Squashing Bugs: Simplifying Validation in Software Development
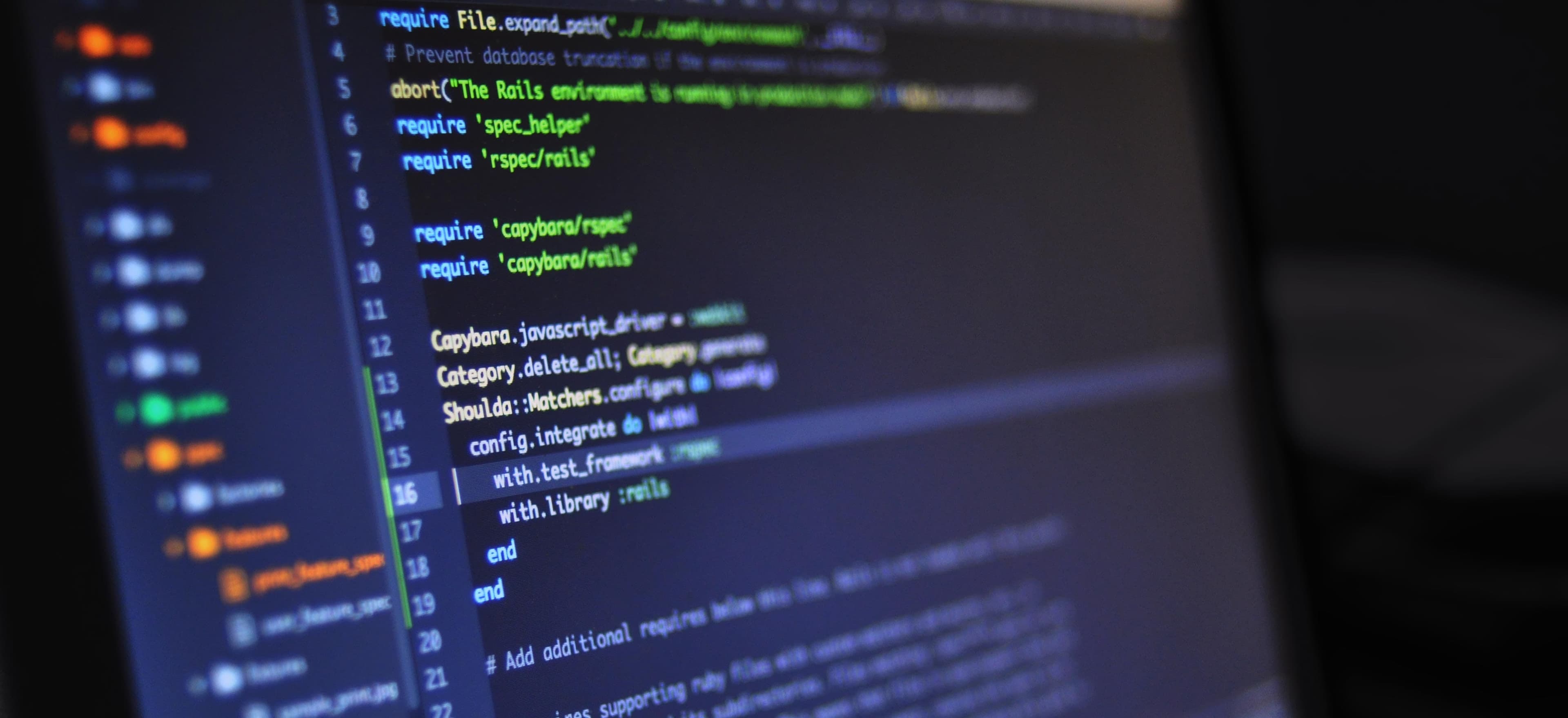
- Published on
Squashing Bugs: Simplifying Validation in Software Development
In the world of software development, validation stands as a crucial step in ensuring the quality, security, and user experience of applications. Specifically, in Java programming, validation plays a pivotal role in guaranteeing data integrity, input correctness, and overall application robustness. In this article, we will delve into the significance of validation in Java applications, the common challenges developers face, and how to simplify the validation process using modern techniques and best practices.
The Necessity of Validation in Java Applications
Java, being a widely used programming language for developing various types of applications, requires robust validation mechanisms. Validation in Java applications encompasses a wide range of aspects, including but not limited to input validation, form validation, and data processing. With Java's strong typing and object-oriented nature, the need for enforcing validation rules becomes even more crucial. Java's ecosystem provides various methods to enforce validations effectively, ensuring that the data used by an application is correct, consistent, and secure.
