Mastering Java 8: Unraveling New BigInteger Methods
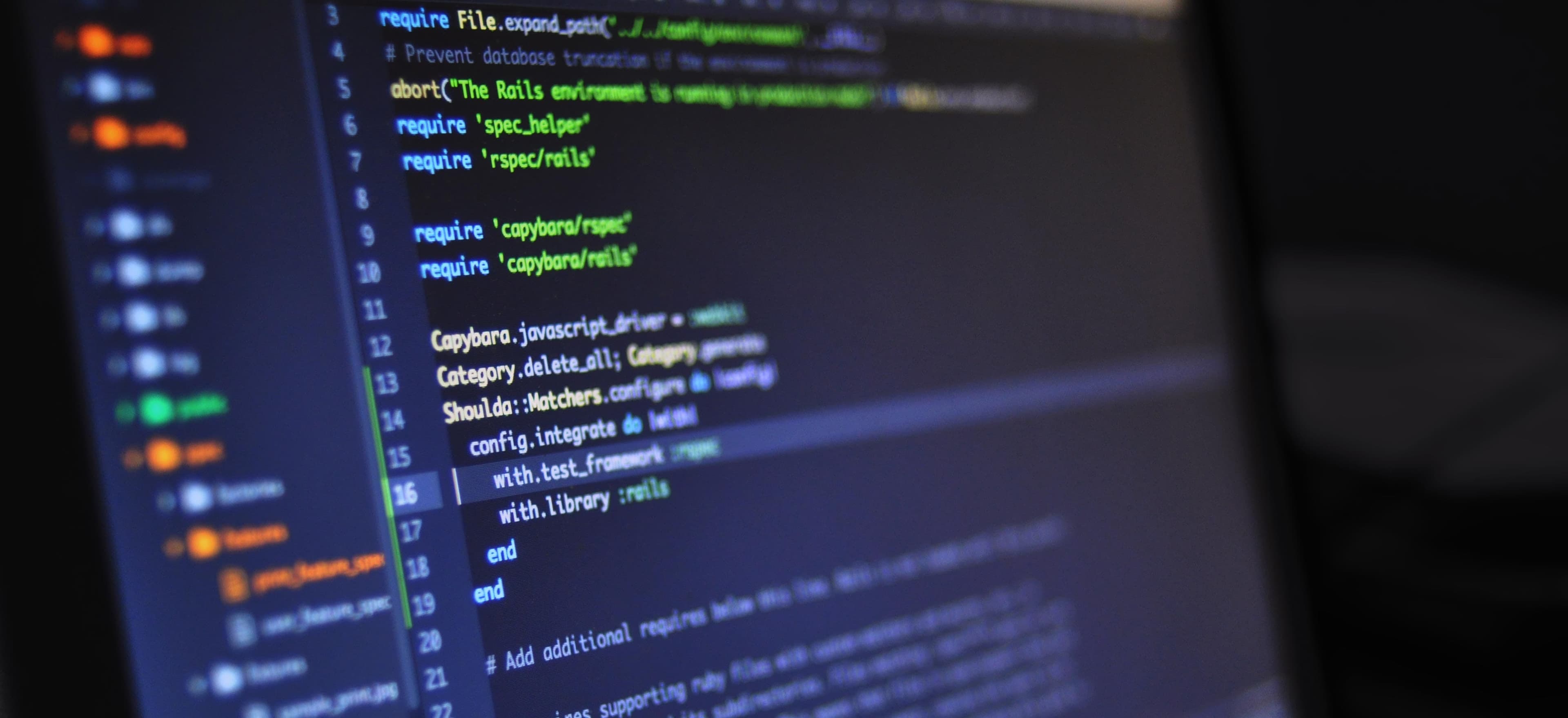
- Published on
Mastering Java 8: Unraveling New BigInteger Methods
Java 8 brought an array of improvements that span across the Java ecosystem, from lambda expressions that promise more concise code to the introduction of new classes and methods that enhance functionality and provide developers with more tools in their arsenal. Among these updates, some of the more subtle yet profoundly impactful enhancements were made to the BigInteger
class. In this post, we will uncover the new BigInteger
methods introduced in Java 8, explain their significance, and showcase how to use them effectively with examples. Whether you're dealing with large numbers in financial calculations, cryptography, or any domain where precision matters, understanding these new methods is essential.
