Overcoming WS-Trust STS Challenges in JMeter Extensions
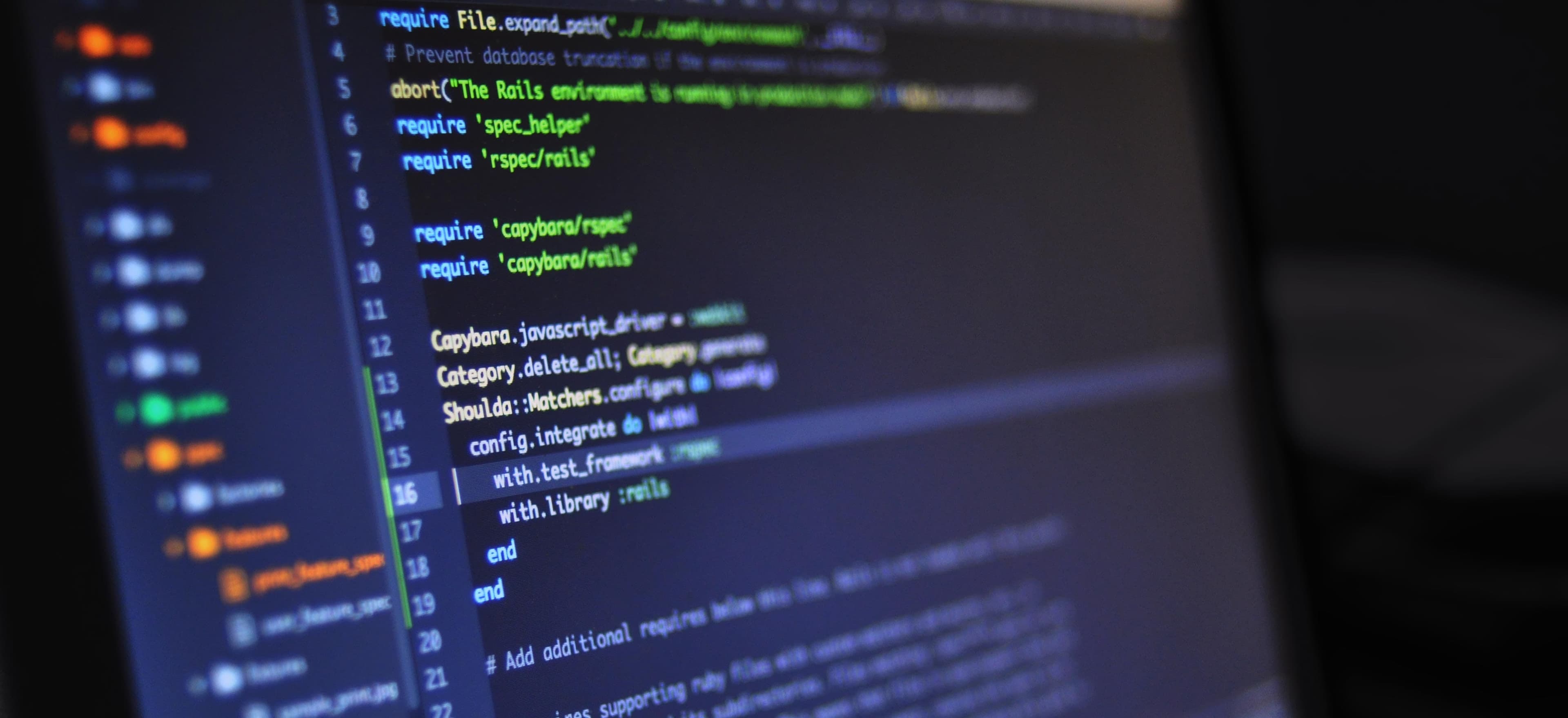
- Published on
Overcoming WS-Trust STS Challenges in JMeter Extensions
When it comes to testing secure web services, Apache JMeter is a popular choice due to its flexibility and extensibility. However, testing web services that use WS-Trust Security Token Service (STS) poses a unique challenge. WS-Trust is a protocol extension to WS-Security that enables applications to obtain, present, and validate security tokens. In this blog post, we'll explore the challenges of testing web services that use WS-Trust STS in JMeter extensions and how to overcome them.
Understanding the Challenge
Testing web services that use WS-Trust STS in JMeter requires the generation and inclusion of security tokens in the SOAP requests. This involves obtaining a security token from the STS, including it in the SOAP message, and handling token expiration and renewal.
