Solving Slow Build Times with Maven & Gradle in Docker
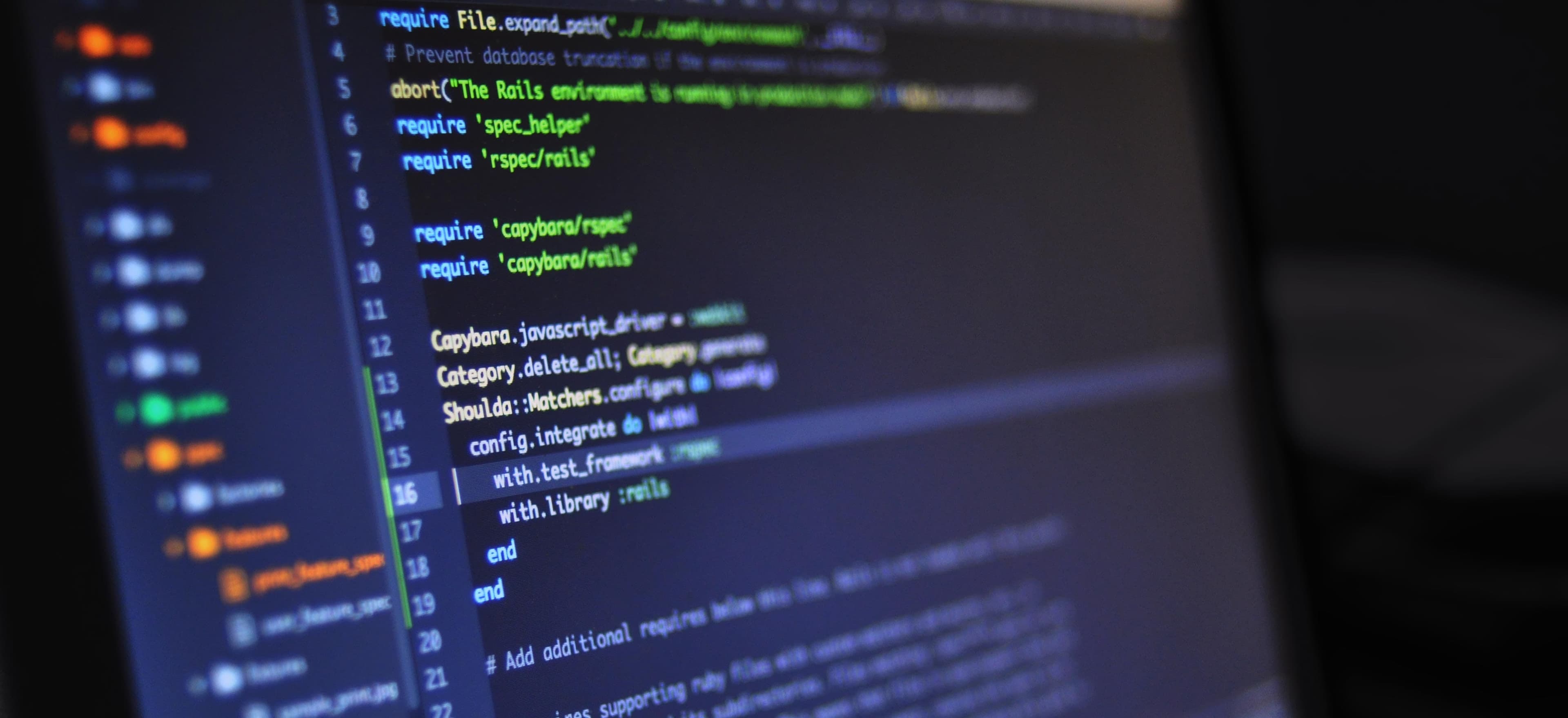
- Published on
Improving Build Times in Docker with Maven & Gradle
In today's software development world, Docker has become an indispensable tool for packaging and distributing applications. However, when it comes to using Docker with build automation tools like Maven and Gradle, developers often encounter issues with slow build times. In this post, we'll explore some strategies to improve build times when using Maven and Gradle in Docker.
Understanding the Issue
Before delving into solutions, it's important to understand why build times can be slow when using Maven and Gradle within Docker containers. The primary reason is the overhead introduced by containerization itself. Docker containers impose a performance penalty due to the isolation they provide, as well as the overhead of running additional processes within the container.
