Avoiding Pitfalls in Event Sourcing & CQRS Implementation
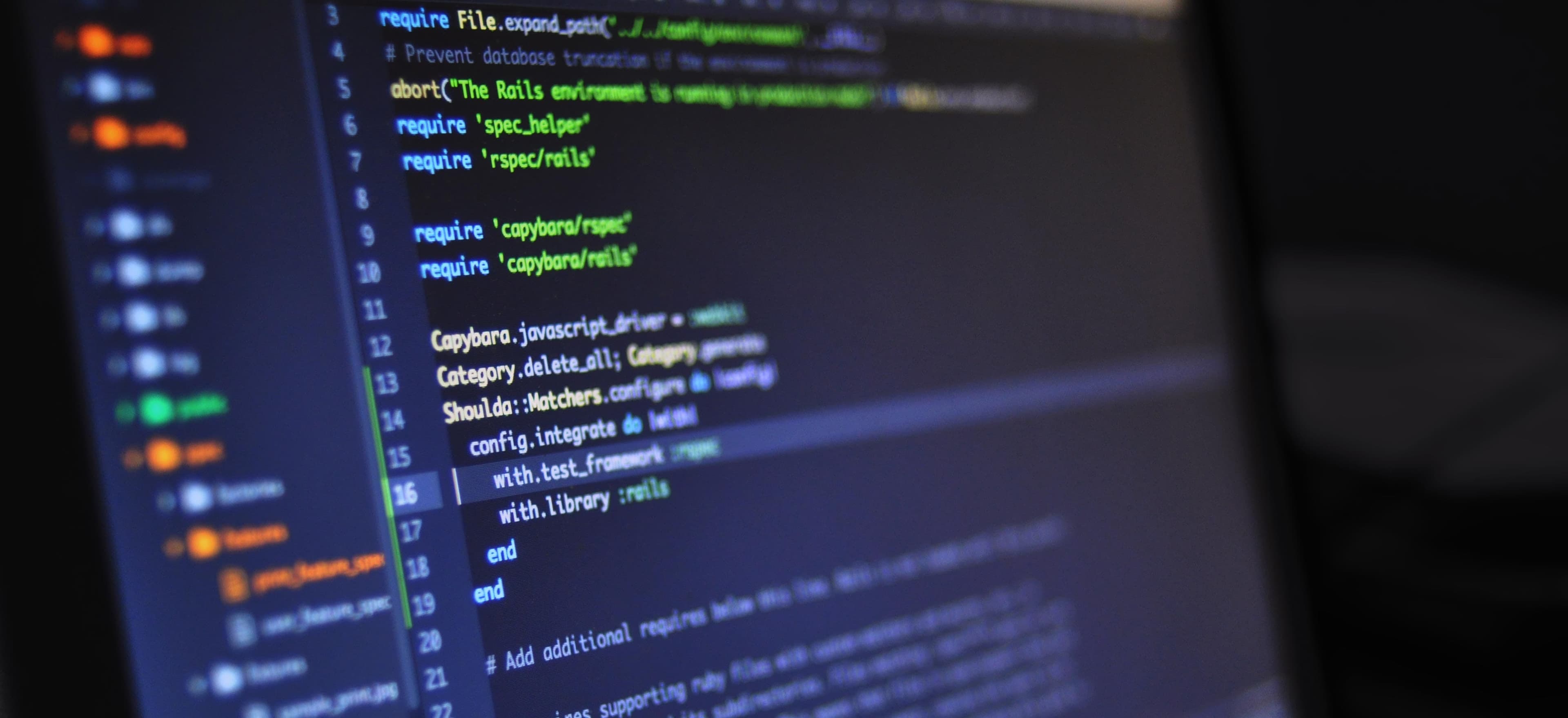
- Published on
Understanding Event Sourcing and CQRS
Event Sourcing and Command Query Responsibility Segregation (CQRS) are architectural patterns that bring significant advantages to the design and implementation of software systems. However, leveraging these patterns effectively requires a deep understanding of their principles and potential pitfalls.
In this post, we will explore the key pitfalls to avoid when implementing Event Sourcing and CQRS in Java applications, and how to address them to ensure a robust and maintainable system.
Pitfall 1: Overusing Event Sourcing
Event Sourcing is a powerful pattern that records all changes to an application's state as a sequence of events. While this approach offers a complete log of changes and enables temporal queries, overusing Event Sourcing can lead to excessive complexity and performance issues.
Solution:
-
Identify the Right Use Cases: Not all parts of the system require Event Sourcing. Focus on areas where auditability, traceability, or temporal queries are crucial.
