Boosting Efficiency: Mastering Spring Data JPA Named Queries
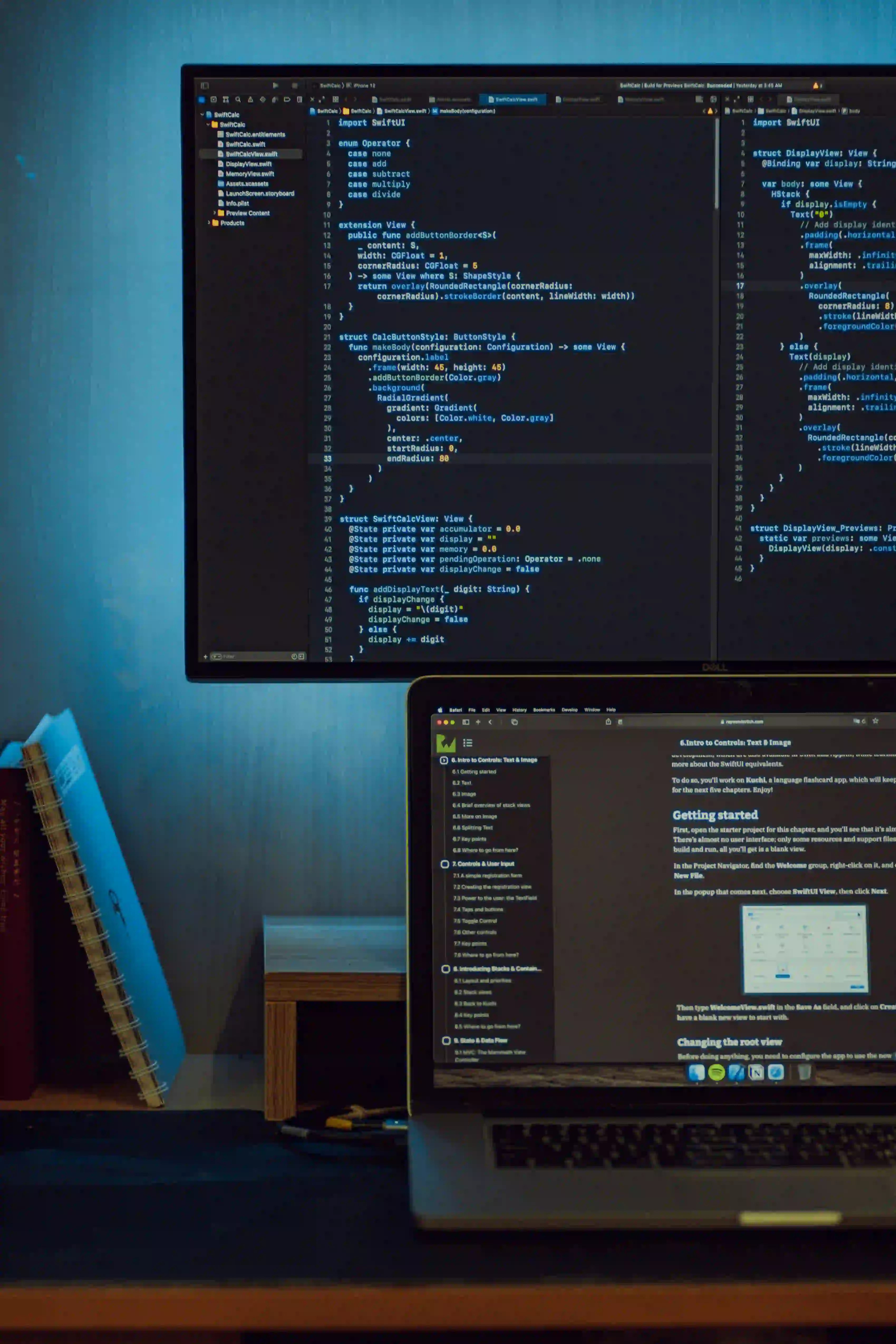
Boosting Efficiency: Mastering Spring Data JPA Named Queries
In the fast-paced world of software development, efficiency is key. One powerful way to enhance efficiency in Java-based applications is through the use of Spring Data JPA Named Queries. This feature not only speeds up the development process but also contributes to cleaner, more maintainable code. In this detailed guide, we'll delve into the nuances of mastering Spring Data JPA Named Queries to turbocharge your Java applications.
