Cracking the Code: Custom I18n Solutions Unveiled
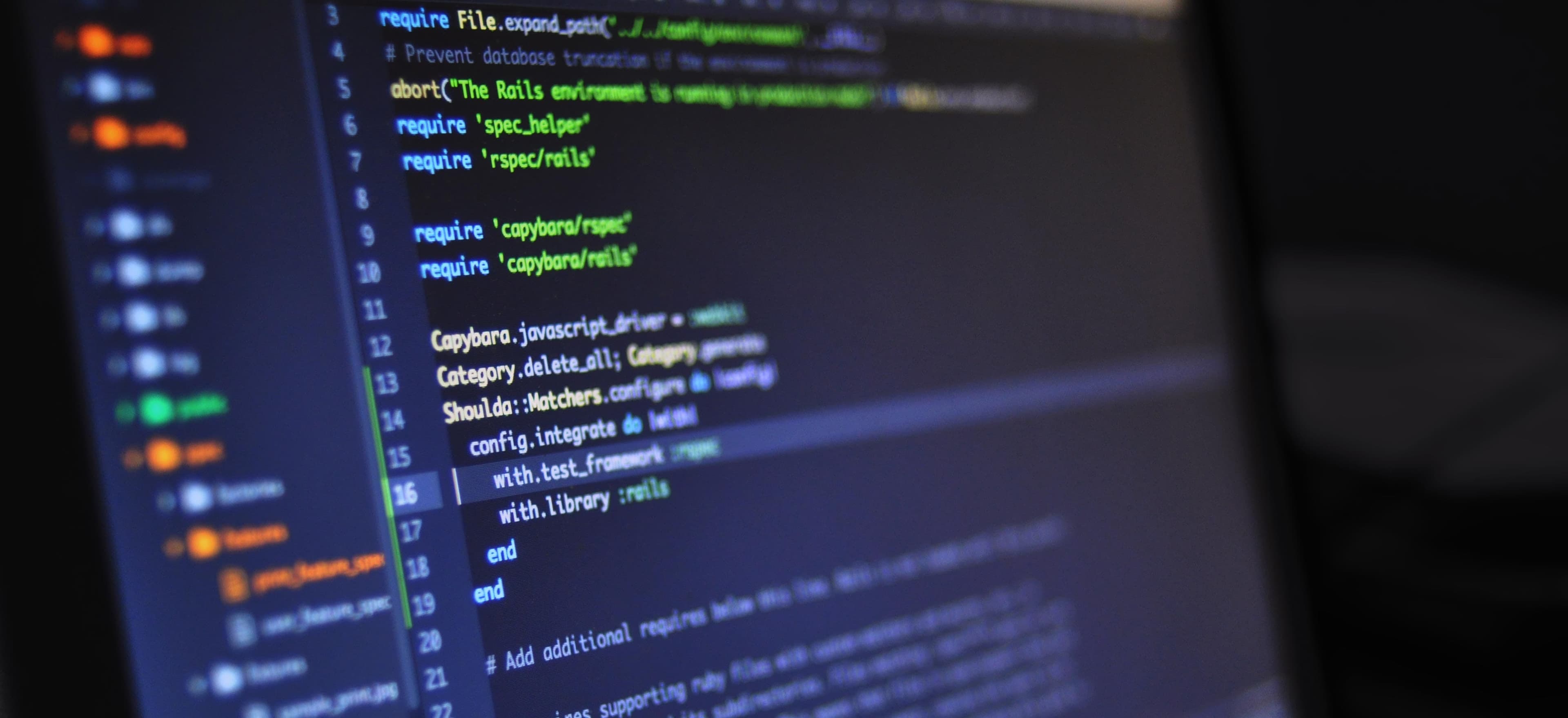
- Published on
Cracking the Code: Custom I18n Solutions Unveiled
Internationalization and localization (I18n) are crucial aspects of software development that often require customized solutions, especially in Java applications. In this blog post, we will delve into the world of custom I18n solutions, exploring the challenges, best practices, and how Java developers can crack the code to build robust and flexible I18n capabilities.
