Unlocking Maven: Effortless Build Number Integration
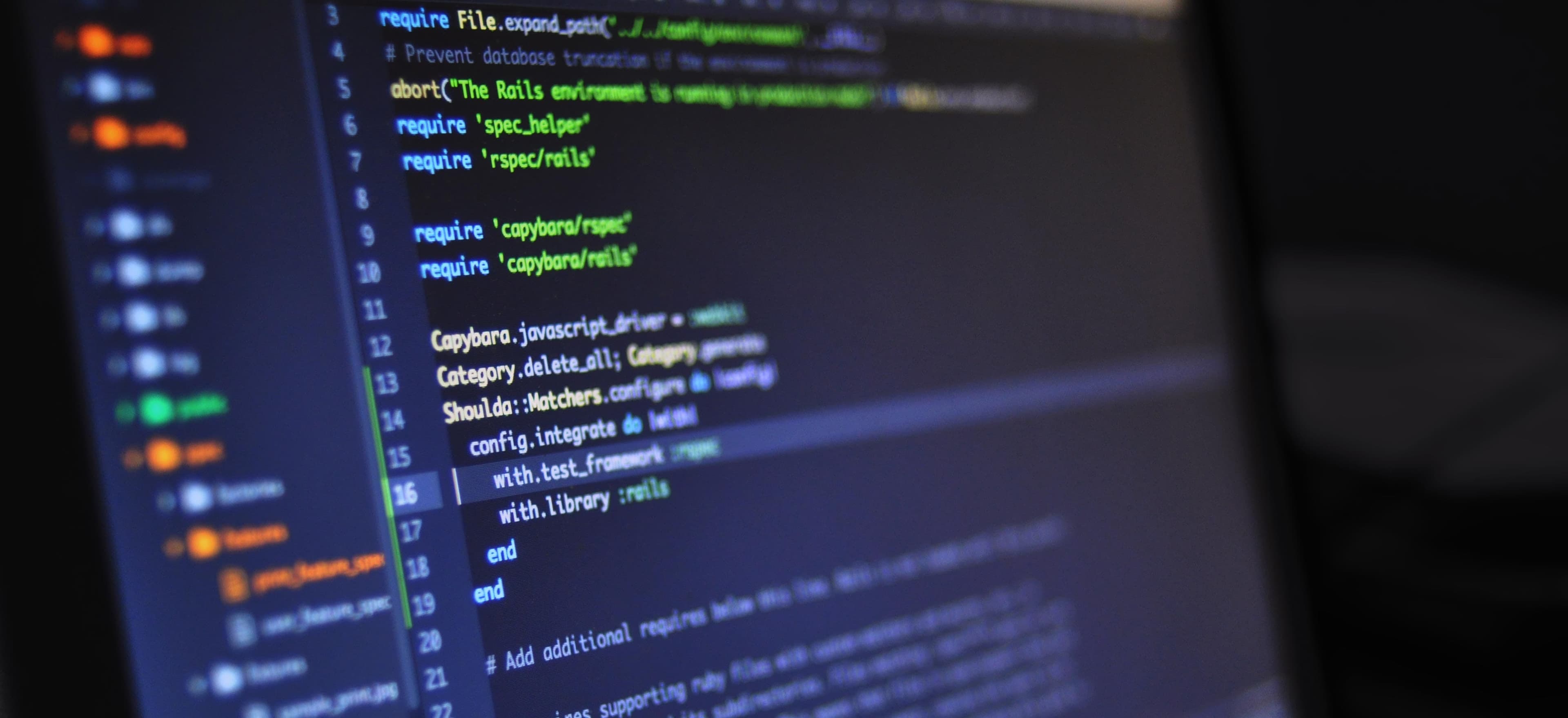
- Published on
Unlocking Maven: Effortless Build Number Integration
In the world of Java development, Maven is the go-to build automation tool, essential for managing dependency, building, testing, and packaging software projects. As projects progress through iterations, it's critical to have a systematic approach to versioning, specifically the build number, to maintain clarity and organization. In this blog post, we'll delve into how you can effortlessly integrate build numbering into your Maven projects, ensuring seamless and systematic version control.
