Mastering Backpressure with Couchbase & RxJava
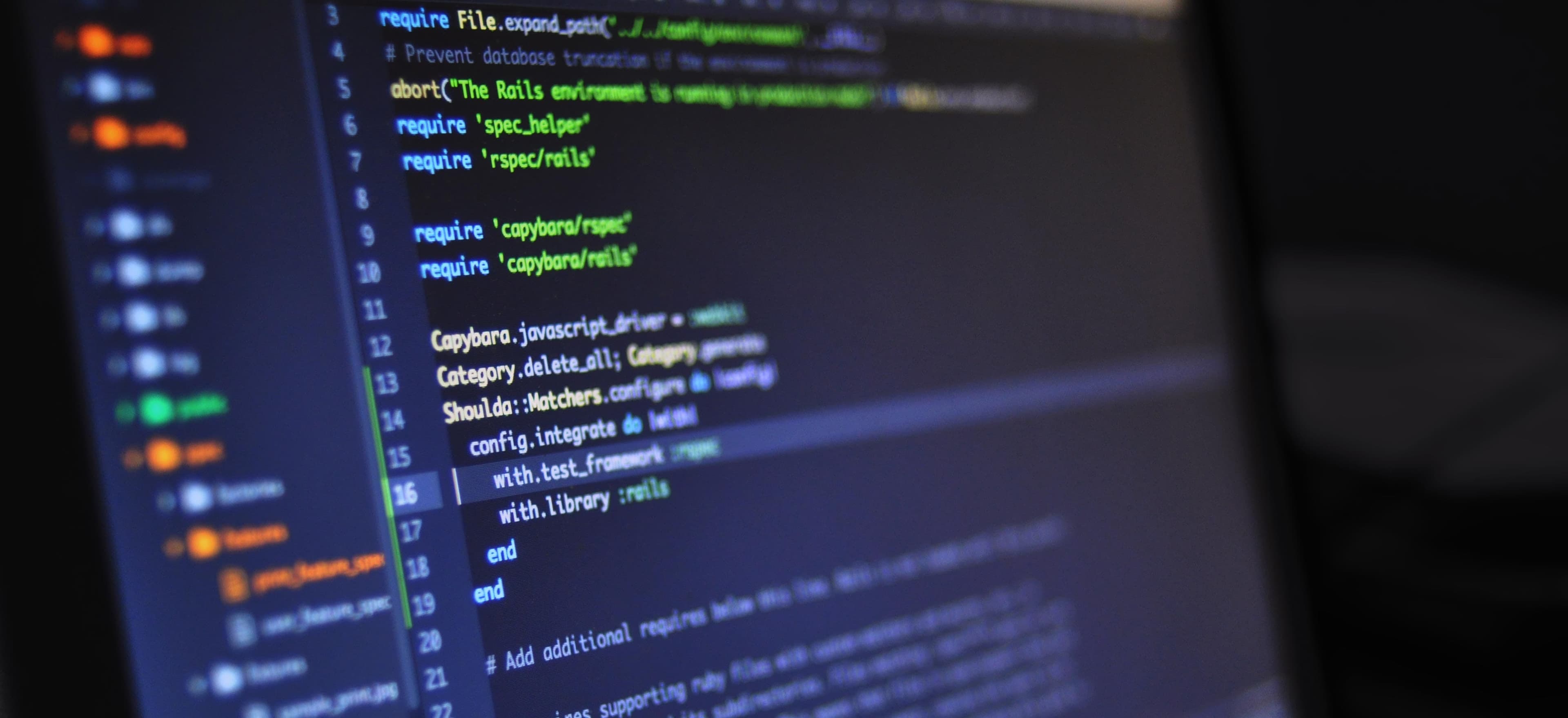
- Published on
Mastering Backpressure with Couchbase & RxJava
In the world of modern, responsive, and scalable applications, backpressure is a critical concept to master in order to prevent overwhelmed systems and ensure smooth, consistent performance. This blog post aims to guide you through understanding and effectively implementing backpressure with the powerful combination of Couchbase and RxJava in a Java application.
Understanding Backpressure
Before diving into the implementation details, let's grasp the essence of backpressure. Backpressure is essentially a way for a consumer to signal to a producer that it is being overwhelmed with data and needs the producer to slow down or stop for a while. This prevents the consumer from being flooded and possibly crashing due to excessive data.
