Logging vs Debugging: Navigating the Minefield in Code
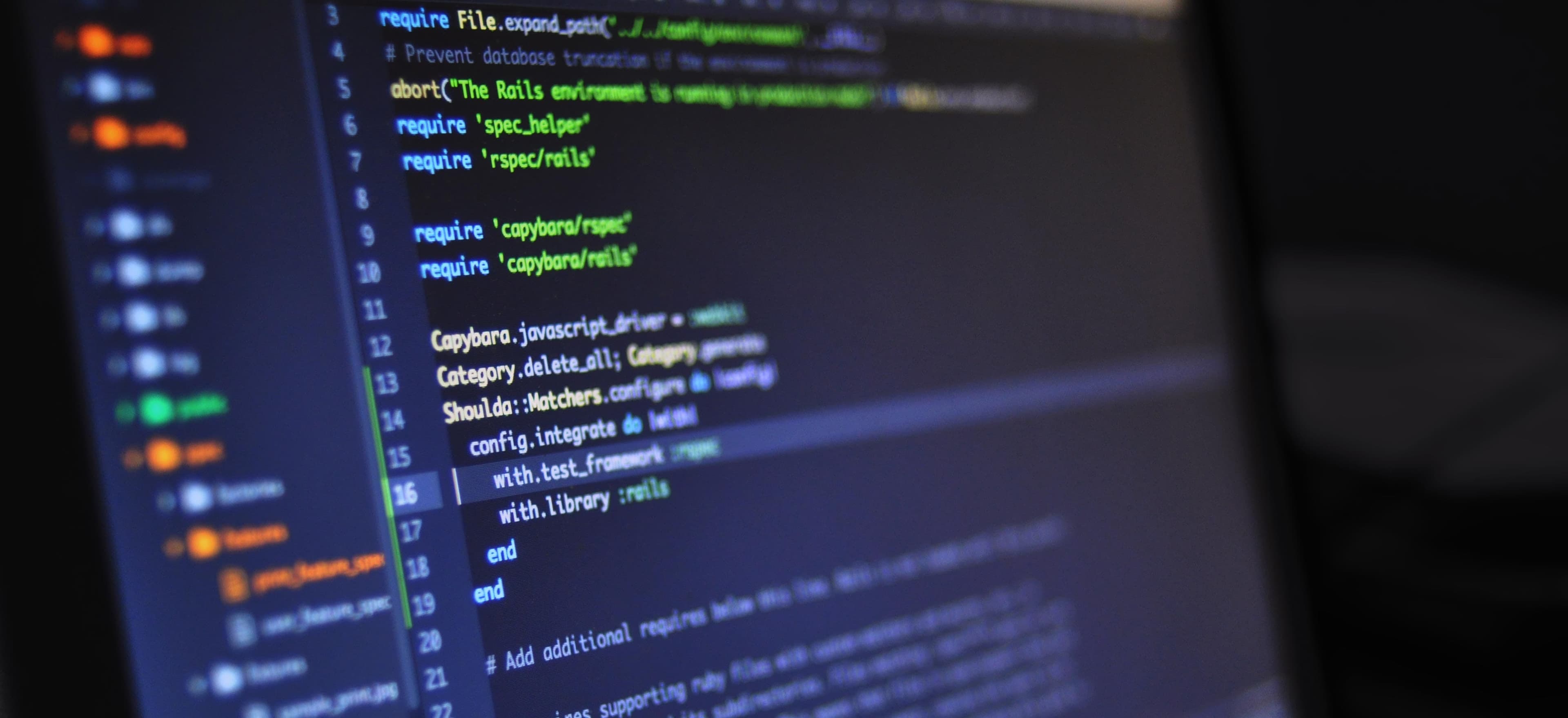
- Published on
Logging vs Debugging: Navigating the Minefield in Code
When it comes to maintaining and troubleshooting Java code, developers often rely on two key techniques: logging and debugging. These methods play a crucial role in identifying and resolving issues within applications. However, it's essential to understand the strengths and limitations of each approach to effectively utilize them in different scenarios. In this blog post, we'll delve into the nuances of logging and debugging in Java, and explore their respective uses, best practices, and considerations.
