Solving Session Issues in Spring Security REST APIs
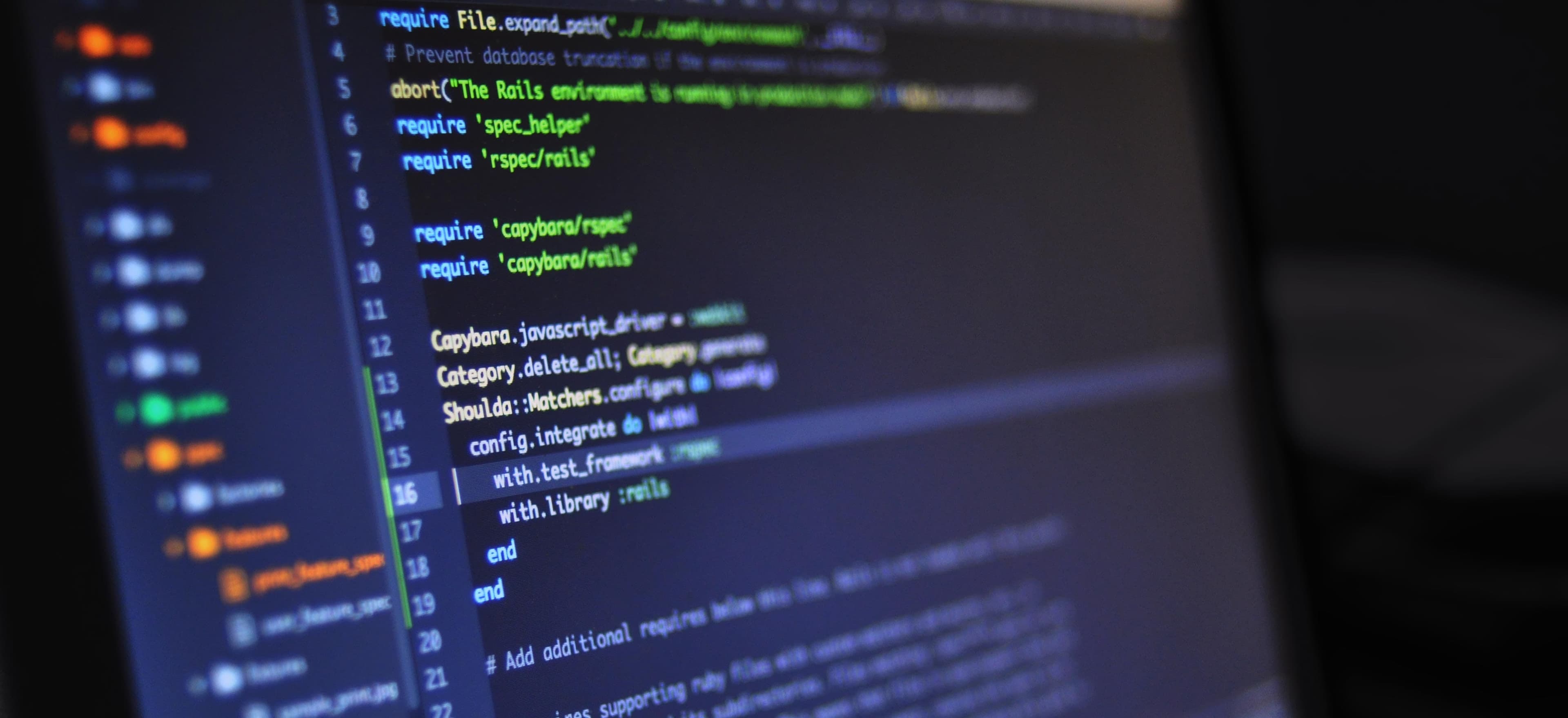
- Published on
Solving Session Issues in Spring Security REST APIs
When developing RESTful APIs using Spring Security, managing sessions can be a challenge. Spring Security's default behavior is to use sessions for authentication and authorization, which can be problematic for stateless RESTful APIs. In this blog post, we will explore the common session-related issues in Spring Security REST APIs and discuss best practices to solve them.
Understanding the Problem
In a traditional web application, sessions are used to maintain user authentication and store user-specific data. However, in RESTful APIs, maintaining sessions contradicts the stateless nature of the architecture. Sessions introduce complexities such as scalability issues, cross-origin resource sharing (CORS) problems, and increased server load.
By default, Spring Security uses sessions for authentication, which can lead to unexpected behavior in RESTful APIs. For example, if a client makes multiple concurrent requests, the server may not be able to handle session synchronization properly, leading to authentication and authorization issues.
