Boost Your App: Why API Performance Monitoring is Key
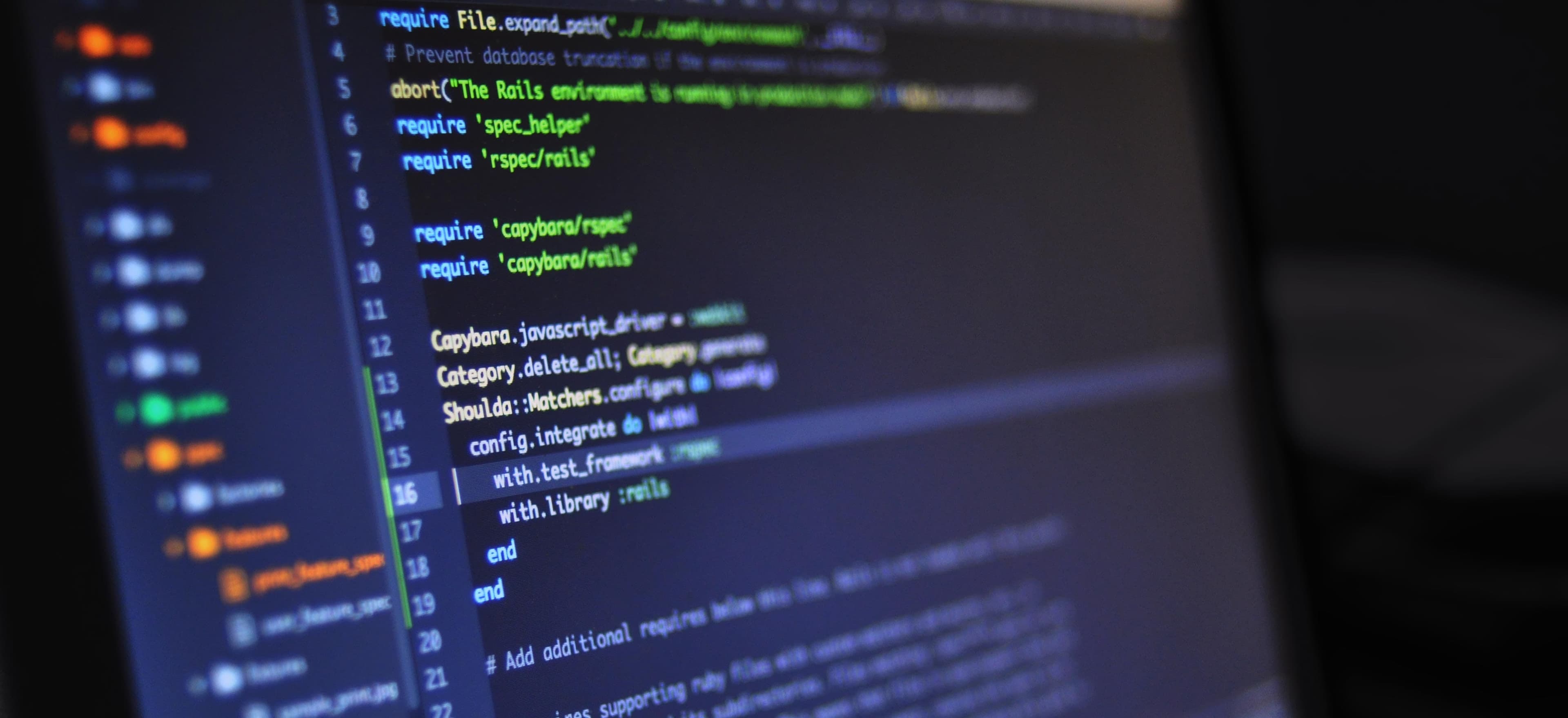
- Published on
Boost Your App: Why API Performance Monitoring is Key
In today's digital age, where user experience is paramount, the performance of your application's API can make or break its success. As a Java developer, you know that APIs play a crucial role in the functionality of modern applications. However, simply creating an API is not enough. Monitoring its performance is equally important to ensure that it meets the demands of your users and delivers a seamless experience. This is where API performance monitoring comes into play.
The Importance of API Performance Monitoring
API performance monitoring involves tracking, measuring, and analyzing the performance of your API to identify potential issues and optimize its speed and reliability. It provides insights into how your API is performing in real-time and helps you understand its impact on the overall user experience.
Ensuring Reliability
API performance monitoring allows you to detect and address any performance bottlenecks or latency issues that may arise. By proactively monitoring your API's performance, you can ensure its reliability and availability, ultimately preventing downtime and minimizing disruptions for your users.
