Mastering Java: Convert Objects to JSON in Simple Steps!
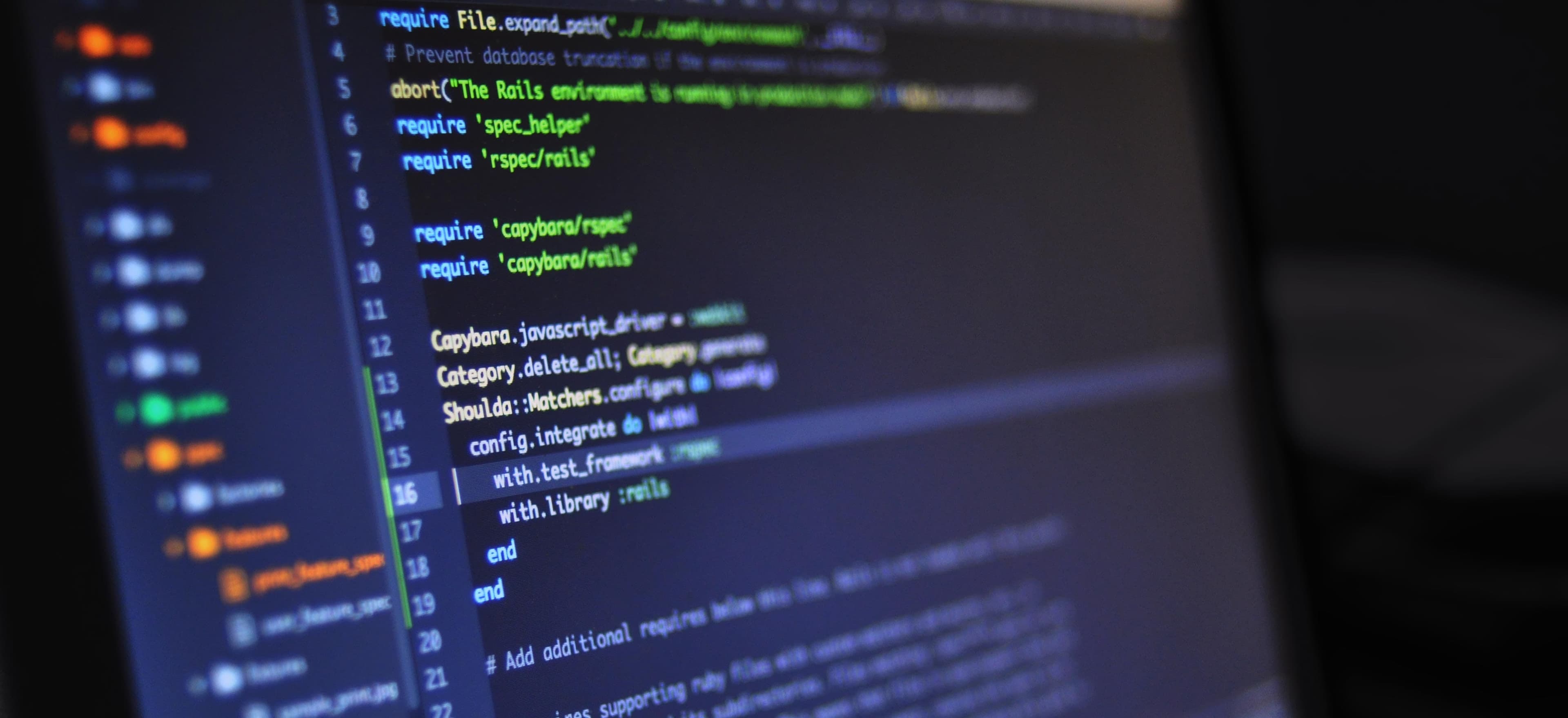
- Published on
Mastering Java: Convert Objects to JSON in Simple Steps!
In the vast and ever-expanding world of software development, Java has consistently remained a powerhouse language due to its versatility, reliability, and widespread use across countless applications. From enterprise-level backend services to compact, efficient mobile applications, Java's capability to adapt and evolve with technological advancements is unparalleled. Among the plethora of methodologies Java enables, converting objects into JSON (JavaScript Object Notation) is a fundamental skill that any Java developer, novice or expert, should have in their toolkit. JSON, with its easy-to-understand format and language-agnostic nature, is crucial for data interchange in the modern web ecosystem. This article demystifies the process of converting Java objects into JSON, providing you with a step-by-step guide, best practices, and exemplary code snippets.
