Java 8 Guide: From Anonymous Classes to Lambda Magic!
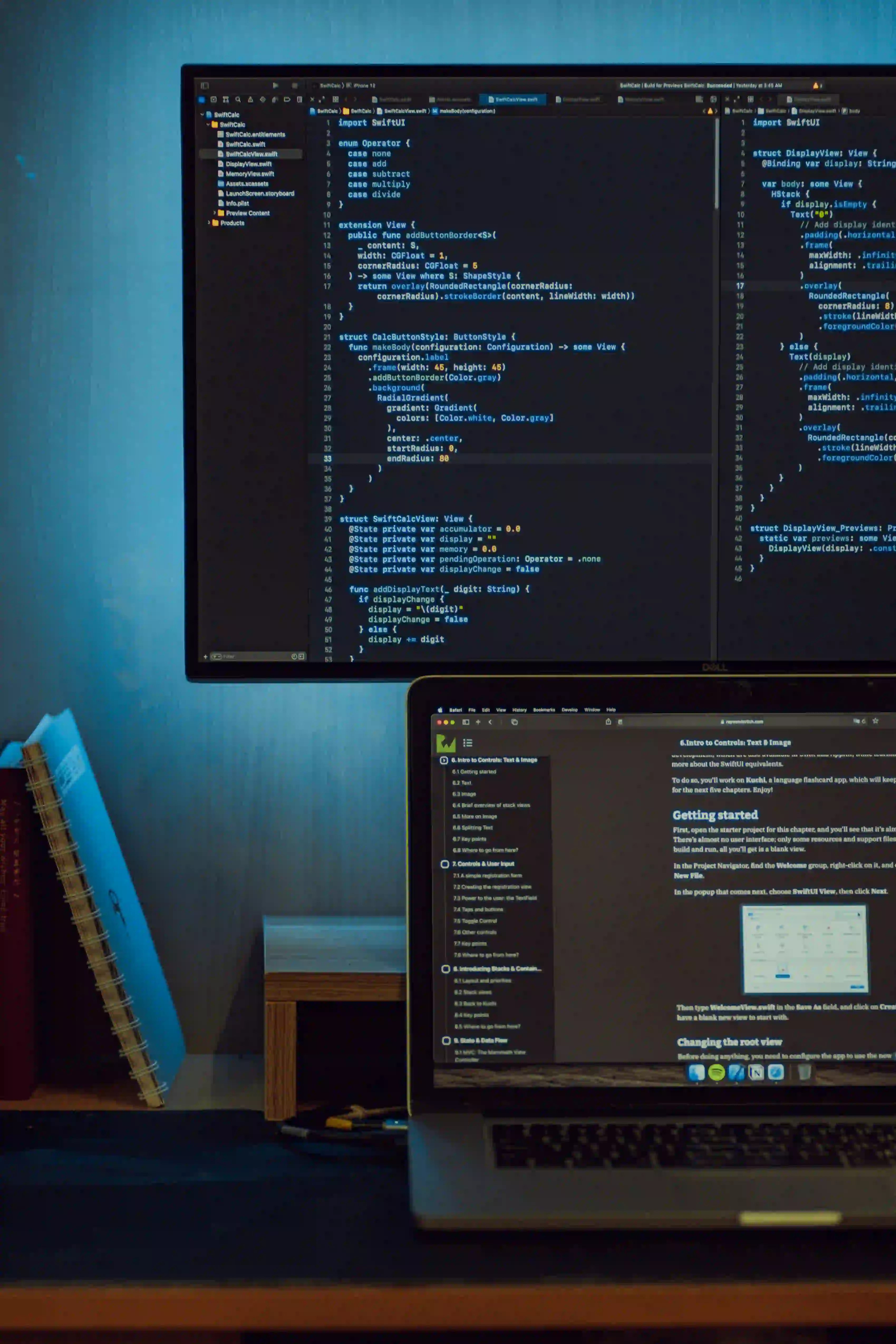
A Complete Guide to Java 8: Harnessing the Power of Lambdas
In the evolution of the Java programming language, Java 8 marks a significant milestone with the introduction of lambda expressions. This release brought a paradigm shift in the way Java developers write code, making it more concise and expressive. In this comprehensive guide, we'll explore the power of lambdas, functional interfaces, and stream API in Java 8.