Understanding the Need for Build Number Integration
Before we dive into the integration process, let's understand why build number integration is a crucial aspect of a software project. When an application is built multiple times in a development cycle, having a distinct build number for each version is vital. It allows for straightforward identification of a specific build, which is essential for debugging, troubleshooting, and maintaining version history.
Maven and Build Number Plugin
Maven has a specialized plugin, the Build Number Maven Plugin, that tackles the generation and integration of build numbers. By leveraging this plugin, you can effortlessly inject build numbers into your project, simplifying the version control process.
Integration Process
To integrate build numbering into your Maven project, begin by configuring the Build Number Maven Plugin within the pom.xml
file. This plugin works seamlessly with Maven, automating the process of generating build numbers.
<build>
<plugins>
<plugin>
<groupId>org.codehaus.mojo</groupId>
<artifactId>buildnumber-maven-plugin</artifactId>
<version>1.4</version>
<executions>
<execution>
<phase>validate</phase>
<goals>
<goal>create</goal>
</goals>
</execution>
</executions>
</plugin>
</plugins>
</build>
Here, we are configuring the plugin to execute during the validate
phase, ensuring that the build number is generated early in the build process.
Utilizing the Build Number
Once the configuration is set, you can access the build number within your project's resources or source code. The plugin populates the build information in the ${buildNumber}
property, which can be utilized within the project.
For example, you can include the build number in your application's manifest.mf
file:
<plugin>
<groupId>org.apache.maven.plugins</groupId>
<artifactId>maven-jar-plugin</artifactId>
<configuration>
<archive>
<manifestEntries>
<Build-Number>${buildNumber}</Build-Number>
</manifestEntries>
</archive>
</configuration>
</plugin>
By doing this, the build number will be embedded into the manifest file of the generated JAR, providing clear and concise version identification without manual intervention.
Benefits of Build Number Integration
Integrating build numbers into your Maven project brings forth numerous benefits:
- Version Identification: Each build is uniquely identified, simplifying tracking and reference.
- Automated Process: The process of generating build numbers is automated, minimizing human error.
- Seamless Integration: The build number seamlessly integrates into various parts of the project, such as the JAR manifest file, ensuring consistent versioning across the application.
Version Control Integration
Upon integrating build numbers, it's pivotal to ensure proper version control management. By combining Maven's build numbers with version control systems like Git or Mercurial, you can establish a comprehensive versioning strategy for your software project.
Committing Build Numbers
When a build is triggered, the build number should be committed back to the version control system. This allows for traceability and provides a clear history of the build numbers associated with each version of the codebase.
git commit -am "Integration: Build Number ${buildNumber}"
By committing the build number alongside other changes, you establish a traceable history that aids in debugging and identifying specific builds as the project evolves.
Tagging Builds
In addition to committing build numbers, tagging builds within the version control system is imperative. This practice labels specific points in the project's history (such as release versions) with the associated build number, allowing for simple referencing and rollback if necessary.
git tag v1.0.${buildNumber}
Creating tags for significant build points further enhances the traceability and organization of the project's version history.
Automated Build Servers
In a continuous integration environment, leveraging automated build servers like Jenkins or TeamCity is commonplace. Integrating build number generation into the automated build process enhances the efficiency and organization of the CI/CD pipeline.
By configuring the Build Number Maven Plugin within the automated build server's build configurations, you ensure that each build is systematically assigned a unique build number. This seamless integration streamlines the development workflow, propelling the project forward with precision and clarity.
Key Takeaways
Incorporating build numbers into your Maven projects is an essential practice for achieving systematic versioning and maintaining a cohesive version control strategy. By harnessing the Build Number Maven Plugin, you can effortlessly automate the generation and integration of build numbers, ensuring each build is distinctly identified, tracked, and organized. When combined with version control systems and automated build servers, this approach paves the way for an efficient and robust software development lifecycle.
As you embark on your journey to unlock the potential of Maven's build number integration, remember that systematic versioning is not only a best practice but a foundational aspect of software development, promoting clarity, organization, and traceability.
So, go ahead, unlock the power of build numbers in Maven, and witness the seamless evolution of your projects with effective versioning at its core. Happy coding!
To explore more about Maven and build number integration, refer to Effective Maven Build Number Plugin Usage.
Don't hesitate to share your thoughts and experiences with build number integration in Maven. Your valuable insights could be the stepping stone for someone else's journey into streamlined version control.