-
Consider Hybrid Approaches: Use a combination of traditional CRUD operations and Event Sourcing where appropriate, known as "hybrid mode." This can help strike a balance between simplicity and the benefits of Event Sourcing.
// Example of a hybrid approach for an OrderService
public class OrderService {
private final OrderRepository orderRepository;
private final EventDispatcher eventDispatcher;
// ...
public void createOrder(Order order) {
orderRepository.saveOrder(order);
eventDispatcher.dispatch(new OrderCreatedEvent(order.getId()));
}
}
Pitfall 2: Ignoring the Write Model in CQRS
In CQRS, the separation of the Command (write) and Query (read) responsibilities is fundamental. However, focusing solely on the Query side and neglecting the design of the Write model can lead to unbalanced and fragile systems.
Solution:
-
Design Command Models Carefully: Give equal attention to the design and validation of the Command model. Avoid directly using the Query model for write operations.
-
Use Domain-Driven Design (DDD) Principles: Apply DDD concepts to the design of the Write model to ensure it accurately represents the business domain and enforces business rules.
// Example of a Command model for creating an Order
public class CreateOrderCommand {
private String customerId;
private List<OrderItem> orderItems;
// Getters and validation logic
}
Pitfall 3: Neglecting Asynchronous Communication
Event Sourcing and CQRS often involve asynchronous communication between different system components. Neglecting this aspect can lead to performance bottlenecks and increased coupling between components.
Solution:
-
Use Message Brokers: Employ message brokers like Apache Kafka or RabbitMQ to decouple the communication between Command and Event handlers, ensuring better scalability and fault tolerance.
-
Apply Idempotent Operations: Design Command and Event handlers to be idempotent, allowing them to safely process messages in an asynchronous manner without causing inconsistencies.
// Example of an event handler for OrderCreatedEvent using Kafka
@KafkaListener(topics = "order-events")
public void onOrderCreatedEvent(OrderCreatedEvent event) {
// Process the event in an idempotent manner
}
Pitfall 4: Lack of Event Versioning and Evolution Strategy
As the system evolves, the structure of events may need to change. Failing to plan for backward and forward compatibility of events can result in data corruption and system instability.
Solution:
-
Use Schema Evolution Techniques: Employ techniques like event versioning, schema registry, or backward-compatible schema changes to ensure smooth evolution of events over time.
-
Implement Event Upcasting: When an event's structure changes, use upcasting to transform older events into the new format when they are read from the event store.
// Example of event upcasting for Order events
public interface EventUpcaster {
Event upcast(Event event);
}
Pitfall 5: Underestimating Data Migration Challenges
Introducing Event Sourcing and CQRS to an existing system often requires migrating existing data to the new paradigm. Underestimating the complexity of this migration can lead to data integrity issues and system downtime.
Solution:
-
Plan for Data Migration: Devise a comprehensive strategy for migrating existing data to the new event-sourced model, considering data consistency, versioning, and rollback scenarios.
-
Use ETL Tools and Techniques: Leverage Extract, Transform, Load (ETL) tools and techniques to ensure smooth migration of data while maintaining data integrity.
// Example of a data migration script for transitioning to Event Sourcing
public class EventSourcingDataMigrator {
public void migrateData() {
// Extract data from the existing model
// Transform and map to events
// Load into the event store
}
}
Pitfall 6: Inadequate Testing Strategies
Testing an Event Sourcing and CQRS-based system poses unique challenges compared to traditional architectures. Neglecting comprehensive testing can result in subtle bugs and regressions that are hard to detect.
Solution:
-
Write Unit Tests for Event Handlers: Ensure thorough unit testing of Command and Event handlers to cover different scenarios and edge cases.
-
Implement Property-Based Testing: Utilize property-based testing frameworks like jqwik or ScalaCheck to validate system properties and invariants against a large set of generated inputs.
// Example of a property-based test for an Order aggregate
@Property
void changingOrderStatusGeneratesOrderStateChangedEvent(OrderStatus newStatus) {
// Generate random valid Order and newStatus
// Apply the change in status
// Verify that the OrderStateChanged event is produced
}
The Bottom Line
Event Sourcing and CQRS offer numerous benefits in terms of scalability, flexibility, and auditability. However, to harness these benefits effectively, it's crucial to be mindful of the potential pitfalls and adopt best practices to address them. By carefully considering the use cases, design principles, communication patterns, evolution strategies, migration approaches, and testing techniques, Java developers can build robust and maintainable systems that leverage the power of Event Sourcing and CQRS.
Remember, mastery of these patterns takes time, and continuous learning and adaptation are key to successful implementation.
For more in-depth insights into Event Sourcing and CQRS, check out the Axon Framework and CQRS Journey by Microsoft.
Stay tuned for future articles exploring advanced techniques and real-world use cases!