Monitoring JDBC Connection Pool Metrics with Micrometer
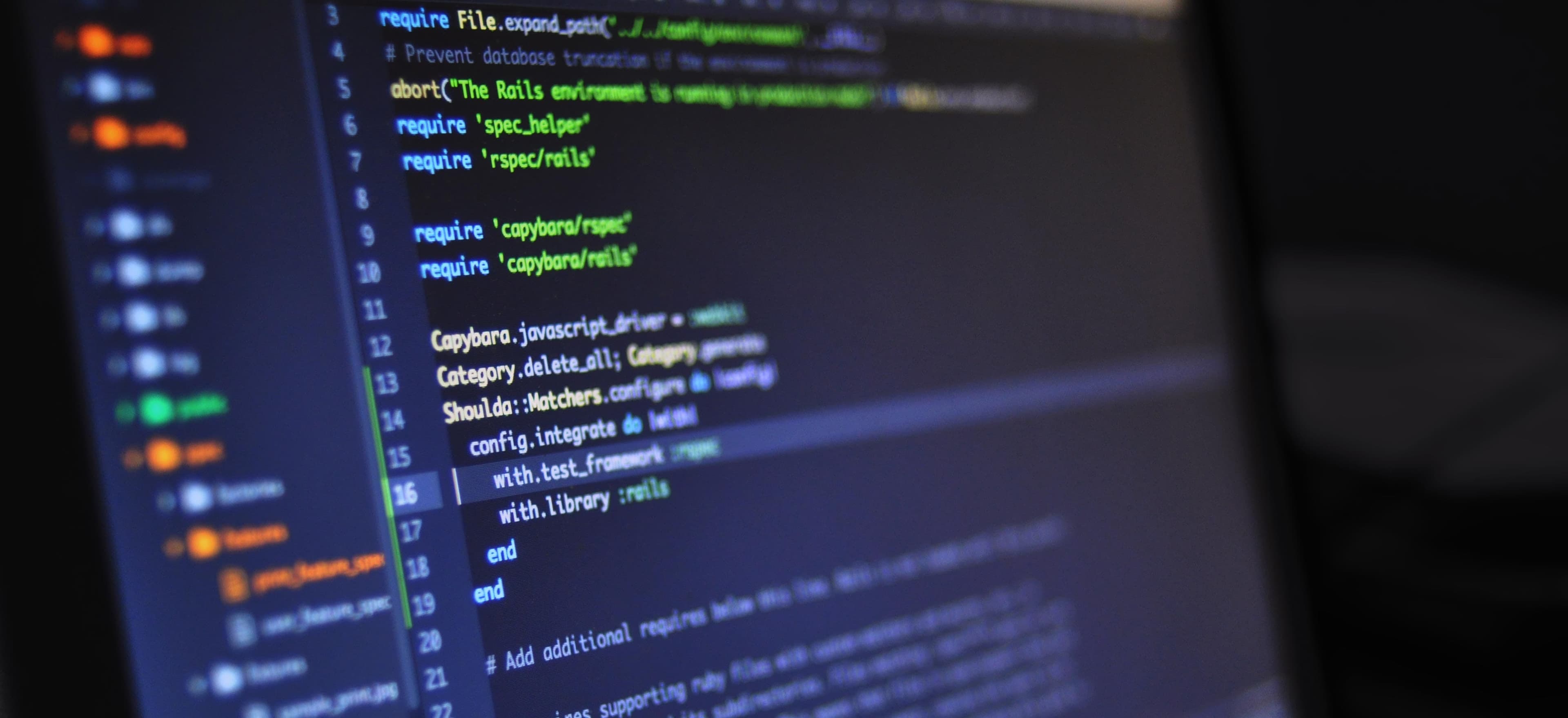
- Published on
Monitoring JDBC Connection Pool Metrics with Micrometer
In a Java application, managing database connections efficiently is crucial for maintaining optimal performance. JDBC connection pools are widely used to achieve this goal, and monitoring their metrics can provide valuable insights into the health and performance of the database interactions. In this blog post, we will explore how to monitor JDBC connection pool metrics using Micrometer, a powerful instrumentation library for Java applications.
What is Micrometer?
Micrometer is a metrics instrumentation library that provides a simple and consistent API for monitoring the performance and health of applications. It supports various monitoring systems such as Prometheus, Graphite, and Datadog, allowing you to visualize and analyze metrics in real-time.
Why Monitor JDBC Connection Pool Metrics?
