Overcoming Integration Hurdles in Enterprise Software
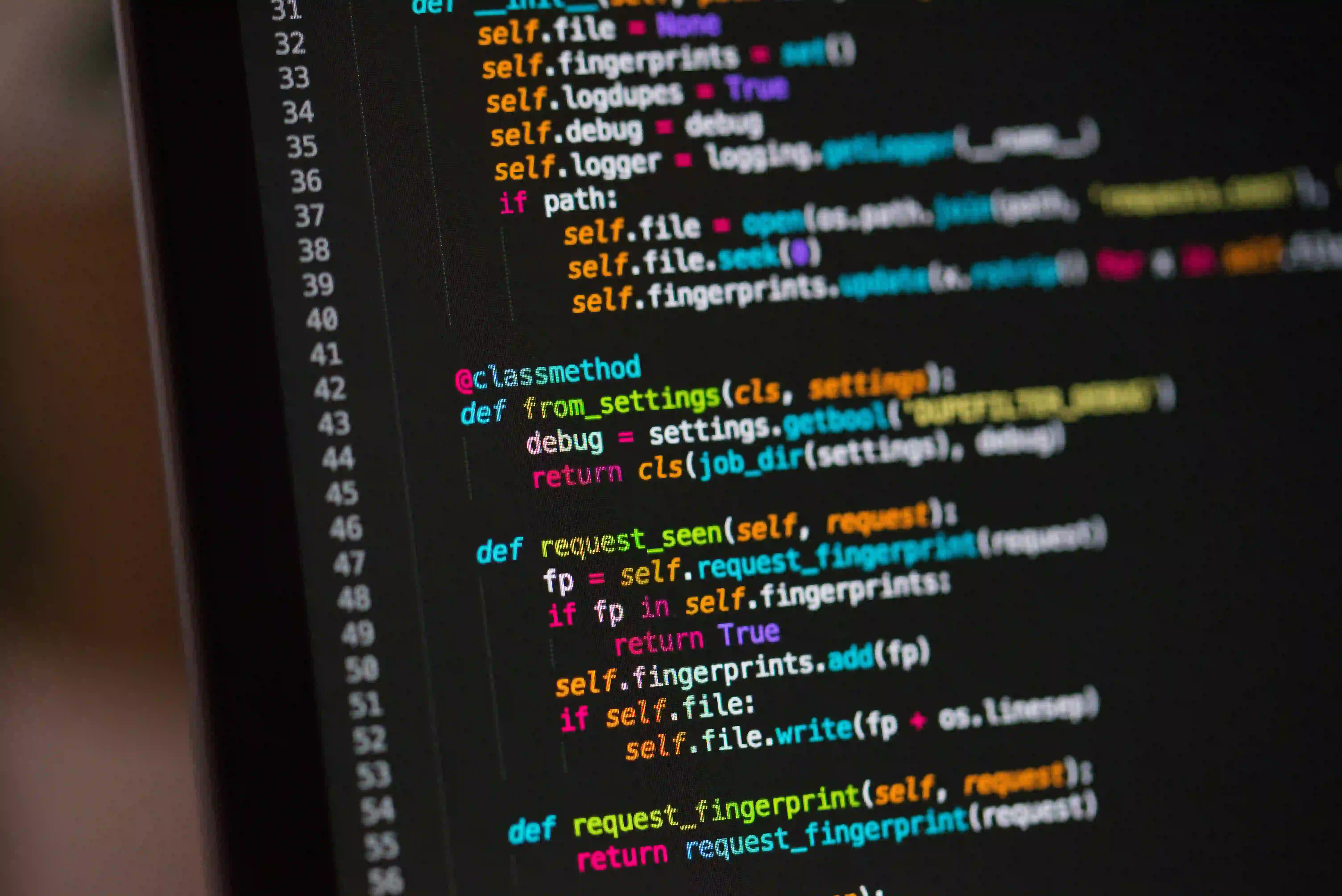
Overcoming Integration Hurdles in Enterprise Software
In today's rapidly evolving technological landscape, enterprises often rely on a multitude of software solutions to streamline their operations and drive productivity. However, integrating these diverse systems can pose significant challenges, including data inconsistency, communication barriers, and workflow disruptions. As a Java developer, it's crucial to understand the complexities of enterprise software integration and how to overcome them effectively.
Understanding the Integration Landscape
The integration landscape for enterprise software is diverse, encompassing various systems such as customer relationship management (CRM), enterprise resource planning (ERP), human resources management (HRM), and more. These systems may be built on different technologies, employ disparate data formats, and operate within distinct network environments. As a result, integrating them seamlessly requires a comprehensive understanding of both the business processes and the technical aspects of these systems.
