Mastering Java: Solving Common Beginner Hurdles
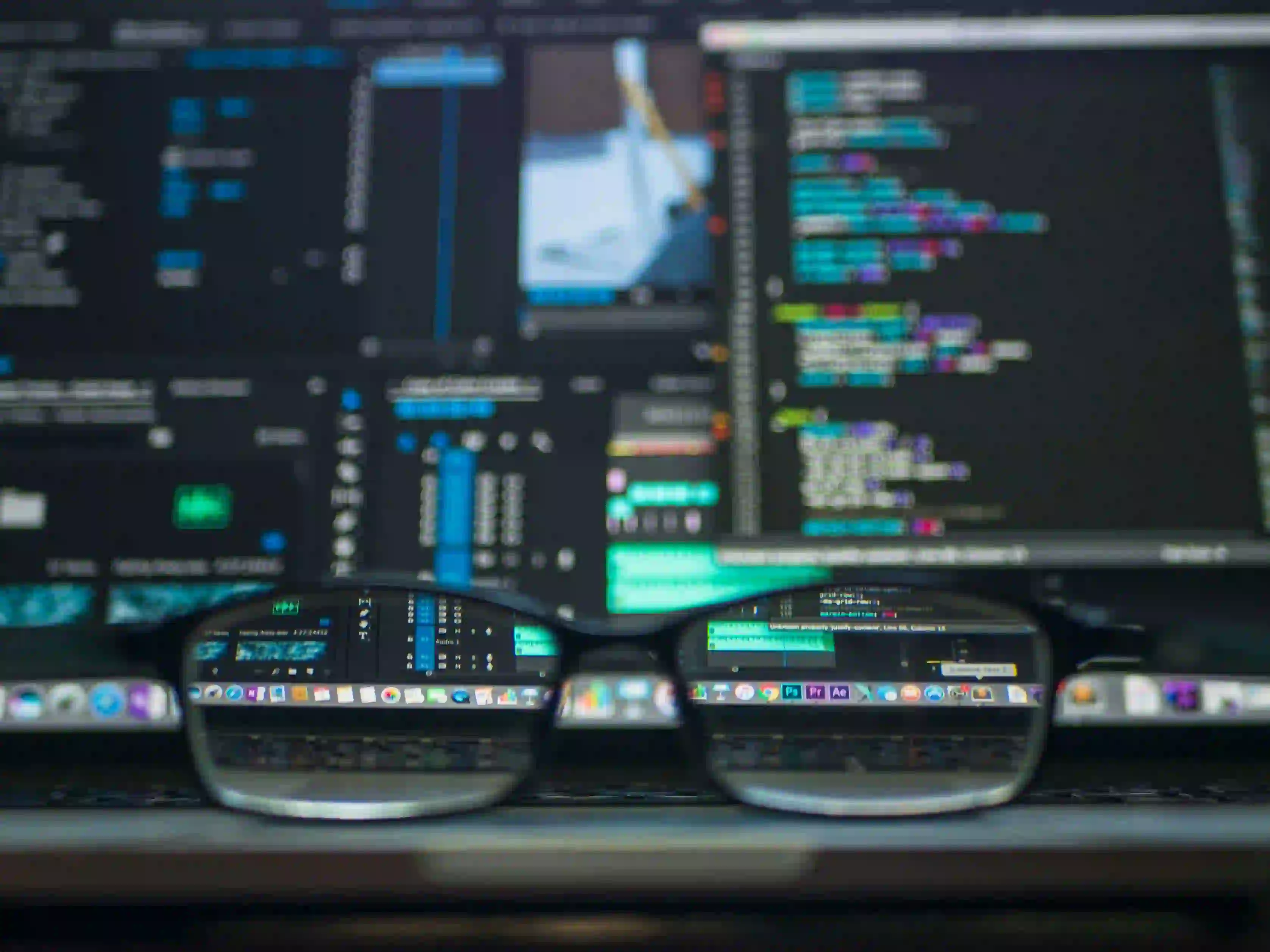
Mastering Java: Solving Common Beginner Hurdles
Java stands as one of the most revered and dynamic programming languages in the world of software development. With its knack for providing versatility, efficiency, and platform independence, it’s no wonder that beginners and seasoned developers alike gravitate towards Java for building robust applications. However, the journey of mastering Java is often dotted with hurdles, especially for those who are just getting their feet wet in the realm of programming. This blog post cuts through the complexity, offering effective strategies and solutions to overcome common beginner challenges in Java programming.