Understanding the Need for Lambdas
Traditional Java programming often involved verbose syntax, especially when dealing with interfaces containing single abstract method (SAM), such as listeners and handlers. Anonymous inner classes were used to implement these functional interfaces, resulting in boilerplate code and reduced readability.
Lambdas offer a more elegant and succinct way to implement functional interfaces. They enable developers to write inline, concise, and more readable code, thereby improving the overall maintainability and understandability of the codebase.
Let's dive into some examples to understand the transition from anonymous classes to lambdas.
Anonymous Class Implementation
Consider the following example of implementing a simple Runnable
using an anonymous class:
public class AnonymousClassExample {
public static void main(String[] args) {
Runnable runnable = new Runnable() {
@Override
public void run() {
System.out.println("Hello from anonymous class!");
}
};
new Thread(runnable).start();
}
}
The anonymous class syntax requires a lot of boilerplate code, making the intention less clear.
Lambda Expression Implementation
Now, let's refactor the same example using a lambda expression:
public class LambdaExample {
public static void main(String[] args) {
Runnable runnable = () -> System.out.println("Hello from lambda!");
new Thread(runnable).start();
}
}
The lambda expression simplifies the code significantly, making the intent explicit and the code more concise.
The Anatomy of Lambda Expressions
A lambda expression is characterized by the following syntax:
(parameters) -> expression
or
(parameters) -> { statements; }
Features of Lambda Expressions
-
Conciseness: Lambdas eliminate the need for excessive boilerplate code, making the code more readable and maintainable.
-
Functional Interface Compatibility: Lambdas can be used only with functional interfaces, which are interfaces with a single abstract method. These interfaces provide a clear contract for lambda usage.
-
Capturing Variables: Lambdas can capture (or close over) variables from their enclosing scope. These variables should be effectively final or explicitly marked as
final
.
Functional Interfaces
Java 8 introduced the @FunctionalInterface
annotation to explicitly mark interfaces as functional interfaces. This annotation ensures that the interface contains only one abstract method, preventing accidental addition of multiple abstract methods in the future.
Example of a Functional Interface
Consider a simple functional interface representing a unary operator:
@FunctionalInterface
interface UnaryOperator<T> {
T apply(T operand);
}
By marking the interface with @FunctionalInterface
, the compiler will flag any attempt to add more than one abstract method in the interface, ensuring its single abstract method contract.
Leveraging the Stream API
In addition to lambdas, Java 8 introduced the Stream API, which revolutionized the way developers work with collections. Streams provide a declarative approach to perform operations on a collection, allowing for concise and expressive code.
Working with Streams and Lambdas
Let's consider an example where we have a list of integers and we want to filter the even numbers and then calculate their squares using streams and lambdas.
import java.util.Arrays;
import java.util.List;
public class StreamExample {
public static void main(String[] args) {
List<Integer> numbers = Arrays.asList(1, 2, 3, 4, 5, 6, 7, 8, 9, 10);
int sumOfEvenSquares = numbers.stream()
.filter(n -> n % 2 == 0) // Filter even numbers
.map(n -> n * n) // Square each number
.reduce(0, Integer::sum); // Sum the squares
System.out.println("Sum of squares of even numbers: " + sumOfEvenSquares);
}
}
In this example, the use of lambdas and the stream API enables us to express the operations in a clear and concise manner, focusing on the "what" instead of the "how".
A Final Look
Java 8's introduction of lambda expressions, functional interfaces, and the Stream API has transformed the way Java developers write code. By embracing these features, developers can write more expressive, concise, and maintainable code. Understanding and leveraging these features is essential for staying current and proficient in Java development.
In conclusion, the transition from anonymous classes to lambdas has made Java code more readable, maintainable, and efficient. The paradigm shift introduced by Java 8 has opened up new avenues for developers to write elegant and expressive code.
So, embrace the power of lambdas, explore the versatility of functional interfaces, and harness the expressiveness of the Stream API to elevate your Java programming skills to the next level!
Start coding with lambdas today and unlock the true potential of Java 8!