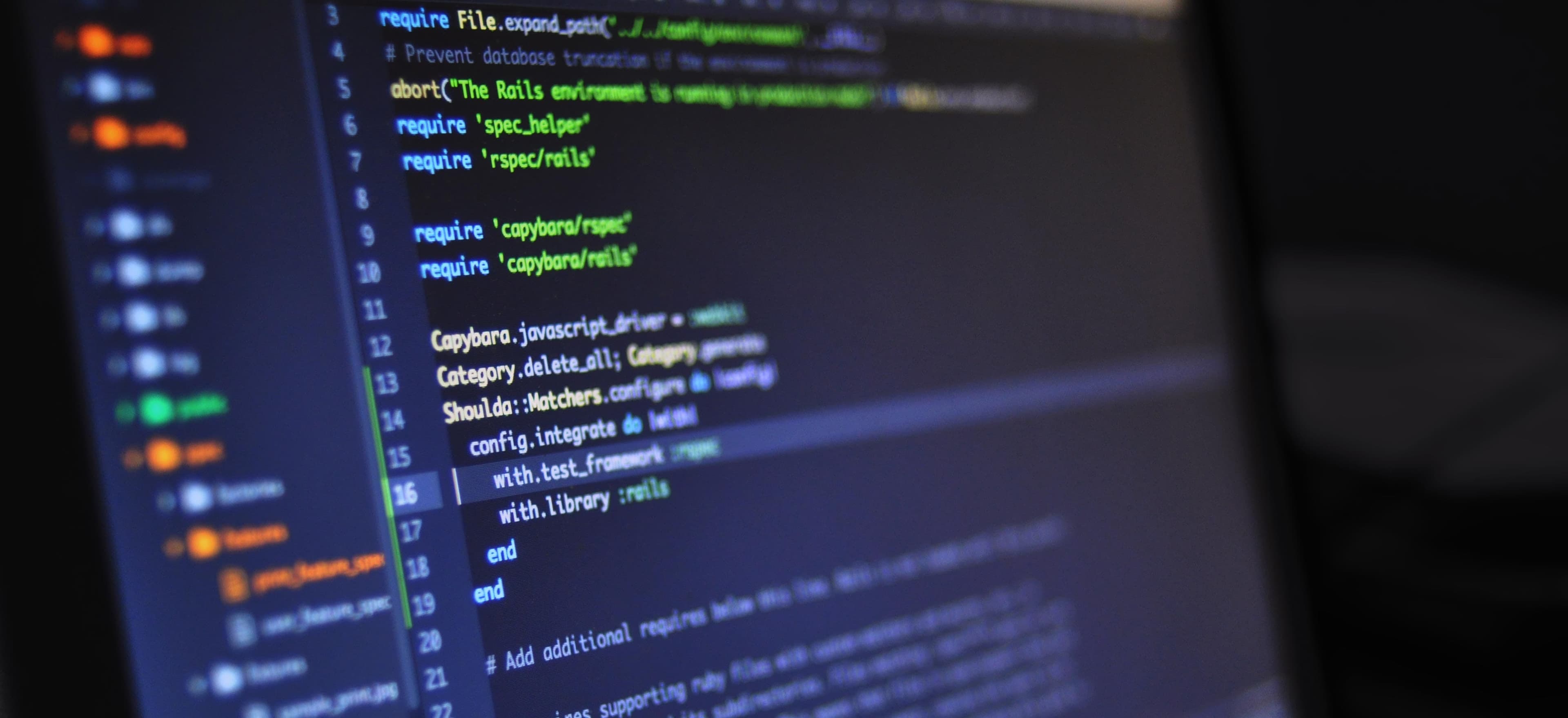
- Published on
Mastering Test Doubles: Navigating Mocks, Spies & Stubs
As a Java developer, writing high-quality code is not limited to just the application logic. Ensuring that the code is thoroughly tested is equally important. Test-driven development (TDD) and writing unit tests are crucial aspects of modern software development. In this blog post, we will delve into the world of test doubles in Java, exploring mocks, spies, and stubs, and how they can be effectively used to write robust and maintainable tests.
Understanding Test Doubles
Before we dive into the specifics, let's establish a common understanding of what test doubles are. Test doubles are objects that are used in unit tests as stand-ins for real components. They are used to isolate the code under test and simulate the behavior of real objects. The three main types of test doubles are mocks, spies, and stubs.
Mocks
Mocks are objects that simulate the behavior of real objects in controlled ways. They are used to verify interactions between the code under test and the dependencies. Mocking frameworks like Mockito and EasyMock provide convenient APIs for creating mocks and specifying expectations on them.
Spies
Spies are similar to mocks, but they allow you to track and verify the interactions with the real objects. When using spies, the actual methods of the real object are called, while still allowing you to make assertions on their behavior. This can be useful when you want to test how the code under test interacts with a real object without fully replacing it with a mock.
Stubs
Stubs are objects that provide canned responses to calls made during the test. They are used to emulate the behavior of real objects and specify the return values for specific inputs. Stubs are typically used when the real dependencies are either slow, non-deterministic, or not easily accessible in a testing environment.
Now that we have a clear understanding of the different types of test doubles, let's explore how they can be effectively utilized in Java unit testing.
Using Mockito for Mocking
Mockito is a popular mocking framework for Java that provides a fluent API for creating and interacting with mocks. Let's consider a simple example to demonstrate how Mockito can be used for mocking dependencies.
