Mastering ConcurrentHashMap: Avoiding Common Pitfalls
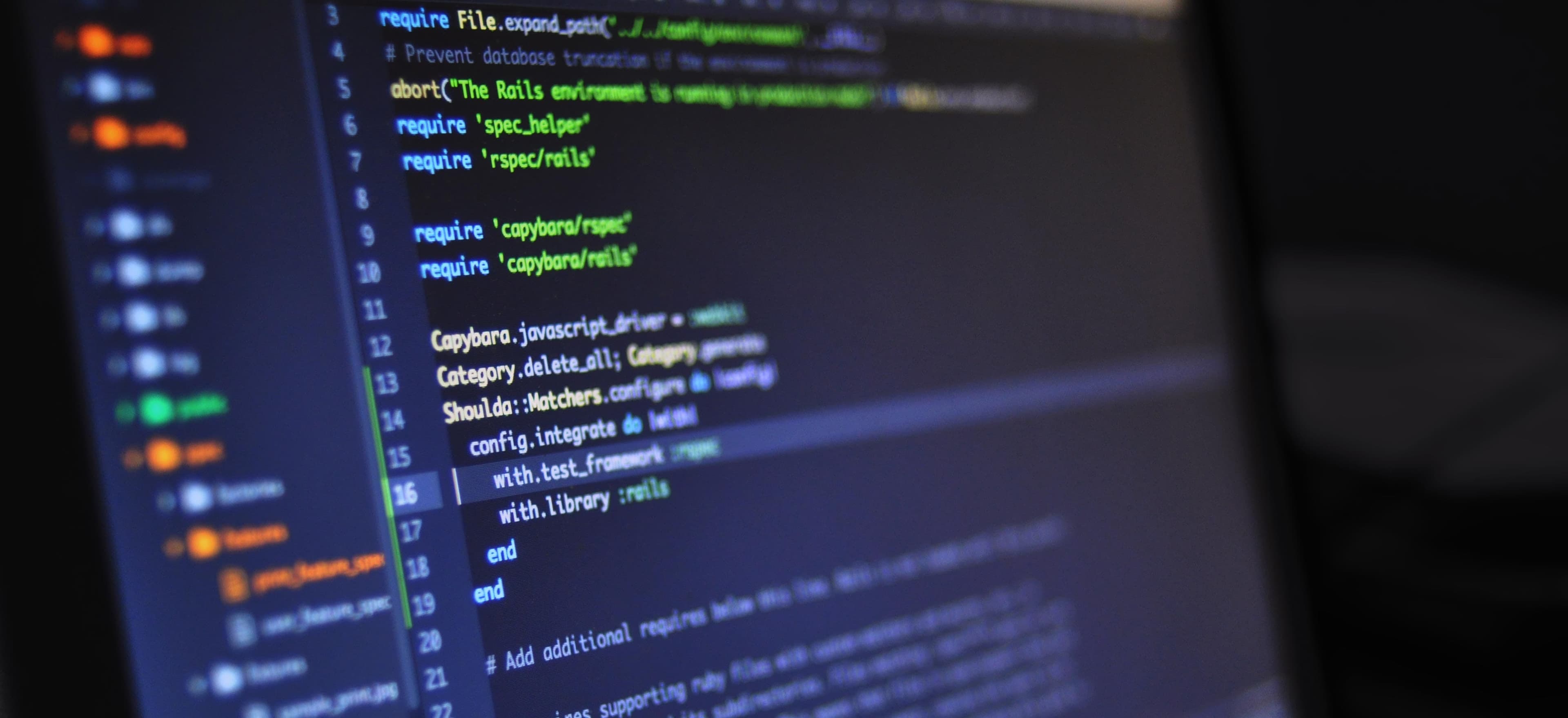
- Published on
Mastering ConcurrentHashMap: Avoiding Common Pitfalls
Concurrency is a fundamental aspect of modern software development. In a multi-threaded environment, concurrent data structures are crucial for ensuring thread safety and avoiding data corruption. In the world of Java, ConcurrentHashMap is a powerful tool for managing concurrent operations. However, improper usage can lead to performance issues, data inconsistencies, and even deadlocks. In this post, we'll explore the common pitfalls associated with ConcurrentHashMap and discuss best practices for utilizing this essential concurrent data structure effectively.
Understanding ConcurrentHashMap
ConcurrentHashMap
is a thread-safe alternative to HashMap
in Java. It provides synchronized access to its entries without locking the entire map. Under the hood, it achieves this by dividing the data into segments, with each segment guarded by a separate lock. This design allows concurrent read and write operations to different parts of the map, resulting in improved performance in multi-threaded scenarios.
Pitfall 1: Incorrect Synchronization
One of the most common mistakes when using ConcurrentHashMap
is erroneously assuming that all operations are atomic. While individual operations like get
, put
, and remove
are thread-safe, compound operations like "check then act" are not inherently atomic. This can lead to race conditions and unexpected behavior.
