Solving Scalability in Enterprise Java: Expert Tips!
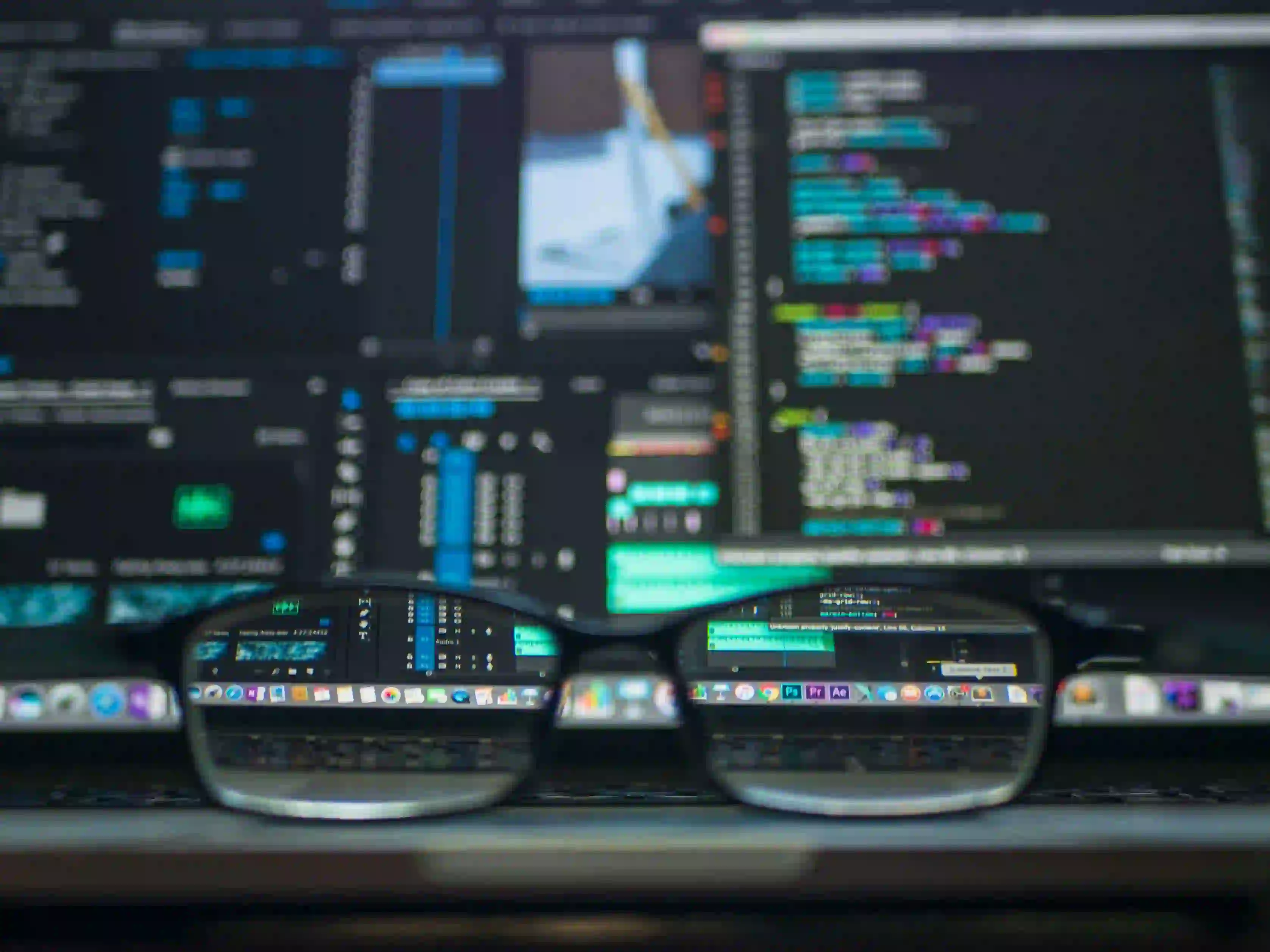
Solving Scalability in Enterprise Java: Expert Tips!
In today’s rapidly evolving digital landscape, the demand for scalable enterprise applications has reached new heights. As data and user numbers continue to surge, businesses are increasingly reliant on applications that can effortlessly accommodate such growth. In this vein, Java has emerged as a leading choice for constructing robust, scalable enterprise systems due to its powerful ecosystem and high-performance capabilities.
Understanding Scalability in Java
The Basics of Scalability
Scalability, in the context of software development, refers to the ability of a system to handle increasing workloads without compromising performance. In Java, scalability is typically approached through two primary avenues: vertical scalability and horizontal scalability.
Vertical scalability involves increasing the capacity of a single server, often by adding more CPUs, memory, or disk space. This approach comes with limitations, as it can only scale up to the capacity of a single server.
Horizontal scalability, on the other hand, revolves around adding more servers to distribute the load. While it offers better potential for handling larger workloads, it requires careful design and architecture to ensure seamless scalability.
Why Java for Enterprise Scalability?
Java's suitability for building scalable enterprise applications stems from a myriad of features. Its platform independence, strong and versatile standard library, and active community support make it an ideal choice for tackling the challenges of building scalable systems.
Common Scalability Challenges in Java
Building scalable applications in Java entails navigating through various challenges, including managing database connections, session management, and efficient garbage collection. These hurdles must be addressed thoughtfully to ensure a system's ability to scale effectively.
Expert Tips for Enhancing Scalability in Java
In the quest to enhance the scalability of Java applications, several strategies and best practices can significantly impact overall performance.
