Boost Your Site Speed: Mastering Spring's Cache Manager
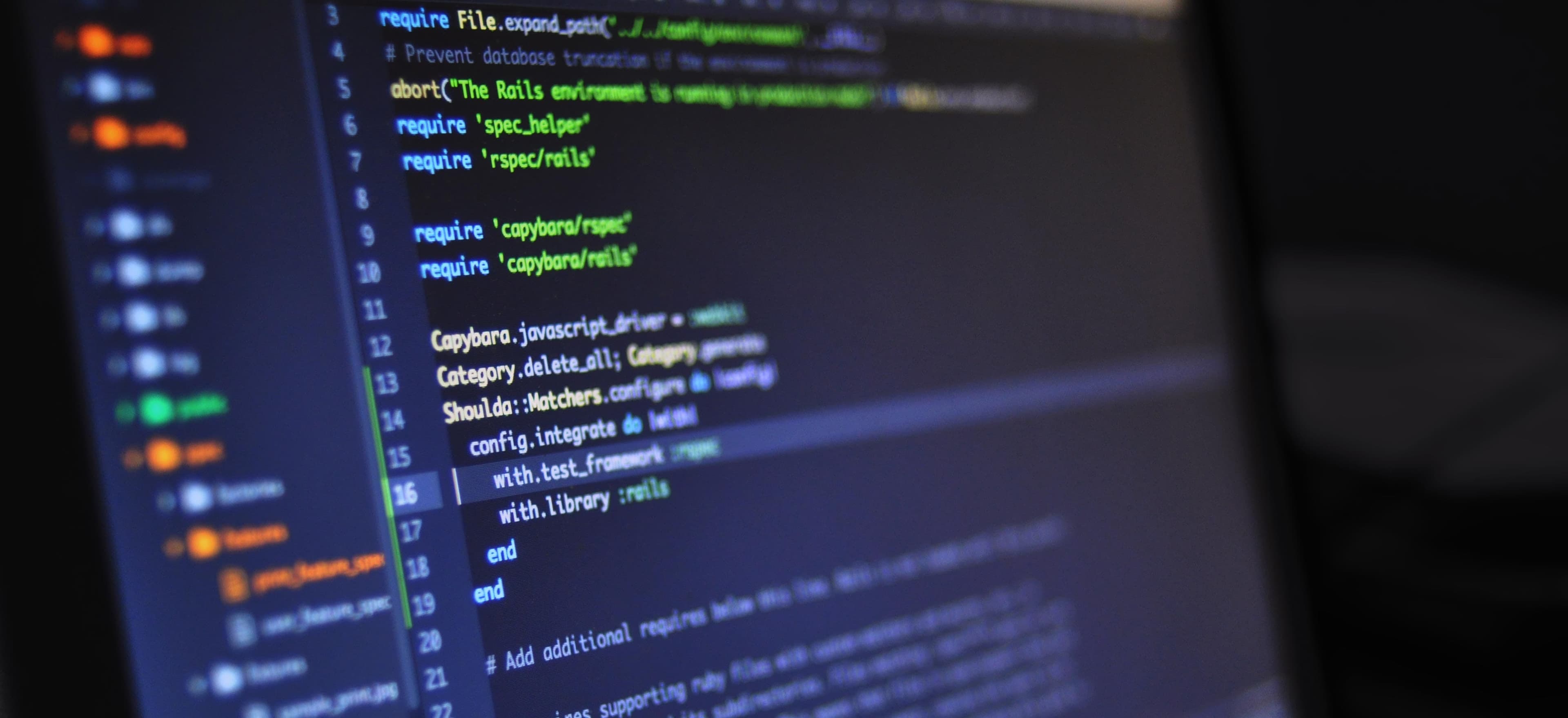
- Published on
Boost Your Site Speed: Mastering Spring's Cache Manager
When it comes to optimizing website performance, site speed is a critical factor. Slow-loading websites not only frustrate visitors but also affect search engine rankings. In the world of Java web development, Spring Framework provides a robust caching mechanism through its Cache Manager. In this article, we'll delve into mastering Spring's Cache Manager to turbocharge your site's speed.
Understanding Caching in Web Applications
Caching involves storing frequently accessed data in a temporary storage area. When a user requests the same data again, it can be served from the cache quickly, thus reducing the load on the server and improving response times.
In web applications, caching can be applied at various levels, including:
- Page Level Caching: Caching the entire HTML content of a page.
- Data Caching: Storing the results of expensive database queries or external API calls.
- Component Caching: Caching specific UI components or fragments.
Introducing Spring's Cache Abstraction
Spring Framework provides a powerful, flexible, and easy-to-use caching abstraction through its @Cacheable
, @CachePut
, and @CacheEvict
annotations. These annotations can be applied to methods, and Spring takes care of the caching implementation, allowing developers to focus on business logic.
By default, Spring uses a simple in-memory cache. However, it also supports integration with popular caching libraries such as Ehcache, Hazelcast, Redis, and Caffeine. This flexibility enables developers to switch between caching providers without changing the application code significantly, making it easy to adapt to changing performance and scalability requirements.
Configuring Spring's Cache Manager
To begin harnessing the power of Spring's caching capabilities, first, you'll need to configure a cache manager. Let's take a look at an example of configuring the Ehcache as the caching provider using Spring Boot.