Logging in Java
Logging is the process of recording events, actions, and state information within an application. In Java, logging is commonly achieved using frameworks like Log4j or SLF4J. These frameworks provide a structured way to capture and store information during the execution of a program.
Why Logging Matters
Logging serves as a valuable tool for monitoring the behavior of an application, especially in production environments. It allows developers to track the flow of execution, identify errors, and gain insights into the inner workings of the code without disrupting the application's functionality.
Example: Logging a Message
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
public class ExampleClass {
private static final Logger logger = LoggerFactory.getLogger(ExampleClass.class);
public void performAction() {
logger.info("Executing performAction method");
// Perform the action
}
}
In this example, the performAction
method logs an informational message, providing visibility into the execution flow.
Best Practices for Logging
-
Use Log Levels Effectively: Choose the appropriate log level (e.g., DEBUG, INFO, WARN, ERROR) based on the significance of the message. This helps in filtering the log output based on severity.
-
Avoid Excessive Logging: Over-logging can lead to performance overhead and bloated log files. Focus on capturing relevant and actionable information.
-
Include Contextual Information: Enhance log messages with relevant context such as timestamps, thread IDs, and user-specific details to facilitate troubleshooting.
Debugging in Java
Debugging is the process of identifying and resolving defects or unexpected behavior in a program. In Java, developers leverage Integrated Development Environments (IDEs) like IntelliJ IDEA or Eclipse, which provide robust debugging capabilities.
Why Debugging Matters
Debugging enables developers to step through code, inspect variables, and analyze runtime behavior, making it indispensable for isolating and fixing complex issues. It's particularly effective for addressing critical bugs that impact the functionality of an application.
Example: Setting Breakpoints in IntelliJ IDEA
public class ExampleClass {
public static void main(String[] args) {
int a = 10;
int b = 20;
int result = a + b;
System.out.println("The result is: " + result);
}
}
By setting a breakpoint at the System.out.println
line, developers can pause the program's execution and examine the values of a
, b
, and result
.
Best Practices for Debugging
-
Use Conditional Breakpoints: Set breakpoints with conditions to halt the program only when specific criteria are met, reducing the need for manual intervention.
-
Inspect Variable Values: Leverage the debugging environment to inspect the state of variables and objects at different stages of execution, aiding in pinpointing issues.
-
Understand Stack Traces: Analyze stack traces to trace the origin of exceptions and understand the sequence of method calls leading to an error.
Choosing the Right Approach
While both logging and debugging are indispensable tools in a developer's arsenal, determining the appropriate approach depends on the nature of the issue and the context in which it arises.
When to Use Logging
Logging is especially effective for:
- Monitoring application behavior
- Capturing informative messages, warnings, and errors
- Tracking events and execution flow in production environments
Tip: Utilize contextual logging to capture relevant information for effective troubleshooting.
When to Use Debugging
Debugging shines in scenarios involving:
- Complex logic or algorithmic errors
- Runtime issues that require real-time inspection of variable states
- Reproducing and analyzing specific edge cases and user-interactions
Tip: Combine logging with debugging to gain comprehensive insights into code behavior during development and testing phases.
Key Takeaways
In the realm of Java development, both logging and debugging play critical roles in ensuring the reliability and functionality of applications. By understanding the distinctions between the two approaches and following best practices for each, developers can navigate the complexities of code troubleshooting with confidence and precision.
Remember, logging empowers you to observe and contextualize the behavior of your application, while debugging equips you to dissect and comprehend the intricacies of your code in action. Leveraging these tools effectively will not only enhance the quality of your software but also streamline your development and maintenance processes.
So, the next time you encounter a bug or need to understand how your software behaves in the wild, make a conscious choice between logging and debugging, and wield the right tool for the job. Happy coding!
Checkout our other articles