This overhead becomes particularly noticeable when running build tools like Maven and Gradle, which perform numerous I/O operations and often require substantial computing resources. As a result, build times can increase significantly when compared to running these tools directly on the host machine.
Solution 1: Caching Dependencies
One of the most effective ways to mitigate slow build times in Docker is to make use of dependency caching. Both Maven and Gradle rely on external dependencies, such as libraries and plugins, which are downloaded during the build process. With Docker, these dependencies are redownloaded each time a container is built, leading to significant overhead.
To address this issue, we can leverage Docker layer caching by separating the dependency installation step from the application build itself. This can be achieved by creating a separate Docker layer specifically for installing dependencies, ensuring that this layer is cached and reused across subsequent builds, unless the dependencies have changed.
Example - Dockerfile for Maven-based Application
# Use a dedicated stage for dependency installation
FROM maven:3.6.3-jdk-11 AS dependency
WORKDIR /app
# Copy only the POM files to ensure layer caching
COPY ./pom.xml .
# Install dependencies
RUN mvn dependency:go-offline
# Use a separate stage for application build
FROM maven:3.6.3-jdk-11 AS build
WORKDIR /app
# Copy the rest of the application source
COPY . .
# Build the application
RUN mvn package
In the above example, the dependency
stage is used to install project dependencies, while the build
stage handles the actual application build. This separation allows Docker to cache the dependency installation stage, reducing the overhead during subsequent builds.
Solution 2: Utilizing Build Caching
Another approach to improve build times in Docker with Maven and Gradle is to take advantage of build caching provided by these build tools themselves. Both Maven and Gradle offer mechanisms to cache build artifacts and intermediate build results, allowing for incremental builds and reducing the need to re-execute tasks that have already been completed.
When running Maven or Gradle builds within Docker, it's crucial to ensure that these build caching mechanisms are utilized effectively. This can involve configuring the build tools to cache dependencies, compilation outputs, and other intermediate artifacts, as well as optimizing the Docker build process to leverage these cached results.
Example - Utilizing Gradle Build Cache
// build.gradle
plugins {
id 'java'
}
// Enable build caching
tasks.withType(JavaCompile) {
options.fork = true
options.forkOptions.jvmArgs += [
'-Dorg.gradle.daemon=false', // Disable Gradle daemon in Docker
'-Dorg.gradle.caching=true' // Enable Gradle build caching
]
}
In this example, we enable the Gradle build cache by modifying the build configuration. By allowing Gradle to cache compilation outputs and other intermediate results, we can significantly reduce build times when running Gradle builds within Docker containers.
Solution 3: Optimizing Dockerfile Instructions
In addition to leveraging caching mechanisms, optimizing the Dockerfile instructions can also contribute to improved build times. When building Docker images for Java applications using Maven or Gradle, it's essential to structure the Dockerfile in a way that minimizes unnecessary rebuilds and maximizes the reuse of cached layers.
One key consideration is the order of instructions within the Dockerfile. By placing instructions that are less likely to change towards the beginning of the file, and those that are more likely to change towards the end, we can ensure that Docker can reuse cached layers as much as possible.
Example - Reordering Dockerfile Instructions
# Good practice: Place static dependencies higher in the file
COPY ./pom.xml /app/
# Bad practice: Placing dynamic source code above static dependencies
COPY . /app/
In the above example, we prioritize copying static dependencies (POM files) early in the Dockerfile, ensuring that changes to the application source code do not invalidate the cached layers containing the dependencies.
Key Takeaways
Incorporating Maven and Gradle builds into Docker workflows can lead to slower build times due to the inherent overhead of containerization. However, by employing strategies such as dependency caching, build caching, and optimized Dockerfile instructions, developers can mitigate this overhead and achieve faster build times within Docker containers.
By understanding the underlying causes of slow builds and applying these optimization techniques, teams can streamline their build processes and improve the overall efficiency of their development pipelines when working with Docker, Maven, and Gradle.
As always, stay updated with the latest news, updates, and best practices in Java development to ensure the smooth performance of your projects.
We hope these solutions proved helpful. For further reading, check out these articles on Docker layer caching and Gradle build caching.
Checkout our other articles