Mastering Integration Testing: Avoiding Common Pitfalls
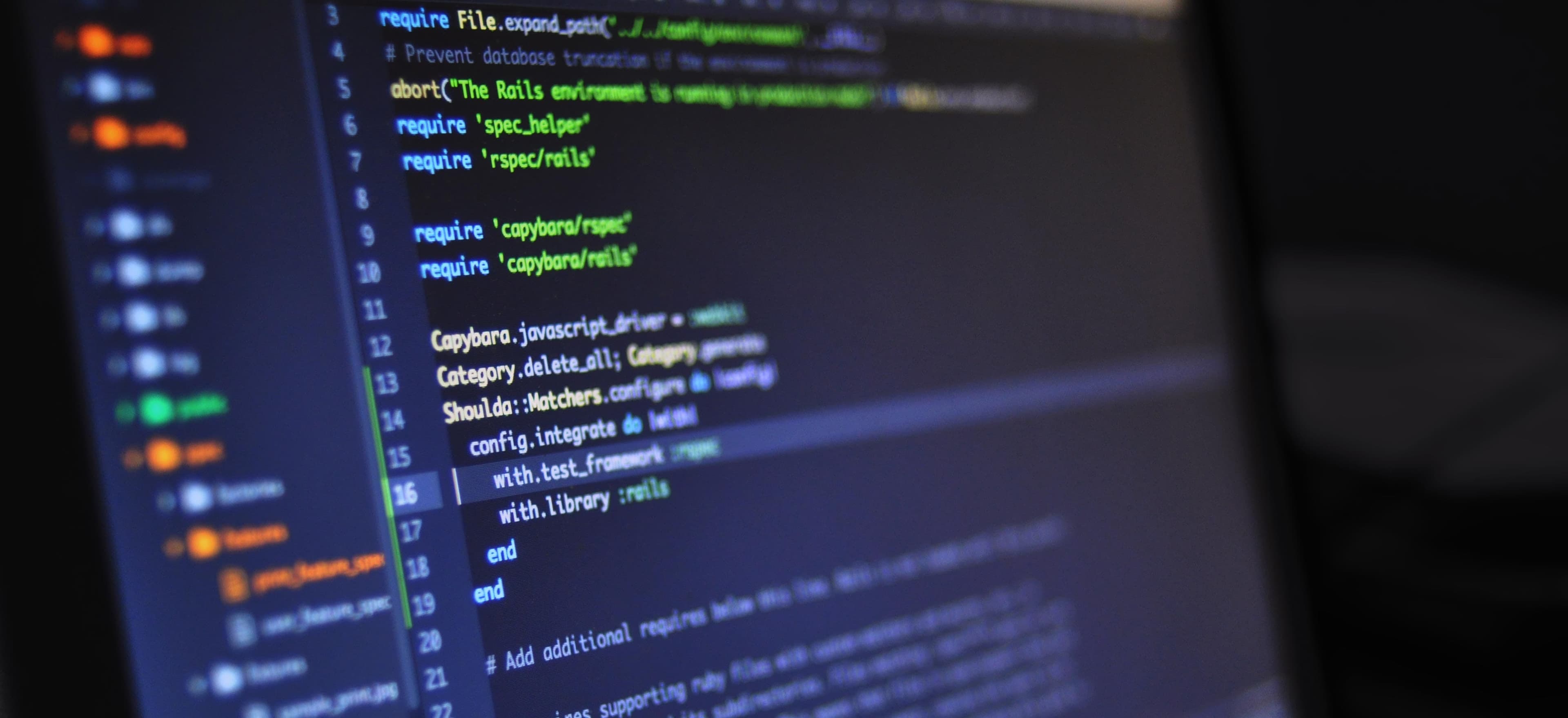
- Published on
Mastering Integration Testing: Avoiding Common Pitfalls
In the realm of software testing, integration testing plays a pivotal role in ensuring that different components of an application work harmoniously together. Especially for Java applications, mastering integration testing is crucial for delivering a seamless and robust software product. In this article, we'll delve into the world of integration testing, exploring its significance, common pitfalls, best practices, practical Java examples, essential tools, and its role in the CI/CD pipeline.
What is Integration Testing?
Integration testing involves testing the interaction between different components or modules of a software system to validate their combined functionality. Unlike unit testing, which focuses on individual parts in isolation, and end-to-end testing, which examines the entire system, integration testing bridges the gap by validating interactions between modules.
To understand the concept better, consider a car assembly line where various components such as the engine, chassis, and wheels are brought together. Integration testing in software development is akin to ensuring that these components seamlessly integrate and function as a complete car should.
