Master Android Logging: Avoid Common Pitfalls Easily
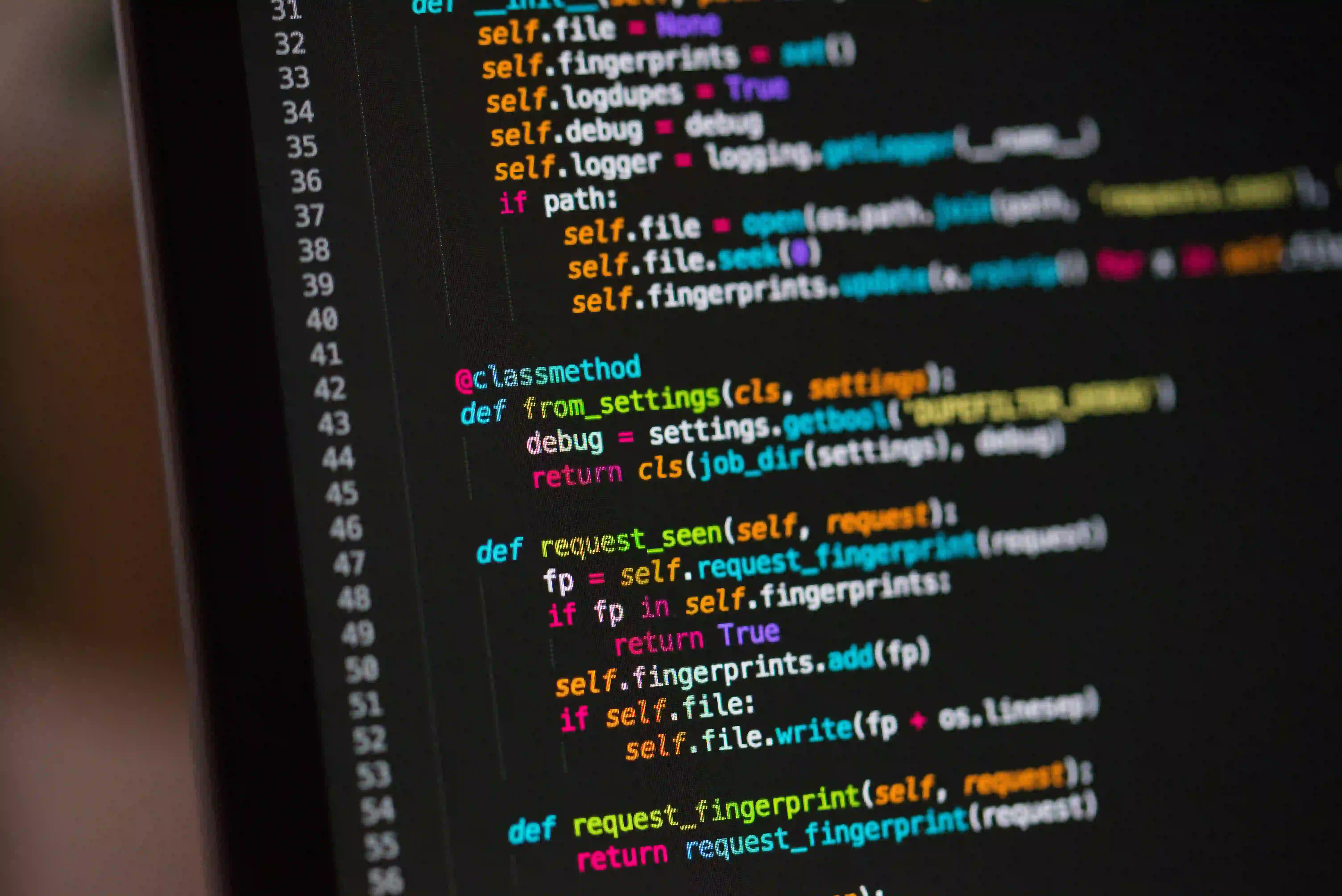
Master Android Logging: Avoid Common Pitfalls Easily
When it comes to developing robust and maintainable Android applications, logging plays a pivotal role. Proper logging not only aids in understanding the flow of the application but also assists in debugging and monitoring the app in a production environment. In this article, we will delve into essential logging practices for Android development, common pitfalls to avoid, and how to address them effectively.
Importance of Logging in Android Development
Logging serves as a fundamental tool for developers to gain insights into the behavior of an application. It provides visibility into the execution flow, variable values, and potential issues. Effective logging helps in diagnosing problems, understanding user behavior, tracking app performance, and much more.
1. Logging Levels
Android offers several logging levels such as VERBOSE, DEBUG, INFO, WARN, ERROR, and ASSERT. Each level is intended for a specific purpose, and understanding their distinctions is crucial for effective logging.