Improving User Experience
A slow or unreliable API can significantly impact the user experience, leading to frustration and a negative perception of your application. By monitoring your API's performance, you can identify areas for improvement and optimize its speed and responsiveness, ultimately enhancing the overall user experience.
Meeting SLAs and Expectations
For many applications, meeting service level agreements (SLAs) is crucial. API performance monitoring enables you to track key performance indicators (KPIs) and ensure that your API meets the defined criteria. By consistently monitoring performance metrics, you can align your API's performance with user expectations and SLAs.
Implementing API Performance Monitoring in Java
Now that we understand the importance of API performance monitoring, let's explore how you can implement it in your Java application. There are several tools and frameworks available to facilitate API performance monitoring, but we'll focus on using Spring Boot Actuator for its simplicity and comprehensive functionality.
Spring Boot Actuator
Spring Boot Actuator provides a set of production-ready features to monitor and manage your application. It includes built-in endpoints that expose useful information about your application's health, metrics, and more. By leveraging Spring Boot Actuator, you can easily add API performance monitoring capabilities to your Java application.
Integration with Micrometer
Micrometer is a metrics facade that supports numerous monitoring systems, making it an excellent choice for integrating with Spring Boot Actuator. By incorporating Micrometer into your application, you can gather important metrics related to your API's performance and expose them through Spring Boot Actuator endpoints.
Example: Configuring Micrometer for API Performance Monitoring
Let's take a look at an example of how you can configure Micrometer to monitor the performance of your API endpoints in a Spring Boot application.
import io.micrometer.core.instrument.MeterRegistry;
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
import org.springframework.boot.actuate.autoconfigure.metrics.MeterRegistryCustomizer;
@Configuration
public class MicrometerConfig {
@Bean
public MeterRegistryCustomizer<MeterRegistry> commonTags() {
return registry -> registry.config().commonTags("application", "my-api");
}
}
In this example, we're creating a configuration class to customize the MeterRegistry by adding common tags. These tags provide contextual information about the application, making it easier to categorize and analyze the collected metrics.
import io.micrometer.core.annotation.Timed;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.RestController;
@RestController
public class ApiController {
@Timed(value = "api.endpoint", description = "Time taken to serve API endpoint")
@GetMapping("/api/endpoint")
public String getApiEndpoint() {
// Your API endpoint logic here
return "Success";
}
}
In this snippet, we have a simple API controller with a GET endpoint. By annotating the endpoint method with @Timed
, we instruct Micrometer to record the time taken to serve the API endpoint. This metric will be exposed through Spring Boot Actuator, allowing us to monitor the performance of this specific API endpoint.
By configuring Micrometer and leveraging annotations like @Timed
, you can easily instrument your API endpoints to collect important performance metrics. These metrics can then be visualized and analyzed through Spring Boot Actuator to gain insights into your API's performance.
Benefits of API Performance Monitoring in Java
Now that you have a clear understanding of how to implement API performance monitoring in your Java application, let's highlight the key benefits that come with it.
Proactive Issue Identification
API performance monitoring empowers you to proactively identify and address performance issues before they escalate. By continuously monitoring your API's performance metrics, you can detect anomalies and take corrective actions to ensure optimal performance.
Data-Driven Optimization
Monitoring your API's performance generates valuable data that can be used to drive optimization efforts. By analyzing performance metrics, you can make informed decisions to optimize critical parts of your API, leading to improved efficiency and responsiveness.
Enhanced Scalability
Understanding how your API performs under different loads is essential for ensuring its scalability. API performance monitoring provides insights into how your API behaves during peak usage, allowing you to make necessary adjustments to accommodate increased demand.
Improved Decision Making
By having real-time visibility into your API's performance, you can make data-driven decisions regarding infrastructure, architecture, and resource allocation. This allows you to align your API's performance with business objectives and user expectations.
Wrapping Up
In conclusion, API performance monitoring is a critical aspect of ensuring the reliability, speed, and responsiveness of your Java application's API. By implementing robust monitoring capabilities and leveraging tools like Spring Boot Actuator and Micrometer, you can gain valuable insights into your API's performance and take proactive measures to optimize it. With a well-monitored API, you can enhance the overall user experience, meet SLAs, and drive the success of your application in the competitive digital landscape.
Embracing API performance monitoring in your Java applications is not just a best practice; it's a strategic move to deliver exceptional user experiences and stay ahead of the curve in today's fast-paced digital world.
So, are you ready to supercharge your app with effective API performance monitoring? Let your Java application shine with optimized API performance!
Checkout our other articles