Overcoming Common Pitfalls in Google Guava Libraries
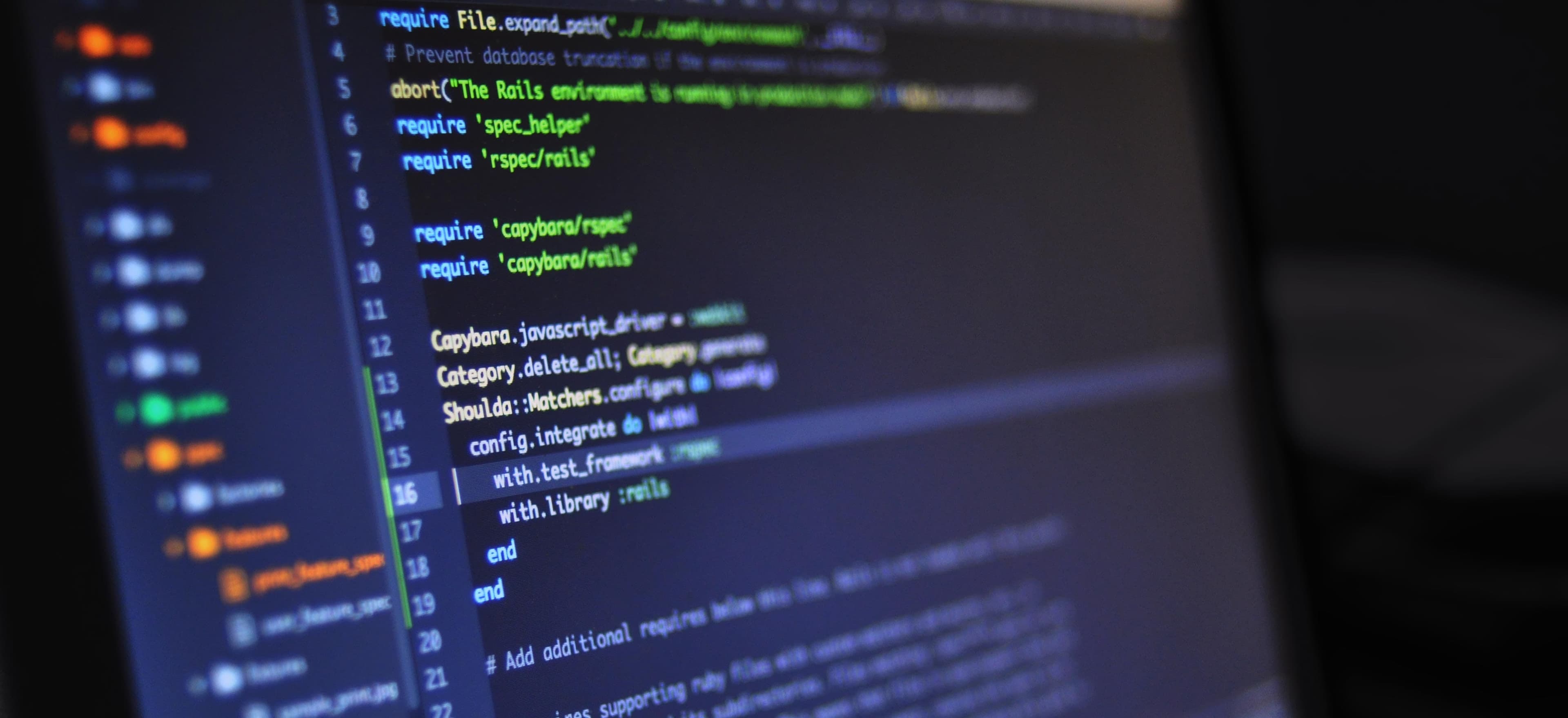
- Published on
Overcoming Common Pitfalls in Google Guava Libraries
Google Guava, also known as Guava, is an open-source set of common libraries for Java programming language. It provides utility methods and immutable collections to ease the development process and improve code quality. However, like any other library, it comes with its own set of pitfalls that developers may encounter. In this article, we will discuss some of the common pitfalls in Google Guava libraries and ways to overcome them.
Pitfall 1: Misusing Immutable Collections
One of the key features of Guava is its immutable collections. It provides classes like ImmutableList
, ImmutableSet
, and ImmutableMap
that guarantee immutability, ensuring that the collection cannot be modified after construction. However, misusing these immutable collections can lead to performance issues and unintended consequences.
