Craft Your Java AOP: Boost Apps with Custom Aspects!
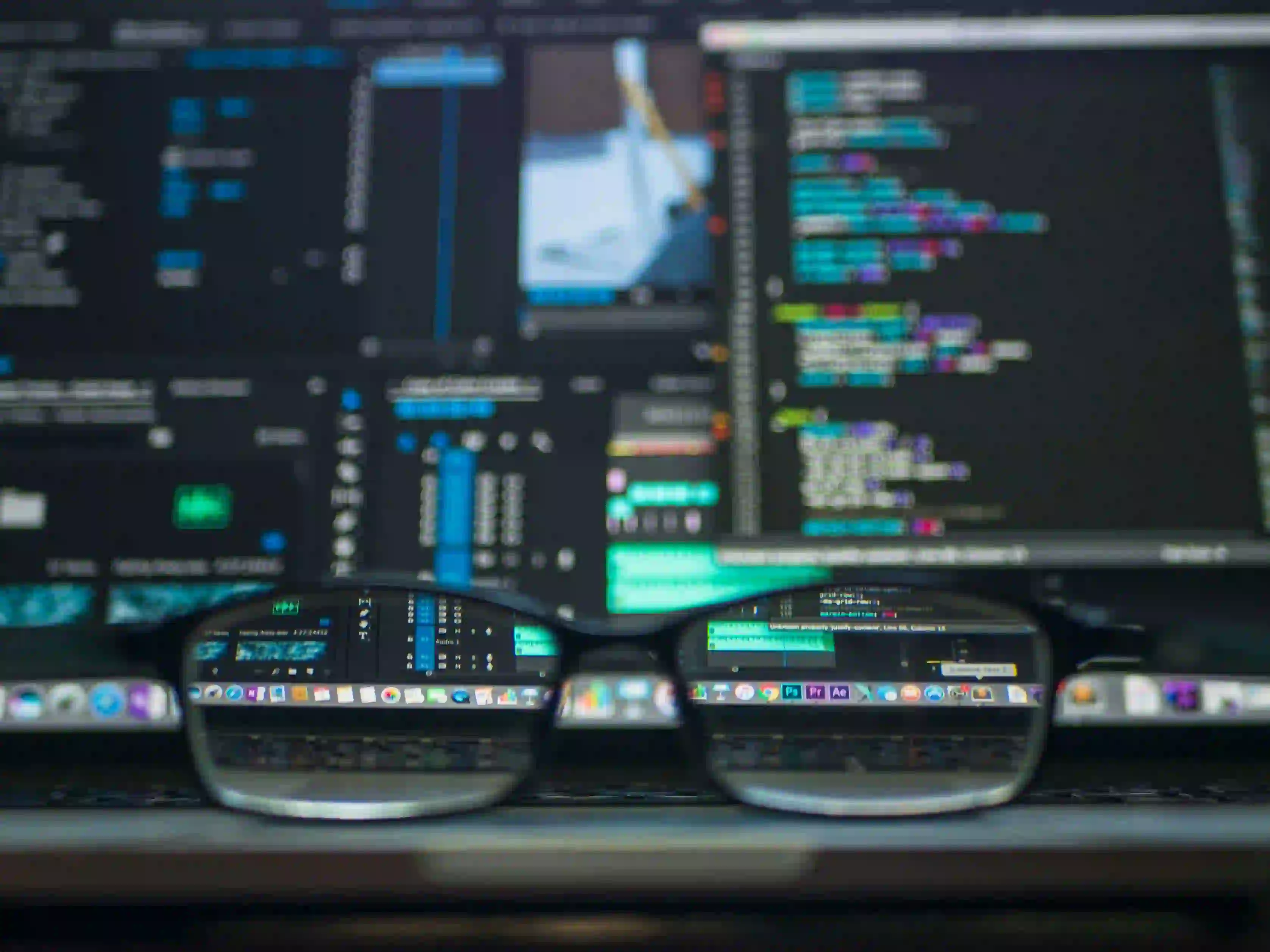
Craft Your Java AOP: Boost Apps with Custom Aspects!
Let us Begin
Statement:
In modern Java development, Aspect-Oriented Programming (AOP) plays a crucial role in creating clean, maintainable, and efficient applications. AOP allows developers to modularize cross-cutting concerns, like logging, security, and data validation, making code more readable and maintainable.
Objective:
In this article, we will cover how to implement custom aspects to boost Java applications. By leveraging AOP, developers can achieve code modularity, separation of concerns, and improved maintenance, resulting in more robust and scalable applications.
What is Aspect-Oriented Programming?
Explanation:
Aspect-Oriented Programming (AOP) is a programming paradigm that complements traditional Object-Oriented Programming (OOP). It allows developers to encapsulate cross-cutting concerns that affect multiple parts of the application. By doing so, AOP promotes better code organization and reusability.
Importance:
AOP is a powerful paradigm in Java development as it helps in separating cross-cutting concerns from the business logic, resulting in cleaner and more maintainable code. Real-world applications benefit greatly from AOP as it improves code readability, promotes modularity, and simplifies the process of adding new features and making changes.
Setting Up the Environment
Instructions:
To set up the environment for Java AOP, you will need the following tools and libraries:
- Java Development Kit (JDK)
- Integrated Development Environment (IDE) like IntelliJ IDEA or Eclipse
- AspectJ or Spring AOP
For setting up AspectJ, you can follow the official installation guide here.
