Mastering Context Injection in JAX-RS for Beginners
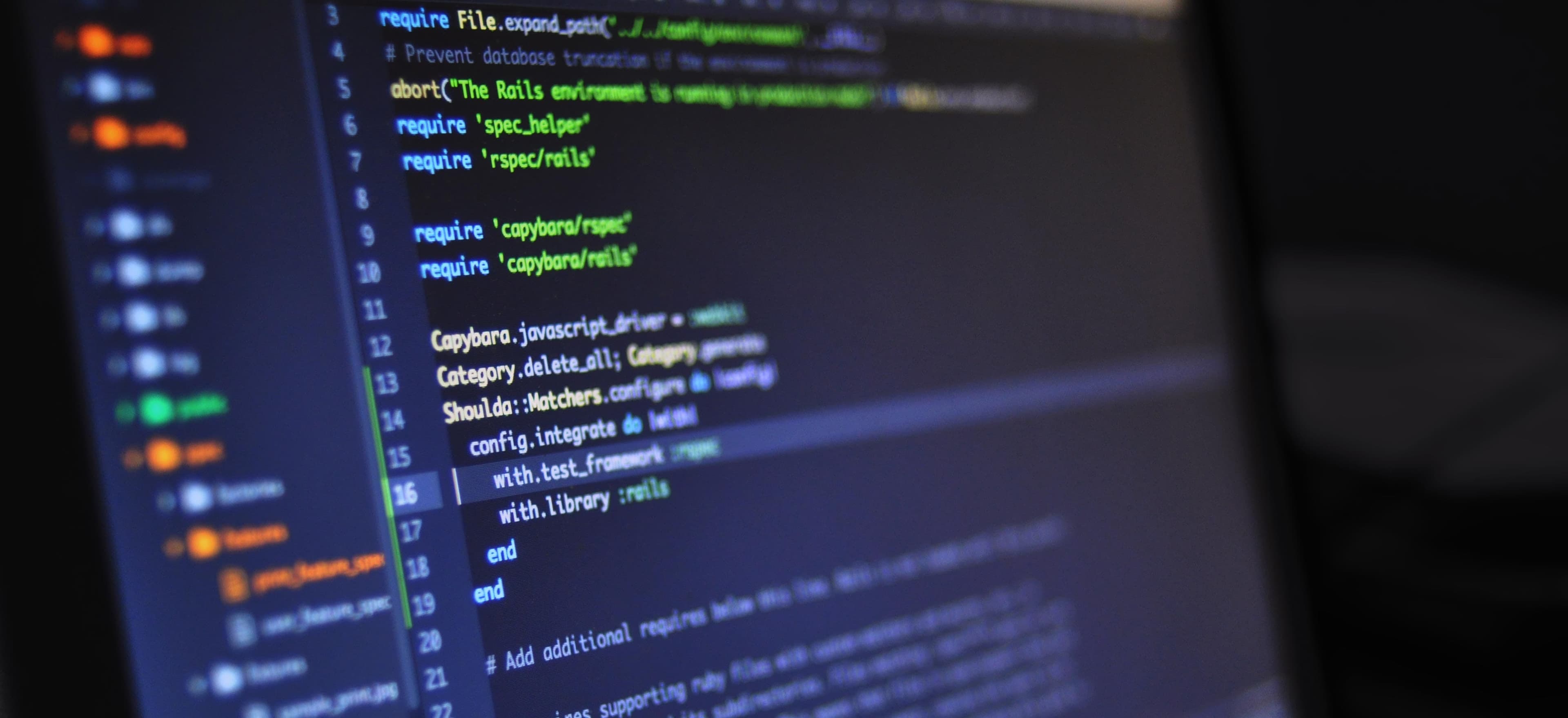
- Published on
Mastering Context Injection in JAX-RS for Beginners
If you are venturing into the world of Java web development, specifically with JAX-RS (Java API for RESTful Web Services), you might have come across the concept of context injection. Context injection allows you to access various contextual information within your JAX-RS resources and providers. This can include details about the incoming HTTP request, the application, or the environment in which the application is running. In this post, we will delve into the fundamentals of context injection in JAX-RS, its importance, and how to effectively leverage it.
Understanding Context in JAX-RS
In the realm of JAX-RS, context refers to the contextual information associated with the current request, application, or environment. This information can be vital for making decisions or performing actions within your JAX-RS components such as resource classes, entity providers, or filters.
The Context interface in JAX-RS provides a way to access this contextual information. It defines methods to obtain instances of various context classes representing different contextual information, including Request, UriInfo, HttpHeaders, SecurityContext, Providers, and more.
Importance of Context Injection
Context injection plays a crucial role in simplifying and enhancing the capabilities of JAX-RS components. By injecting context, you can obtain valuable information without the need for complex manual extraction from the request or other sources. This leads to cleaner and more maintainable code, as well as improved testability by allowing easy mocking of contextual information.
Moreover, context injection promotes the adherence to the principle of Inversion of Control (IoC), wherein the control over the dependencies is inverted from the component to the framework, in this case, JAX-RS. This results in more modular and loosely coupled components, thereby increasing the overall flexibility and extensibility of the application.
Context Injection in JAX-RS
To demonstrate context injection in JAX-RS, let's consider a simple resource class that handles HTTP GET requests for a hypothetical "Product" entity.