Code Refactoring for Performance
In the pursuit of optimal performance, code refactoring plays a pivotal role. By rethinking and rewriting code to improve its structure and efficiency, developers can pave the way for enhanced scalability.
Avoid Synchronization Overhead
One common area for optimization is synchronization overhead. Consider the following example:
// Original synchronized block
public synchronized void performOperation() {
// Critical section
}
// Optimized synchronization using Lock interface
private final Lock lock = new ReentrantLock();
public void performOperation() {
lock.lock();
try {
// Critical section
} finally {
lock.unlock();
}
}
In scenarios where multiple threads compete for shared resources, utilizing the Lock
interface to manage synchronization can yield performance improvements. However, it's essential to judiciously assess the trade-offs and apply such optimizations only when necessary.
Lazy Loading
Another effective strategy for improving scalability is the implementation of lazy loading. By deferring the loading of non-essential resources until they are actually required, unnecessary resource usage is minimized, thus enhancing scalability.
Effective Use of Java Collections
The choice of Java collections can significantly impact the scalability of an application. Different types of collections offer distinct performance characteristics, and selecting the appropriate one can make a notable difference in the system's ability to scale.
Choosing the Right Collection
When choosing between collections such as ArrayList
and LinkedList
, it's crucial to consider factors like the expected size of the collection, the frequency of insertions and deletions, and the need for random access. Tailoring the collection choice to the specific requirements of the use case can lead to improved scalability.
Optimizing Database Interactions
Efficient database interactions are fundamental to the scalability of Java applications. Through careful optimization and utilization of relevant techniques, the impact of database operations on an application's scalability can be minimized.
Connection Pooling
Connection pooling is a widely adopted technique for enhancing the scalability of database interactions. By reusing existing connections, connection pooling reduces the overhead of establishing new connections and can significantly improve overall performance.
Caching Strategies
Caching plays a crucial role in improving the scalability of applications by reducing the load on primary resources. Smart implementation of caching mechanisms can lead to substantial performance enhancements, especially in scenarios involving repetitive data retrieval.
Implementing Caching
Integrating caching frameworks like EHCache or leveraging in-memory data stores such as Redis enables Java applications to efficiently cache frequently accessed data. This, in turn, alleviates the strain on backend systems and contributes to improved scalability.
Scaling with Microservices
The paradigm of microservices offers numerous benefits for scalability, as it facilitates the development of independently deployable and scalable components. Transitioning from a monolithic architecture to a microservices-based approach can empower enterprises to achieve greater scalability and agility.
Microservices Architecture
Microservices embrace the principles of modularity, fault isolation, and independent scaling, enabling organizations to scale individual components as needed. This further strengthens the overall scalability and resilience of the application architecture.
Leveraging Cloud Solutions
Cloud platforms such as AWS and Azure provide a compelling environment for enhancing the scalability of Java applications. With features like auto-scaling and managed services, organizations can capitalize on the scalability advantages offered by cloud infrastructure.
Cloud Scalability
Cloud platforms offer the flexibility to dynamically scale resources based on fluctuating workloads. Auto-scaling features, coupled with managed services, relieve organizations of much of the burden associated with scalability, allowing them to focus on delivering value through their applications.
Tools and Frameworks to Aid Scalability
Several tools and frameworks are instrumental in supporting the development of scalable Java applications. By leveraging these technologies, developers can streamline the process of building and maintaining scalable systems.
Spring Boot for Microservices
Spring Boot has emerged as a prominent choice for building microservices due to its streamlined development experience and strong support for scalable architecture.
Apache Kafka for Event Streaming
Apache Kafka’s distributed, resilient, and high-throughput nature makes it an invaluable asset for handling event streaming at scale.
Docker for Containerization
Docker provides a lightweight, portable containerization solution that simplifies deployment and scaling of Java applications.
Kubernetes for Orchestration
Kubernetes excels in orchestrating containerized applications at scale, delivering automation and efficiency across distributed environments.
Case Studies: Java Scalability Success Stories
Real-world case studies offer compelling insights into how Java’s scalability challenges have been overcome, often through the strategic application of the concepts and tools discussed. Let’s delve into a couple of notable success stories.
Case Study 1: e-commerce Platform X
E-commerce Platform X, which experienced rapid growth, successfully addressed scalability challenges by adopting a microservices architecture with Spring Boot. By leveraging Kubernetes for orchestration and Apache Kafka for event streaming, the platform achieved remarkable scalability, accommodating a surge in user traffic without compromising performance.
Case Study 2: Financial Service Provider Y
Financial Service Provider Y enhanced the scalability of its trading platform by optimizing database interactions through connection pooling. The platform’s transition to a cloud-based infrastructure on AWS, with robust auto-scaling capabilities, further fortified its scalability and resilience in the face of fluctuating demand.
Closing Remarks
In today's dynamic technological landscape, scalability stands as a critical pillar for the success of enterprise applications. Java's intrinsic capabilities, augmented by best practices and cutting-edge tools, equip organizations to surmount scalability challenges and deliver exceptional performance at scale. As application requirements continue to evolve, embracing scalability as an integral aspect of the development process is imperative for fostering resilience and maintaining a competitive edge.
Call-to-Action
We encourage you to share your experiences, challenges, and successes in scaling Java applications. Join the conversation and delve deeper into the realm of scalability in enterprise Java. Your insights and contributions are valuable in fostering a vibrant community of learning and growth.
As you embark on your journey to mastering scalability in Java, remember that the quest for scalability is not a solitary pursuit – it thrives in the exchange of knowledge and experiences. Let’s embark on this adventure together, striving for scalable, resilient, and high-performing Java applications.