Maximizing Java Performance: Client vs Server JVM Options
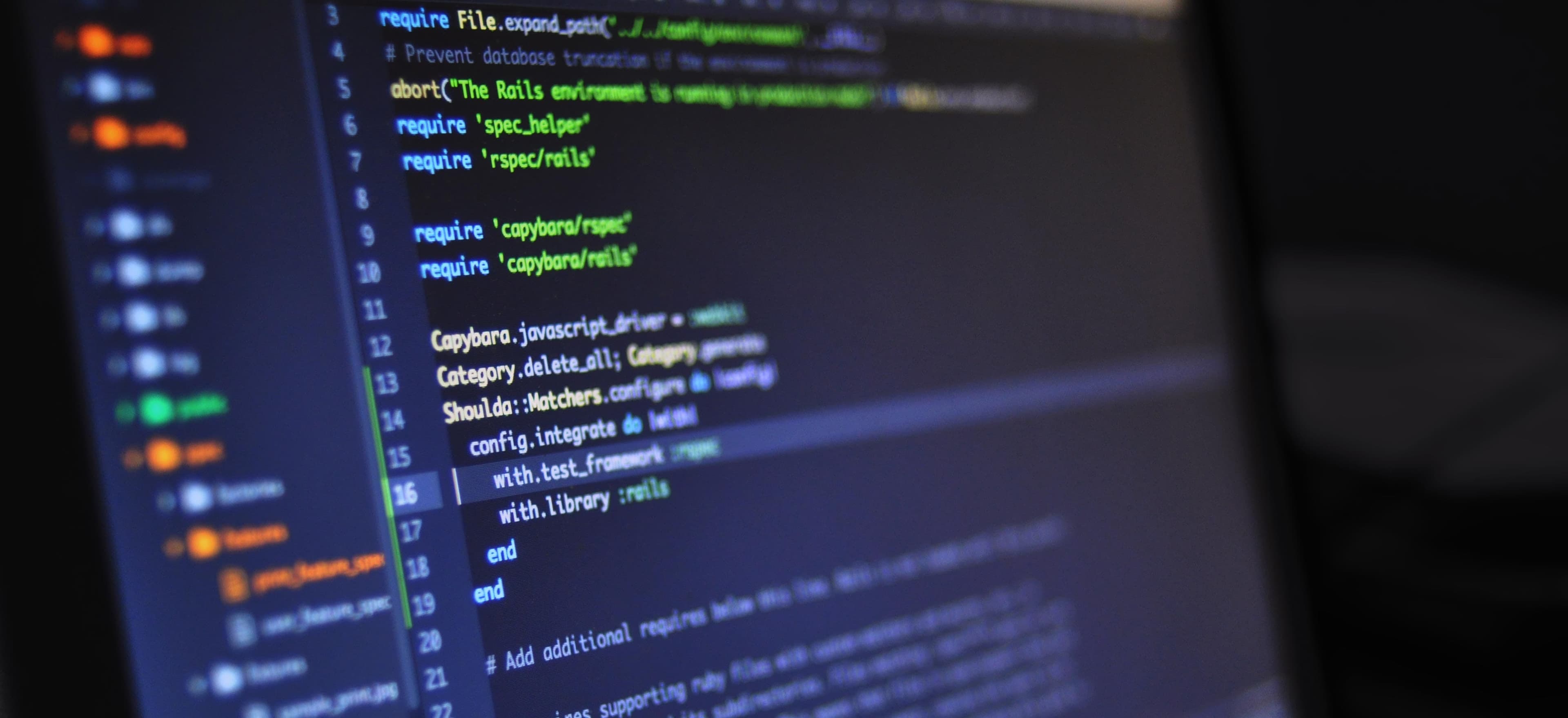
- Published on
Maximizing Java Performance: Client vs Server JVM Options
When it comes to getting the best performance out of your Java applications, understanding the differences between client and server JVM options is crucial. Java Virtual Machine (JVM) is the cornerstone of Java's platform independence, and choosing the right JVM option can significantly impact the performance of your application. In this post, we will delve into the differences between client and server JVM options, and how to leverage them to achieve optimal performance for your Java applications.
Understanding the Client and Server JVM Options
The client and server JVM options are part of Java's HotSpot Virtual Machine. These options affect the behavior of the Just-In-Time (JIT) compiler and the runtime optimizations performed by the JVM.