Leveraging Java for Integration
Java, with its robust features and extensive libraries, is a preferred choice for enterprise software integration. Its platform independence, strong networking capabilities, and support for diverse integration technologies make it well-suited for bridging the gaps between disparate systems. Whether it's integrating legacy systems with modern cloud-based applications or connecting on-premises software with external APIs, Java provides the tools and frameworks necessary to facilitate smooth integration.
Challenges in Enterprise Software Integration
Data Mapping and Transformation
One of the primary challenges in software integration is mapping and transforming data between different systems. This involves reconciling differences in data structures, formats, and semantics to ensure seamless communication. Complex data transformation requirements often arise when integrating systems from different vendors or eras, requiring careful handling to preserve data integrity and consistency.
Service Orchestration
Enterprise software integration frequently involves orchestrating services across multiple systems to execute end-to-end business processes. Coordinating these services, managing their interactions, and handling exceptions and compensations are critical aspects of integration that demand careful attention. Furthermore, ensuring the reliability and fault tolerance of orchestrated services is paramount in guaranteeing smooth operation.
Security and Compliance
Integrating enterprise software brings forth security and compliance concerns, particularly when sensitive data traverses multiple systems. Implementing secure authentication, authorization, and data encryption mechanisms is essential to protect the integrity and confidentiality of integrated data. Moreover, adhering to industry-specific regulations such as GDPR, HIPAA, or PCI DSS adds another layer of complexity to integration efforts.
Overcoming Integration Hurdles with Java
Utilizing Enterprise Integration Patterns
Enterprise Integration Patterns (EIP) offer a valuable repertoire of design solutions for addressing integration challenges. By leveraging EIP in Java, developers can employ proven patterns such as Message Channel, Message Router, and Content Enricher to tackle communication complexities, routing logic, and data enrichment tasks. This approach fosters a standardized, reusable integration architecture that streamlines development and maintenance efforts.
// Example of using the Message Channel pattern in Java
MessageChannel channel = new DirectChannel();
channel.send(MessageBuilder.withPayload(payload).build());
Adopting Integration Frameworks
Java boasts a rich ecosystem of integration frameworks, with Apache Camel and Spring Integration standing out as prominent choices. These frameworks provide a wealth of components, connectors, and abstractions for implementing integration solutions. Whether it's integrating with messaging systems, performing data transformation, or orchestrating services, these frameworks empower developers to expedite integration development while adhering to best practices.
// Using Apache Camel to route and transform messages
from("direct:start")
.choice()
.when(header("type").isEqualTo("customer"))
.to("jms:queue:customerQueue")
.when(header("type").isEqualTo("order"))
.to("jms:queue:orderQueue");
Employing RESTful Integration
In the era of web APIs, RESTful integration has gained immense traction as a lightweight, scalable approach to connecting disparate systems. Java's robust support for building and consuming RESTful APIs through frameworks like Spring MVC and JAX-RS enables seamless integration with web-based systems. By adhering to REST principles, developers can establish uniform interfaces for system interaction, fostering interoperability and ease of integration.
// Creating a RESTful endpoint in Java using Spring MVC
@RestController
public class OrderController {
@GetMapping("/orders/{id}")
public Order getOrder(@PathVariable Long id) {
// Retrieve and return the order details
}
}
Implementing Asynchronous Communication
Asynchronous communication plays a pivotal role in mitigating integration challenges such as latency, scalability, and resilience. Java's support for asynchronous messaging paradigms through JMS, Kafka, or RabbitMQ enables decoupled, event-driven integration architectures. By embracing asynchronous communication, developers can design integrations that exhibit high responsiveness, fault tolerance, and adaptability to fluctuating workloads.
// Publishing messages asynchronously using Kafka in Java
producer.send(new ProducerRecord<>("topic", key, value));
Embracing Microservices Architecture
The rise of microservices has redefined the landscape of enterprise integration, advocating for distributed, independent services that collaborate seamlessly. Java's versatility in developing microservices through frameworks like Spring Boot facilitates granular, decentralized integration approaches. By decomposing monolithic systems into cohesive microservices, organizations can achieve greater agility, resilience, and scalability in their integrated software landscape.
Key Takeaways
Effective enterprise software integration demands a strategic blend of technical expertise, architectural acumen, and pragmatic tooling. As a Java developer venturing into the realm of enterprise integration, mastering the intricacies of data transformation, service orchestration, security, and compliance is imperative. By harnessing Java's rich ecosystem of integration tools, embracing established patterns, and aligning with contemporary integration paradigms, developers can surmount integration hurdles and architect cohesive, interoperable software ecosystems for enterprises.
Navigating the intricate terrain of enterprise software integration with Java is a compelling journey, rife with opportunities to harmonize disparate systems, fortify business processes, and propel organizations towards digital excellence.
So, let's embrace the integration challenges and leverage Java's prowess to engineer seamless, interconnected software landscapes that empower enterprises for sustained success.