Avoid Common Pitfalls in Java With These String Tricks
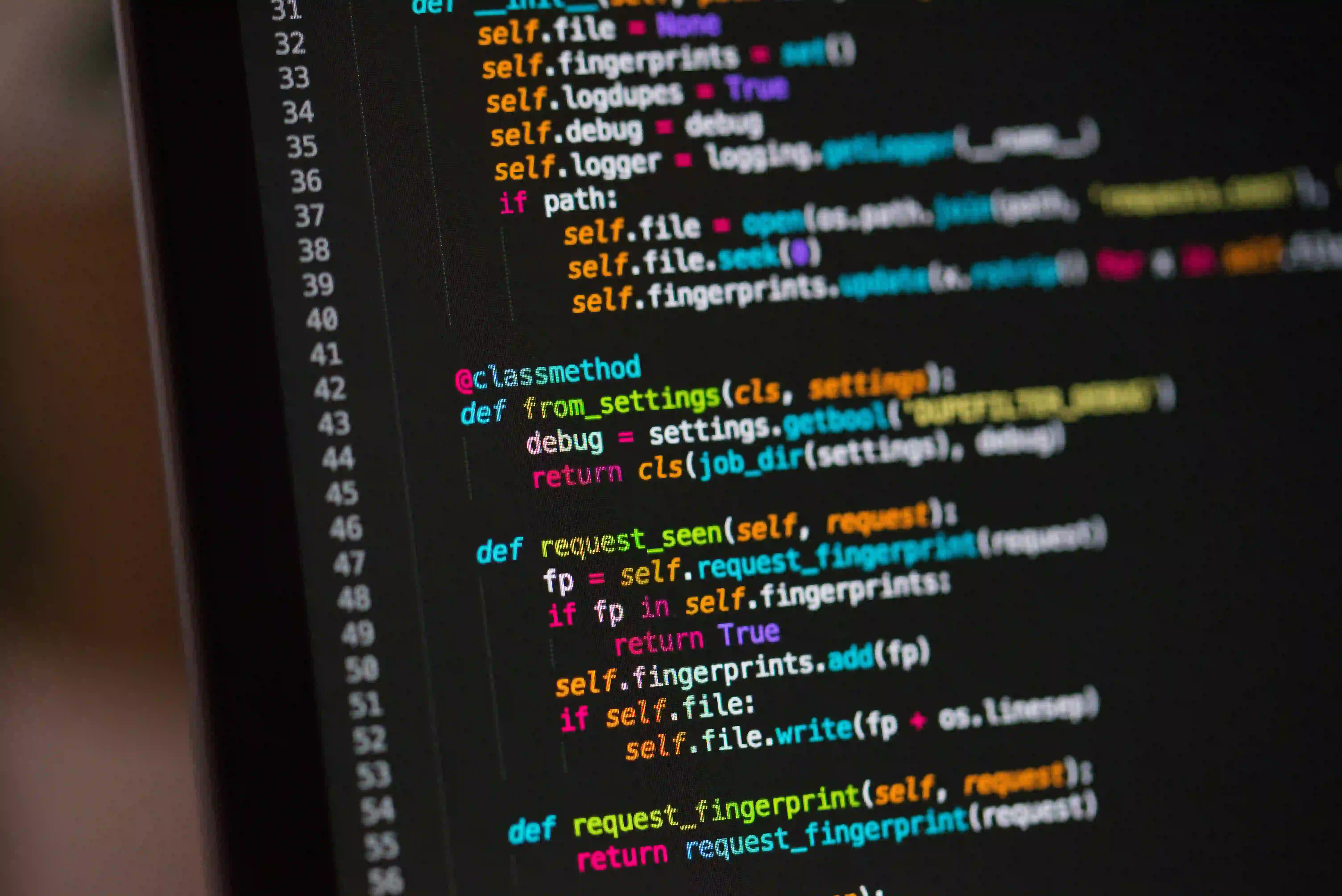
Avoid Common Pitfalls in Java With These String Tricks
Java, a cornerstone in the vast empire of software engineering, is celebrated for its robustness, security, and simplicity. However, mastering Java involves navigating around its intricacies and avoiding common pitfalls, especially when dealing with Strings. This blog post delves into indispensable String tricks that can significantly boost your productivity and safeguard your applications from common bugs. Whether you're a Java novice or a seasoned developer, these insights will enhance your coding toolkit.
