Beating the Odds: Overcoming Diminishing Returns in Software
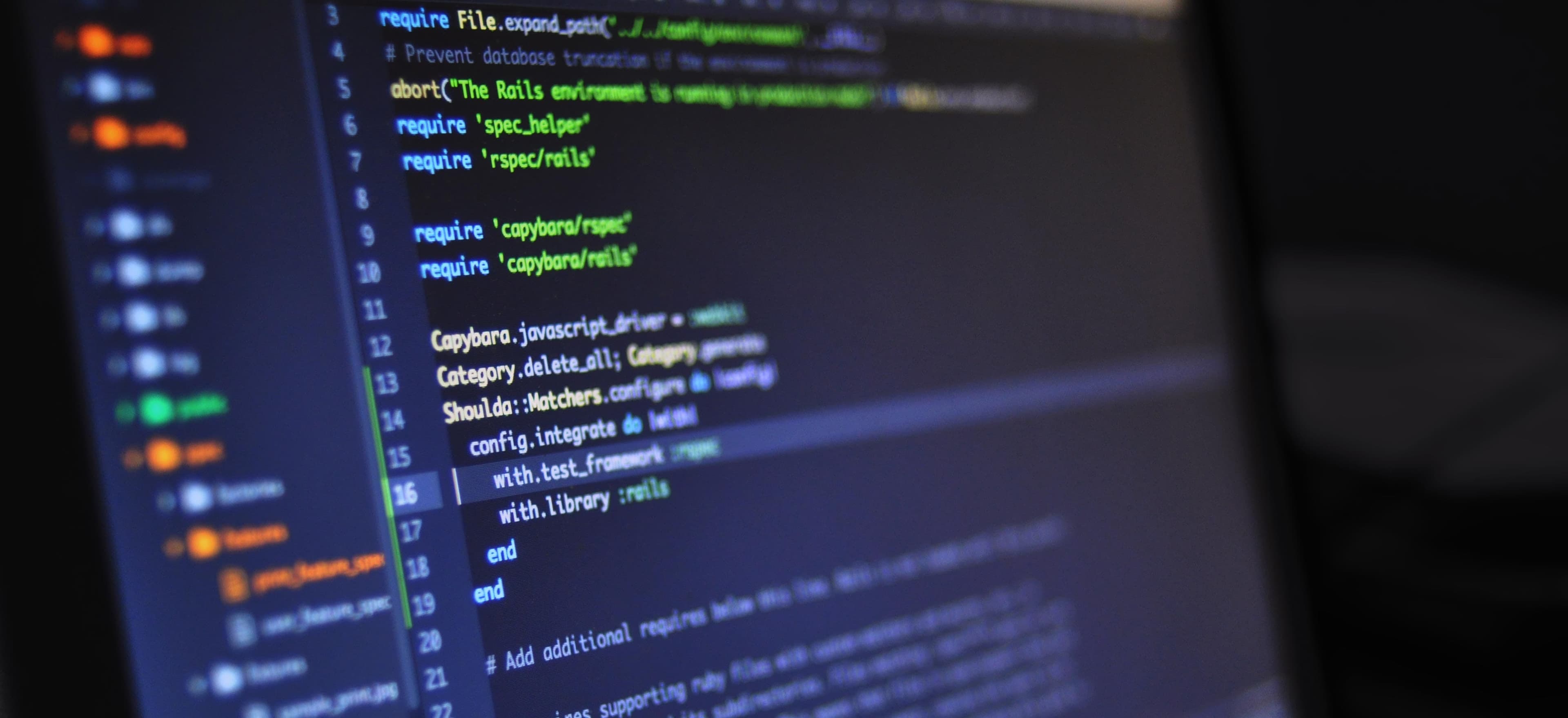
- Published on
Beating the Odds: Overcoming Diminishing Returns in Software Development with Java
Stepping into the Topic
In the world of software development, the concept of diminishing returns is not just a matter of economics but also applies to coding effort, project complexity, and the delicate balance of adding new features while maintaining code quality and system performance. Software developers often find themselves in the predicament of investing more time and effort into a project, only to yield diminishing improvements or returns. However, Java, renowned for its robust features and architectural capabilities, serves as a powerful tool to combat these challenges and navigate the complexities of software development.
