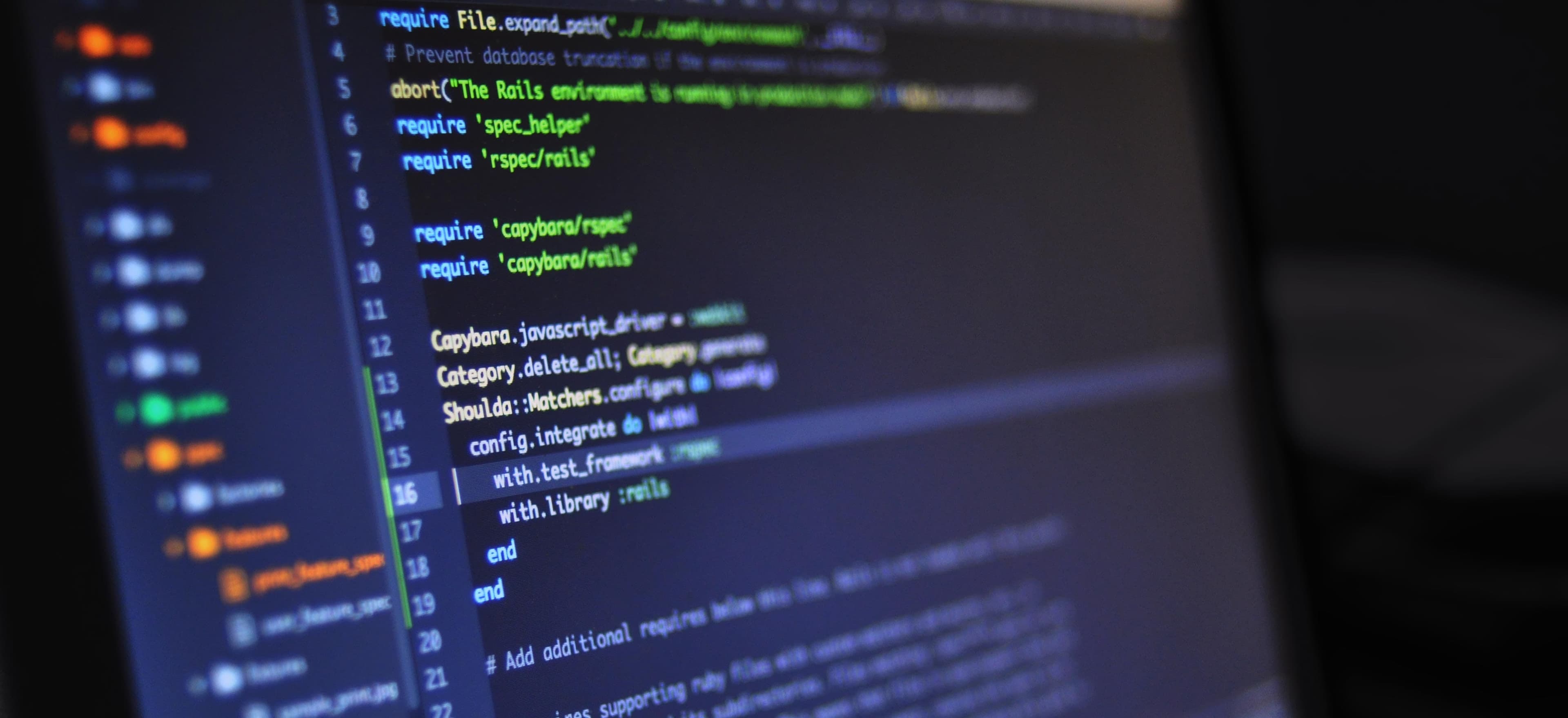
- Published on
Streamlining Data: Mastering Spring Integration APIs
In the realm of Java development, the Spring framework continues to be a bastion of versatility and efficiency. One of its most powerful facets is Spring Integration, which provides a seamless means of integrating disparate systems. Through this article, we will delve into the integral concepts and practices of Spring Integration, elucidating how it strengthens the processing and manipulation of data.
Understanding the Essence of Spring Integration
At its core, Spring Integration is centered around the concept of enterprise integration patterns (EIP) – a set of design patterns used to facilitate the integration of various systems within an enterprise. By leveraging these patterns, Spring Integration fosters a cohesive and modular approach to system integration, bolstering the development of robust and efficient solutions.