Consider the following code snippet:
public class ExampleClass {
private static final String TAG = "ExampleClass";
public void exampleMethod() {
Log.d(TAG, "This is a debug message");
Log.e(TAG, "This is an error message");
}
}
In this example, Log.d
is used for debugging messages, while Log.e
is employed for error messages. It is imperative to utilize the appropriate logging level to ensure that log output is clear, consistent, and beneficial for troubleshooting.
2. Proguard and Logging
When utilizing Proguard for code obfuscation and optimization in Android applications, log messages might be stripped out, resulting in missing or incomplete logs. To prevent this, it is essential to configure Proguard properly to retain the desired logging.
By including the following configuration in Proguard rules:
-keep class android.util.Log {
public static *** d(...);
public static *** e(...);
// Add other log methods if required
}
By explicitly specifying the preservation of Android's logging methods, the issue of missing logs due to Proguard can be mitigated.
Common Logging Pitfalls in Android Development
While logging is an indispensable aspect of Android development, several common pitfalls are often encountered by developers. Recognizing these pitfalls and understanding how to circumvent them is crucial for proficient Android logging.
1. Over-Logging
Over-logging occurs when an excessive amount of log messages inundate the codebase. This practice not only hampers readability but also impacts performance. Each log statement incurs a certain computational overhead, and an inundation of logs can degrade the application's performance.
The solution to over-logging is to exercise discretion and only log pertinent information that is essential for debugging, monitoring, and understanding the app's behavior.
2. Non-Descriptive Log Messages
Another common pitfall is the utilization of non-descriptive log messages. Vague or ambiguous log messages hinder effective troubleshooting and debugging. Messages such as "Error occurred" or "Data processed" provide little insight into the specific context or cause of the issue.
To address this, it is vital to craft descriptive and informative log messages that convey the exact context, including relevant variable values, method names, and exceptional conditions.
Best Practices for Effective Android Logging
To mitigate the aforementioned pitfalls and streamline the logging process, adhering to best practices is imperative. Let's explore some of the essential best practices for effective Android logging.
1. Utilize Timber Library
Timber, a lightweight and efficient logging library for Android, simplifies the logging process and offers several advantages over the standard Android logging mechanism. It provides support for formatted log messages, tagging, and custom log strategies, making logging more intuitive and robust.
Consider the following implementation using Timber:
public class ExampleApplication extends Application {
@Override
public void onCreate() {
super.onCreate();
if (BuildConfig.DEBUG) {
Timber.plant(new Timber.DebugTree());
}
// Additional configuration for non-debug builds
}
}
By integrating Timber and configuring it with a DebugTree
in the application's onCreate
method, debug-specific logging can be facilitated seamlessly.
2. Contextual Logging with Tagging
Utilizing appropriate tags in log messages lends contextual information, thereby simplifying log filtering and comprehension. Meaningful tags associated with different components or functional areas aid in categorizing and isolating log output effectively.
An example illustrating the use of tags:
public class DataProcessor {
private static final String TAG = "DataProcessor";
public void processData(String data) {
Log.i(TAG, "Processing data: " + data);
}
}
In this example, the tag "DataProcessor" contextualizes the log message, facilitating easy identification and categorization.
3. Centralized Logging Configuration
Maintaining a centralized logging configuration ensures consistency and simplifies the process of modifying logging behavior across the application. By encapsulating logging configuration, such as log levels, formatting, and destinations, in a dedicated module or class, it becomes straightforward to manage and modify logging behavior uniformly.
4. Effective Use of Exception Logging
When handling exceptions, logging the stack trace and pertinent details is vital for comprehensive error analysis. Utilizing the appropriate logging level and crafting informative messages when exceptions occur assists in identifying the root cause of issues and expediting the debugging process.
Closing the Chapter
In the realm of Android development, logging holds immense significance in facilitating app maintenance, troubleshooting, and performance analysis. By grasping essential logging practices, recognizing common pitfalls, and embracing best practices, developers can fortify their logging capabilities and steer clear of potential challenges.
Effective Android logging entails a judicious approach, leveraging appropriate tools and methodologies, and ensuring that log messages are insightful, contextual, and conducive to problem resolution. As logging constitutes a cornerstone of application development, mastering it is indispensable for crafting resilient and maintainable Android applications.
Employing the insights and strategies outlined in this article, developers can elevate their logging proficiency, surmount common pitfalls effortlessly, and orchestrate a robust and elucidative logging infrastructure for Android applications.
For more in-depth guidance on Android development, discover our Android Development Guide for comprehensive insights and best practices.