Nail Your Android Interview: Top Questions Decoded!
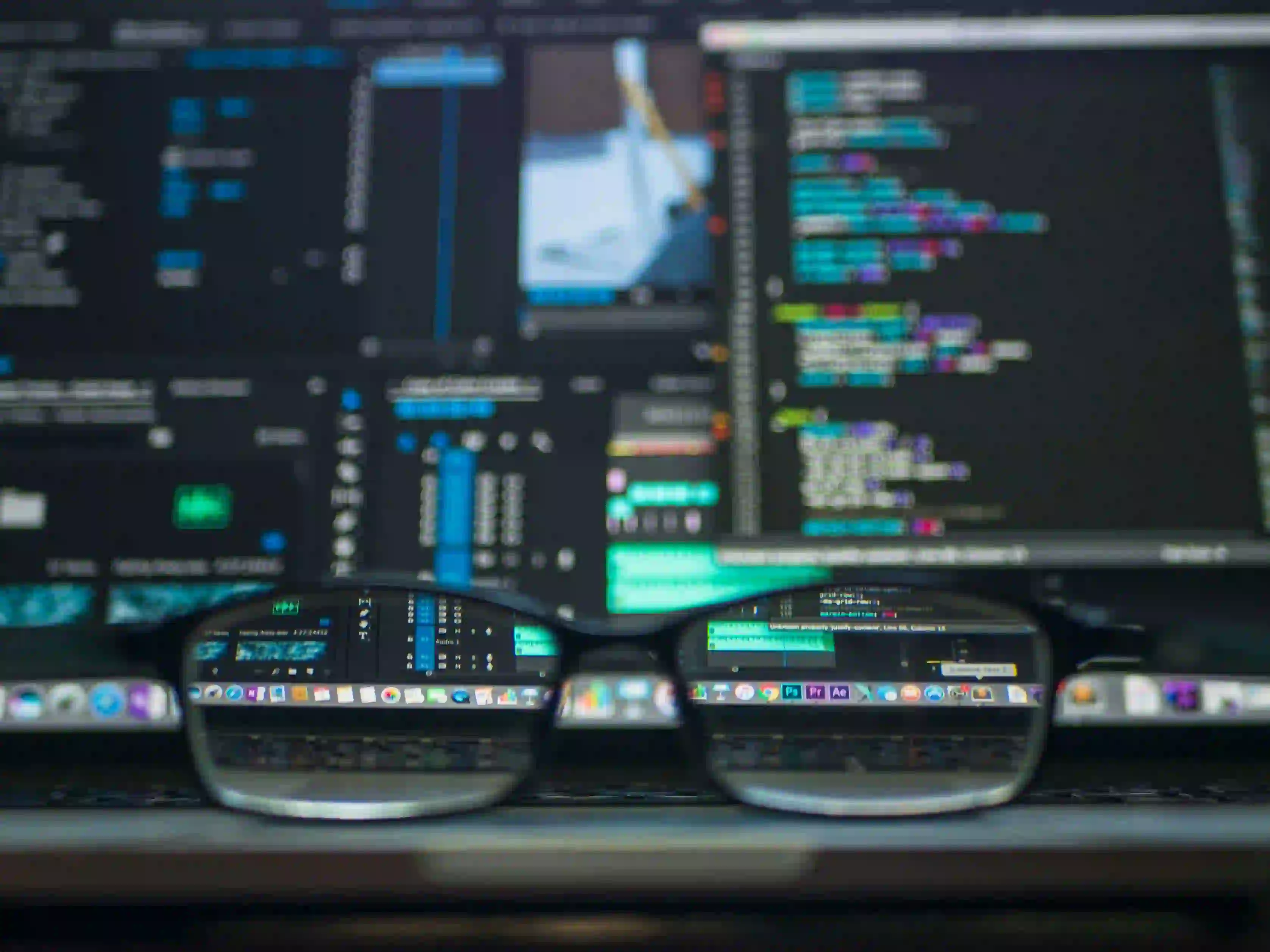
Nail Your Android Interview: Top Questions Decoded!
