Boosting Code Flexibility with Visitor Design Pattern
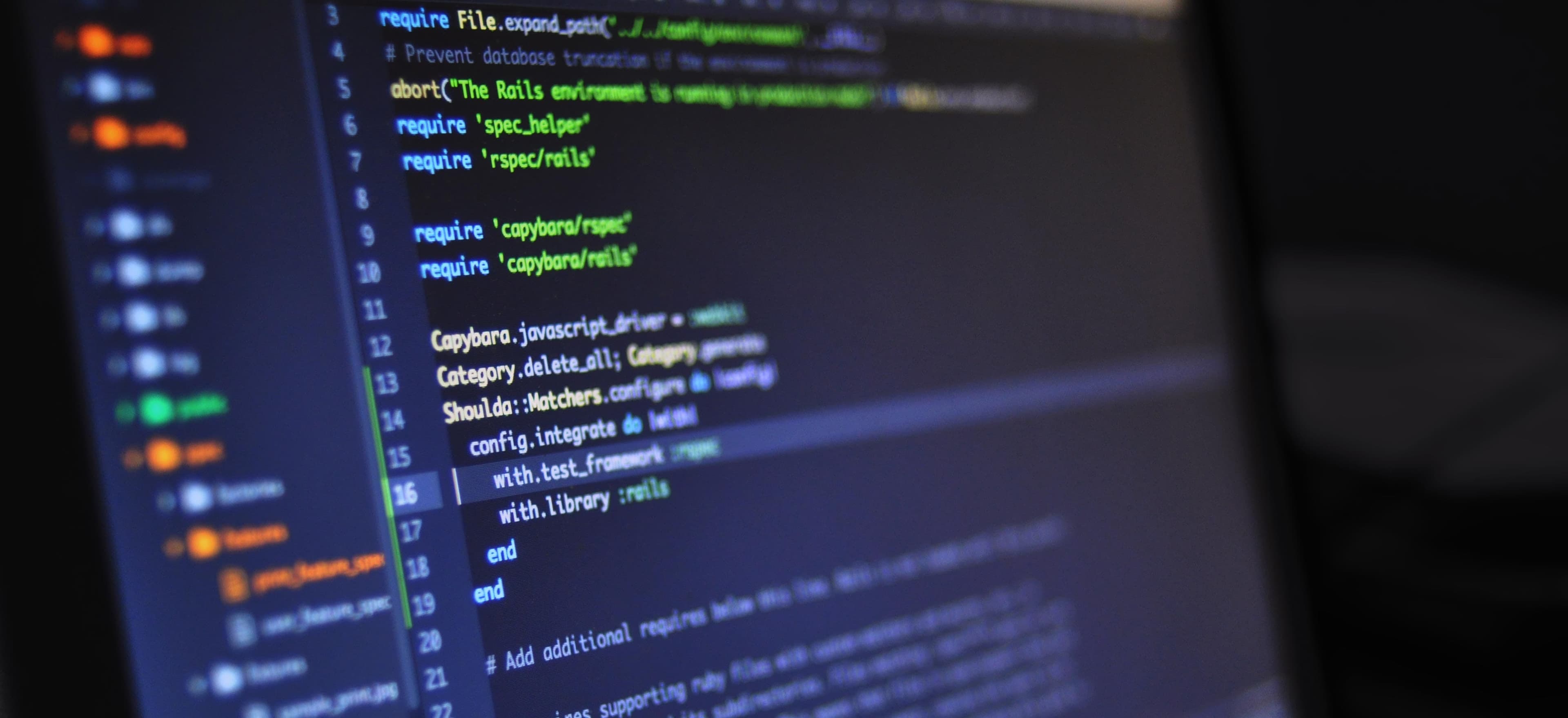
- Published on
Boosting Code Flexibility with Visitor Design Pattern
In the realm of object-oriented programming, developers often encounter scenarios where adding new operations to classes with static structures becomes a challenge. This is where the Visitor design pattern comes to the rescue. The Visitor pattern allows for the addition of new operations to classes without altering their structures, resulting in enhanced code flexibility and maintainability.