Common Pitfalls in Integration Testing
Despite its importance, integration testing presents several challenges and pitfalls. Let's explore a few common ones:
Lack of Clear Testing Strategy
Without a well-defined strategy, integration testing can devolve into an unfocused and inefficient endeavor. A clear testing strategy should outline the scope, approach, and objectives of integration testing.
Environment Mismatches
Differences between development, testing, and production environments can lead to tests passing in one environment but failing in another. Ensuring consistency across environments is vital for reliable integration testing.
Flaky Tests
Flaky tests, which inconsistently pass or fail, can erode trust in the test suite and impede the development process. Identifying and addressing the root causes of flakiness is essential.
Over-Mocking
Overusing mocks in integration testing can create an unrealistic testing environment, potentially masking genuine issues and leading to a false sense of security.
Best Practices to Avoid Pitfalls
To navigate these pitfalls, implementing best practices in integration testing is essential. Here are some strategies to consider:
Consistent Environment Setup
Leverage tools like Docker to create consistent testing environments, minimizing variations that can lead to test failures.
Incremental Testing
Rather than testing everything in a monolithic manner, adopt an incremental approach where parts of the application are integrated and tested gradually.
Effective Use of Mocks
Maintain a balance in using mocks; they should be utilized judiciously to simulate dependencies without creating an overly artificial testing environment.
Practical Example: Integration Testing in Java
Now, let's dive into a practical example of integration testing in a Java application. Consider a straightforward web application built using a Java framework such as Spring Boot.
Setup and Tool Choice
For integration testing in Java, tools like JUnit and Spring Boot Test are instrumental. They provide a robust framework for writing and executing integration tests within the context of a Java application. Their integration capabilities, along with the ease of use and community support, make them ideal choices for this scenario.
Code Snippet: Writing Integration Tests
import org.junit.jupiter.api.Test;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.boot.test.context.SpringBootTest;
import static org.junit.jupiter.api.Assertions.assertEquals;
@SpringBootTest
class UserControllerIntegrationTest {
@Autowired
private UserController userController;
@Test
void testGetUserById() {
String expectedUserName = "John Doe";
String actualUserName = userController.getUserNameById(123);
assertEquals(expectedUserName, actualUserName, "Usernames do not match");
}
}
Explanation and Commentary
In this code snippet, we have an integration test for a UserController in a Spring Boot application. The goal of this test is to verify that when the getUserNameById
method is called with a specific user ID, the correct username is returned. The @SpringBootTest
annotation sets up the Spring context for the test, allowing the UserController to be automatically injected. The assertEquals
statement then compares the expected username with the actual username retrieved from the controller.
This example demonstrates best practices by utilizing the Spring framework's testing support and JUnit to write a concise and effective integration test.
Tools and Frameworks for Integration Testing in Java
Several tools and frameworks empower developers to conduct effective integration testing in Java applications. Here's a list of popular choices and their brief descriptions:
- JUnit: The de facto standard for unit and integration testing in Java, offering powerful assertion libraries and annotation support for writing tests.
- Mockito: A widely used mocking framework that integrates seamlessly with JUnit, enabling the creation of mock objects for testing.
- Spring Boot Test: Specifically tailored for testing Spring Boot applications, it provides features for loading the application context and writing integration tests.
- Testcontainers: Ideal for testing with external dependencies such as databases or message brokers, offering lightweight, disposable instances of containers specifically for testing purposes.
Each of these tools brings unique capabilities to the table, catering to the diverse needs of integration testing in Java applications.
Integration Testing in the CI/CD Pipeline
Integration testing is an integral part of the Continuous Integration/Continuous Deployment (CI/CD) pipeline. By automating integration tests as part of the pipeline, developers can catch integration failures early, enabling faster and more reliable deployments. This leads to increased confidence in the application’s stability and reduces the risk of introducing regressions into production.
A Final Look
Mastering integration testing in Java applications is a crucial aspect of delivering high-quality software. By understanding and addressing common pitfalls, leveraging best practices, and utilizing the right tools and frameworks, developers can ensure that their applications function seamlessly in integrated environments. Embracing integration testing as an integral part of the development process paves the way for more resilient and reliable software products.
To further enhance your understanding of integration testing in Java or delve into advanced topics, explore our additional resources on JUnit, Mockito, and advanced Docker setup for Java applications. We encourage you to share your experiences and insights on integration testing and contribute to the ongoing dialogue on mastering this essential aspect of Java development. Let's continue to elevate our skills and practices in integration testing, ensuring the robustness and dependability of Java applications for years to come.