If you're gearing up for an Android developer interview, you know how important it is to nail the technical questions. To help you prepare effectively, we've decoded some of the top Android interview questions that you're likely to encounter. These questions cover a range of topics, from Java basics to advanced Android concepts.
Understanding Java Concepts
-
Explain the concept of OOP (Object-Oriented Programming) in Java.
Java is an object-oriented programming language, which means it's based on the concept of objects. Everything in Java is an object, and objects can communicate with each other to perform certain actions. The key principles of OOP in Java are inheritance, encapsulation, polymorphism, and abstraction. Inheritance allows a class to inherit properties and methods from another class, encapsulation refers to the binding of data with the methods that manipulate the data, polymorphism allows objects of different classes to be treated as objects of a common superclass, and abstraction focuses on hiding the implementation details while exposing the functionality.
-
What are the differences between
final
,finally
, andfinalize
in Java?-
final
is a keyword used to apply restrictions on class, method, and variable. When a class is declared as final, it cannot be subclassed. When a method is declared as final, it cannot be overridden in the subclass. When a variable is declared as final, its value cannot be changed.-
finally
is a block that always executes, whether an exception is thrown or not.-
finalize
is a method in theObject
class that the garbage collector calls just before an object is destroyed.
Android Concepts and Best Practices
-
What is the activity lifecycle in Android?
The activity lifecycle in Android represents the different states an activity can be in. These states include
onCreate()
,onStart()
,onResume()
,onPause()
,onStop()
,onDestroy()
, andonRestart()
. Understanding the activity lifecycle is crucial for managing an app's behavior and resources efficiently. -
Explain the significance of the AndroidManifest.xml file.
The
AndroidManifest.xml
file is a crucial component of an Android application. It contains essential information about the app, such as the app's package name, components (activities, services, broadcast receivers, and content providers), permissions required by the app, hardware and software features required by the app, and more. This file is essential for the Android system to properly execute the app.
Code Optimization and Best Practices
-
What are some best practices for optimizing Android app performance?
-
Use a ViewHolder pattern for efficient
RecyclerView
implementations. -
Minimize the APK size by using ProGuard for code shrinking and obfuscation.
-
Utilize asynchronous tasks or threads for time-consuming operations to avoid blocking the main UI thread.
-
Employ tools like the Android Profiler to identify performance issues and bottlenecks in the app.
-
Real-World Problem-Solving
-
How would you handle the task of integrating a RESTful API in an Android app?
When integrating a RESTful API in an Android app, it's essential to use libraries like Retrofit or Volley for making network requests. These libraries provide easy-to-use interfaces for defining API endpoints, handling request parameters, and parsing responses. Additionally, it's important to execute network operations on a background thread to prevent blocking the UI thread and handle network connectivity and configuration changes gracefully.
-
Describe a situation where you had to optimize an Android app for different screen sizes and orientations.
Optimizing an Android app for various screen sizes and orientations involves using appropriate layout types, such as LinearLayout, RelativeLayout, and ConstraintLayout, and creating different layout files for different screen sizes and orientations. Additionally, utilizing scalable units like
dp
(density-independent pixels) and%
for dimensions and avoiding hardcoding sizes are crucial for ensuring a consistent user experience across different devices.
In conclusion, mastering these Android interview questions is essential for showcasing your technical skills and problem-solving abilities. By understanding Java concepts, Android fundamentals, code optimization best practices, and real-world problem-solving scenarios, you can confidently tackle the toughest of Android interviews.
Remember, practical knowledge and hands-on experience are just as important as theoretical understanding, so be sure to complement your interview preparation with practical projects and coding exercises.
Good luck with your interview prep, and remember to stay confident and calm during the interview! For additional in-depth insights into Android development and best practices, consider exploring resources like Android Developers and Vogella. Happy coding!
Keep evolving, keep coding!