The Commanding Role of Message Endpoints
In the context of Spring Integration, message endpoints play a pivotal role. These endpoints serve as the gateways for messages to enter and exit the integration flow. By encapsulating the messaging logic within these endpoints, the system’s modularity and resilience are enhanced, facilitating efficient message processing.
@ServiceActivator(inputChannel = "inputChannel", outputChannel = "outputChannel")
public String handleMessage(String input) {
// Message handling logic
return processedMessage;
}
In the depicted code snippet, the @ServiceActivator
annotation designates the method as a message endpoint. The inputChannel
and outputChannel
attributes delineate the input and output channels for message transmission. This segregation of channels augments the clarity and maintainability of the integration flow.
Embracing Messaging Channels
Messaging channels form the conduits through which messages traverse within the integration flow. As the foundation of communication within Spring Integration, these channels afford various implementations, such as direct, pub-sub, and point-to-point channels. Their versatility and adaptability render them conducive to diverse integration scenarios.
@Bean
public MessageChannel inputChannel() {
return new DirectChannel();
}
In the provided code snippet, a DirectChannel
is instantiated to serve as the inputChannel
. This instantiation exemplifies the simplicity and flexibility of configuring messaging channels within Spring Integration.
Orchestrating Message Routing with Message Routers
Message routers ascertain the appropriate routing path for incoming messages based on predetermined criteria. By employing message routers, the integration flow’s robustness and adaptability are amplified, as messages are dynamically directed to designated endpoints.
@Router(inputChannel = "inputChannel")
public String routeMessage(String input) {
// Routing logic to determine the output channel
return outputChannel;
}
The annotated method above embodies a message router, denoted by the @Router
annotation. Within this method, the logic to determine the output channel based on the incoming message is encapsulated, exemplifying the dynamic routing capabilities imbued within Spring Integration.
Adhering to Messaging Adapters
In the world of integration, heterogeneity in system interfaces is ubiquitous. Messaging adapters serve as the linchpins, harmonizing the disparity by interfacing with specific external systems. Spring Integration nurtures this adaptability through a myriad of predefined adapters and also extends the provision to create custom adapters, tailoring to the diverse integration requisites.
@Bean
@ServiceActivator(inputChannel = "inputChannel")
public MessageHandler outboundAdapter() {
return new JmsSendingMessageHandler(jmsTemplate);
}
In the above configuration snippet, a JMS outbound adapter is declared as a @ServiceActivator
. This adapter encapsulates the logic for sending messages to a Java Message Service (JMS) destination, encapsulating the intricacies of JMS interaction within an adaptable and seamless facade.
Empowering Transformation with Message Transformers
Oftentimes, the disparity between incoming and outgoing messages warrants transformation. This is where message transformers come into play, effectuating the requisite message conversions and encodings to ensure compatibility and coherence within the integration flow.
@Transformer(inputChannel = "inputChannel", outputChannel = "outputChannel")
public String transformMessage(String input) {
// Transformation logic
return transformedMessage;
}
The annotated method above embodies a message transformer, designated by the @Transformer
annotation. Here, the incoming message undergoes the stipulated transformation logic before being routed to the output channel, illustrating the pivotal role of message transformers in ensuring seamless data transformation within Spring Integration.
Wrapping Up
In essence, Spring Integration stands as a formidable ally for streamlining data integration within Java applications. Its adept handling of enterprise integration patterns, coupled with its seamless implementation of messaging endpoints, channels, routers, adapters, and transformers, fortifies the development of resilient, modular, and efficient integration solutions.
By embracing the tenets and utilities of Spring Integration, Java developers can elevate the efficacy and cohesion of their systems, fortifying the data integration capabilities and propelling their applications towards greater resilience and adaptability.
Inculcating a deep understanding of Spring Integration not only enhances the mastery of enterprise integration patterns but also augments the adeptness in navigating the intricacies of system integration, thus empowering developers to architect robust and efficient solutions to meet the burgeoning demands of the modern enterprise landscape.
In conclusion, with Spring Integration at their disposal, Java developers are empowered to navigate the capricious seas of system integration with finesse, fortitude, and unparalleled efficiency.
As always, the documentation and official resources for Spring Integration present an invaluable wellspring of knowledge and insights for delving deeper into the intricacies and applications of Spring Integration within Java development. Additionally, the illustrious book "Spring Integration in Action" by Mark Fisher, Jonas Partner, Marius Bogoevici, and Iwein Fuld serves as an invaluable compendium for an in-depth exploration of Spring Integration and its multifaceted capabilities.
Checkout our other articles