Stateless Authentication with JSON Web Tokens (JWT)
To address the session-related issues in Spring Security RESTful APIs, a common approach is to implement stateless authentication using JSON Web Tokens (JWT). JWT is a compact, URL-safe means of representing claims to be transferred between two parties. It can be digitally signed, making it secure and tamper-proof.
When a client authenticates with the server, the server generates a JWT containing the user's credentials and other necessary information. The client includes this JWT in the Authorization header of subsequent requests, and the server validates and processes the token for authentication and authorization.
Configuration for Stateless Authentication
Let's take a look at how we can configure Spring Security for stateless authentication using JWT. First, we need to add the necessary dependencies to our project:
<dependency>
<groupId>io.jsonwebtoken</groupId>
<artifactId>jjwt-api</artifactId>
<version>0.11.2</version>
</dependency>
<dependency>
<groupId>io.jsonwebtoken</groupId>
<artifactId>jjwt-impl</artifactId>
<version>0.11.2</version>
<scope>runtime</scope>
</dependency>
<dependency>
<groupId>io.jsonwebtoken</groupId>
<artifactId>jjwt-jackson</artifactId>
<version>0.11.2</version>
<scope>runtime</scope>
</dependency>
We also need to configure Spring Security to use JWT for authentication. We can do this by extending the WebSecurityConfigurerAdapter
class and overriding the configure
method:
@EnableWebSecurity
public class SecurityConfig extends WebSecurityConfigurerAdapter {
@Override
protected void configure(HttpSecurity http) throws Exception {
http.csrf().disable()
.sessionManagement().sessionCreationPolicy(SessionCreationPolicy.STATELESS)
.and()
.authorizeRequests()
.antMatchers("/api/public").permitAll()
.anyRequest().authenticated()
.and()
.addFilter(new JwtAuthenticationFilter(authenticationManager()))
.addFilter(new JwtAuthorizationFilter(authenticationManager()))
.exceptionHandling().authenticationEntryPoint(new JwtAuthenticationEntryPoint())
.and()
.httpBasic();
}
}
In the above configuration, we disable CSRF protection (as it's not relevant for stateless authentication), set the session creation policy to STATELESS
, and define the endpoints that are accessible without authentication.
We also add JwtAuthenticationFilter
and JwtAuthorizationFilter
to handle authentication and authorization using JWT, and JwtAuthenticationEntryPoint
to handle unauthorized requests.
Handling Token Expiration
JWT tokens have an expiration time, after which they become invalid. When implementing JWT-based authentication in Spring Security, it's essential to handle token expiration appropriately. We can do this by setting the expiration time for the tokens and implementing a mechanism to refresh them.
Here's an example of how we can set the expiration time for JWT tokens:
public class JwtTokenProvider {
private static final long JWT_EXPIRATION = 86400000; // 1 day in milliseconds
// Other methods and configurations...
public String generateToken(Authentication authentication) {
Date expiryDate = new Date(System.currentTimeMillis() + JWT_EXPIRATION);
// Create and sign the JWT token
//...
}
// Other methods...
}
In this example, we set the expiration time for the JWT tokens to 1 day. When a token expires, the client needs to obtain a new token by re-authenticating with the server.
Revoking Tokens
Another challenge in stateless authentication is handling token revocation. In traditional session-based authentication, revoking a user's session is straightforward. However, in stateless authentication with JWT, we need to implement a mechanism to revoke tokens if necessary.
One approach is to maintain a blacklist of revoked tokens on the server side. When a user logs out or when a token needs to be revoked for any reason, we add the token to the blacklist. When processing incoming requests, we check if the token is blacklisted before accepting it as valid.
public class JwtTokenBlacklist {
private Set<String> blacklist = new HashSet<>();
public void revokeToken(String token) {
blacklist.add(token);
}
public boolean isTokenBlacklisted(String token) {
return blacklist.contains(token);
}
}
In the above example, we maintain a simple set of blacklisted tokens. When a token needs to be revoked, we add it to the blacklist, and during token validation, we check if the token is blacklisted before accepting it.
Final Considerations
In this blog post, we explored the common session-related issues in Spring Security RESTful APIs and discussed how to solve them by implementing stateless authentication using JSON Web Tokens (JWT). We also looked at handling token expiration and revocation, which are essential aspects of stateless authentication.
By implementing stateless authentication with JWT, we can overcome the session-related challenges and build secure, scalable, and stateless RESTful APIs with Spring Security.
In summary, stateless authentication with JWT in Spring Security:
- Solves session-related issues in RESTful APIs
- Provides a secure and scalable authentication mechanism
- Requires handling token expiration and revocation
With these best practices and approaches, you can effectively manage session issues in Spring Security RESTful APIs and build robust and secure API architectures.
For more in-depth information on Spring Security and JWT, you can refer to the official documentation of Spring Security and JWT.io.
Checkout our other articles