Why Convert Java Objects to JSON?
Before diving into the technicalities, let's unpack why converting Java objects to JSON is a practice you can't afford to overlook:
- API Development: JSON is the de facto standard for data interchange between servers and web applications, making it essential for RESTful API development.
- Data Storage: JSON files are lightweight, human-readable, and easily parsed by various programming languages, making them ideal for configuration files or data storage.
- Cross-language Compatibility: JSON serves as a bridge for data exchange between systems written in different programming languages, fostering interoperability.
Prerequisites
- Basic understanding of Java programming
- Familiarity with JSON structure
- An IDE (Integrated Development Environment) like Eclipse, IntelliJ IDEA, or Visual Studio Code
- Java Development Kit (JDK) installed on your system
Step-by-Step Guide to Convert Java Objects into JSON
Step 1: Choose a JSON Processing Library
Java, by default, does not have inbuilt support for converting objects to JSON. However, numerous libraries can handle this, such as Jackson, Google Gson, and Json-simple. For this guide, we'll focus on Jackson and Gson, two of the most popular libraries that offer a high level of efficiency, flexibility, and ease of use.
- Jackson Project Page
- Gson Github Repository
Step 2: Add the Library to Your Project
Depending on the library you choose, you'll need to include it in your project. If you're using Maven or Gradle, add the corresponding dependency to your pom.xml
or build.gradle
file.
For Jackson:
<dependency>
<groupId>com.fasterxml.jackson.core</groupId>
<artifactId>jackson-databind</artifactId>
<version>2.13.0</version>
</dependency>
For Gson:
dependencies {
implementation 'com.google.code.gson:gson:2.8.8'
}
Step 3: Create Your Java Object
Let's consider a simple Java class named Book
for this example.
public class Book {
private String title;
private String author;
private int publicationYear;
// Standard constructors, getters, and setters
}
Step 4: Convert Java Object to JSON
Using Jackson:
import com.fasterxml.jackson.databind.ObjectMapper;
public class Main {
public static void main(String[] args) {
ObjectMapper mapper = new ObjectMapper();
Book book = new Book("Effective Java", "Joshua Bloch", 2008);
try {
String jsonResult = mapper.writeValueAsString(book);
System.out.println(jsonResult);
} catch (Exception e) {
e.printStackTrace();
}
}
}
Using Gson:
import com.google.gson.Gson;
public class Main {
public static void main(String[] args) {
Gson gson = new Gson();
Book book = new Book("Clean Code", "Robert C. Martin", 2008);
String jsonResult = gson.toJson(book);
System.out.println(jsonResult);
}
}
Why This Code Works
Both snippets showcase a simple yet powerful way of converting a Java object into a JSON string.
-
Jackson's
ObjectMapper
: This class is a part of the Jackson library that facilitates serialization (converting Java objects into JSON) and deserialization (converting JSON into Java objects). The methodwriteValueAsString
is what performs the serialization process in our example. -
Gson's
Gson
class: Similar to Jackson'sObjectMapper
, Gson provides aGson
class that can serialize Java objects into JSON and deserialize JSON strings into Java objects. The methodtoJson
is responsible for serialization.
These libraries abstract away the complexity behind converting Java objects into a format that can be easily shared and utilized across different programming environments, highlighting their robustness and simplicity.
Best Practices
- Always Null Check: Before serialization, check if the object is null to avoid
NullPointerExceptions
. - Custom Serializers: For more complex objects or specific serialization needs, consider writing custom serializers.
- Format and Indent JSON: For better readability, especially in development environments, format the JSON output. Both Gson and Jackson provide ways to pretty-print JSON.
Closing Remarks
Converting Java objects into JSON is a crucial skill in the modern development landscape, empowering Java applications to communicate effectively in web environments and beyond. By leveraging powerful libraries like Jackson and Gson, developers can streamline data exchange processes, ensuring that applications remain scalable, maintainable, and interoperable. This guide has walked you through the process, equipping you with the knowledge to implement this functionality in your Java applications.
Remember, the choice between Jackson and Gson ultimately boils down to personal or project-specific preferences. Both libraries are fully capable of handling the serialization and deserialization needs of most Java projects. Experiment with both and choose the one that best fits your requirements.
Happy coding!
For further reading and to deepen your understanding of Java and JSON integration, consider exploring the official documentation for Jackson and Gson.