Common Validation Challenges in Java
Developers often encounter several challenges when implementing validation logic in Java applications. One of the primary challenges is the prevalence of boilerplate code. Writing repetitive code for validations not only affects the codebase's readability but also increases the probability of errors and inconsistencies. Additionally, performance overhead is a concern, especially when dealing with extensive validation logic. Ensuring security while validating user inputs and managing complex validations across different application layers are also challenging tasks that developers face.
Simplifying Validation Process
Using Java Bean Validation (JSR 380)
Java Bean Validation, specified by JSR 380, is a significant step towards simplifying the validation process in Java applications. This specification, through its annotation-based configuration, reduces the need for boilerplate code, making the validation logic concise and more maintainable. Let's look at a simple code snippet demonstrating the usage of Java Bean Validation annotations on a model class:
public class User {
@NotNull
private String username;
@Size(min = 8, max = 20)
private String password;
@Email
private String email;
// Getters and setters
}
In this example, the annotations @NotNull
, @Size
, and @Email
are used to define validation rules for the User
class. The @NotNull
annotation ensures that the username
field is not null, while the @Size
annotation specifies the length constraints for the password
field. The @Email
annotation, provided by additional validation libraries like Hibernate Validator, validates the email
field for proper email format. By utilizing these annotations, developers can significantly improve data integrity and eliminate cumbersome validation code.
Leveraging Frameworks and Libraries
Apart from Java Bean Validation, various frameworks and libraries in the Java ecosystem simplify the implementation of complex validation logic. Frameworks like Hibernate Validator and Spring Validation provide powerful tools and abstractions, allowing developers to define and enforce validation rules with minimal effort. Additionally, these frameworks help in handling cross-field validations and integrate seamlessly with Java Bean Validation, further streamlining the validation process.
For instance, consider the use of Spring Validation for validating an input in a web application:
public class UserController {
@RequestMapping(value = "/user", method = RequestMethod.POST)
public String addUser(@Valid User user, BindingResult result) {
if (result.hasErrors()) {
// Handle validation errors
}
// Process the validated user data
}
}
In this example, the @Valid
annotation triggers the validation of the User
object, and BindingResult
captures any validation errors. By leveraging Spring Validation, developers can effortlessly integrate validation into their web applications, ensuring the correctness of user-provided data.
Best Practices for Effective Validation in Java
Implementing effective validation in Java applications necessitates adhering to best practices to ensure the robustness and maintainability of the validation logic. Here are some best practices that developers should consider:
- Adopt a central approach for validation logic to maintain consistency and ease of maintenance.
- Ensure user-friendly error messages to provide meaningful feedback to users when validation fails.
- Use custom validation rules where necessary to accommodate domain-specific validation requirements.
- Keep validations performant and non-intrusive to avoid impacting application performance.
- Stay updated with the latest validation standards and frameworks to leverage modern techniques for validation.
Advanced Validation Scenarios
In addition to standard validation scenarios, handling more complex validation cases is essential for comprehensive validation in Java applications. Cross-field validation, where the value of one field depends on another, requires custom validator implementations to enforce validation logic effectively. Group sequence validation, a mechanism provided by Java Bean Validation, allows developers to define a sequence of validation groups, enabling scenario-based validation. Moreover, integrating with third-party sources for validation, such as validating emails through external services, extends the validation capabilities of Java applications.
public class PasswordResetForm {
@NotEmpty
private String newPassword;
@NotEmpty
private String confirmPassword;
@AssertTrue(message = "Passwords do not match")
public boolean isPasswordMatching() {
return newPassword.equals(confirmPassword);
}
}
In the code snippet above, a custom validator method isPasswordMatching
is implemented to ensure that the newPassword
and confirmPassword
fields match. This custom validation logic addresses the cross-field validation scenario efficiently, providing a seamless user experience.
Closing Remarks
In conclusion, validation in Java applications is a critical aspect of software development, essential for maintaining the integrity and security of the applications. By adopting modern validation techniques and best practices, developers can significantly reduce bugs and vulnerabilities, ensuring the robustness of their software. We encourage all developers to explore the tools and strategies discussed in this article and experiment with them in their Java projects.
Call to Action
To further delve into the techniques and frameworks discussed in this article, we encourage readers to explore the official Java and framework documentation for deeper insights. By experimenting with these tools and techniques, developers can enhance their understanding of validation in Java applications and improve the quality and security of their software.
In crafting this article, the goal was to present a comprehensive view of validation in Java applications, highlighting the challenges, modern techniques, and best practices. By incorporating relevant code examples and real-world scenarios, the aim was to provide practical insights that readers can apply in their software development endeavors. For further exploration of Java validation techniques, it is recommended to refer to the official documentation and source materials.
Checkout our other articles