In the context of reactive programming, backpressure is about managing the flow of data from the source (producer) to the consumer, ensuring that the consumer can handle the incoming data at its own pace. This is particularly crucial in scenarios where the producer is capable of generating data at a faster rate than the consumer can process.
Introducing Couchbase and RxJava
Couchbase is a leading NoSQL database that provides a flexible data model and seamless scalability. RxJava, on the other hand, is a popular Java library for composing asynchronous and event-based programs using observable sequences.
By combining the power of Couchbase with the reactive capabilities of RxJava, developers can efficiently handle data streams with backpressure in mind.
Implementing Backpressure with Couchbase & RxJava
Setting up the Environment
First, ensure that you have the Couchbase Java SDK and RxJava library included in your project. You can add the following dependencies in your pom.xml
if you're using Maven.
<dependencies>
<!-- Couchbase Java SDK -->
<dependency>
<groupId>com.couchbase.client</groupId>
<artifactId>java-client</artifactId>
<version>{latest_version}</version>
</dependency>
<!-- RxJava -->
<dependency>
<groupId>io.reactivex.rxjava3</groupId>
<artifactId>rxjava</artifactId>
<version>{latest_version}</version>
</dependency>
</dependencies>
Creating a Couchbase Connection
To establish a connection with Couchbase, initialize a Cluster
object using the Couchbase Java SDK.
import com.couchbase.client.java.Cluster;
import com.couchbase.client.java.env.ClusterEnvironment;
ClusterEnvironment env = ClusterEnvironment.builder().build();
Cluster cluster = Cluster.connect("yourHostname", "yourUsername", "yourPassword");
Handling Backpressure with RxJava
Now, let's see how RxJava comes into play for managing backpressure. Suppose we want to retrieve a large number of documents from a Couchbase bucket and process them asynchronously while considering backpressure.
import com.couchbase.client.java.ReactiveCollection;
import com.couchbase.client.java.json.JsonObject;
import io.reactivex.rxjava3.core.Flowable;
import io.reactivex.rxjava3.schedulers.Schedulers;
ReactiveCollection collection = cluster.bucket("yourBucketName").defaultCollection().reactive();
Flowable<JsonObject> dataStream = collection.getAllData()
.observeOn(Schedulers.io())
.subscribeOn(Schedulers.computation())
.toFlowable()
.onBackpressureBuffer(100, () -> LOGGER.info("Buffer is full, waiting for space to be available"));
dataStream
.onBackpressureBuffer(100)
.observeOn(Schedulers.computation())
.subscribe(data -> {
// Process each document
}, Throwable::printStackTrace);
In this example, the collection.getAllData()
method returns a reactive stream of Couchbase documents. We then apply backpressure handling using RxJava operators. The observeOn()
and subscribeOn()
operators help in offloading work to different threads while considering the backpressure.
The onBackpressureBuffer()
operator is especially important in this scenario. It creates a buffer of a specified size, allowing the producer to continue producing even when the consumer is overwhelmed. This way, backpressure is managed by temporarily storing excess data until the consumer is ready to process it.
Understanding the Code
It's important to understand the implications of each line of code. The observeOn(Schedulers.io())
and subscribeOn(Schedulers.computation())
operators ensure that the data processing work occurs on appropriate threads. The onBackpressureBuffer(100, () -> LOGGER.info("Buffer is full, waiting for space to be available"))
line helps in logging and monitoring the buffer's status.
Additionally, the chained onBackpressureBuffer(100)
and observeOn(Schedulers.computation())
in the subscription demonstrate the proactive application of backpressure handling at both the producer and consumer ends of the data stream.
Lessons Learned
With the combination of Couchbase and RxJava, you have the tools to efficiently handle backpressure in your Java applications. By understanding the principles of backpressure and leveraging the reactive capabilities of RxJava, you can build robust and scalable systems that gracefully manage the flow of data, preventing overwhelming consumers and ensuring smooth, responsive performance.
By mastering backpressure, you pave the way for reliable and resilient systems capable of handling varying loads and maintaining optimum performance. Embrace backpressure as a core concept in your reactive programming arsenal, and leverage the powerful capabilities of Couchbase and RxJava to build resilient, scalable, and responsive applications.
Now that you have a solid understanding of backpressure and its implementation with Couchbase and RxJava, put this knowledge into practice and elevate the efficiency and responsiveness of your Java applications. Happy coding!
For more in-depth information on Couchbase's reactive programming support and best practices, refer to the official Couchbase documentation, and for an in-depth understanding of RxJava's backpressure handling, delve into the RxJava documentation.