Step 1: Add the Ehcache Dependency
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-cache</artifactId>
</dependency>
<dependency>
<groupId>net.sf.ehcache</groupId>
<artifactId>ehcache</artifactId>
</dependency>
Step 2: Enable Caching in the Application
@SpringBootApplication
@EnableCaching
public class MyApplication {
public static void main(String[] args) {
SpringApplication.run(MyApplication.class, args);
}
}
Step 3: Configure the Cache Manager
@Configuration
@EnableCaching
public class CacheConfig {
@Bean
public CacheManager cacheManager() {
return new EhCacheCacheManager(ehCacheCacheManager().getObject());
}
@Bean
public EhCacheManagerFactoryBean ehCacheCacheManager() {
EhCacheManagerFactoryBean cmfb = new EhCacheManagerFactoryBean();
cmfb.setConfigLocation(new ClassPathResource("ehcache.xml"));
cmfb.setShared(true);
return cmfb;
}
}
In this example, we've added the necessary dependencies, enabled caching in the Spring Boot application, and configured the EhCacheCacheManager
bean. We've also specified the location of the Ehcache configuration file (ehcache.xml
).
Using Spring's Cache Abstraction
Once the cache manager is configured, you can start leveraging Spring's caching capabilities using the @Cacheable
, @CachePut
, and @CacheEvict
annotations.
@Cacheable: Caching Method Results
The @Cacheable
annotation allows you to specify that the result of a method should be cached. Let's consider an example where we have a method to fetch user details by ID.
@Service
public class UserService {
@Cacheable(value = "users", key = "#userId")
public User getUserById(Long userId) {
// Database or external API call to fetch user details
return userRepository.findById(userId);
}
}
In this example, the getUserById
method's return value will be cached with the key as the user's ID (userId
). Subsequent calls to this method with the same user ID will be served from the cache, eliminating the need to perform the expensive data retrieval operation.
@CachePut: Updating the Cache
The @CachePut
annotation allows you to update the cache after a method is invoked, ensuring that the cache remains consistent with the underlying data.
@Service
public class UserService {
@CachePut(value = "users", key = "#user.id")
public User updateUser(User user) {
// Update user details in the database
return userRepository.save(user);
}
}
In this example, the updateUser
method updates the user details in the database and ensures that the updated user object is cached with the key as the user's ID.
@CacheEvict: Evicting Cache Entries
The @CacheEvict
annotation allows you to evict one or more entries from the cache. This can be useful when data is updated or deleted.
@Service
public class UserService {
@CacheEvict(value = "users", key = "#userId")
public void deleteUser(Long userId) {
// Delete user from the database
userRepository.deleteById(userId);
}
}
In this example, the deleteUser
method evicts the user entry from the cache when a user is deleted from the database.
Advanced Caching Strategies
While the basic caching annotations cover most use cases, Spring's Cache Manager offers more advanced features to cater to diverse caching requirements.
Conditional Caching with SpEL
Spring Expression Language (SpEL) can be used to define conditions for caching. This can be useful in scenarios where you want to cache data based on certain conditions.
@Service
public class ProductService {
@Cacheable(value = "products", condition = "#discount gt 0")
public List<Product> getDiscountedProducts(double discount) {
// Logic to fetch discounted products
return productRepository.findByDiscountGreaterThan(discount);
}
}
In this example, the getDiscountedProducts
method caches the results only if the discount
parameter is greater than 0, effectively excluding non-discounted products from being cached.
Cache Entry Expiration
Spring's Cache Manager allows you to define expiration policies for cache entries. This ensures that stale data is evicted from the cache, and fresh data is fetched when necessary.
@Configuration
@EnableCaching
public class CacheConfig {
@Bean
public CacheManager cacheManager() {
SimpleCacheManager cacheManager = new SimpleCacheManager();
cacheManager.setCaches(Arrays.asList(
new ConcurrentMapCache("users"),
new ConcurrentMapCache("products", CacheBuilder.newBuilder().expireAfterAccess(1, TimeUnit.HOURS).build())
));
return cacheManager;
}
}
In this example, we've configured the expiration policy for the products
cache, ensuring that the cache entries expire after 1 hour of access.
Cache Eviction at Intervals
Spring's Cache Manager also provides support for periodic cache eviction, allowing you to refresh the cache at regular intervals.
@Scheduled(fixedDelay = 60000) // Evict cache every minute
@CacheEvict(allEntries = true, value = {"products"})
public void evictAllProductsCache() {
System.out.println("Evicting all products from cache");
}
In this example, the evictAllProductsCache
method is annotated with @CacheEvict
and @Scheduled
, ensuring that the products
cache is evicted every minute, refreshing the cached data.
Lessons Learned
In this article, we've explored the power of Spring's Cache Manager and its ability to significantly improve site speed and overall performance. By effectively utilizing Spring's caching annotations and advanced caching strategies, you can optimize your Java web applications, reduce server load, and enhance the user experience. Mastering Spring's Cache Manager is indeed a valuable skill for any Java developer aiming to build high-performance web applications.
By mastering Spring's Cache Manager, you can give your website the speed boost it needs to stay ahead in the competitive digital landscape.
Start implementing caching in your Spring applications and witness the remarkable performance enhancements it brings!
Remember, when it comes to site speed, every millisecond counts.
Happy caching!