Cracking Twitter Mood: Navigating Spark Streaming Sentiment Woes
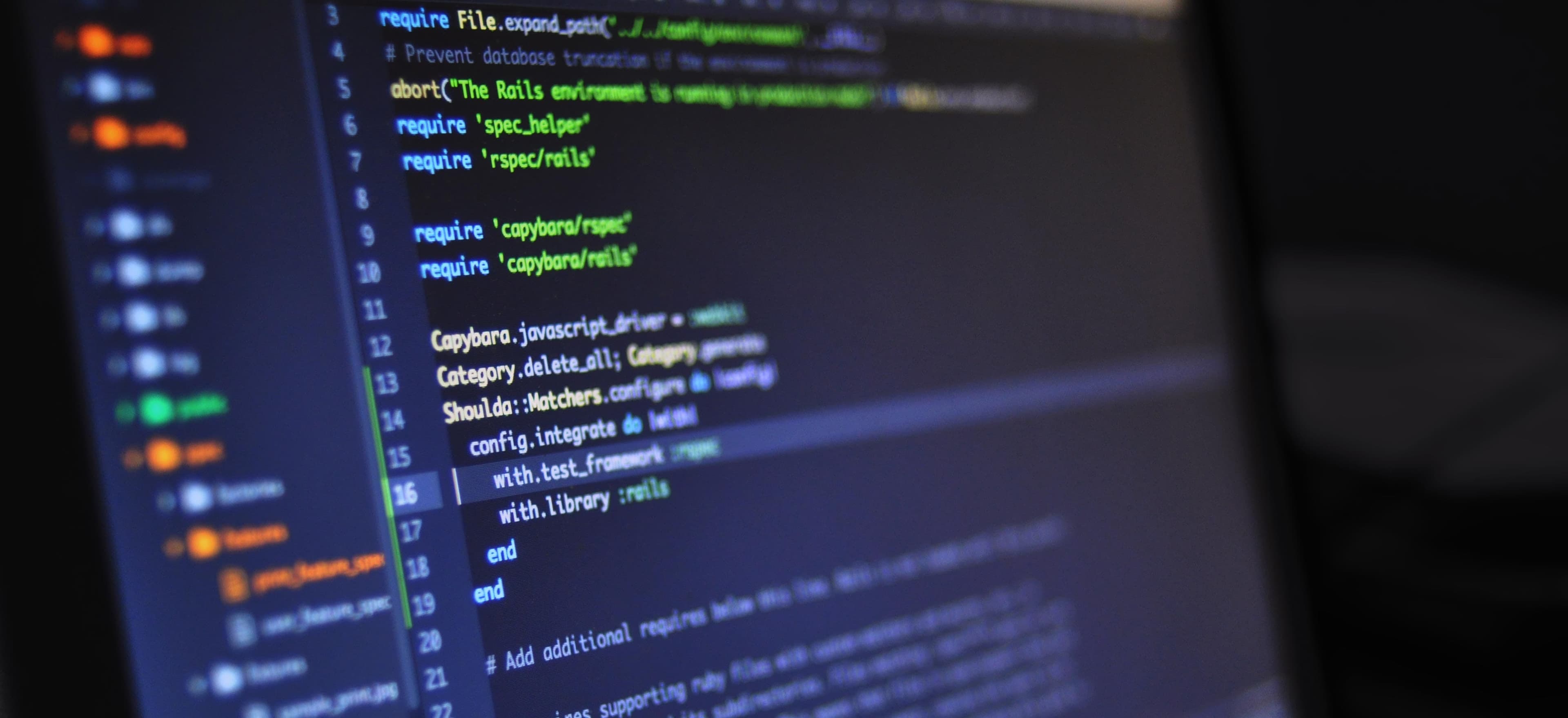
- Published on
An Introduction to Twitter Sentiment Analysis using Spark Streaming
In recent years, sentiment analysis has become a critical part of understanding and interpreting data on social media platforms such as Twitter. The ability to gauge public sentiment towards a particular topic, product, or event is invaluable for businesses, marketers, and researchers. In this blog post, we will explore how to use Spark Streaming to perform sentiment analysis on live Twitter data. We'll navigate the challenges and complexities involved in processing and analyzing continuous streams of tweets to derive meaningful sentiment insights.
Setting the Stage with Apache Spark
Apache Spark is a powerful and popular open-source distributed computing system that provides an easy-to-use and unified analytics engine for big data processing. Spark's streaming capabilities allow us to process and analyze data in real-time, making it an ideal choice for working with live Twitter streams. By leveraging Spark's structured streaming API, we can build robust and scalable systems for real-time sentiment analysis.