Example:
// Misusing ImmutableSet
ImmutableSet<String> set = ImmutableSet.of("a", "b", "c");
Set<String> mutableSet = new HashSet<>(set);
mutableSet.add("d");
In the above example, we are trying to modify an ImmutableSet
by converting it to a mutable HashSet
and adding an element to it. This defeats the purpose of using an immutable collection and can lead to unexpected behavior.
Solution:
Use immutable collections as intended and avoid converting them to mutable collections unless absolutely necessary. Immutable collections provide thread-safety and are ideal for sharing data between multiple threads without the need for synchronization.
Pitfall 2: Overusing Preconditions
Guava provides the Preconditions
class, which allows developers to validate method arguments with the use of static utility methods like checkNotNull
, checkArgument
, and checkState
. However, overusing these preconditions can clutter the code and make it harder to read and maintain.
Example:
// Overusing Preconditions
public void setValue(String value) {
Preconditions.checkNotNull(value, "value cannot be null");
Preconditions.checkArgument(value.length() > 0, "value cannot be empty");
// Some operation
}
In the above example, the setValue
method contains multiple Preconditions
to validate the value
parameter. While it's important to validate method arguments, excessive use of preconditions can lead to code clutter.
Solution:
Use preconditions judiciously and focus on validating critical conditions that may cause the method to fail or produce incorrect results. Use descriptive error messages to provide meaningful feedback to the caller.
Pitfall 3: Unnecessarily Verbose Code with Optional
Guava provides the Optional
class, which is used to represent an immutable object that may contain a non-null reference or may contain nothing. However, using Optional
inappropriately can lead to verbose and convoluted code.
Example:
// Unnecessarily Verbose Optional
Optional<String> optionalValue = Optional.ofNullable(getValue());
if (optionalValue.isPresent()) {
String actualValue = optionalValue.get();
// Some operation with actualValue
}
In the above example, we are using Optional
to handle a potentially nullable value, but the code becomes verbose with the use of isPresent
and get
methods.
Solution:
Prefer using methods like orElse
and ifPresent
to streamline the code when working with Optional
. These methods provide a concise and readable way to handle the presence or absence of a value within an Optional
instance.
Pitfall 4: Not Leveraging Functional Programming Features
Guava provides support for functional programming features such as Function
, Predicate
, and Supplier
interfaces. However, not leveraging these features can lead to verbose and imperative code that may be harder to maintain and understand.
Example:
// Not Leveraging Function and Predicate
List<String> names = Arrays.asList("Alice", "Bob", "Charlie");
List<String> filteredNames = Lists.newArrayList();
for (String name : names) {
if (name.length() > 5) {
filteredNames.add(name.toUpperCase());
}
}
In the above example, we are using an imperative approach to filter and transform elements in a list, which can be replaced with a more concise and declarative approach using Function
and Predicate
interfaces.
Solution:
Leverage functional programming features provided by Guava to write cleaner, more expressive, and maintainable code. Use functional interfaces to encapsulate behavior and make the code more composable and reusable.
Pitfall 5: Ignoring Best Practices for EventBus
Guava provides the EventBus
class to facilitate communication between components in an application. Ignoring best practices when using EventBus
can lead to tight coupling between components and potential performance issues.
Example:
// Ignoring Best Practices for EventBus
public class EventListener {
@Subscribe
public void handleEvent(String event) {
// Handle event
}
}
In the above example, the EventListener
class directly subscribes to events on the EventBus
, potentially leading to tight coupling and reduced flexibility.
Solution:
Follow best practices for using EventBus
by defining clear event contracts, decoupling event producers from subscribers, and using hierarchical event buses to manage event communication effectively. This approach promotes better organization and loose coupling between components in the application.
Lessons Learned
Google Guava provides a rich set of libraries that can significantly improve the development process for Java applications. However, it's essential to be aware of common pitfalls and best practices to leverage Guava effectively. By understanding these pitfalls and adopting best practices, developers can maximize the benefits of Guava while avoiding potential issues and pitfalls.
In this article, we discussed some common pitfalls such as misusing immutable collections, overusing preconditions, writing unnecessarily verbose code with Optional
, not leveraging functional programming features, and ignoring best practices for EventBus
. By being mindful of these challenges, developers can write cleaner, more maintainable, and efficient code using Google Guava libraries.
Remember, while libraries like Guava can greatly simplify and improve the development process, it's equally important to understand their proper usage and potential pitfalls to avoid introducing unintended issues into your codebase.
By following these best practices and understanding the potential pitfalls, you can make the most of Google Guava and ensure that your code is robust, maintainable, and efficient.
For more information on Google Guava libraries, consider checking out the official documentation and GitHub repository. These resources provide in-depth information and examples to help you master the use of Guava in your Java projects.
Checkout our other articles