@Path("/products")
public class ProductResource {
@Context
private UriInfo uriInfo;
@GET
@Path("/{id}")
public Response getProductById(@PathParam("id") int productId) {
// Accessing the injected UriInfo context
URI productUri = uriInfo.getAbsolutePathBuilder().build();
// ...
}
// Other methods
}
In the above example, the @Context
annotation is used to inject the UriInfo
context into the uriInfo
field within the ProductResource
class. This allows easy access to URI-related information within the getProductById
method. The UriInfo
instance provides methods for building and accessing various aspects of the request URI.
Why Context Injection?
1. Reduced Boilerplate Code:
Without context injection, you would have to manually obtain the UriInfo
instance from the request's context, leading to repetitive boilerplate code across various methods and classes, as well as the risk of inconsistency.
2. Simplified Testing: With context injection, you can easily mock the context objects during unit testing, thereby isolating the testing scope to the specific method being tested without worrying about the complexities of setting up the contextual information.
3. Encourages Modularity: Context injection encourages the separation of concerns by allowing each component to focus solely on its designated functionality, rather than being burdened with extracting contextual details.
Supported Context Types
JAX-RS provides support for context injection of various types, some of which include:
UriInfo
: For accessing URI-related information.HttpHeaders
: For accessing HTTP header details.SecurityContext
: For obtaining security-related information.Providers
: For accessing provider instances, such as message body readers and writers.
Code Example: Accessing HttpHeaders
Let's take a quick look at how context injection can be utilized to access HTTP headers within a JAX-RS resource class.
@Path("/example")
public class ExampleResource {
@Context
private HttpHeaders headers;
@GET
public Response getHeaderValue(@HeaderParam("Authorization") String authorizationHeader) {
// Accessing the injected HttpHeaders context
String userAgent = headers.getHeaderString("User-Agent");
// ...
}
// Other methods
}
In this example, the @Context
annotation injects the HttpHeaders
context into the headers
field, allowing easy access to various HTTP header values within the getHeaderValue
method. This simplifies the extraction of header information and promotes a cleaner, more readable codebase.
Why HttpHeaders Context Injection?
Improved Readability:
By injecting the HttpHeaders
context, the code becomes more readable as the intention to access HTTP headers is clearly conveyed through the use of context injection.
Simplified Maintenance: In the absence of context injection, obtaining header values would involve repeated boilerplate code, leading to code duplication and increased maintenance efforts.
Custom Context Providers
In addition to the built-in context types, JAX-RS allows you to define and inject custom context provider classes. This can be achieved using the @Context
annotation along with a custom context provider class that implements the javax.ws.rs.ext.Providers
interface.
Creating custom context providers can be beneficial when you need to encapsulate and centralize the logic for providing custom contextual information that is not directly available through the built-in context types.
Code Example: Custom Context Provider
Consider a scenario where you want to inject a custom context object, CustomContext
, into your JAX-RS resource classes. First, you need to create a custom context provider class that implements the Providers
interface.
@Provider
public class CustomContextProvider implements Providers {
@Context
private MessageContext messageContext;
public CustomContext getContext() {
// Logic to create and return the custom context
// ...
}
// Other methods
}
In this example, CustomContextProvider
is a custom provider class that injects the MessageContext
context using the @Context
annotation. It contains a method getContext
to provide the custom context instance, which can then be injected into JAX-RS resource classes.
Why Custom Context Providers?
Encapsulation of Logic: Custom context providers encapsulate the logic for creating and providing custom contextual information, promoting a cleaner and more maintainable codebase by adhering to the principle of encapsulation.
Reusability: By centralizing the logic within custom context providers, the same custom context can be easily injected into multiple resource classes without duplicating the creation logic.
Best Practices for Context Injection
When working with context injection in JAX-RS, it's important to adhere to certain best practices to ensure the effectiveness and maintainability of your codebase.
1. Use Field Injection Sparingly:
While field injection with the @Context
annotation is convenient, it can lead to tight coupling and make unit testing more challenging. Consider using constructor or method parameter injection for better control and testability.
2. Maintain Consistency:
Follow a consistent naming convention for the injected context fields to improve readability and maintainability. For example, prefix context fields with ctx
or use descriptive names to indicate the type of context being injected.
3. Be Mindful of Scope: Understand the scope of the injected context objects and avoid storing them as instance variables unless necessary, as some context objects might have request-specific or method-specific relevance.
4. Utilize Proper Exception Handling:
When working with injected context objects, be mindful of potential exceptions that may arise, such as NullPointerException
when accessing context fields. Handle such scenarios gracefully to prevent unexpected runtime failures.
The Last Word
Mastering context injection in JAX-RS is fundamental for harnessing the full potential of the framework and building robust, maintainable web applications. By understanding the importance of context injection, leveraging the supported context types, exploring custom context providers, and following best practices, you can elevate your proficiency in JAX-RS development and streamline the handling of contextual information within your web resources and providers.
In essence, context injection empowers you to focus on the business logic of your JAX-RS components while letting the framework take care of providing the necessary contextual information, thereby promoting cleaner, modular, and testable code.
Start integrating context injection into your JAX-RS applications, and unlock a world of streamlined development and improved code quality!
Note: This blog post covers the fundamentals of context injection in JAX-RS, serving as a comprehensive guide for beginners aiming to grasp the concept and its practical implementation. Mastery of context injection in JAX-RS is an essential skill for Java developers venturing into web services and RESTful API development.