Mastering Kafka: Overcoming Initial Setup Challenges
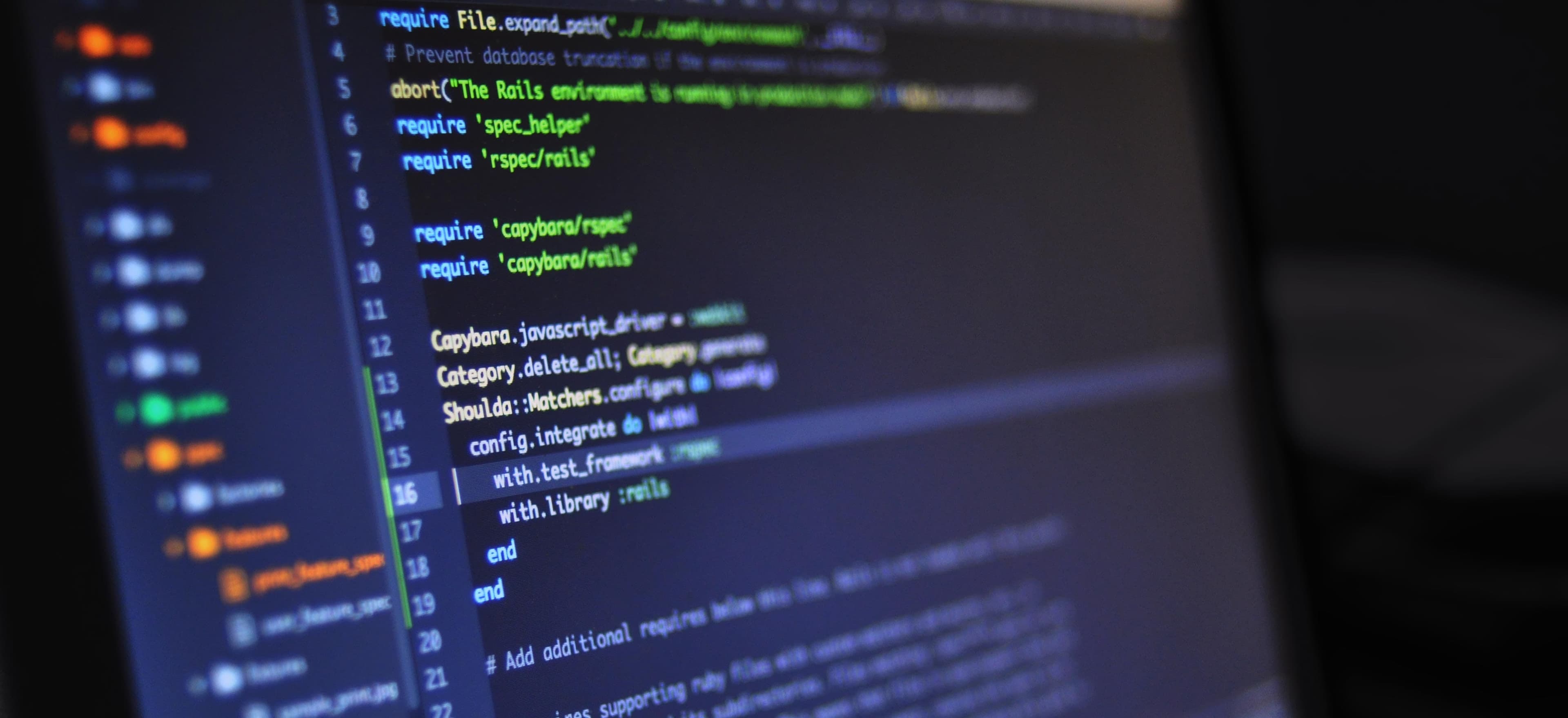
- Published on
Mastering Kafka: Overcoming Initial Setup Challenges
Apache Kafka is a distributed event streaming platform that has gained immense popularity due to its ability to handle high throughput, fault tolerance, and scalability. While Kafka offers powerful features, setting it up for the first time can be daunting for many developers. In this post, we will explore some common challenges faced during the initial setup of Kafka and provide solutions to overcome them.
Understanding Kafka's Architecture
Before diving into the setup challenges, let's briefly recap Kafka's architecture. Kafka is comprised of brokers, topics, partitions, producers, and consumers. Brokers form the cluster, topics are the categorization of data streams, partitions within topics enable parallelism and fault tolerance, producers publish messages to topics, and consumers retrieve messages from topics.