What is the Visitor Design Pattern?
The Visitor design pattern is a behavioral pattern that enables defining new operations on an object structure without changing the classes of the elements on which it operates. It achieves this by separating the algorithm from the object structure on which it operates.
How does it work?
The Visitor pattern involves the following key components:
-
Visitor: This interface or abstract class declares a
visit()
method for each class of element in the object structure. Eachvisit()
method accepts an element of a specific class as an argument. -
ConcreteVisitor: This class implements the
Visitor
interface and defines the operations to be performed on each element of the object structure. -
Element: This interface or abstract class declares an
accept()
method that accepts aVisitor
as an argument. Each element class in the object structure implements this method. -
ConcreteElement: These classes represent the elements of the object structure and implement the
accept()
method to call the appropriatevisit()
method on theVisitor
. -
ObjectStructure: This class holds a collection of
Element
objects and provides a way to iterate over them, invoking theaccept()
method of each element.
Example Implementation
Let's consider an example where we have a set of geometric shapes (e.g., circles, squares, triangles) and we want to calculate the area and perimeter of each shape without altering their class structures. We can employ the Visitor design pattern to achieve this.
// Visitor interface
public interface ShapeVisitor {
void visit(Circle circle);
void visit(Square square);
void visit(Triangle triangle);
}
// Concrete visitor
public class AreaCalculator implements ShapeVisitor {
@Override
public void visit(Circle circle) {
// Calculate area of circle
}
@Override
public void visit(Square square) {
// Calculate area of square
}
@Override
public void visit(Triangle triangle) {
// Calculate area of triangle
}
}
// Element interface
public interface Shape {
void accept(ShapeVisitor visitor);
}
// Concrete elements
public class Circle implements Shape {
// Other methods and attributes
@Override
public void accept(ShapeVisitor visitor) {
visitor.visit(this);
}
}
// Similar concrete classes for Square and Triangle
In this example, the ShapeVisitor
interface declares methods for visiting different shapes, while the AreaCalculator
concrete visitor implements the operations for calculating areas of different shapes. The Shape
interface declares the accept()
method, which is implemented by concrete shape classes to call the appropriate visit()
method on the visitor.
This approach allows adding new operations (e.g., calculating perimeter, drawing) on shapes without modifying their class structures.
Benefits of using the Visitor Design Pattern
-
Separation of Concerns: The Visitor pattern separates the algorithm from the object structure, promoting a cleaner and more maintainable codebase.
-
Adding new operations: It allows for adding new operations without modifying the classes of the elements, which is especially useful when dealing with third-party or legacy code.
-
Enhanced flexibility: The pattern enables the addition of new operations or algorithms without affecting the existing codebase, thereby boosting flexibility.
-
Double Dispatch: It enables double dispatch, meaning the method to be invoked is determined at runtime based on the object type. This facilitates more dynamic behavior.
When to use the Visitor Design Pattern?
The Visitor pattern is beneficial in scenarios where the object structure is stable, but new operations (algorithms) need to be added to it frequently. It is also useful when dealing with a hierarchy of classes where operations need to be applied across different class types without altering their structures.
Wrapping Up
In the journey of software development, the Visitor design pattern serves as a powerful tool to enhance the flexibility and maintainability of codebases. By decoupling the algorithms from the object structure, it allows for the seamless addition of new operations, thereby ensuring the codebase remains open for extension but closed for modification.
By understanding and leveraging the Visitor design pattern, developers can craft elegant solutions to accommodate new requirements without causing ripples across the existing codebase.
Visit refactoring.guru for further insights into the Visitor design pattern.
Now go forth and let the Visitor pattern empower your code with enhanced flexibility and maintainability!