Java Mastery: Efficiently Processing Massive Files!
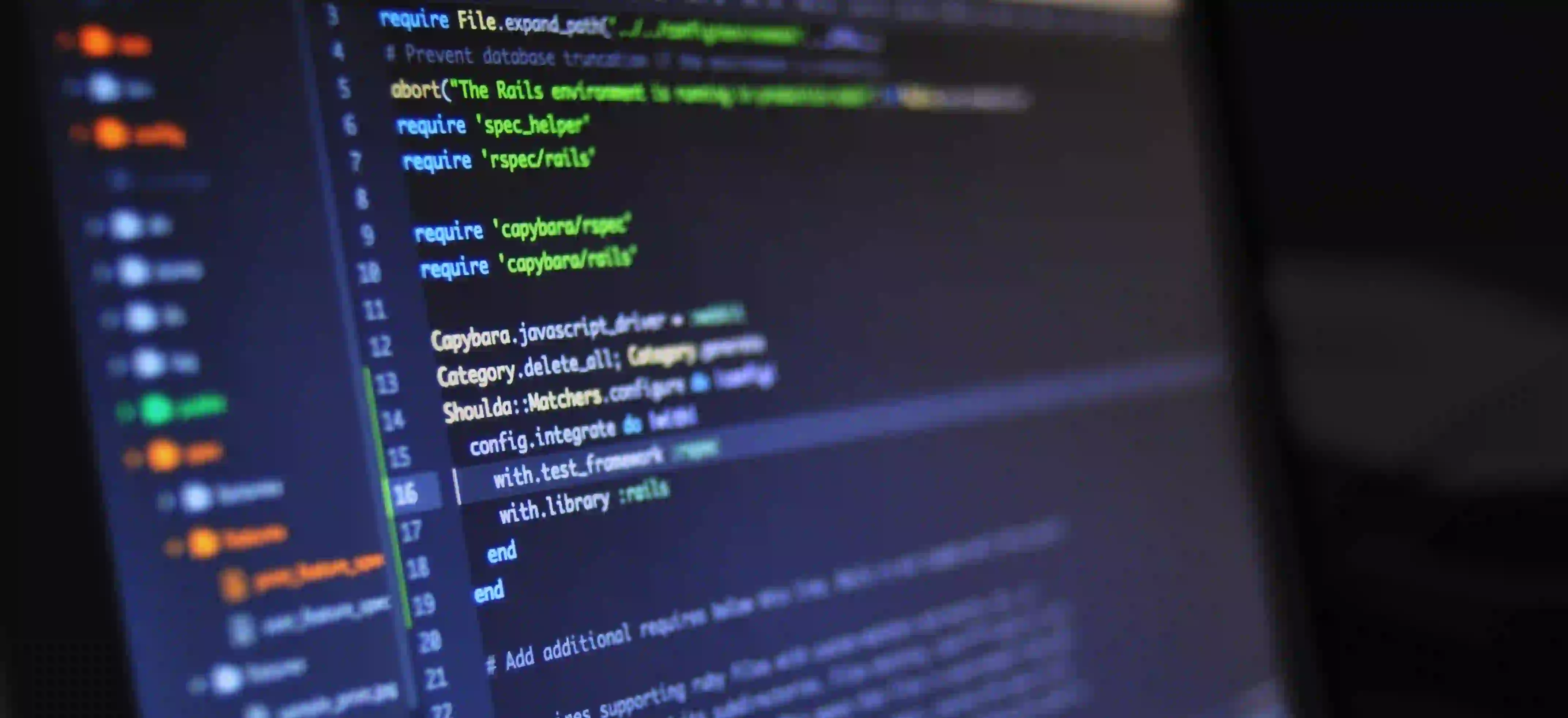
Mastering Java for Efficient Processing of Massive Files
As software engineers, we often encounter scenarios where we need to process massive files efficiently. In such cases, writing code that can handle large volumes of data while maintaining optimal performance becomes crucial. Java, with its robust features and libraries, is a popular choice for efficiently processing massive files. In this blog post, we will delve into the strategies, best practices, and Java features that enable us to tackle this challenge effectively.
Understanding the Challenge
Processing massive files presents several challenges, including memory management, processing speed, and scalability. When dealing with files that are too large to fit into memory, traditional approaches like reading the entire file into memory may not be feasible and can lead to performance bottlenecks.
Inefficient file processing can result in application crashes, slow performance, and increased resource consumption. Therefore, it is essential to employ strategies that allow for the seamless processing of massive files while optimizing memory usage and processing speed.
Leveraging Java's Features
Java offers several features and tools that are well-suited for efficiently processing massive files. Leveraging these features can significantly improve the performance and scalability of file processing operations.