// Incorrect synchronization leading to race condition
if (!concurrentMap.containsKey(key)) {
concurrentMap.put(key, value);
}
To address this issue, it's crucial to rely on atomic operations, such as putIfAbsent
for check-then-act scenarios. This ensures that the check and the act are performed atomically and eliminates the need for external synchronization.
concurrentMap.putIfAbsent(key, value);
Pitfall 2: Overlooking the Impact of Iterators
Iterating over a ConcurrentHashMap
requires careful consideration due to its concurrent nature. Unlike a regular HashMap
, the iterator returned by ConcurrentHashMap
may reflect changes made to the map after the iterator was created. This can result in concurrent modification exceptions or missed elements during iteration.
// Potential concurrent modification issue
for (String key : concurrentMap.keySet()) {
if (someCondition) {
concurrentMap.remove(key);
}
}
To avoid concurrent modification issues when iterating over ConcurrentHashMap
, use the Iterator
and its remove
method instead of the enhanced for-loop.
Iterator<String> iterator = concurrentMap.keySet().iterator();
while (iterator.hasNext()) {
String key = iterator.next();
if (someCondition) {
iterator.remove(); // Safe removal using Iterator's remove method
}
}
Pitfall 3: Performance Bottlenecks with Size Estimation
Calculating the size of a ConcurrentHashMap
using the size
method comes with a potential performance penalty. In a concurrent environment with ongoing insertions and deletions, the size
method may need to traverse the entire map, leading to increased overhead.
// Inefficient size estimation
int mapSize = concurrentMap.size();
To mitigate the performance impact of size estimation, consider using alternative approaches, such as maintaining a separate counter for tracking the number of elements added or removed.
// Efficient size estimation using a separate counter
AtomicInteger counter = new AtomicInteger(0);
// Increment the counter upon addition
concurrentMap.put(key, value);
counter.incrementAndGet();
// Decrement the counter upon removal
concurrentMap.remove(key);
counter.decrementAndGet();
// Retrieve the size from the counter
int mapSize = counter.get();
Best Practices for Utilizing ConcurrentHashMap
Now that we've delved into the potential pitfalls of using ConcurrentHashMap
, let's explore some best practices to maximize its effectiveness in concurrent scenarios.
Practice 1: Leverage Atomic Operations
Always favor atomic operations available in ConcurrentHashMap
, such as putIfAbsent
, remove
, and replace
. These methods ensure that compound operations are performed atomically, eliminating the need for external synchronization and minimizing the potential for race conditions.
Practice 2: Use Bulk Operations Wisely
ConcurrentHashMap
provides bulk operations like putAll
and clear
for efficient manipulation of multiple entries. When using these operations, consider their impact on the overall concurrency and performance, especially in scenarios where a large number of entries are being added or removed concurrently.
Practice 3: Employ Concurrent Computing Techniques
When dealing with complex concurrent computations using ConcurrentHashMap
, explore concurrent computing techniques such as compute
, computeIfAbsent
, and computeIfPresent
. These methods provide a way to perform operations atomically and are ideal for scenarios requiring computation based on the current mapping.
Practice 4: Understand Thread-Safety Guarantees
It's essential to have a clear understanding of the thread-safety guarantees provided by ConcurrentHashMap
. While it offers concurrent read and write access, it's important to be aware of the limitations and potential visibility issues, especially when dealing with compound operations and data consistency.
Practice 5: Monitor and Tune for Performance
Regularly monitor the performance of ConcurrentHashMap
in your application. Consider tuning the concurrency level based on the specific workload and usage patterns. The ConcurrentHashMap
constructor allows you to specify the initial concurrency level, and adjusting this parameter can have a significant impact on performance in high-concurrency scenarios.
Bringing It All Together
In the realm of concurrent programming in Java, mastering ConcurrentHashMap
is pivotal for building robust and efficient multi-threaded applications. By understanding the common pitfalls and embracing best practices, developers can harness the full potential of ConcurrentHashMap while avoiding performance bottlenecks and data inconsistencies. With careful consideration of synchronization, iteration, and performance aspects, ConcurrentHashMap
becomes a reliable ally in managing concurrent data access with confidence.
Incorporating these best practices into your concurrent programming arsenal will not only enhance the reliability and performance of your applications but also solidify your expertise in mastering ConcurrentHashMap. As you continue to explore the intricacies of concurrent programming, remember that a thorough understanding of Java's concurrent data structures is the cornerstone of building scalable, responsive, and resilient software systems.
Remember, mastering ConcurrentHashMap isn't just about avoiding common pitfalls—it's about embracing its potential to elevate your concurrent programming skills to new heights.
As you continue your journey in Java development, keep mastering ConcurrentHashMap in your toolkit and watch as your concurrent programming prowess reaches new levels of excellence.
Happy coding!
Link 1: Oracle's ConcurrentHashMap Documentation
Link 2: Understanding Java Concurrency
In this blog post, we delved into the potential pitfalls and best practices for utilizing ConcurrentHashMap, shedding light on essential aspects of its usage in concurrent Java programming. By exploring these insights and incorporating the recommended best practices, developers can harness the full potential of ConcurrentHashMap while avoiding common pitfalls and achieving optimal performance in multi-threaded environments.
Checkout our other articles