Understanding Spring Data JPA Named Queries
Before we dive into the specifics, let's establish a foundational understanding of what Spring Data JPA and Named Queries are. Spring Data JPA (Java Persistence API) is a part of the larger Spring Data family, designed to simplify data access operations in Java applications by leveraging JPA. Named Queries, on the other hand, are pre-defined queries expressed directly in the domain model, serving as a repository of SQL or JPQL (Java Persistence Query Language) queries.
Why use Named Queries? They offer several compelling benefits:
- Performance Optimizations: Named Queries allow for the query statement to be precompiled and validated at application startup, which can potentially improve performance.
- Code Clarity and Maintenance: By defining queries directly within the entity class or in an XML file, it promotes separation of concerns and keeps the repository interfaces clean.
- Reduced Errors: Having a single source for query definitions minimizes the risk of typo-related bugs and simplifies debugging.
Defining Named Queries in Spring Data JPA
Spring Data JPA provides two primary ways to define Named Queries:
- Annotation-Based Configuration: Directly within the JPA entity class using the
@NamedQuery
and@NamedQueries
annotations. - XML Configuration: By specifying queries in an
orm.xml
file located in theMETA-INF
directory.
Let's explore both methods with examples.
1. Annotation-Based Named Queries
Consider an entity Book
in a library management system. We can define a Named Query to find books by title.
@Entity
@NamedQuery(name = "Book.findByTitle",
query = "SELECT b FROM Book b WHERE b.title = :title")
public class Book {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
private String title;
// Standard getters and setters
}
In the @NamedQuery
annotation, name
uniquely identifies the query, following a convention (entity name followed by the operation), while query
defines the JPQL query itself.
2. XML Configuration
XML configuration is equally straightforward. You define your Named Queries in an orm.xml
file like so:
<entity-mappings xmlns="http://java.sun.com/xml/ns/persistence/orm">
<named-query name="Book.findByTitle">
<query><![CDATA[SELECT b FROM Book b WHERE b.title = :title]]></query>
</named-query>
</entity-mappings>
This approach is particularly useful when you have a large number of Named Queries or prefer to keep your domain model classes free of persistence-specific annotations.
Implementing Named Query in Repositories
Once Named Queries are defined, leveraging them in repository interfaces is a breeze. Spring Data JPA repositories can automatically detect and use Named Queries based on method names.
public interface BookRepository extends JpaRepository<Book, Long> {
List<Book> findByTitle(String title);
}
Here, the findByTitle
method corresponds to the Book.findByTitle
Named Query. Spring Data JPA handles the implementation, making it incredibly efficient to execute complex queries.
Best Practices for Using Named Queries
While Named Queries are extremely useful, following some best practices can amplify their benefits:
- Keep Queries Readable: Opt for clarity over cleverness in your query definition. Maintainability is key.
- Use Named Parameters: Instead of positional parameters, named parameters (
:title
) make the query more readable and less error-prone. - Organize Queries Thoughtfully: Whether using annotations or XML, keep your Named Queries organized and easy to find.
- Evaluate Query Performance: Always check the performance of your Named Queries, especially for complex joins or operations.
To Wrap Things Up
Spring Data JPA Named Queries stand out as a robust feature for enhancing the efficiency and maintainability of Java applications. By predefining queries, you can achieve significant performance optimizations, reduce the likelihood of errors, and maintain cleaner repository interfaces. Whether you're a seasoned Java developer or new to the Spring ecosystem, mastering Named Queries in Spring Data JPA can be a game-changer for your data access operations.
To further explore Spring Data JPA and Named Queries, the official Spring Data JPA documentation (Spring Data JPA) and the JPA specification (JPA 2.1 Specification) offer comprehensive insights and examples to deepen your understanding and skillset.
Embrace the power of Named Queries in your next Java project, and experience the difference in development speed, efficiency, and code quality.
Happy coding!