Buffered I/O
Java provides the BufferedReader
and BufferedWriter
classes, which offer efficient reading and writing of large files by minimizing I/O operations. By using buffered I/O, we can reduce disk access and improve the overall performance of file processing operations.
try (BufferedReader br = new BufferedReader(new FileReader("largefile.txt"))) {
String line;
while ((line = br.readLine()) != null) {
// Process each line
}
} catch (IOException e) {
// Handle exception
}
The above code snippet demonstrates the use of BufferedReader
to efficiently read a large file line by line, minimizing I/O overhead.
Memory-Mapped Files
Java provides the java.nio
package, which includes support for memory-mapped files. Memory-mapped files allow for the efficient mapping of a file into memory, enabling seamless random access to its content. This approach is particularly useful for processing large files without loading the entire content into memory.
try (FileChannel channel = FileChannel.open(Paths.get("largefile.bin"), StandardOpenOption.READ)) {
MappedByteBuffer buffer = channel.map(FileChannel.MapMode.READ_ONLY, 0, channel.size());
// Process the content using the buffer
} catch (IOException e) {
// Handle exception
}
In the above code snippet, we use memory-mapped files to efficiently process the content of a large binary file without loading it entirely into memory.
Effective Memory Management
Efficient memory management is crucial when processing massive files in Java. Improper memory allocation and deallocation can lead to memory leaks and degraded performance. Java provides several mechanisms to optimize memory usage during file processing tasks.
Garbage Collection Optimization
Java's garbage collector plays a vital role in managing memory. By understanding the different garbage collection algorithms and tuning the garbage collection parameters, we can optimize memory usage and reduce the impact of garbage collection pauses on file processing performance.
Streaming APIs
Java 8 introduced the java.util.stream
package, which provides a powerful set of APIs for processing data in a functional style. When dealing with massive files, streaming APIs enable us to process data in a memory-efficient and parallel manner, leveraging the power of multi-core processors.
try (Stream<String> lines = Files.lines(Paths.get("massivefile.txt"))) {
lines.parallel() // Enable parallel processing
.filter(line -> line.startsWith("pattern"))
.forEach(System.out::println);
} catch (IOException e) {
// Handle exception
}
The above code snippet demonstrates the use of streaming APIs to process the content of a massive file in a parallel and memory-efficient manner.
Scalability and Performance Tuning
Scalability and performance are critical factors when processing massive files, especially in high-throughput and latency-sensitive applications. Java provides several tools and techniques for optimizing the performance of file processing operations.
Multithreading
Leveraging multithreading can significantly improve the performance of file processing tasks by parallelizing the workload across multiple threads. Java's java.util.concurrent
package offers a rich set of utilities for creating scalable and efficient multithreaded file processing solutions.
ExecutorService executor = Executors.newFixedThreadPool(4);
try (BufferedReader br = new BufferedReader(new FileReader("massivefile.txt"))) {
String line;
while ((line = br.readLine()) != null) {
executor.submit(() -> processLine(line));
}
} catch (IOException e) {
// Handle exception
} finally {
executor.shutdown();
}
In the above example, we use a fixed thread pool to parallelize the processing of lines from a massive file, improving overall throughput and performance.
Asynchronous I/O
Java's java.nio
package also provides support for asynchronous I/O operations, which can be beneficial for handling large volumes of data without blocking threads. By leveraging asynchronous I/O, we can achieve higher throughput and improved responsiveness in file processing tasks.
AsynchronousFileChannel channel = AsynchronousFileChannel.open(Paths.get("massivefile.txt"), StandardOpenOption.READ);
ByteBuffer buffer = ByteBuffer.allocate(1024);
channel.read(buffer, 0, buffer, new CompletionHandler<Integer, ByteBuffer>() {
@Override
public void completed(Integer result, ByteBuffer attachment) {
// Process the read data
}
@Override
public void failed(Throwable exc, ByteBuffer attachment) {
// Handle failure
}
});
In the above code snippet, we use asynchronous file channel to read data from a massive file without blocking the calling thread, thereby enhancing the performance of file processing operations.
In Conclusion, Here is What Matters
Efficiently processing massive files in Java requires a combination of effective techniques, leveraging the language's features, and optimizing memory usage, scalability, and performance. By employing strategies such as buffered I/O, memory-mapped files, efficient memory management, and performance tuning, Java developers can build robust and high-performance file processing solutions.
Mastering Java for efficient processing of massive files empowers developers to tackle real-world challenges related to handling large volumes of data with grace and precision, making Java a top choice for such endeavors.
By implementing these best practices and leveraging Java's powerful features, developers can build scalable, high-performance file processing solutions that ensure optimal resource utilization while efficiently handling massive files.
So, the next time you're faced with the challenge of processing massive files, remember to leverage Java's capabilities to their fullest potential and employ the best practices outlined in this blog post for efficient and effective file processing.
Start mastering Java for massive file handling and witness how it transforms your file processing capabilities for the better!
And for further reading, check out this insightful article on Java File I/O and this comprehensive guide on Java Performance Tuning.