The Solution: Custom JMeter Samplers
To overcome these challenges, we can leverage custom JMeter Samplers to interact with the STS, obtain security tokens, and include them in the SOAP requests. One approach is to create a custom Java Request Sampler that communicates with the STS, obtains the security token, and attaches it to the subsequent SOAP request.
Below is an example of a custom JMeter Sampler in Java that handles the WS-Trust STS challenge:
import org.apache.jmeter.protocol.java.sampler.AbstractJavaSamplerClient;
import org.apache.jmeter.protocol.java.sampler.JavaSamplerContext;
import org.apache.jmeter.samplers.SampleResult;
import org.apache.wss4j.dom.WSConstants;
import org.apache.wss4j.dom.message.token.UsernameToken;
import org.apache.wss4j.common.ext.WSSecurityException;
public class CustomWSTrustSampler extends AbstractJavaSamplerClient {
@Override
public SampleResult runTest(JavaSamplerContext context) {
SampleResult result = new SampleResult();
// STS communication and token retrieval logic
// Include token in subsequent SOAP request
return result;
}
@Override
public Arguments getDefaultParameters() {
Arguments params = new Arguments();
// Define STS endpoint, credentials, and other parameters
return params;
}
}
In this example, we extend the AbstractJavaSamplerClient
class and implement the runTest
method to handle the interaction with the STS and inclusion of the security token in the subsequent SOAP request. Additionally, the getDefaultParameters
method is overridden to define the necessary parameters such as the STS endpoint and credentials.
Why Use Custom JMeter Samplers?
Custom JMeter Samplers provide the flexibility to tailor the sampler logic to the specific requirements of testing web services that use WS-Trust STS. By leveraging custom Java code, we can implement the intricate STS interaction and token handling, which may not be feasible with out-of-the-box JMeter components.
Benefits of Custom Samplers:
- Tailored logic to handle STS interaction
- Ability to incorporate token retrieval and inclusion seamlessly
- Greater control over token expiration and renewal
Integration with WS-Security and WS-Trust Libraries
To interact with WS-Trust STS and handle security tokens, we can utilize libraries such as Apache WSS4J, which provides comprehensive support for WS-Security and WS-Trust. By including the necessary libraries in the JMeter classpath, the custom sampler can leverage their functionality for STS communication and token processing.
Including WSS4J Libraries in JMeter:
- Download the WSS4J libraries from the official website
- Place the JAR files in the JMeter
lib/ext
directory - Restart JMeter for the libraries to be available in the classpath
By integrating these libraries, our custom JMeter sampler can access the capabilities of WSS4J to handle the WS-Trust STS interaction seamlessly.
Handling Token Expiration and Renewal
One critical aspect of testing web services with WS-Trust STS is dealing with token expiration and renewal. Security tokens obtained from the STS have a limited validity period, and JMeter needs to manage token renewal to ensure uninterrupted testing.
To address this, the custom JMeter sampler should incorporate logic to monitor token expiration and automatically renew the token when it nears expiration. This ensures that the security token is always valid during the testing process, without manual intervention.
Token Expiration Handling in Custom Sampler:
- Periodic token validity check
- Token renewal before expiration
- Seamless inclusion of renewed token in subsequent requests
By implementing these functionalities in the custom JMeter sampler, we can automate the handling of token expiration and renewal, enabling continuous and uninterrupted testing of web services that use WS-Trust STS.
Bringing It All Together
Testing web services that utilize WS-Trust STS in JMeter extensions requires custom solutions to overcome the challenges of token retrieval, inclusion, and renewal. By developing custom JMeter Samplers in Java and integrating with libraries such as WSS4J, we can effectively address these challenges and conduct thorough testing of secure web services.
Custom JMeter Samplers provide the necessary flexibility and control to interact with STS, manage security tokens, and ensure seamless testing of WS-Trust enabled web services. By incorporating custom Java logic and leveraging external libraries, we can extend JMeter's capabilities to support complex security protocols like WS-Trust, empowering comprehensive and effective testing of secure web services.
By integrating custom Java logic and leveraging external libraries like WSS4J, JMeter can be extended to seamlessly support the complexities of WS-Trust STS. With the ability to create tailored solutions to overcome the challenges posed by secure web services, JMeter remains a powerful tool for comprehensive testing.