Fixing View Resolution: Master InternalResourceViewResolver
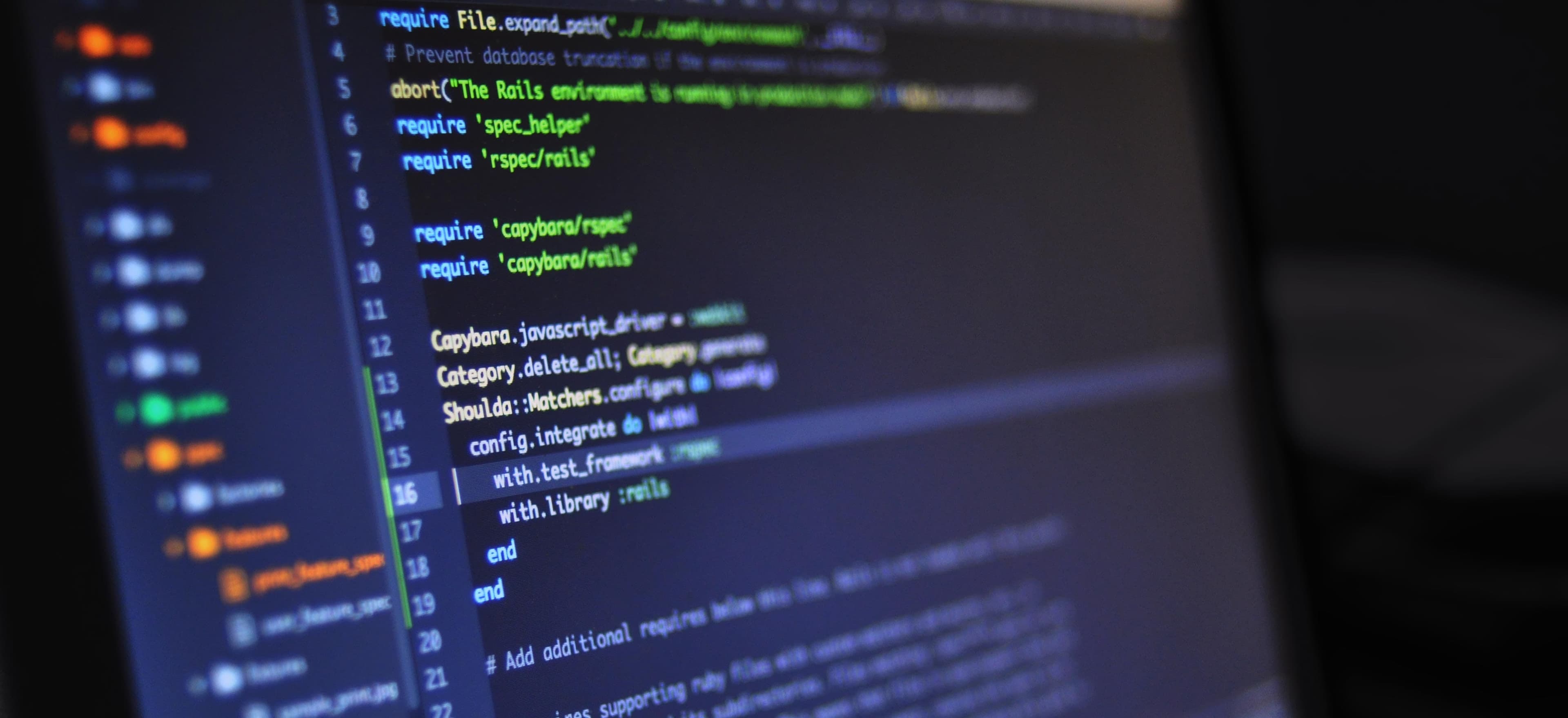
- Published on
Solving View Resolution Issues with Mastering InternalResourceViewResolver in Spring MVC
In a Spring MVC web application, configuring and managing view resolution is a crucial aspect. The InternalResourceViewResolver
is a core component that tackles the task of resolving view names to actual view resources. Understanding and effectively utilizing this resolver is essential for seamless view management in a Spring MVC application.
What is InternalResourceViewResolver?
