Boost Java App Performance: 5 Docker Monitoring Tips
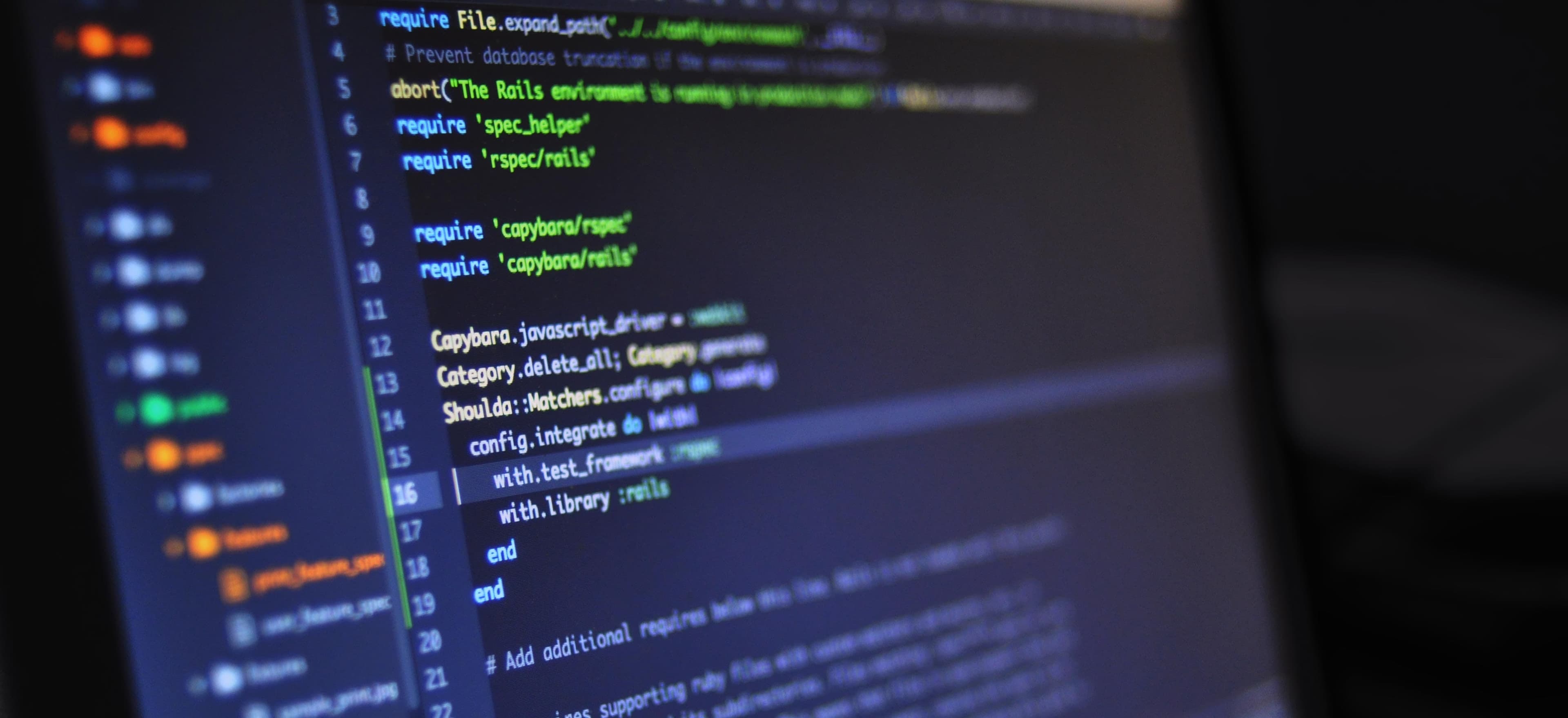
- Published on
Boost Java App Performance: 5 Docker Monitoring Tips for Exceptional Efficiency
As Java continues to cement its status as a cornerstone for a plethora of applications, from web apps to microservices, the technology stack used to deploy and manage Java applications has evolved significantly. Docker, with its promise of simplifying deployment processes and improving scalability and consistency across environments, has become a go-to choice for developers. However, as with any technology, the benefits come with their own set of challenges – monitoring and performance optimization being key among them.