For Spring AOP, you can refer to the official documentation here.
Prerequisites:
Before implementing AOP, it is essential to have a basic understanding of Java syntax, OOP principles, and familiarity with the Spring Framework if using Spring AOP.
Crafting Your First Aspect in Java
Let us Begin to AspectJ and Spring AOP:
AspectJ and Spring AOP are two popular frameworks for implementing AOP in Java. AspectJ provides strong support for AOP and allows developers to define aspects using a specialized syntax. Spring AOP, on the other hand, integrates AOP capabilities into the Spring Framework, making it an attractive choice for Spring-based applications.
Code Snippet and Explanation:
import org.aspectj.lang.annotation.Aspect;
import org.aspectj.lang.annotation.Before;
import org.aspectj.lang.JoinPoint;
@Aspect
public class LoggingAspect {
@Before("execution(* com.example.service.*.*(..))")
public void beforeServiceMethodExecution(JoinPoint joinPoint) {
System.out.println("Before executing: " + joinPoint.getSignature());
}
}
In this code snippet, we define a simple logging aspect using AspectJ. The @Aspect
annotation marks the class as an aspect, and the @Before
annotation indicates that the beforeServiceMethodExecution
method should be executed before the execution of any method in the com.example.service
package. The method takes a JoinPoint
as a parameter, which provides access to the method signature and arguments being executed.
Step-by-Step Guide:
To integrate this aspect into a Java application, follow these steps:
- Add the AspectJ library to your project's dependencies.
- Create the
LoggingAspect
class and define your aspects using annotations. - Enable AspectJ in your application configuration or Spring context.
- Use the defined aspect in your application's code, and run the application to see the logging aspect in action.
Advanced AOP Concepts
Different Types of Advice:
In AOP, advice represents the action taken by an aspect at a particular join point. There are different types of advice:
- Before advice: Executed before the method invocation.
- After returning advice: Executed after a method successfully returns a result.
- After throwing advice: Executed if a method exits by throwing an exception.
- Around advice: Wraps the method invocation and allows the aspect to control the behavior of the advised method.
Each advice type offers a unique way to intercept and modify the behavior of the application.
Pointcut Expressions:
Pointcut expressions define the join points where aspects should be applied. They allow developers to precisely target cross-cutting concerns within the application. An example of a pointcut expression is "execution(* com.example.service.*.*(..))"
, where com.example.service.*.*(..)
represents the package and method signature to target.
Code Snippet and Explanation:
import org.aspectj.lang.annotation.Aspect;
import org.aspectj.lang.annotation.Around;
import org.aspectj.lang.ProceedingJoinPoint;
@Aspect
public class PerformanceAspect {
@Around("execution(* com.example.service.*.*(..))")
public Object measureExecutionTime(ProceedingJoinPoint joinPoint) throws Throwable {
long start = System.nanoTime();
Object result = joinPoint.proceed();
long elapsedTime = System.nanoTime() - start;
System.out.println(joinPoint.getSignature() + " executed in " + elapsedTime + " nanoseconds");
return result;
}
}
In this code snippet, we define an around advice that measures the execution time of methods in the com.example.service
package. The @Around
annotation marks the method as an around advice, and the ProceedingJoinPoint
allows us to control the method invocation, including its execution and return value.
Best Practices and Tips
Tips:
When crafting aspects in Java, consider the following tips:
- Naming conventions: Use meaningful and descriptive names for aspects and advice methods to enhance readability.
- Annotation vs. XML configuration: Use annotation-based configuration for simplicity and conciseness, unless XML configuration is necessary for specific requirements.
- Error handling: Ensure proper error handling within advice methods to prevent unexpected behavior in the application.
Patterns and Strategies:
Several design patterns and strategies work well with AOP, such as the Singleton pattern, Proxy pattern, and Dependency Injection. These patterns can be leveraged to create more maintainable and flexible applications by encapsulating cross-cutting concerns in aspects and applying them across the application.
Final Considerations
Recap:
In this article, we have explored the importance of Aspect-Oriented Programming in Java and how it can benefit Java applications by separating cross-cutting concerns from the core business logic. We have covered the fundamentals of AOP, including setting up the environment, crafting custom aspects, and delving into advanced AOP concepts.
Encouragement:
We encourage you to experiment with creating your own aspects and integrating them into your Java applications. By utilizing AOP, you can significantly improve the modularity, maintainability, and scalability of your codebase, ultimately enhancing your development workflow.
Further Reading:
For further exploration of AOP, AspectJ, Spring AOP, and related topics, consider these resources:
- AspectJ Official Documentation
- Spring AOP Official Documentation
- Books on Aspect-Oriented Programming and Java design patterns
- Reputable community resources and forums for discussions and insights on AOP implementation in Java.
Call to Action
Engage:
We invite you to share your experiences or challenges with implementing AOP in your projects in the comments section. Your insights can provide valuable perspectives for other developers looking to implement AOP in their Java applications.
Subscribe and Share:
If you found this article helpful, consider subscribing to our blog for more Java tutorials, tips, and best practices. Don't forget to share this article with your peers who might find it useful in their Java development journey.
By following the guidelines of Aspect-Oriented Programming, developers can enhance and optimize their Java applications by encapsulating cross-cutting concerns and promoting cleaner, maintainable code. With a solid understanding of AOP, developers can achieve modular, scalable, and efficient Java applications.