Suppose we have a UserService
that depends on a UserRepository
to fetch user details. We want to test the UserService
, but we don't want to interact with the actual UserRepository
during the test.
public class UserService {
private UserRepository userRepository;
public UserService(UserRepository userRepository) {
this.userRepository = userRepository;
}
public String getUserFullName(Long userId) {
User user = userRepository.findById(userId);
return user != null ? user.getFullName() : "User not found";
}
}
In our test for the UserService
, we can use Mockito to create a mock UserRepository
and specify its behavior.
import static org.mockito.Mockito.*;
public class UserServiceTest {
@Test
public void testGetUserFullName() {
UserRepository userRepository = mock(UserRepository.class);
when(userRepository.findById(1L)).thenReturn(new User(1L, "John Doe"));
UserService userService = new UserService(userRepository);
String fullName = userService.getUserFullName(1L);
assertEquals("John Doe", fullName);
verify(userRepository, times(1)).findById(1L);
}
}
In this example, we created a mock UserRepository
using Mockito.mock()
and used when().thenReturn()
to specify the behavior of the mock when its findById()
method is invoked. We then tested the UserService
using the mock UserRepository
, asserting the expected behavior and verifying the interactions with the mock.
Leveraging Spies for Interaction Testing
Spies can be particularly useful when you want to verify interactions with real objects. Let's consider an example where we want to test the interaction between a NotificationService
and a real EmailSender
.
public class NotificationService {
private EmailSender emailSender;
public NotificationService(EmailSender emailSender) {
this.emailSender = emailSender;
}
public void sendNotification(String recipient, String message) {
emailSender.sendEmail(recipient, message);
}
}
In the test for the NotificationService
, we can use Mockito to create a spy on the real EmailSender
and verify its interaction.
public class NotificationServiceTest {
@Test
public void testSendNotification() {
EmailSender emailSender = new EmailSender(); // This is the real object
EmailSender emailSenderSpy = spy(emailSender);
NotificationService notificationService = new NotificationService(emailSenderSpy);
notificationService.sendNotification("user@example.com", "Test notification");
verify(emailSenderSpy, times(1)).sendEmail("user@example.com", "Test notification");
}
}
In this example, we created a spy on the real EmailSender
using spy()
and then used verify()
to assert that the sendEmail()
method was called with the expected arguments.
Emulating Behavior with Stubs
Stubs allow us to emulate the behavior of real dependencies. Let's say we have a TaxCalculator
that depends on an external TaxRateService
to fetch the tax rate for a given location.
public class TaxCalculator {
private TaxRateService taxRateService;
public TaxCalculator(TaxRateService taxRateService) {
this.taxRateService = taxRateService;
}
public double calculateTax(double amount, String location) {
double taxRate = taxRateService.getTaxRate(location);
return amount * (taxRate / 100.0);
}
}
For testing the TaxCalculator
, we can use a stub TaxRateService
to provide canned responses.
public class TaxCalculatorTest {
@Test
public void testCalculateTax() {
TaxRateService taxRateServiceStub = new TaxRateServiceStub(); // Stub implementation
TaxCalculator taxCalculator = new TaxCalculator(taxRateServiceStub);
double tax = taxCalculator.calculateTax(1000.0, "NY");
assertEquals(80.0, tax, 0.01);
}
private class TaxRateServiceStub implements TaxRateService {
@Override
public double getTaxRate(String location) {
return 8.0; // Fixed tax rate for testing
}
}
}
In this example, we created a stub implementation of TaxRateService
that provides a fixed tax rate for testing purposes. We then used this stub in the test for TaxCalculator
to emulate the behavior of the real TaxRateService
.
Best Practices and Considerations
While test doubles are powerful tools for writing effective unit tests, it is essential to consider some best practices and potential pitfalls when using them.
-
Keep Tests Readable: Ensure that your tests remain readable and maintainable. Avoid overly complex setups and verifications that hinder comprehension.
-
Focus on Behavior, Not Implementation: Test doubles should be used to test the behavior of the code under test, not its implementation details. Avoid overly prescriptive mocks that tightly couple the test to the implementation.
-
Avoid Over-Mocking: Over-mocking can lead to brittle tests that break with minor changes in the code. Use mocks, spies, and stubs judiciously, and favor real objects where appropriate.
-
Update Tests with Refactoring: When refactoring your code, make sure to update the tests for the affected components. Test doubles should evolve alongside the code they are testing.
Closing the Chapter
In this blog post, we explored the world of test doubles in Java, including mocks, spies, and stubs. We learned how Mockito can be used for creating and interacting with mocks and spies, as well as how stubs can emulate the behavior of real dependencies. By mastering test doubles, you can write robust and maintainable unit tests that effectively isolate the code under test and verify its interactions with dependencies.
Effective utilization of test doubles not only leads to better-tested code but also provides confidence in the stability and correctness of your Java applications.
So, the next time you sit down to write unit tests for your Java code, remember the power of test doubles and how they can elevate the quality of your testing suite. Happy testing!
With the powerful tool of test doubles, mastering them offers a vast field of possibilities and opportunities to flex your testing muscles, while ensuring a robust, reliable, and maintainable codebase. If you're eager to learn more about Java and its intricacies, don't hesitate to dive into Java's intricacies.
Checkout our other articles