Client JVM
The client JVM is designed to optimize for faster startup time and lower memory footprint. It achieves this by minimizing the amount of time spent on initial compilation and emphasizing quick interpretation of code. This makes the client JVM suitable for desktop applications and scenarios where applications are short-lived or where responsiveness is critical.
Server JVM
On the other hand, the server JVM aims to maximize long-running application performance by investing more time in initial code compilation and employing aggressive runtime optimizations. It is tailored for server-side applications, such as enterprise systems and web applications, where sustained throughput and high performance are paramount.
Choosing the Right JVM Option
When to Use Client JVM
The client JVM is a good choice for desktop applications, command-line utilities, or scenarios where quick startup time and lower memory consumption are essential. For instance, applications with heavy user interaction, graphical user interfaces, or small-scale utilities can benefit from the client JVM's emphasis on fast startup and responsiveness. Additionally, the client JVM is suitable for development and testing environments, where rapid application iterations and quick feedback are crucial.
When to Use Server JVM
Conversely, the server JVM is well-suited for long-running server-side applications or backend systems that require sustained high performance and scalability. Enterprise applications, web servers, and large-scale data processing systems can leverage the server JVM's extensive optimizations to achieve better overall performance. When the goal is to prioritize throughput and efficient resource utilization over fast startup, the server JVM is the preferred choice.
JVM Option Selection in Practice
Code Examples
Let's consider a simplified example to illustrate the practical implications of JVM option selection.
Client JVM Example
public class ClientJVMExample {
public static void main(String[] args) {
// Perform application logic
}
}
In this hypothetical scenario, the ClientJVMExample
class represents a simple desktop application with a graphical user interface. The emphasis here is on quick startup time and responsive user interactions, making it a suitable candidate for the client JVM option.
Server JVM Example
public class ServerJVMExample {
public static void main(String[] args) {
// Perform server-side application logic
}
}
In contrast, the ServerJVMExample
class depicts a backend server application responsible for handling incoming requests and processing large volumes of data. Here, the focus is on sustained performance and efficient resource utilization, aligning with the characteristics of the server JVM option.
Optimization Considerations
When choosing between client and server JVM options, it's crucial to consider the specific requirements and usage patterns of your application. Assessing factors such as startup time, memory consumption, sustained throughput, and runtime performance characteristics can guide your decision towards the most suitable JVM option.
Leveraging JVM Tuning Options
In addition to selecting the appropriate JVM option, fine-tuning the JVM parameters can further enhance the performance of your Java applications. The JVM provides a range of tuning options that enable developers to customize memory management, garbage collection behavior, and runtime optimizations based on the specific needs of their applications.
Example of JVM Tuning
public class JVMConfiguration {
public static void main(String[] args) {
// Set JVM options for memory management and garbage collection
// -Xms2G : Set initial heap size to 2 gigabytes
// -Xmx4G : Set maximum heap size to 4 gigabytes
// -XX:NewRatio=3 : Set the ratio of young generation to old generation
// -XX:+UseG1GC : Enable the Garbage-First (G1) garbage collector
// -XX:MaxGCPauseMillis=200 : Set the maximum pause time goal for G1 GC
}
}
In this example, we showcase the use of JVM options to configure memory allocation and garbage collection behavior. By customizing these parameters, developers can optimize the JVM's memory management to align with the memory requirements of their applications, thereby improving overall performance.
Final Considerations
In conclusion, understanding the distinctions between client and server JVM options, and their respective implications on application performance, is essential for maximizing the potential of Java applications. By carefully evaluating the characteristics and requirements of your application, and leveraging JVM tuning options, you can optimize the JVM configuration to achieve the best performance outcomes. Whether it's prioritizing fast startup time and responsiveness with the client JVM, or aiming for sustained high performance and scalability with the server JVM, making informed decisions regarding JVM options is pivotal in maximizing Java performance.
In your Java development journey, consider exploring further insights on JVM optimization and Java performance tuning to deepen your understanding and proficiency in crafting high-performing Java applications.
By embracing the nuances of Java's JVM options, you are empowered to elevate the performance of your Java applications to unprecedented levels, ensuring they deliver exceptional user experiences and operational efficiency.
Make informed choices, optimize effectively, and unleash the full potential of Java performance with the right JVM options.
Checkout our other articles