Monitoring JDBC connection pool metrics is essential for identifying potential performance bottlenecks, detecting connection leaks, and optimizing resource utilization. By tracking metrics such as active connections, idle connections, and connection acquisition time, you can gain insights into the behavior of the connection pool and take proactive measures to improve the overall database performance.
Integrating Micrometer with JDBC Connection Pool
To start monitoring JDBC connection pool metrics with Micrometer, we need to integrate Micrometer with our chosen connection pool library. In this example, we will use HikariCP as the JDBC connection pool implementation.
First, we need to add the Micrometer and HikariCP dependencies to our project:
<dependency>
<groupId>io.micrometer</groupId>
<artifactId>micrometer-core</artifactId>
<version>1.7.0</version>
</dependency>
<dependency>
<groupId>com.zaxxer</groupId>
<artifactId>HikariCP</artifactId>
<version>4.0.3</version>
</dependency>
Once the dependencies are added, we can configure Micrometer to monitor the JDBC connection pool metrics. Micrometer provides a convenient way to bind HikariCP metrics to the registry.
import com.zaxxer.hikari.HikariDataSource;
import io.micrometer.core.instrument.Clock;
import io.micrometer.core.instrument.Metrics;
import io.micrometer.core.instrument.binder.hikaricp.HikariCpMetricsTracker;
public class Database {
public HikariDataSource initializeDataSource() {
HikariDataSource dataSource = new HikariDataSource();
// Configure the data source
// Bind HikariCP metrics to the Micrometer registry
HikariCpMetricsTracker.monitor(
HikariCpMetricsTracker.MetricsAwareHikariCPProxyFactory.create(dataSource, Clock.SYSTEM));
return dataSource;
}
}
In the code snippet above, we initialize the HikariCP data source and bind the HikariCP metrics to the Micrometer registry using the HikariCpMetricsTracker
class.
Monitoring JDBC Connection Pool Metrics
Once the integration is in place, we can start monitoring JDBC connection pool metrics using Micrometer. Micrometer provides a rich set of predefined metrics for monitoring HikariCP connection pools, including metrics for total connections, active connections, idle connections, connection acquisition time, and more.
import io.micrometer.core.instrument.MeterRegistry;
public class DatabaseService {
private final MeterRegistry meterRegistry;
private final HikariDataSource dataSource;
public DatabaseService(MeterRegistry meterRegistry, HikariDataSource dataSource) {
this.meterRegistry = meterRegistry;
this.dataSource = dataSource;
monitorConnectionPoolMetrics();
}
private void monitorConnectionPoolMetrics() {
meterRegistry.gauge("connection.pool.active.connections",
dataSource, HikariCpMetricsTracker.getActiveConnections());
meterRegistry.gauge("connection.pool.idle.connections",
dataSource, HikariCpMetricsTracker.getIdleConnections());
meterRegistry.timer("connection.pool.acquire.time",
DataSource::getConnection, HikariCpMetricsTracker.getAcquireConnection());
// Add more metrics as per your monitoring requirements
}
}
In the code above, we create a DatabaseService
class that takes a MeterRegistry
and the HikariCP data source as constructor parameters. We then use the meterRegistry
to define gauges and timers for monitoring various connection pool metrics.
Visualizing JDBC Connection Pool Metrics
With the metrics being recorded by Micrometer, we can now visualize and analyze the JDBC connection pool metrics using monitoring systems such as Prometheus, Graphite, or Datadog. By setting up dashboards and alerts based on these metrics, we can gain real-time insights into the performance and health of the database connections.
In Conclusion, Here is What Matters
In this blog post, we have demonstrated how to monitor JDBC connection pool metrics using Micrometer in a Java application. By integrating Micrometer with the JDBC connection pool library and defining custom metrics, we can gain valuable insights into the behavior of the connection pool and make informed decisions to optimize database performance.
Monitoring JDBC connection pool metrics is a critical aspect of maintaining a healthy and performant database interaction layer. With Micrometer, you can easily gain visibility into the inner workings of your JDBC connection pool and take proactive measures to ensure optimal performance.
Incorporating Micrometer for monitoring JDBC connection pool metrics is a powerful way to enhance the observability of your Java applications, ultimately leading to improved reliability and performance.
To learn more about JDBC, check out this comprehensive guide on Java Database Connectivity (JDBC). Additionally, for a deeper understanding of Micrometer, visit the official Micrometer documentation.
Start monitoring your JDBC connection pool metrics with Micrometer today to unlock valuable insights and optimize the performance of your Java applications. Happy monitoring!