Understanding Strings in Java
A String
in Java represents a sequence of characters. Unlike primitive data types, Strings are objects in Java, providing a rich array of methods for manipulating character sequences. However, this object nature of String in Java introduces certain subtleties that, if overlooked, can lead to perplexing bugs and performance hitches.
Immutability of Strings
One of the most fundamental aspects to grasp about Java Strings is their immutability. Once a String object is created, its content cannot be altered. This might sound restrictive, but it is actually a blessing in disguise, promoting security and thread-safety in your applications.
Consider the following code snippet:
String greeting = "Hello, ";
greeting += "World!";
It appears as if we're modifying greeting
, but in reality, a new String object is created and assigned to greeting
. The original "Hello, "
, orphaned, is left for garbage collection.
Why Care About Immutability?
Immutability has profound implications, especially in terms of performance. Continuous modification of String objects, especially within loops, can lead to a significant performance drag due to the constant creation and destruction of objects.
The StringBuilder Class
Java provides StringBuilder
as a solution for scenarios requiring mutable sequences of characters. StringBuilder
is designed for such use cases, offering an efficient way to concatenate or manipulate strings.
Here’s a quick example:
StringBuilder builder = new StringBuilder();
builder.append("Hello, ");
builder.append("World!");
String result = builder.toString();
StringBuilder
maintains a mutable sequence of characters, making it highly efficient for concatenating strings, especially within loops.
The Pitfall of String Literal Comparison
A common mistake among Java developers is using ==
to compare strings. This operator compares object references, not the content of the strings. For content comparison, always use .equals()
.
Here’s an illustration:
String a = "Hello";
String b = new String("Hello");
System.out.println(a == b); // false
System.out.println(a.equals(b)); // true
Though a
and b
contain identical sequences of characters, ==
yields false
because they reference different objects in memory. .equals()
, however, correctly identifies their content as identical.
Dealing with String Encodings
Encoding issues can cause baffling problems in your applications. Java Strings are internally represented as UTF-16. However, when interfacing with systems or files that use different encodings, it's essential to explicitly manage encodings.
Here’s how you can specify the encoding when reading from a file:
Path path = Paths.get("example.txt");
String content = Files.readString(path, StandardCharsets.UTF_8);
Explicitly specifying UTF_8
as the encoding ensures that the file's content is interpreted correctly. For more on handling files and character encodings in Java, the official Files documentation is an invaluable resource.
Regular Expressions and String.split()
Another powerful feature of Java Strings is their support for regular expressions, providing a potent tool for string manipulation. A common use case is using the .split()
method to divide a string into an array based on a delimiter.
However, .split()
sometimes works in unexpected ways, particularly with special characters. For instance, consider splitting a string with "."
as a delimiter:
String data = "one.two.three";
String[] parts = data.split(".");
Intuitively, you might expect parts
to contain {"one", "two", "three"}
, but it'll actually be an empty array. The reason is that .
is a special character in regular expressions, representing any character. To achieve the intended outcome, escape .
using \\.
:
String[] parts = data.split("\\.");
System.out.println(Arrays.toString(parts)); // [one, two, three]
Regular expressions in Java are a broad topic, deserving a detailed exploration on their own. The official Pattern documentation offers comprehensive insights into regular expressions in Java.
Closing Remarks
Strings are a fundamental part of Java programming, and understanding their nuances is crucial for effective coding. By mastering the nuances of Java Strings—embracing immutability, using StringBuilder
for concatenation, understanding the importance of .equals()
for content comparison, managing encodings appropriately, and leveraging regular expressions—you equip yourself to avoid common pitfalls that can sidetrack your development workflow.
In addition to the practices and tricks outlined above, always keep exploring and experimenting. Java, with its vast ecosystem and community, offers an abundance of resources to bolster your coding journey. Engage with code examples, participate in forums, and peruse through the extensive documentation available to you. Remember, mastery comes with practice and persistence.
Your journey with Java is bound to be exciting and enriching. Happy coding!