Understanding Java Syntax
Java syntax is the set of rules that defines how Java programs are written and interpreted by the machine. For beginners, Java syntax can seem daunting, but the key is to tackle it one step at a time.
Below is an example of a simple Java program that prints "Hello, World!" to the console:
public class HelloWorld {
public static void main(String[] args) {
System.out.println("Hello, World!");
}
}
Why this code matters: This "Hello, World!" program is more than just a tradition in programming. It introduces newcomers to the basic structure of a Java program, highlighting the class declaration, the main
method, and the System.out.println
method for output.
For further exploration of Java syntax and program structure, refer to the official Java tutorials.
Tackling Java Exceptions
Handling exceptions is a fundamental aspect of writing reliable Java applications. Exceptions in Java are events that disrupt the normal flow of a program's instructions, often resulting from errors like trying to access a file that doesn't exist or attempting to divide by zero.
Consider the following example:
try {
int result = 30 / 0;
} catch (ArithmeticException e) {
System.out.println("Cannot divide by zero!");
}
Why this code matters: This snippet gracefully handles an ArithmeticException
caused by dividing an integer by zero. By wrapping risky code in a try-catch
block, we can catch and manage exceptions, preventing the program from crashing and enhancing its robustness.
For a comprehensive guide on exception handling in Java, the official documentation provides extensive insights and examples: Java Exceptions.
Debugging Java Programs
Debugging is an essential skill in programming, involving identifying and fixing errors or "bugs" in your code. Java offers several tools and IDEs (Integrated Development Environments), such as Eclipse and IntelliJ IDEA, that come with powerful debugging features.
A common debugging technique is to use breakpoints and step through your code. This allows you to pause the execution of your program at certain points and inspect the values of variables to understand how your program is behaving at runtime.
Why debugging matters: Debugging offers a window into the execution of your program, allowing you to pinpoint where things are going wrong. Mastering debugging techniques can significantly cut down development time and increase your efficiency as a Java programmer.
Mastering Object-Oriented Programming (OOP) in Java
Java is an object-oriented programming language, which means it allows developers to structure their software as a collection of objects that incorporate both data and behavior. Understanding OOP concepts such as classes, objects, inheritance, polymorphism, and encapsulation is crucial for writing effective Java programs.
Let’s encapsulate a simple idea into a Java class:
public class Bicycle {
private int gear;
private int speed;
public Bicycle(int gear, int speed) {
this.gear = gear;
this.speed = speed;
}
public void applyBrake(int decrement) {
speed -= decrement;
}
public void speedUp(int increment) {
speed += increment;
}
}
Why this code matters: This Bicycle
class encapsulates the data (properties like gear
and speed
) and behavior (applyBrake
and speedUp
methods) of a bicycle. It is a prime example of using classes to model real-world concepts in a way that is easy to understand and manipulate within our programs.
For an in-depth dive into OOP in Java, check out the Oracle tutorials on OOP.
Leveraging Java Libraries and Frameworks
Java’s ecosystem is rich with libraries and frameworks that can help you build anything from web applications to machine learning models. Libraries like Apache Commons, Google Guava, and frameworks like Spring and Hibernate, significantly extend the functionality of Java, enabling developers to build more complex and high-performing applications efficiently.
Why libraries and frameworks matter: They save time and effort. Instead of reinventing the wheel, you can leverage these tools to implement complex functionalities with less code and in less time.
Parting Thoughts
Mastering Java is a journey, one that is filled with learning and growth. By focusing on and overcoming these common hurdles, beginners can not only sharpen their Java programming skills but also pave their way toward becoming proficient Java developers. Remember, consistent practice, leveraging resources, and actively engaging with the Java community are key to unlocking your full potential as a Java programmer.
In your path to mastering Java, remember that every expert was once a beginner. Embrace challenges, keep experimenting, and never stop learning. The world of Java programming is vast and ever-evolving, and it holds immense possibilities for those willing to explore it diligently.