In this blog post, we'll dive deep into five Docker monitoring tips that can significantly boost your Java application's performance. From leveraging native Docker commands to employing specialized monitoring tools, we'll explore strategies that will help you gain insights into your containers' behavior and ensure they are running optimally.
1. Leverage Docker Stats for Real-time Performance Insights
Before diving into complex monitoring solutions, it's worth exploring the tools Docker provides out-of-the-box. The docker stats
command is a powerful ally, offering real-time data on container resource usage statistics, including CPU, memory, network I/O, and disk I/O.
docker stats --all
This simple command can be your first line of defense in identifying performance bottlenecks. By keeping an eye on resource consumption, you can quickly pinpoint which containers are overutilizing resources and may need optimization or scaling.
2. Implement Custom Java Application Metrics
While Docker stats provide a high-level overview of container health, diving deeper into your Java application's performance is essential. Implementing custom metrics within your Java code is a powerful strategy to get detailed insights.
Java Management Extensions (JMX) is a Java technology that provides tools for monitoring and managing applications, system objects, devices, and service-oriented networks. By exposing key performance metrics through JMX, you can monitor detailed application performance aspects, such as JVM heap usage, thread counts, and custom application metrics.
@Bean
public MBeanExporter exporter(ObjectNameNamingStrategy namingStrategy, MBeanServer mBeanServer) {
MBeanExporter exporter = new MBeanExporter();
exporter.setAutodetect(true);
exporter.setNamingStrategy(namingStrategy);
exporter.setServer(mBeanServer);
return exporter;
}
This snippet demonstrates how to configure an MBeanExporter
in a Spring Boot application, enabling JMX metrics exposure. Custom metrics can help you identify inefficiencies at the code level, providing valuable insights for optimization.
3. Utilize Advanced Monitoring Tools
While Docker’s native tools and custom metrics offer valuable data, sometimes you need more advanced insights. This is where third-party monitoring tools like Prometheus and Grafana come into play. These tools can provide detailed analytics and visualization capabilities, taking your monitoring to the next level.
By configuring Prometheus to scrape your application metrics and visualizing them in Grafana, you can gain a comprehensive overview of your Java application's health and performance. Here's a quick guide on setting up Prometheus and Grafana for Docker monitoring.
Incorporating these tools into your monitoring strategy allows for setting up alerts based on specific thresholds, ensuring you're immediately aware of any potential issues before they impact users.
4. Monitor and Optimize the Java Virtual Machine (JVM)
The JVM is the heart of any Java application, and its performance is crucial. Monitoring JVM metrics such as heap size, garbage collection (GC) frequency, and thread counts can reveal potential bottlenecks.
One effective tool for JVM monitoring is VisualVM, a visual interface for viewing detailed information about Java applications running on the JVM. By attaching VisualVM to your Dockerized Java application, you can obtain in-depth insights into the JVM performance, helping you make informed optimization decisions.
docker exec -it <container_id> jcmd
This command lists the Java processes within your Docker container, which you can then monitor using VisualVM or similar tools. Understanding JVM behavior is essential for optimizing memory management and garbage collection processes, leading to improved performance.
5. Network Performance Considerations
Finally, don't overlook the impact of network performance on your Java application. Docker containers communicate over a virtual network, and issues like high latency or limited bandwidth can significantly affect application performance.
Monitoring tools like cAdvisor can provide insights into network I/O metrics, helping identify any network-related bottlenecks. Additionally, optimizing Docker networking configurations, such as choosing the right network driver or optimizing DNS settings, can lead to better performance.
Advanced tip: Consider implementing network policies to control the flow of traffic between your containers, ensuring efficient communication paths and reducing unnecessary latency.
By implementing these five Docker monitoring tips, you can significantly enhance the performance of your Java applications. Regularly monitoring resource usage, implementing custom application metrics, using advanced visualization tools, optimizing JVM settings, and paying attention to network performance can lead to substantial improvements.
Remember, the key to effective monitoring and optimization is continuous iteration. By consistently analyzing performance data and making informed adjustments, you can ensure your Java applications run smoothly and efficiently, offering a better experience for your users.
For further reading on optimizing Java applications, check out the official Java SE Performance Documentation. Happy monitoring!