In Spring MVC, InternalResourceViewResolver
is a class that resolves the view names returned by the controller handlers to JSP files. It prefaces and appends the prefix and suffix to the view names to translate them into the actual JSP page locations.
Common Issues with InternalResourceViewResolver
View resolution issues can often pop up during development, causing frustration and hindering progress. Typical problems include:
- View Not Found: The resolver is unable to locate the specified view.
- Incorrect View Path: The resolved view path doesn't match the expected location.
- Missing Prefix/Suffix: Incorrect configuration of the prefix and suffix.
Mastering InternalResourceViewResolver
To effectively utilize InternalResourceViewResolver
and resolve common issues, follow these best practices and strategies:
1. Configure InternalResourceViewResolver in the Spring Configuration
First, configure the InternalResourceViewResolver
in the Spring configuration (often defined in a servlet XML file).
<bean class="org.springframework.web.servlet.view.InternalResourceViewResolver">
<property name="prefix" value="/WEB-INF/views/"/> <!-- Prefix for JSP files -->
<property name="suffix" value=".jsp"/> <!-- Suffix for JSP files -->
</bean>
Here, the prefix
property denotes the common prefix for all views, such as "/WEB-INF/views/", and the suffix
property specifies the file extension, such as ".jsp".
2. Verify View Location and File Extension
Ensure that the JSP files are placed in the correct directory (/WEB-INF/views/
in this case) and that their file extensions match the ones specified in the resolver (".jsp").
3. Understanding the View Naming in Controllers
In controller methods, return the view names without the prefix and suffix. For example, if the JSP file is "/WEB-INF/views/home.jsp", the return value from the controller method should simply be "home".
4. Check servlet mapping in web.xml
Ensure that the url-pattern
specified for the DispatcherServlet in the web.xml
matches the URL patterns used in the @RequestMapping
annotations in the controller classes.
5. Debugging and Logging
Employ logging statements to track the resolved view names and paths. This helps in diagnosing any discrepancies in the view resolution process.
6. Error Pages and Exception Handling
Be mindful of error pages and exception handling. Improperly configured error pages or exception handling could lead to unexpected view resolution behavior.
Resolving Common Issues
Let’s address the common issues encountered when using InternalResourceViewResolver
.
Issue 1: View Not Found
Problem: The resolver is unable to locate the specified view.
Solution: Verify the view location and ensure that the JSP file is placed in the correct directory as specified by the resolver's prefix. Check for typos in the view name.
Issue 2: Incorrect View Path
Problem: The resolved view path doesn't match the expected location.
Solution: Double-check the prefix and suffix configuration in the resolver. Ensure that the JSP file is in the correct directory and has the appropriate file extension.
Issue 3: Missing Prefix/Suffix
Problem: Incorrect configuration of the prefix and suffix.
Solution: Review the InternalResourceViewResolver
configuration in the Spring context file. Confirm that the prefix and suffix are accurately defined.
Example Usage
Let’s consider a basic example to showcase the usage of InternalResourceViewResolver
in a Spring MVC application.
Suppose we have the following folder structure:
src
└── main
└── webapp
└── WEB-INF
└── views
├── home.jsp
└── error.jsp
The Spring configuration file (dispatcher-servlet.xml
) includes the configuration for InternalResourceViewResolver
:
<bean class="org.springframework.web.servlet.view.InternalResourceViewResolver">
<property name="prefix" value="/WEB-INF/views/"/>
<property name="suffix" value=".jsp"/>
</bean>
In the controller, a method returns the view names without prefix and suffix:
@Controller
public class MyController {
@RequestMapping("/home")
public String home() {
return "home";
}
@RequestMapping("/error")
public String error() {
return "error";
}
}
Here, when the /home
URL is accessed, the home()
method will return "home", and InternalResourceViewResolver
will resolve it to /WEB-INF/views/home.jsp
. Likewise, the /error
URL will resolve to /WEB-INF/views/error.jsp
.
To Wrap Things Up
Effectively mastering InternalResourceViewResolver
in Spring MVC is crucial for seamless view resolution. By understanding its configuration, common issues, and best practices, you can streamline the handling of views in your Spring MVC application. It's essential to be aware of the resolver’s behavior, the correct setup, and the handling of common issues to ensure a smooth and trouble-free view resolution process.
Checkout our other articles