Why BigInteger
?
Before diving into the specifics, let's remind ourselves why BigInteger
is a crucial part of the Java language. Unlike standard numeric types (int
, long
, etc.) that have a fixed size and thus a limit to the numbers they can represent, BigInteger
allows for arithmetic operations on integers of virtually unlimited size. This capability is indispensable in certain fields of computing.
New Additions in Java 8
Java 8 enriched the BigInteger
class with methods that enhance its functionality, making certain operations more convenient and efficient. Here are some of the standout additions:
static BigInteger probablePrime(int bitLength, Random rnd)
Generating prime numbers is a fundamental operation in cryptography. Prior to Java 8, developers had to rely on less efficient or more convoluted methods. The probablePrime
method simplifies this by directly generating a prime number of the desired bit length.
import java.math.BigInteger;
import java.util.Random;
public class PrimeGenerator {
public static void main(String[] args) {
BigInteger prime = BigInteger.probablePrime(512, new Random());
System.out.println("Generated prime: " + prime);
}
}
In this example, we generate a 512-bit prime number. This method is particularly efficient for cryptographic applications where the generation of large prime numbers is frequently required.
BigInteger nextProbablePrime()
Continuing with the theme of prime numbers, the nextProbablePrime
method finds the next prime number greater than the current BigInteger
instance. This is incredibly useful for algorithms that need to find a series of prime numbers in sequence.
import java.math.BigInteger;
public class PrimeSequencer {
public static void main(String[] args) {
BigInteger start = new BigInteger("10000");
for (int i = 0; i < 5; i++) {
start = start.nextProbablePrime();
System.out.println("Next prime: " + start);
}
}
}
This code snippet sequentially finds and prints five prime numbers starting from 10,000. Such functionality simplifies tasks in cryptographic key generation or any domain requiring sequential prime numbers.
byte[] getBytes()
Serialization and deserialization of BigInteger
instances become straightforward with the getBytes
method, which returns a byte array representation of the BigInteger
. This method is crucial for networking and storing numbers in a binary format.
import java.math.BigInteger;
public class SerializationExample {
public static void main(String[] args) {
BigInteger number = new BigInteger("123456789");
byte[] byteArray = number.getBytes();
// Use byteArray for networking or storage
BigInteger reconstructed = new BigInteger(byteArray);
System.out.println("Reconstructed Number: " + reconstructed);
}
}
In this example, we demonstrate converting a BigInteger
to a byte array and back, showcasing a simple serialization/deserialization process. This functionality is particularly useful when working with large numbers in distributed systems or when saving them to databases.
Practical Applications
The new BigInteger
methods in Java 8 open up a range of possibilities. In cryptography, the generation and handling of large prime numbers become more efficient, which directly impacts the performance and security of cryptographic algorithms. In finance, accurate and efficient handling of large numbers ensures precise calculations for transactions, interest, and more. Moreover, in scientific computing, where large integers often come into play, these methods enhance both the performance and convenience of numerical computations.
Further Reading
To deepen your understanding of BigInteger
and its applications, the official Java documentation is an invaluable resource. Additionally, for those interested in cryptographic applications, exploring the RSA algorithm provides practical insights into how BigInteger
can be utilized in real-world security.
Closing Remarks
Java 8's new BigInteger
methods significantly enhance the power and convenience of working with large integers. By understanding and applying these methods, developers can write more efficient, cleaner, and more effective code in domains where precision and scale of numbers matter the most. Whether it's generating prime numbers for cryptography, handling large numerical computations, or simply dealing with numbers beyond the scope of standard data types, the updated BigInteger
class is an essential tool in the developer's toolkit.
Embrace these additions and explore how they can benefit your specific use cases. Happy coding!