The Importance of Custom I18n Solutions
In a globalized world, catering to diverse languages, regions, and cultures is not just a good practice but a necessity for reaching a wider audience. While standard I18n libraries and frameworks provide a solid foundation, custom solutions are often required to meet specific project requirements, such as dynamic language switching, integration with third-party translation services, or unique formatting rules.
Challenges of Standard I18n Solutions
Standard I18n solutions in Java, such as Java’s ResourceBundle and MessageFormat, offer a solid foundation for handling language-specific resources and formatting. However, they may fall short when it comes to more complex requirements, such as dynamic content, pluralization, or the need to integrate with external translation services and APIs.
Building Custom I18n Solutions in Java
Dynamic Language Switching
In many applications, the ability to dynamically switch languages without requiring a restart is a key requirement. By leveraging a custom I18n solution, developers can design language switching mechanisms that seamlessly update the UI and content based on user preferences, enhancing the user experience.
// Example code for dynamic language switching
public void switchLanguage(String newLanguage) {
Locale newLocale = new Locale(newLanguage);
ResourceBundle newBundle = ResourceBundle.getBundle("messages", newLocale);
// Update UI with newBundle content
}
In the above code, switchLanguage
demonstrates how a custom method can be used to switch the language dynamically by updating the ResourceBundle based on the user's language preference, improving the interactivity and personalization of the application.
Integration with Third-Party Translation Services
Integrating with third-party translation services, such as Google Translate or Microsoft Translator, can enhance the scalability and quality of translations. Custom I18n solutions can accommodate seamless integration with such services, allowing for on-the-fly translation of content within the application.
// Example code for integrating with Google Translate API
public String translateWithGoogle(String content, String targetLanguage) {
// Integration code to call Google Translate API with content and targetLanguage
// Return translated content
}
By incorporating custom methods like translateWithGoogle
, Java developers can seamlessly integrate with third-party translation services to provide real-time, high-quality translations, enriching the multilingual capabilities of the application.
Flexible Formatting Rules
Custom I18n solutions enable developers to define and implement flexible formatting rules specific to their application's requirements. Whether it involves custom date/time formatting, currency display, or numeral formatting, a tailored approach allows for precise control over how content is presented in different locales.
// Example code for custom date formatting
public String formatDate(Date date, Locale locale) {
SimpleDateFormat dateFormat = new SimpleDateFormat("dd MMMM yyyy", locale);
return dateFormat.format(date);
}
In the above code snippet, formatDate
showcases how custom date formatting can be achieved by using a SimpleDateFormat with a locale, ensuring that the date format adheres to the conventions of the specified locale.
Best Practices for Custom I18n Solutions
When developing custom I18n solutions in Java, it’s essential to adhere to best practices to ensure maintainability, scalability, and consistency across the application.
Use Property Files for Resource Bundles
Organize localized resources in property files, following a clear naming convention and structure. This promotes maintainability and allows for easy addition of new languages without impacting the codebase.
Externalize Dynamic Content
Separate dynamic content, such as user-generated text or database-driven content, from static localized resources. This facilitates easier management and updates of dynamic content without impacting the localization process.
Implement Caching for Translations
To optimize performance, consider implementing caching mechanisms for translated content to reduce the overhead of frequent resource loading and parsing. This can significantly improve the responsiveness of multilingual applications.
Final Thoughts
Custom I18n solutions in Java empower developers to address the complexities and nuances of internationalization and localization, enabling applications to cater to diverse audiences with precision and flexibility. By embracing the power of custom solutions, Java developers can crack the code to unlock a world of multilingual possibilities, delivering exceptional user experiences across the globe.
In conclusion, the journey to mastering custom I18n solutions in Java involves embracing the challenges, leveraging the language's versatility, and adhering to best practices to create robust and scalable internationalized applications.
To explore further about advanced internationalization techniques in Java, consider checking out the Java Internationalization guide and the official Java Internationalization documentation by Oracle.