Boost Your App: Direct SQLite Loading with Custom Android Loader
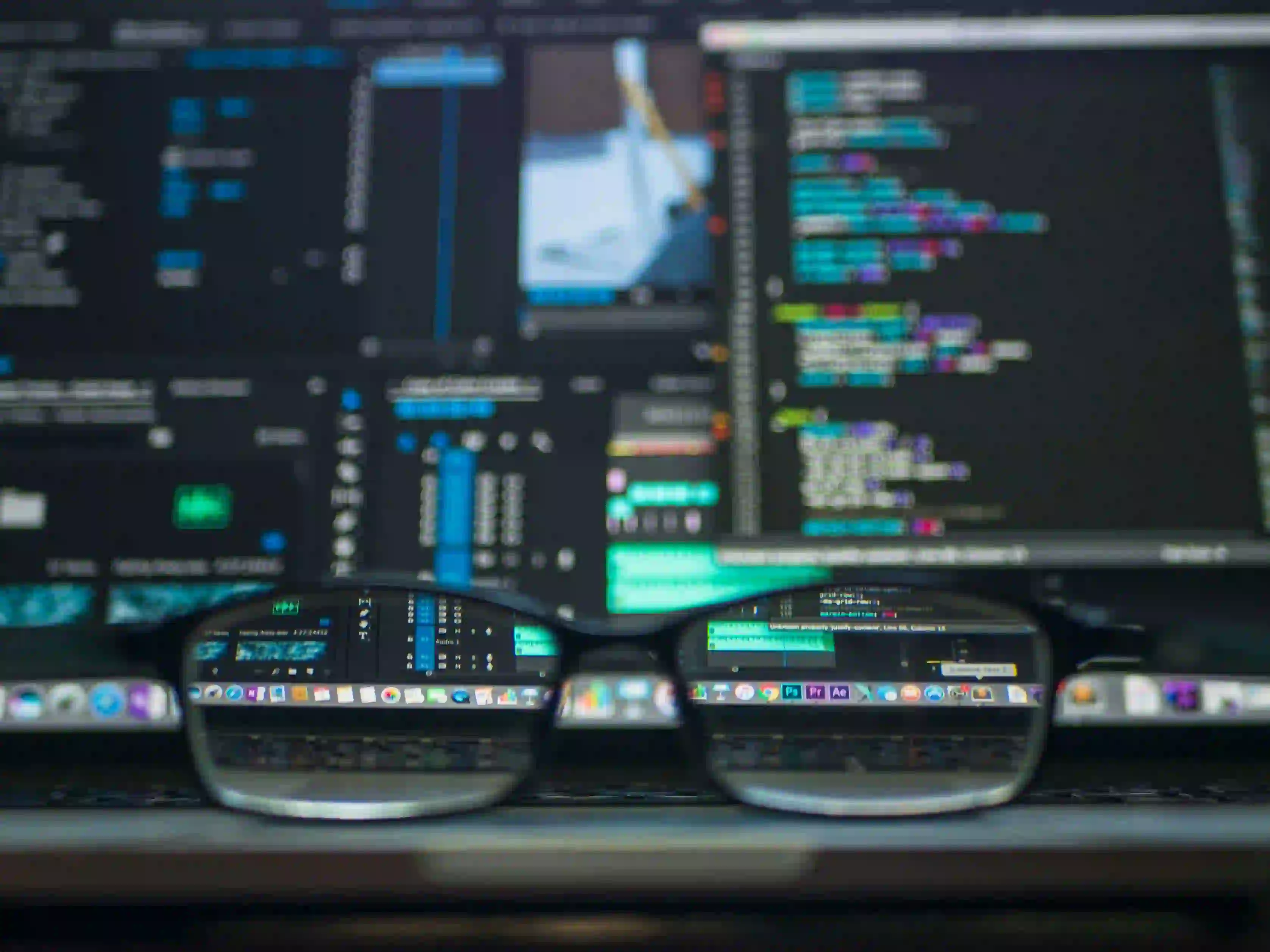
Boost Your App: Direct SQLite Loading with Custom Android Loader
When it comes to developing a high-performance Android app, efficient data loading is crucial. One way to achieve this is through the direct loading of SQLite data using a custom Android Loader. In this blog post, we'll explore the benefits of using a custom Loader to fetch data directly from SQLite in your Android app, along with a step-by-step guide to implement it. Let's dive in!
Why Use a Custom Android Loader for SQLite?
-
Asynchronous Data Loading: By using a custom Android Loader, you can load data from SQLite in a background thread, preventing any potential UI freezing and providing a smooth user experience. This is especially important when dealing with large datasets or complex queries.
-
Efficient Data Handling: Custom Loaders handle data efficiently, automatically managing the data lifecycle when the containing activity or fragment is destroyed and re-created.
-
Improved Performance: Directly loading data from SQLite through a custom Loader helps in optimizing app performance by reducing the time it takes to fetch and display data.
Now that we understand the benefits, let's move on to the implementation.
Implementation Steps
Step 1: Create a Custom Loader Class
public class CustomDataLoader extends AsyncTaskLoader<Cursor> {
public CustomDataLoader(Context context) {
super(context);
}
@Override
public Cursor loadInBackground() {
// Perform SQLite query and return the Cursor
SQLiteDatabase db = dbHelper.getReadableDatabase();
return db.query(TABLE_NAME, null, null, null, null, null, null);
}
@Override
protected void onStartLoading() {
forceLoad();
}
}
In this custom Loader class, we extend AsyncTaskLoader
and override the loadInBackground
method to perform our SQLite query and return the Cursor. The onStartLoading
method ensures the Loader starts loading the data.
Step 2: Implement Loader Callbacks in Activity/Fragment
public class MainActivity extends AppCompatActivity
implements LoaderManager.LoaderCallbacks<Cursor> {
private CustomAdapter adapter;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
adapter = new CustomAdapter(this, null, 0);
ListView listView = findViewById(R.id.listView);
listView.setAdapter(adapter);
getSupportLoaderManager().initLoader(1, null, this);
}
@NonNull
@Override
public Loader<Cursor> onCreateLoader(int id, @Nullable Bundle args) {
return new CustomDataLoader(this);
}
@Override
public void onLoadFinished(@NonNull Loader<Cursor> loader, Cursor data) {
// Update adapter with new Cursor data
adapter.swapCursor(data);
}
@Override
public void onLoaderReset(@NonNull Loader<Cursor> loader) {
// Reset adapter data
adapter.swapCursor(null);
}
}
In the above example, our MainActivity
implements LoaderManager.LoaderCallbacks<Cursor>
and overrides the necessary methods to initialize the Loader, deliver the results, and handle reset scenarios.
Final Thoughts
Using a custom Android Loader for direct SQLite data loading is a powerful way to enhance your app's performance, provide a seamless user experience, and ensure efficient data handling. By following the implementation steps outlined in this post, you can take advantage of the benefits that custom Loaders offer, ultimately boosting your app's capabilities.
To further delve into this topic, you can explore the official Android Developer Guide on Loaders and deepen your understanding of asynchronous data loading and efficient data management.
Incorporate custom Android Loaders in your Android app development arsenal, and witness the remarkable improvements in data loading efficiency and overall app performance.
Start leveraging custom Android Loaders today for a more responsive and performant app!