Fetching Live Twitter Data
Before we delve into sentiment analysis, the first step is to establish a connection to the Twitter API and fetch live tweet streams. Spark provides a seamless integration with Twitter's streaming API through the twitter4j
library, which simplifies the process of pulling live tweets into our Spark application.
import org.apache.spark.streaming.twitter.TwitterUtils;
import twitter4j.auth.Authorization;
import twitter4j.auth.AuthorizationFactory;
import twitter4j.conf.Configuration;
import twitter4j.conf.ConfigurationBuilder;
ConfigurationBuilder cb = new ConfigurationBuilder();
cb.setDebugEnabled(true)
.setOAuthConsumerKey("YOUR_CONSUMER_KEY")
.setOAuthConsumerSecret("YOUR_CONSUMER_SECRET")
.setOAuthAccessToken("YOUR_ACCESS_TOKEN")
.setOAuthAccessTokenSecret("YOUR_ACCESS_TOKEN_SECRET");
Configuration config = cb.build();
Authorization auth = AuthorizationFactory.getInstance(config);
JavaDStream<Status> twitterStream = TwitterUtils.createStream(ssc, auth);
In the code snippet above, we configure the Twitter API credentials and create a connection to the Twitter stream using Spark's TwitterUtils
class. This allows us to receive a continuous stream of tweets that we can then process for sentiment analysis.
Processing and Analyzing Tweets
Once we have the live tweet stream, the next step is to preprocess and analyze the tweets to extract sentiment information. We can use natural language processing (NLP) techniques and sentiment lexicons to infer the sentiment of each tweet.
JavaDStream<String> tweets = twitterStream.map(Status::getText);
JavaDStream<Tuple2<String, Integer>> tweetSentiments = tweets.map(tweet -> {
int sentimentScore = analyzeSentiment(tweet); // Custom sentiment analysis function
return new Tuple2<>(tweet, sentimentScore);
});
In the code above, we first extract the text content of each tweet from the stream. We then apply a custom analyzeSentiment
function to assign a sentiment score to each tweet. This function could utilize NLP libraries like Apache OpenNLP or Stanford CoreNLP to perform sentiment analysis based on the textual content of the tweets.
Handling Streaming Data Challenges
Working with streaming data poses unique challenges compared to batch processing. One of the key challenges is handling the continuous influx of data while maintaining low-latency processing and high throughput. Spark's micro-batch processing model addresses these challenges by discretizing the streaming data into small, manageable batches, allowing for efficient processing and fault tolerance.
tweetSentiments.foreachRDD(rdd -> {
// Process the sentiment scores in each batch
// Perform aggregations, filtering, and further analysis
});
In the code snippet above, we use the foreachRDD
function to apply operations on each RDD (Resilient Distributed Dataset) representing a micro-batch of tweet sentiments. This allows us to perform batch-level processing, such as aggregations and analysis, on the streaming data.
Visualizing Sentiment Trends
Visualizing the sentiment trends derived from the live Twitter data can provide valuable insights into public opinion and reaction to specific events or topics. Tools like Apache Zeppelin or Jupyter notebooks can be leveraged to create interactive visualizations that showcase the sentiment analysis results in real-time.
tweetSentiments.foreachRDD(rdd -> {
// Update a real-time dashboard or visualization
// Display sentiment trends, word clouds, or sentiment distributions
});
In the code snippet above, we can update a real-time dashboard or visualization with the latest sentiment trends derived from the tweet sentiments. This could include visualizations like word clouds, sentiment distributions, or time-series charts depicting sentiment trends over time.
Wrapping Up
In this blog post, we've explored the intricacies of performing sentiment analysis on live Twitter data using Apache Spark Streaming. From fetching live tweets to processing and analyzing sentiment, we've maneuvered through the challenges and complexities of real-time data processing. With the ability to derive valuable insights from live social media streams, Spark Streaming empowers us to unravel the ever-changing landscape of public sentiment on Twitter. By mastering the art of sentiment analysis with Spark Streaming, we pave the way for informed decision-making and trend analysis in the fast-paced realm of social media.
Start harnessing the power of live Twitter sentiment analysis with Spark Streaming and unlock the potential of real-time insights.
Explore more about Apache Spark and Spark Streaming to deepen your understanding of real-time data processing and analytics.