Challenge 1: Setting Up a Kafka Cluster
-
Problem: Setting up a Kafka cluster involves configuring multiple broker instances and managing their intercommunication.
-
Solution: Utilize tools like Docker and Docker Compose to simplify the cluster setup process. Docker allows defining a multi-broker Kafka cluster using a configuration file and running it using Docker Compose. This approach eases the complexity of setting up and managing individual broker instances.
version: '3'
services:
zookeeper:
image: wurstmeister/zookeeper:3.4.6
ports:
- "2181:2181"
kafka:
image: wurstmeister/kafka:2.12-2.3.0
ports:
- "9092:9092"
environment:
KAFKA_ADVERTISED_LISTENERS: INSIDE://kafka:9093,OUTSIDE://localhost:9092
KAFKA_LISTENER_SECURITY_PROTOCOL_MAP: INSIDE:PLAINTEXT,OUTSIDE:PLAINTEXT
KAFKA_LISTENERS: INSIDE://0.0.0.0:9093,OUTSIDE://0.0.0.0:9092
KAFKA_INTER_BROKER_LISTENER_NAME: INSIDE
KAFKA_ZOOKEEPER_CONNECT: zookeeper:2181
volumes:
- /var/run/docker.sock:/var/run/docker.sock
Challenge 2: Defining and Managing Topics
-
Problem: Creating and managing topics with appropriate configurations for retention, replication, and partitions can be overwhelming.
-
Solution: Leverage the Kafka command-line tools to create, list, and describe topics. For example, creating a topic named "my_topic" with three partitions and a replication factor of two can be done using the following command:
bin/kafka-topics.sh --create --zookeeper localhost:2181 --replication-factor 2 --partitions 3 --topic my_topic
This command creates a topic with the specified properties, ensuring fault tolerance and parallelism.
Challenge 3: Integrating Producers and Consumers
-
Problem: Integrating producers and consumers with Kafka for publishing and consuming messages involves understanding the complexities of the Kafka client library.
-
Solution: Leverage high-level Kafka client APIs like the KafkaProducer and KafkaConsumer APIs provided by the Kafka Java client library. Using these APIs abstracts the low-level details of interacting with Kafka, simplifying the integration process.
Here's an example of using the KafkaProducer to publish a message:
Properties props = new Properties();
props.put("bootstrap.servers", "localhost:9092");
props.put("key.serializer", "org.apache.kafka.common.serialization.StringSerializer");
props.put("value.serializer", "org.apache.kafka.common.serialization.StringSerializer");
KafkaProducer<String, String> producer = new KafkaProducer<>(props);
ProducerRecord<String, String> record = new ProducerRecord<>("my_topic", "key", "value");
producer.send(record);
Using the KafkaConsumer to consume messages is similarly straightforward.
Challenge 4: Monitoring and Management
-
Problem: Monitoring and managing a Kafka cluster to ensure optimal performance and reliability can be complex.
-
Solution: Utilize Kafka management and monitoring tools such as Kafka Manager, Confluent Control Center, or commercial solutions like Datadog and New Relic. These tools provide insights into cluster health, lagging consumers, throughput, and other crucial metrics.
Challenge 5: Handling Data Retention and Compaction
-
Problem: Configuring data retention and compaction settings to manage the size of logs and ensure efficient data retention can be challenging.
-
Solution: Understand the available configurations for log.retention.ms, log.retention.bytes, and log.cleanup.policy. These configurations dictate the duration or size for which Kafka retains messages and the compaction strategy for topics. Additionally, implementing a data lifecycle management strategy can help maintain optimal data retention and efficient resource utilization.
My Closing Thoughts on the Matter
Mastering Kafka involves overcoming the initial setup challenges by understanding its core concepts, leveraging available tools and APIs, and implementing best practices for management and monitoring. By addressing the challenges outlined in this post, developers can build a robust and scalable Kafka infrastructure to power their event-driven applications.
Now that we have demystified the initial setup challenges, it's time to unleash the full potential of Kafka and harness its capabilities to build real-time data pipelines and event-driven architectures.
Happy Kafka-ing!
Remember, mastering Kafka is an ongoing journey, and continually exploring its features, best practices, and community insights will elevate your Kafka proficiency to unparalleled heights.