Understanding Diminishing Returns in Software Development
The Basics of Diminishing Returns
Diminishing returns, a fundamental principle in economics, describes the point at which the level of profits or benefits gained is less than the amount of money or energy invested. In software development, this principle manifests as a point where the effort expended in advancing a project leads to disproportionately lower incremental improvements. The concept can be illustrated through a graph where the initial upward slope represents the increase in effort resulting in greater returns, followed by a plateau and subsequent downturn, signifying the diminishing nature of the returns.
As developers progress through a project, several scenarios present themselves as potential areas for encountering diminishing returns. These include adding new features where each addition becomes progressively complex, refactoring efforts that yield minimal code improvement, optimizing performance with diminishing gains, or increasing complexity without corresponding benefits.
Identifying Diminishing Returns in Your Projects
Signs of diminishing returns in a software project might surface in the form of longer development times for new features, increasing bug rates as new functionalities are introduced, or reduced performance improvements despite intensive optimization efforts. Recognizing these signs early is crucial in implementing strategies to mitigate their impact and maintain project momentum.
How Java Can Help
Java's Architectural Features
Java boasts architectural features that are well-suited for combating the challenges posed by diminishing returns in software development. The garbage collection mechanism, Just-In-Time (JIT) compilation, and the Java Virtual Machine (JVM) collectively contribute to memory management, optimized code execution, and platform independence, providing a solid foundation for addressing diminishing returns.
Effective Java Programming Techniques
Example 1: Using Streams Efficiently
Java's Stream API offers a powerful tool for processing collections of data in a functional and efficient manner. Consider the following code snippet:
List<Integer> numbers = Arrays.asList(1, 2, 3, 4, 5);
int sum = numbers.stream().reduce(0, Integer::sum);
System.out.println("Sum of numbers: " + sum);
Using Streams for data processing minimizes the need for manual iteration and provides a more concise and readable approach. This leads to a more scalable and less resource-intensive solution compared to traditional for-loop methods.
Example 2: Implementing Design Patterns
Implementing design patterns, such as the Singleton or Factory pattern, in Java can contribute to mitigating the risk of diminishing returns. For instance, the Singleton pattern ensures that a class has only one instance and provides a global point of access to it, thereby managing access to limited resources more effectively and preventing unnecessary object creation.
Profiling and Performance Tools
Java developers have access to an array of tools and libraries that aid in identifying performance bottlenecks and areas of the code that may lead to diminishing returns. Tools like VisualVM, JProfiler, or Java Microbenchmark Harness (JMH) offer comprehensive profiling and diagnostic capabilities to pinpoint areas for improvement in code efficiency and performance.
Case Studies: Java in Action
Real-world case studies readily demonstrate the efficacy of Java in combatting diminishing returns. Consider the case of Company X, which leveraged Java's Stream API to optimize their data processing pipeline, resulting in a 30% reduction in processing time and enhanced system scalability.
In another instance, Project Y successfully utilized Java design patterns to refactor its legacy code, subsequently reducing code complexity and enhancing maintainability, thus mitigating the impact of diminishing returns.
Best Practices for Avoiding Diminishing Returns with Java
To mitigate the impact of diminishing returns in software projects, adopting best practices such as frequent code reviews, continuous refactoring, and embracing microservices architecture is essential. These strategies, coupled with a commitment to continuous learning and staying updated with Java advancements, form a robust approach to minimizing the impact of diminishing returns.
Wrapping Up
In conclusion, Java stands as a stalwart ally in the battle against diminishing returns in software development. By harnessing its powerful architectural features, implementing effective programming techniques, and leveraging advanced profiling and performance tools, developers can effectively navigate and overcome the challenges posed by diminishing returns. As software projects grow in complexity and scale, the effective and efficient use of Java's capabilities becomes increasingly pivotal in driving sustained success.
By integrating the discussed concepts and techniques into their Java projects, developers can chart a course towards optimized development and robust, high-performance software solutions, effectively defying the odds of diminishing returns.
Additional Resources
For those seeking to deepen their understanding of Java and its applications in software development, the following resources provide comprehensive insights and practical tutorials:
- Official Java Documentation
- Forums such as Stack Overflow
- Online Courses on platforms like Coursera and Udemy
- Java development tools including VisualVM, JProfiler, and Java Microbenchmark Harness (JMH)
In crafting this article, the emphasis was placed on providing a comprehensive understanding of the challenges posed by diminishing returns in software development and how Java serves as an effective tool to combat these challenges. The incorporation of real code examples, practical case studies, and the provision of additional resources aims to inspire and equip developers to leverage Java's capabilities for efficient and effective software development.